MauiList
#include <mauilist.h>
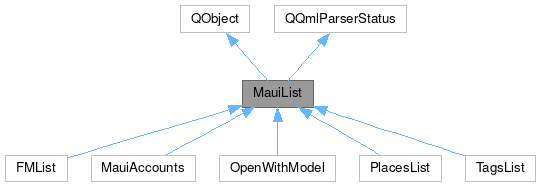
Properties | |
QML_ANONYMOUSint | count |
![]() | |
objectName | |
Signals | |
void | countChanged () |
void | itemMoved (int index, int to) |
void | postItemAppended () |
void | postItemRemoved () |
void | postListChanged () |
void | preItemAppended () |
void | preItemAppendedAt (int index) |
void | preItemRemoved (int index) |
void | preItemsAppended (uint count) |
void | preListChanged () |
void | updateModel (int index, QVector< int > roles) |
Public Slots | |
QVariantMap | get (const int &index) const |
Public Member Functions | |
MauiList (QObject *parent=nullptr) | |
virtual void | classBegin () override |
virtual void | componentComplete () override |
int | getCount () const |
FMH::MODEL | getItem (const int &index) const |
virtual const FMH::MODEL_LIST & | items () const =0 |
virtual void | modelHooked () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
bool | exists (const FMH::MODEL_KEY &key, const QString &value) const |
int | indexOf (const FMH::MODEL_KEY &key, const QString &value) const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
MauiList class.
A helper class for easily setting up a list model to be feed into MauiModel::list, and to be used by the browsing views in Mauikit controls.
- Warning
- This method of setting up a data model is very limited by the lingo supported by the FMH::MODEL_KEY dictionary. So only consider using this class if your data model structure is based on only string text and can be represented by the FMH::MODEL_KEY dictionary entries.
- See also
- FMH::MODEL_KEY
This inherits from the QQmlParserStatus class, so t can be aware of its creation status in the QML engine. This is useful to lazy-loading parts when needed, as when the component presenting the data model is ready and loaded.
The list generated by sub-classing MauiList is meant to be used as the list for the MauiModel class, exposed to QML as the BaseModel
type. The MauiModel supports features, such as filtering and sorting.
- See also
- MauiModel
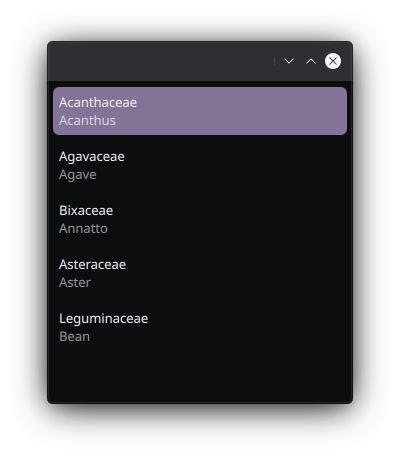
Minimal Example
This is a simple example of a MauiList based data model.
First wee need to setup the MauiList data. In the example below the data is manually added. The data must be modeled using the FMH::MODEL_LIST, which is an array list of FMH::MODEL, which is a map of key pairs, where the key must be a FMH::MODEL_KEY enum type, and the value a string text.
In this example the data is only retrieved once the QML engine has completely loaded the component using the MauiList, for this we override the componentComplete()
virtual method, and there we inform the MauiModel with the signals that the list data is ready.
Now we register our own custom PlanstList class to the QML engine as a type.
And finally, we can consume the data list by hooking it up to the MauiModel exposed type BaseModel
.
You can find a more complete example at this link.
Definition at line 179 of file mauilist.h.
Property Documentation
◆ count
|
read |
The total amount of elements in the list.
- Note
- This needs to be setup manually, as in emitting the signal when new items are appended or removed, etc.
Definition at line 192 of file mauilist.h.
Constructor & Destructor Documentation
◆ MauiList()
|
explicit |
Default constructor.
The usage of this class is meant to be via inheritance by sub-classing it.
Definition at line 22 of file mauilist.cpp.
Member Function Documentation
◆ classBegin()
|
inlineoverridevirtual |
See the Qt documentation on the QQmlParserStatus.
Implements QQmlParserStatus.
Definition at line 211 of file mauilist.h.
◆ componentComplete()
|
inlineoverridevirtual |
See the Qt documentation on the QQmlParserStatus.
Implements QQmlParserStatus.
Definition at line 216 of file mauilist.h.
◆ countChanged
|
signal |
This signal should be emitted by the implementation when the number of elements in the list data model varies.
For example when an item is removed or added.
◆ exists()
|
protected |
Whether an item with a given key-par value exists in the list.
- Parameters
-
key the FMH::MODEL_KEY to look for value the value associated with the key to look for
- Returns
- an exact match exists or not
Definition at line 46 of file mauilist.cpp.
◆ get
|
slot |
Request to get an item in the list, the item is represented as a QVariantMap for easy consumption within the QML scope.
This function is exposed to be invoked from QML.
- Parameters
-
index the position of the item to retrieve
- Returns
- The found item/map in the list at the index. If not item is found at the given index, then an empty map is returned.
Definition at line 32 of file mauilist.cpp.
◆ getCount()
int MauiList::getCount | ( | ) | const |
Definition at line 27 of file mauilist.cpp.
◆ getItem()
FMH::MODEL MauiList::getItem | ( | const int & | index | ) | const |
Request to get an item in the list, the item is represented as a FMH::MODEL key pair value.
- Parameters
-
index the position of the item to retrieve
- Returns
- The found item/map in the list at the index. If not item is found at the given index, then an empty map is returned.
Definition at line 37 of file mauilist.cpp.
◆ indexOf()
|
protected |
The index number of an item in the list of a given key-par value.
- Parameters
-
key the FMH::MODEL_KEY to look for value the value associated with the key to look for
- Returns
- the found index or
-1
if not found
Definition at line 51 of file mauilist.cpp.
◆ itemMoved
|
signal |
This signal should be emitted by the implementation when an item has been moved from one index position to another.
- Parameters
-
index the original index position of the item in the list data model to the new index destination of the item
◆ items()
|
pure virtual |
The modeled data represented by a FMH::MODEL_LIST.
- Note
- The data must be modeled using the FMH::MODEL_LIST, which is an array list of FMH::MODEL elements, which is a map of key pairs, where the key must be a FMH::MODEL_KEY enum type, and the value a string text.
- Returns
- The data model.
Implemented in FMList.
◆ modelHooked()
|
inlinevirtual |
This function is called once the MauiList has been hooked to the MauiModel, using the MauiModel::list property.
Definition at line 221 of file mauilist.h.
◆ postItemAppended
|
signal |
This signal should be emitted by the implementation after one or multiple new items have finished being added into the list data model.
◆ postItemRemoved
|
signal |
This signal should be emitted by the implementation after an item has been successfully removed from the list data model.
◆ postListChanged
|
signal |
This signal should be emitted by the implementation after the list data model is set and done.
◆ preItemAppended
|
signal |
This signal should be emitted by the implementation before appending a new item to the list data model.
◆ preItemAppendedAt
|
signal |
This signal should be emitted by the implementation before a new item has been inserted at a given index to the list data model.
- Parameters
-
index the position index where the new item was inserted at
◆ preItemRemoved
|
signal |
This signal should be emitted by the implementation before an item has been removed at the given index position.
- Parameters
-
index the index position of the element that will be removed
◆ preItemsAppended
|
signal |
This signal should be emitted by the implementation before appending a multiple new items to the list data model.
- Parameters
-
count the total amount of new items that will be added
◆ preListChanged
|
signal |
This signal should be emitted by the implementation before the list data model has been assigned or populated.
◆ updateModel
|
signal |
This signal should be emitted by the implementation when changes have been done in the list data model.
- Parameters
-
index the index position of the item that was modified in the list data model roles the keys that were modified, the key values can be FMH::MODEL_KEY
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri May 17 2024 11:56:16 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.