FileBrowser
Properties | |
alias | browser |
FBFMList | currentFMList |
MauiBaseModel | currentFMModel |
int | currentIndex |
alias | currentPath |
QtObject | currentView |
alias | dialog |
alias | dropArea |
alias | gridItemSize |
bool | isSearchView |
alias | listItemSize |
alias | readOnly |
MauiSelectionBar | selectionBar |
alias | selectionMode |
alias | settings |
alias | view |
Signals | |
void | areaClicked (var mouse) |
void | itemClicked (int index) |
void | itemDoubleClicked (int index) |
void | itemLeftEmblemClicked (int index) |
void | itemRightClicked (int index) |
void | keyPress (var event) |
void | rightClicked () |
void | urlsDropped (var urls) |
Detailed Description
This control displays the file system entries in a given directory, allows subsequent browsing of the file hierarchy and exposes basic file handling functionality.
This controls inherits from MauiKit Page, to checkout its inherited properties refer to the docs.
- See also
- MauiKit::Page
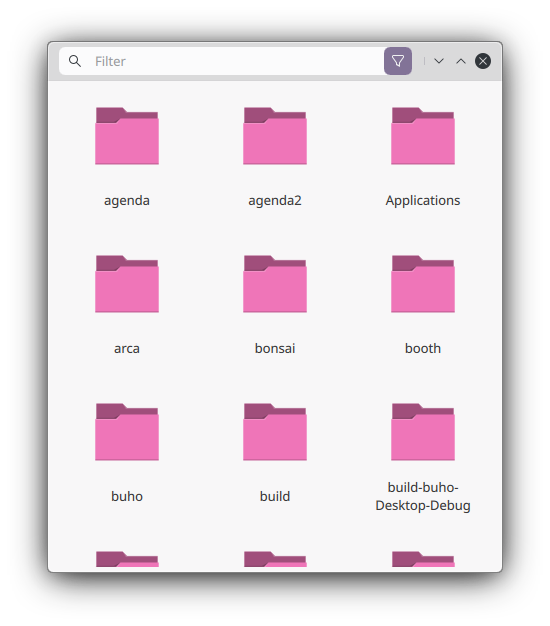
Features
This control exposes a series of convenient properties and functions for filtering and sorting, for creating new entries, perform searches, and modify the entries.
There are two different possible ways to display the contents: Grid and List, using the settings.viewType
property.
- See also
- FMList::VIEW_TYPE
More browsing properties can be tweaked via the exposed settings
alias property.
- See also
- settings
Some basic file item actions are implemented by default, like copy, cut, rename and remove, and exposed as methods.
- See also
- copy
- cut
- paste
- remove
- Note
- This component functionality can be easily expanded to be more feature rich, see for example the Maui Index application.
This control allows to perform recursive searches, or simple filtering of elements in the current location by using the search bar. Besides these two, the user can quickly start fly-typing within the browser, to jump quickly to a matching entry.
Shortcuts
This control supports multiple keyboard shortcuts, for selecting multiple elements, creating new entries, copying, deleting and renaming files.
The supported keyboard shortcuts are:
Ctrl + N
Create a new entryCtrl + F
Open the search barF5
Refresh/reload the contentF2
Rename an entryCtrl + A
Select all the entriesCtrl + N
Create a new entryCtrl + Shift + ← ↑ → ↓
Select items in any directionCtrl + Return
Open an entryCtrl + V
Paste element in the clipboard to the current locationCtrl + X
Cut an entryCtrl + C
Copy an entryCtrl + Delete
Remove an entryCtrl + Backspace
Clear selection/Go back to previous locationCtrl + Esc
Clear the selection/Clear the filter
Structure
This control inherits from MauiKit Page, so it has a header and footer. The header bar has a search field for performing searches, by default it is hidden.
The footer bar by default has contextual buttons, depending on the current location. For example, for the trash location, it has contextual actions for emptying the trash can, etc.
- Warning
- It is recommended to not add elements to the footer or header bars, instead - if needed - wrap this control into a MauiKit Page, and utilize its toolbars.
You can find a more complete example at this link.
Definition at line 96 of file FileBrowser.qml.
Property Documentation
◆ browser
|
read |
An alias to the control listing the entries.
- Remarks
- This property is read-only
This component is handled by BrowserView, and exposed for fine tuning its properties
Definition at line 145 of file FileBrowser.qml.
◆ currentFMList
|
read |
The file browser model list.
The List and Grid views use the same FMList.
- See also
- FMList
- Remarks
- This property is read-only
Definition at line 173 of file FileBrowser.qml.
◆ currentFMModel
|
read |
The file browser model controller.
This is the controller which allows for filtering, index mapping, and sorting.
- See also
- MauiModel
- Remarks
- This property is read-only
Definition at line 179 of file FileBrowser.qml.
◆ currentIndex
|
read |
Current index of the item selected in the file browser.
Definition at line 158 of file FileBrowser.qml.
◆ currentPath
|
read |
The current URL path for the directory or location.
To list a directory path, or other location, use the right schema, some of them are file://
, webdav://
, trash://
/, tags://
, fish://
. By default a URL without a well defined schema will be assumed as a local file path.
- Note
- KDE KIO is used as the back-end technology, so any of the supported plugins by KIO will also work here.
Definition at line 114 of file FileBrowser.qml.
◆ currentView
|
read |
Current view of the file browser.
- Remarks
- This property is read-only
Possible views are:
- List = MauiKit::ListBrowser
- Grid = MauiKit::GridBrowser
Definition at line 166 of file FileBrowser.qml.
◆ dialog
|
read |
An alias to the currently loaded dialog, if not dialog is loaded then null.
- Remarks
- This property is read-only
Definition at line 262 of file FileBrowser.qml.
◆ dropArea
|
read |
Drop area component, for dropping files.
- Remarks
- This property is read-only
By default some of the drop actions are handled, for other type of URIs this alias can be used to handle those.
The urlsDropped signal is emitted once one or multiple valid file URLs are dropped, and a popup contextual menu is opened with the supported default actions.
Definition at line 154 of file FileBrowser.qml.
◆ gridItemSize
|
read |
Size of the items in the grid view.
The size is for the combined thumbnail/icon and the title label.
Definition at line 197 of file FileBrowser.qml.
◆ isSearchView
|
read |
Whether the file browser current view is the search view.
- Remarks
- This property is read-only
Definition at line 184 of file FileBrowser.qml.
◆ listItemSize
|
read |
Size of the items in the list view.
- Note
- The size is actually taken as the size of the icon thumbnail. And it is mapped to the nearest size of the standard icon sizes.
Definition at line 203 of file FileBrowser.qml.
◆ readOnly
|
read |
Whether the browser is on a read only mode, and modifications are now allowed, such as pasting, moving, removing or renaming.
Definition at line 267 of file FileBrowser.qml.
◆ selectionBar
|
read |
The SelectionBar to be used for adding items to the selected group.
This is optional, but if it is not set, then some feature will not work, such as selection mode, selection shortcuts etc.
- See also
- MauiKit::SelectionBar
Definition at line 255 of file FileBrowser.qml.
◆ selectionMode
|
read |
Whether the file browser enters selection mode, allowing the selection of multiple items.
This will make all the browsing entries checkable.
Definition at line 190 of file FileBrowser.qml.
◆ settings
|
read |
A group of properties for controlling the sorting, listing and other behaviour of the browser.
- Remarks
- This property is read-only
For more details check the BrowserSettings documentation.
- See also
- BrowserSettings
Definition at line 132 of file FileBrowser.qml.
◆ view
|
read |
The browser could be in two different view states: [1]the file browsing or [2]the search view.
This property gives access to the current view in use.
- Remarks
- This property is read-only
Definition at line 138 of file FileBrowser.qml.
Member Function Documentation
◆ areaClicked
|
signal |
Emitted when an empty area of the browser has been clicked.
- Parameters
-
mouse the object with the event information
◆ itemClicked
|
signal |
Emitted when an item has been clicked.
- Parameters
-
index the index position of the item
◆ itemDoubleClicked
|
signal |
Emitted when an item has been double clicked.
- Parameters
-
index the index position of the item
◆ itemLeftEmblemClicked
|
signal |
Emitted when an item left emblem badge has been clicked.
This is actually a signal to checkable item being toggled.
- Parameters
-
index the index position of the item
◆ itemRightClicked
|
signal |
Emitted when an item has been right clicked.
On mobile devices this is translated from a long press and release.
- Parameters
-
index the index position of the item
◆ keyPress
|
signal |
A key, physical or not, has been pressed.
- Parameters
-
event the event object contains the relevant information
◆ rightClicked
|
signal |
Emitted when an empty area of the browser has been right clicked.
◆ urlsDropped
|
signal |
Emitted when a list of file URLS has been dropped onto the file browser area.
- Parameters
-
urls the list of URLs of the files dropped
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 4 2024 16:32:33 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.