TagsList
#include <tagslist.h>
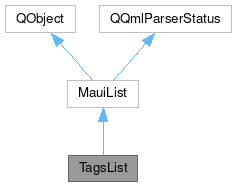
Properties | |
QStringList | newTags |
QML_ELEMENTbool | strict |
QStringList | tags |
QStringList | urls |
![]() | |
QML_ANONYMOUSint | count |
![]() | |
objectName | |
Signals | |
void | strictChanged () |
void | tagsChanged () |
void | urlsChanged () |
![]() | |
void | countChanged () |
void | itemMoved (int index, int to) |
void | postItemAppended () |
void | postItemRemoved () |
void | postListChanged () |
void | preItemAppended () |
void | preItemAppendedAt (int index) |
void | preItemRemoved (int index) |
void | preItemsAppended (uint count) |
void | preListChanged () |
void | updateModel (int index, QVector< int > roles) |
Public Slots | |
void | append (const QString &tag) |
void | append (const QStringList &tags) |
void | appendItem (const QVariantMap &tag) |
bool | contains (const QString &tag) |
void | erase (const int &index) |
bool | insert (const QString &tag) |
void | insertToUrls (const QString &tag) |
void | refresh () |
bool | remove (const int &index) |
void | removeFrom (const int &index, const QString &url) |
void | removeFromUrls (const int &index) |
void | removeFromUrls (const QString &tag) |
void | updateToUrls (const QStringList &tags) |
![]() | |
QVariantMap | get (const int &index) const |
Public Member Functions | |
TagsList (QObject *parent=nullptr) | |
void | componentComplete () override final |
QStringList | getNewTags () const |
bool | getStrict () const |
QStringList | getTags () const |
QStringList | getUrls () const |
const FMH::MODEL_LIST & | items () const override |
void | setStrict (const bool &value) |
void | setUrls (const QStringList &value) |
![]() | |
MauiList (QObject *parent=nullptr) | |
virtual void | classBegin () override |
FMH::MODEL | getItem (const int &index) const |
virtual void | modelHooked () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
bool | exists (const FMH::MODEL_KEY &key, const QString &value) const |
int | indexOf (const FMH::MODEL_KEY &key, const QString &value) const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The TagsList class A model of the system tags, ready to be consumed by QML.
This model has basic support for browsing, associating, adding and removing tags.
This is a basic model for most actions supported by the MauiKit File Browsing Tagging system. For more details on supported actions and complete API documentation refer to the Tagging page.
- See also
- Tagging
- Note
- This class is exposed as a QML type with the alias name of
TagsListModel
.
Definition at line 18 of file tagslist.h.
Property Documentation
◆ newTags
|
read |
The list of tags that are still not written to the urls, and are pending to be saved.
Definition at line 44 of file tagslist.h.
◆ strict
|
readwrite |
Whether the retrieved tags should be only associated to the current application or to any other app.
By default this is set to true
, so the lookup will be only matching tags associated to the given URLs that were created by the current application.
Definition at line 29 of file tagslist.h.
◆ tags
|
read |
The resulting list of tag names that were found.
Or compossed, some existing tags might still not be added permanently.
Definition at line 39 of file tagslist.h.
◆ urls
|
readwrite |
The list of file URLs to look for their tags.
Definition at line 34 of file tagslist.h.
Constructor & Destructor Documentation
◆ TagsList()
|
explicit |
Definition at line 6 of file tagslist.cpp.
Member Function Documentation
◆ append [1/2]
|
slot |
Adds a given tag to the model, if the tag already exists in the model then nothing happens.
- Note
- This operation does not inserts the tag to the tagging data base. To insert a new tag see the insert function.
- See also
- insert
- Parameters
-
tag the tag to be added to the model
Definition at line 187 of file tagslist.cpp.
◆ append [2/2]
|
slot |
Adds a given list of tags to the model.
Tags that already exists in the model are ignored.
- Parameters
-
tags list of tags to be added to the model.
- See also
- append
Definition at line 208 of file tagslist.cpp.
◆ appendItem
|
slot |
Adds a given tag map to the model, if the tag map already exists in the model then nothing happens.
- Note
- This operation does not inserts the tag to the tagging data base.
- Parameters
-
tag the tag map to be added to the model. The supported key values are: tag
,color
,date
Definition at line 192 of file tagslist.cpp.
◆ componentComplete()
|
finaloverridevirtual |
Reimplemented from MauiList.
Definition at line 221 of file tagslist.cpp.
◆ contains
|
slot |
Checks whether a given tag name is already in the model list.
- Parameters
-
tag tag name to look up
- Returns
- whether the tag exists
Definition at line 216 of file tagslist.cpp.
◆ erase
|
slot |
Removes a tag from the tagging data base.
This operation will remove the association of the tag to the current application making the request, meaning that if the tag is also associated to another application then the tag will be conserved.
- Parameters
-
index
Definition at line 130 of file tagslist.cpp.
◆ getNewTags()
QStringList TagsList::getNewTags | ( | ) | const |
Definition at line 159 of file tagslist.cpp.
◆ getStrict()
bool TagsList::getStrict | ( | ) | const |
Definition at line 140 of file tagslist.cpp.
◆ getTags()
QStringList TagsList::getTags | ( | ) | const |
Definition at line 154 of file tagslist.cpp.
◆ getUrls()
QStringList TagsList::getUrls | ( | ) | const |
Definition at line 173 of file tagslist.cpp.
◆ insert
|
slot |
Inserts a tag to the tagging data base.
- Parameters
-
tag to be inserted
- Returns
- if the tag already exists in the data base then it return false, if the operation is successful returns true otherwise false
Definition at line 47 of file tagslist.cpp.
◆ insertToUrls
|
slot |
Associates a given tag to the current file URLs set to the URLs property.
- Parameters
-
tag a tag to be associated, if the tag doesn't exists then it gets created
Definition at line 55 of file tagslist.cpp.
◆ items()
|
overridevirtual |
Implements MauiList.
Definition at line 135 of file tagslist.cpp.
◆ refresh
|
slot |
Reloads the model, checking the tags from the given list of file URLs.
Definition at line 42 of file tagslist.cpp.
◆ remove
|
slot |
Removes a tag from the model at a given index.
The tag is removed from the model but not from the tagging data base
- Parameters
-
index index position of the tag in the model. If the model has been filtered or ordered using the MauiKit BaseModel then it should use the mapped index.
- Returns
Definition at line 108 of file tagslist.cpp.
◆ removeFrom
|
slot |
Removes a tag at the given index in the model from the given file URL.
This removes the associated file URL from the tagging data base and the tag from the model
- Parameters
-
index index of the tag in the model url file URL
Definition at line 121 of file tagslist.cpp.
◆ removeFromUrls [1/2]
|
slot |
Removes a tag at a given index in the model from the all the file URLs currently set.
- Parameters
-
index index of the tag in the model.
Definition at line 84 of file tagslist.cpp.
◆ removeFromUrls [2/2]
|
slot |
Removes a given tag name from the current list of file URLs set.
- Parameters
-
tag the tag name
Definition at line 102 of file tagslist.cpp.
◆ setStrict()
void TagsList::setStrict | ( | const bool & | value | ) |
Definition at line 145 of file tagslist.cpp.
◆ setUrls()
void TagsList::setUrls | ( | const QStringList & | value | ) |
Definition at line 178 of file tagslist.cpp.
◆ updateToUrls
|
slot |
Updates a list of tags associated to the current file URLs.
All the previous tags associated to each file URL are removed and replaced by the new ones
- Parameters
-
tags tags to be updated
Definition at line 64 of file tagslist.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:55:53 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.