FMList
#include <fmlist.h>
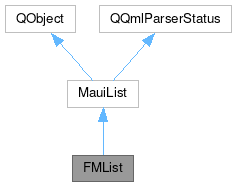
Public Types | |
enum | FILTER : uint_fast8_t { AUDIO = FMStatic::FILTER_TYPE::AUDIO , VIDEO = FMStatic::FILTER_TYPE::VIDEO , TEXT = FMStatic::FILTER_TYPE::TEXT , IMAGE = FMStatic::FILTER_TYPE::IMAGE , DOCUMENT = FMStatic::FILTER_TYPE::DOCUMENT , COMPRESSED = FMStatic::FILTER_TYPE::COMPRESSED , FONT = FMStatic::FILTER_TYPE::FONT , NONE = FMStatic::FILTER_TYPE::NONE } |
enum | PATHTYPE : uint_fast8_t { PLACES_PATH = FMStatic::PATHTYPE_KEY::PLACES_PATH , FISH_PATH = FMStatic::PATHTYPE_KEY::FISH_PATH , MTP_PATH = FMStatic::PATHTYPE_KEY::MTP_PATH , REMOTE_PATH = FMStatic::PATHTYPE_KEY::REMOTE_PATH , DRIVES_PATH = FMStatic::PATHTYPE_KEY::DRIVES_PATH , REMOVABLE_PATH = FMStatic::PATHTYPE_KEY::REMOVABLE_PATH , TAGS_PATH = FMStatic::PATHTYPE_KEY::TAGS_PATH , BOOKMARKS_PATH = FMStatic::PATHTYPE_KEY::BOOKMARKS_PATH , APPS_PATH = FMStatic::PATHTYPE_KEY::APPS_PATH , TRASH_PATH = FMStatic::PATHTYPE_KEY::TRASH_PATH , CLOUD_PATH = FMStatic::PATHTYPE_KEY::CLOUD_PATH , QUICK_PATH = FMStatic::PATHTYPE_KEY::QUICK_PATH , OTHER_PATH = FMStatic::PATHTYPE_KEY::OTHER_PATH } |
enum | SORTBY : uint_fast8_t { SIZE = FMH::MODEL_KEY::SIZE , MODIFIED = FMH::MODEL_KEY::MODIFIED , DATE = FMH::MODEL_KEY::DATE , LABEL = FMH::MODEL_KEY::LABEL , MIME = FMH::MODEL_KEY::MIME , ADDDATE = FMH::MODEL_KEY::ADDDATE } |
enum | VIEW_TYPE : uint_fast8_t { ICON_VIEW , LIST_VIEW } |
![]() | |
typedef | QObjectList |
Properties | |
QML_ELEMENTbool | autoLoad |
int | cloudDepth = 1 |
QStringList | filters = {} |
FMList::FILTER | filterType = FMList::FILTER::NONE |
bool | foldersFirst = false |
bool | hidden = false |
bool | mergeFilters |
bool | onlyDirs = false |
QUrl | parentPath |
QString | path |
QString | pathName = QString() |
FMList::PATHTYPE | pathType = FMList::PATHTYPE::PLACES_PATH |
bool | readOnly |
FMList::SORTBY | sortBy |
PathStatus | status |
![]() | |
QML_ANONYMOUSint | count |
![]() | |
objectName | |
Signals | |
void | autoLoadChanged () |
void | cloudDepthChanged () |
void | filtersChanged () |
void | filterTypeChanged () |
void | foldersFirstChanged () |
void | hiddenChanged () |
void | mergeFiltersChanged () |
void | onlyDirsChanged () |
void | pathChanged () |
void | pathNameChanged () |
void | pathTypeChanged () |
void | progress (int percent) |
void | readOnlyChanged () |
void | sortByChanged () |
void | statusChanged () |
void | warning (QString message) |
![]() | |
void | countChanged () |
void | itemMoved (int index, int to) |
void | postItemAppended () |
void | postItemRemoved () |
void | postListChanged () |
void | preItemAppended () |
void | preItemAppendedAt (int index) |
void | preItemRemoved (int index) |
void | preItemsAppended (uint count) |
void | preListChanged () |
void | updateModel (int index, QVector< int > roles) |
Public Slots | |
bool | clipboardHasContent () const |
void | copyInto (const QStringList &urls) |
void | createDir (const QString &name) |
void | createFile (const QString &name) |
void | createSymlink (const QString &url) |
void | cutInto (const QStringList &urls) |
int | indexOfFile (const QString &url) |
int | indexOfName (const QString &query) |
void | moveToTrash (const QStringList &urls) |
void | paste () |
const QUrl | posteriorPath () |
const QUrl | previousPath () |
void | refresh () |
void | remove (const int &index) |
void | removeFiles (const QStringList &urls) |
void | renameFile (const QString &url, const QString &newName) |
void | search (const QString &query, bool recursive=true) |
void | setDirIcon (const int &index, const QString &iconName) |
![]() | |
QVariantMap | get (const int &index) const |
Public Member Functions | |
FMList (QObject *parent=nullptr) | |
bool | getAutoLoad () const |
int | getCloudDepth () const |
QStringList | getFilters () const |
FMList::FILTER | getFilterType () const |
bool | getFoldersFirst () const |
bool | getHidden () const |
bool | getOnlyDirs () const |
const QUrl | getParentPath () |
QString | getPath () const |
QString | getPathName () const |
FMList::PATHTYPE | getPathType () const |
FMList::SORTBY | getSortBy () const |
PathStatus | getStatus () const |
const FMH::MODEL_LIST & | items () const final override |
bool | mergeFilters () const |
bool | readOnly () const |
void | resetFilters () |
void | resetFilterType () |
void | setAutoLoad (bool value) |
void | setCloudDepth (const int &value) |
void | setFilters (const QStringList &filters) |
void | setFilterType (const FMList::FILTER &type) |
void | setFoldersFirst (const bool &value) |
void | setHidden (const bool &state) |
void | setMergeFilters (bool value) |
void | setOnlyDirs (const bool &state) |
void | setPath (const QString &path) |
void | setReadOnly (bool value) |
void | setSortBy (const FMList::SORTBY &key) |
![]() | |
MauiList (QObject *parent=nullptr) | |
virtual void | classBegin () override |
FMH::MODEL | getItem (const int &index) const |
virtual void | modelHooked () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | exists (const FMH::MODEL_KEY &key, const QString &value) const |
int | indexOf (const FMH::MODEL_KEY &key, const QString &value) const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The FMList class Model for listing the file system files and directories and perform relevant actions upon it.
Member Enumeration Documentation
◆ FILTER
enum FMList::FILTER : uint_fast8_t |
The possible values to filter the a location content by a mime-type.
◆ PATHTYPE
enum FMList::PATHTYPE : uint_fast8_t |
◆ SORTBY
enum FMList::SORTBY : uint_fast8_t |
The possible values to sort the location contents.
◆ VIEW_TYPE
enum FMList::VIEW_TYPE : uint_fast8_t |
The possible view types for listing the entries in the FileBrowser visual control.
Enumerator | |
---|---|
ICON_VIEW | Display the file system entries in a grid view. |
LIST_VIEW | Display the file system entries in a list, with more information details visible. |
Property Documentation
◆ autoLoad
|
readwrite |
◆ cloudDepth
|
readwrite |
When the location if a remote cloud directory, this allows to define the depth of the levels for listing the contents.
By default this is set to 1
, which will only lists the entries in the current location, a bigger depth will start listing sub-directories too.
◆ filters
|
readwrite |
◆ filterType
|
readwrite |
◆ foldersFirst
|
readwrite |
◆ hidden
|
readwrite |
◆ mergeFilters
|
readwrite |
Merge the name filters and mimetype filters together for filtering the requested location contentent's.
The filters
and filterType
are the properties to be merged. If this is set to false, then the filters
property will have priority over the filterType
one, unless it is empty.
◆ onlyDirs
|
readwrite |
◆ parentPath
|
read |
◆ path
|
readwrite |
The URL to location path to proceed listing all of its file entries.
There is support for multiple type of location depending on the scheme, for example local file system uses file://
, while you can browser networks using ftp://
or fish://
. Support for those locations depends on KIO and its slaves - to know more about it read the KIO slaves documentation.
◆ pathName
◆ pathType
|
read |
◆ readOnly
|
readwrite |
◆ sortBy
|
readwrite |
The sorting value.
By default this is set to SORTBY::MODIFIED
.
◆ status
|
read |
Constructor & Destructor Documentation
◆ FMList()
FMList::FMList | ( | QObject * | parent = nullptr | ) |
Member Function Documentation
◆ clipboardHasContent
|
slot |
Whether the clipboard has a supported type of content.
- Returns
- whether the clipboard content is a supported file URL or a text or image raw data.
Definition at line 599 of file fmlist.cpp.
◆ copyInto
|
slot |
Copy a list of file URLs into the current directory.
- Parameters
-
urls list of files
Definition at line 614 of file fmlist.cpp.
◆ createDir
|
slot |
Create a new directory within the current directory.
- Parameters
-
name the name of the directory
Definition at line 467 of file fmlist.cpp.
◆ createFile
|
slot |
Create a new file.
- Note
- To create a custom file, please supply with the correct suffix.
- Parameters
-
name the name of the new file, for example new.txt
Definition at line 481 of file fmlist.cpp.
◆ createSymlink
|
slot |
Create a symbolic link to the given URL in the current location.
- Parameters
-
url the file URL to create the link from
Definition at line 513 of file fmlist.cpp.
◆ cutInto
|
slot |
Cut/move a list of file URLs to the current directory.
- Parameters
-
urls list of files
Definition at line 622 of file fmlist.cpp.
◆ getAutoLoad()
bool FMList::getAutoLoad | ( | ) | const |
Definition at line 839 of file fmlist.cpp.
◆ getCloudDepth()
int FMList::getCloudDepth | ( | ) | const |
Definition at line 790 of file fmlist.cpp.
◆ getFilters()
QStringList FMList::getFilters | ( | ) | const |
Definition at line 393 of file fmlist.cpp.
◆ getFilterType()
FMList::FILTER FMList::getFilterType | ( | ) | const |
Definition at line 413 of file fmlist.cpp.
◆ getFoldersFirst()
bool FMList::getFoldersFirst | ( | ) | const |
Definition at line 677 of file fmlist.cpp.
◆ getHidden()
bool FMList::getHidden | ( | ) | const |
Definition at line 433 of file fmlist.cpp.
◆ getOnlyDirs()
bool FMList::getOnlyDirs | ( | ) | const |
Definition at line 447 of file fmlist.cpp.
◆ getParentPath()
const QUrl FMList::getParentPath | ( | ) |
Definition at line 646 of file fmlist.cpp.
◆ getPath()
QString FMList::getPath | ( | ) | const |
Definition at line 331 of file fmlist.cpp.
◆ getPathName()
QString FMList::getPathName | ( | ) | const |
Definition at line 326 of file fmlist.cpp.
◆ getPathType()
FMList::PATHTYPE FMList::getPathType | ( | ) | const |
Definition at line 388 of file fmlist.cpp.
◆ getSortBy()
FMList::SORTBY FMList::getSortBy | ( | ) | const |
Definition at line 246 of file fmlist.cpp.
◆ getStatus()
PathStatus FMList::getStatus | ( | ) | const |
Definition at line 805 of file fmlist.cpp.
◆ indexOfFile
|
slot |
Definition at line 869 of file fmlist.cpp.
◆ indexOfName
|
slot |
Given a file-name-query, check if it exists in the current location.
- Parameters
-
index the index of the found file entry otherwise -1
Definition at line 827 of file fmlist.cpp.
◆ items()
|
finaloverridevirtual |
◆ mergeFilters()
bool FMList::mergeFilters | ( | ) | const |
Definition at line 231 of file fmlist.cpp.
◆ moveToTrash
|
slot |
Remove and move to the trash the provided set of file URLs.
- Parameters
-
urls the list of file URLS to be removed
Definition at line 497 of file fmlist.cpp.
◆ paste
|
slot |
Handle the paste action.
This allows to quickly paste into the current location any file URL in the clipboard, and raw image data and text snippets into a new file.
Definition at line 559 of file fmlist.cpp.
◆ posteriorPath
|
slot |
The immediate posterior path location that was navigated.
- Returns
- the path URL location
Definition at line 657 of file fmlist.cpp.
◆ previousPath
|
slot |
The immediate previous path location that was navigated.
- Returns
- the path URL location
Definition at line 667 of file fmlist.cpp.
◆ progress
|
signal |
Emitted while the file listing is still in progress.
- Parameters
-
percent the loading progress - it goes from 0 to 100.
◆ readOnly()
bool FMList::readOnly | ( | ) | const |
Definition at line 855 of file fmlist.cpp.
◆ refresh
|
slot |
Refresh the model for new changes.
h content listing ill be regenerated.
Definition at line 462 of file fmlist.cpp.
◆ remove
|
slot |
Remove an item from the model, this does not remove the file from the file system.
- Parameters
-
index the index position of the file entry
Definition at line 816 of file fmlist.cpp.
◆ removeFiles
|
slot |
Completely remove the set of file URLs provided.
This action can not be undone
- Parameters
-
urls the list of file URLS to be removed
Definition at line 505 of file fmlist.cpp.
◆ renameFile
Rename a file with from a given URL to the a new name provided.
- Parameters
-
url the file URL to be renamed newName the new name for the file
Definition at line 489 of file fmlist.cpp.
◆ resetFilters()
void FMList::resetFilters | ( | ) |
Definition at line 408 of file fmlist.cpp.
◆ resetFilterType()
void FMList::resetFilterType | ( | ) |
Definition at line 428 of file fmlist.cpp.
◆ search
|
slot |
Start a search - starting from the current location - for a given name query.
- Parameters
-
query the search query name recursive whether the search should be recursive and look into the subsequent sub-directories structure. By default this is set to false
.
Definition at line 724 of file fmlist.cpp.
◆ setAutoLoad()
void FMList::setAutoLoad | ( | bool | value | ) |
Definition at line 844 of file fmlist.cpp.
◆ setCloudDepth()
void FMList::setCloudDepth | ( | const int & | value | ) |
Definition at line 795 of file fmlist.cpp.
◆ setDirIcon
|
slot |
Changes the icon of a directory by making use of the directory config file.
- Parameters
-
index the index position of the directory in the model iconName then name of the new icon
Definition at line 630 of file fmlist.cpp.
◆ setFilters()
void FMList::setFilters | ( | const QStringList & | filters | ) |
Definition at line 398 of file fmlist.cpp.
◆ setFilterType()
void FMList::setFilterType | ( | const FMList::FILTER & | type | ) |
Definition at line 418 of file fmlist.cpp.
◆ setFoldersFirst()
void FMList::setFoldersFirst | ( | const bool & | value | ) |
Definition at line 682 of file fmlist.cpp.
◆ setHidden()
void FMList::setHidden | ( | const bool & | state | ) |
Definition at line 438 of file fmlist.cpp.
◆ setMergeFilters()
void FMList::setMergeFilters | ( | bool | value | ) |
Definition at line 222 of file fmlist.cpp.
◆ setOnlyDirs()
void FMList::setOnlyDirs | ( | const bool & | state | ) |
Definition at line 452 of file fmlist.cpp.
◆ setPath()
void FMList::setPath | ( | const QString & | path | ) |
Definition at line 336 of file fmlist.cpp.
◆ setReadOnly()
void FMList::setReadOnly | ( | bool | value | ) |
Definition at line 860 of file fmlist.cpp.
◆ setSortBy()
void FMList::setSortBy | ( | const FMList::SORTBY & | key | ) |
Definition at line 251 of file fmlist.cpp.
◆ warning
|
signal |
Emitted when the listing process has any error message that needs to be notified.
- Parameters
-
message the warning message text
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:50:40 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.