PlacesList
#include <placeslist.h>
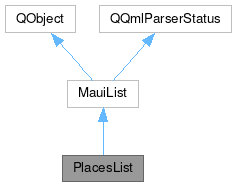
Properties | |
QML_ELEMENTQVariantList | groups |
![]() | |
QML_ANONYMOUSint | count |
![]() | |
objectName | |
Signals | |
void | bookmarksChanged () |
void | groupsChanged () |
![]() | |
void | countChanged () |
void | itemMoved (int index, int to) |
void | postItemAppended () |
void | postItemRemoved () |
void | postListChanged () |
void | preItemAppended () |
void | preItemAppendedAt (int index) |
void | preItemRemoved (int index) |
void | preItemsAppended (uint count) |
void | preListChanged () |
void | updateModel (int index, QVector< int > roles) |
Public Slots | |
static void | addBookmark (const QUrl &url) |
bool | contains (const QUrl &path) |
bool | containsGroup (const int &group) |
int | indexOfPath (const QUrl &url) const |
bool | isDevice (const int &index) |
void | removePlace (const int &index) |
void | requestEject (const int &index) |
void | requestSetup (const int &index) |
bool | setupNeeded (const int &index) |
void | toggleSection (const int §ion) |
![]() | |
QVariantMap | get (const int &index) const |
Public Member Functions | |
PlacesList (QObject *parent=nullptr) | |
QVariantList | getGroups () const |
void | setGroups (const QVariantList &value) |
![]() | |
MauiList (QObject *parent=nullptr) | |
virtual void | classBegin () override |
FMH::MODEL | getItem (const int &index) const |
virtual void | modelHooked () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() |
Protected Member Functions | |
void | setList () |
![]() | |
bool | exists (const FMH::MODEL_KEY &key, const QString &value) const |
int | indexOf (const FMH::MODEL_KEY &key, const QString &value) const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Detailed Description
The list model of the system locations, such as bookmarks, standard places, networks and devices.
A graphical interface for this controller functionality is available for quick usage as PlacesListBrowser.
Definition at line 39 of file placeslist.h.
Property Documentation
◆ groups
|
readwrite |
The groups to be listed.
The possible list of groups are defined at FMList::PATHTYPE To set it from QML:
Definition at line 53 of file placeslist.h.
Constructor & Destructor Documentation
◆ PlacesList()
PlacesList::PlacesList | ( | QObject * | parent = nullptr | ) |
Definition at line 58 of file placeslist.cpp.
Member Function Documentation
◆ addBookmark
|
staticslot |
Add a location to the bookmarks sections.
- Parameters
-
url The URL path of the location or directory
Definition at line 325 of file placeslist.cpp.
◆ bookmarksChanged
|
signal |
Emitted when a new bookmark entry has been added.
◆ contains
|
slot |
Checks of a file URL exists in the places model.
- Parameters
-
path file URL to be checked
- Returns
- Whether it exists
Definition at line 263 of file placeslist.cpp.
◆ containsGroup
|
slot |
Whether the current listing contains a group type.
The possible values are defined in FMStatic::PATHTYPE_KEY
- Parameters
-
group the group type
- Returns
- whether it is being listed
Definition at line 362 of file placeslist.cpp.
◆ getGroups()
QVariantList PlacesList::getGroups | ( | ) | const |
Definition at line 231 of file placeslist.cpp.
◆ indexOfPath
|
slot |
Given an URL path, if it exists in the places list return its index position.
- Parameters
-
url The URL path to be checked
- Returns
- the index position if it exists otherwise
-1
Definition at line 344 of file placeslist.cpp.
◆ isDevice
|
slot |
Check if a entry at the given index is a device.
- Parameters
-
index index position of the entry in the list
- Returns
- whether it is a device type
Definition at line 268 of file placeslist.cpp.
◆ removePlace
|
slot |
Removes a place from the model and if the data at the given index is a file URL bookmark then it gets removed from the bookmarks.
- Parameters
-
index index of the item to be removed in the model
Definition at line 245 of file placeslist.cpp.
◆ requestEject
|
slot |
Request to eject a removable device type at the given index.
- Parameters
-
index the index position of the entry
Definition at line 297 of file placeslist.cpp.
◆ requestSetup
|
slot |
Request to setup or mount the device type entry at the given index.
- Parameters
-
index index position of the entry
Definition at line 311 of file placeslist.cpp.
◆ setGroups()
void PlacesList::setGroups | ( | const QVariantList & | value | ) |
Definition at line 236 of file placeslist.cpp.
◆ setList()
|
protected |
Definition at line 184 of file placeslist.cpp.
◆ setupNeeded
|
slot |
Check if a device type entry needs to be setup, as in mounted.
- Parameters
-
index the index position of the entry
- Returns
- whether it needs to be setup
Definition at line 281 of file placeslist.cpp.
◆ toggleSection
|
slot |
Hide/show a section.
- Parameters
-
The section type to be toggle. The possible values are defined in FMStatic::PATHTYPE_KEY.
Definition at line 349 of file placeslist.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:10:48 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.