WheelHandler
#include <wheelhandler.h>
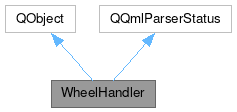
Properties | |
bool | blockTargetWheel |
bool | filterMouseEvents |
qreal | horizontalStepSize |
bool | keyNavigationEnabled |
Qt::KeyboardModifiers | pageScrollModifiers |
Qt::Orientation | primaryOrientation |
bool | scrollFlickableTarget |
QML_ELEMENTQQuickItem * | target |
qreal | verticalStepSize |
![]() | |
objectName | |
Signals | |
void | blockTargetWheelChanged () |
void | filterMouseEventsChanged () |
void | horizontalStepSizeChanged () |
void | keyNavigationEnabledChanged () |
void | pageScrollModifiersChanged () |
void | primaryOrientationChanged () |
void | scrollFlickableTargetChanged () |
void | targetChanged () |
void | verticalStepSizeChanged () |
void | wheel (KirigamiWheelEvent *wheel) |
Public Member Functions | |
WheelHandler (QObject *parent=nullptr) | |
bool | filterMouseEvents () const |
qreal | horizontalStepSize () const |
bool | keyNavigationEnabled () const |
Qt::KeyboardModifiers | pageScrollModifiers () const |
Qt::Orientation | primaryOrientation () const |
void | resetHorizontalStepSize () |
void | resetPageScrollModifiers () |
void | resetVerticalStepSize () |
Q_INVOKABLE bool | scrollDown (qreal stepSize=-1) |
Q_INVOKABLE bool | scrollLeft (qreal stepSize=-1) |
Q_INVOKABLE bool | scrollRight (qreal stepSize=-1) |
Q_INVOKABLE bool | scrollUp (qreal stepSize=-1) |
void | setFilterMouseEvents (bool enabled) |
void | setHorizontalStepSize (qreal stepSize) |
void | setKeyNavigationEnabled (bool enabled) |
void | setPageScrollModifiers (Qt::KeyboardModifiers modifiers) |
void | setPrimaryOrientation (Qt::Orientation orientation) |
void | setTarget (QQuickItem *target) |
void | setVerticalStepSize (qreal stepSize) |
QQuickItem * | target () const |
qreal | verticalStepSize () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
bool | eventFilter (QObject *watched, QEvent *event) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
Handles scrolling for a Flickable and 2 attached ScrollBars.
WheelHandler filters events from a Flickable, a vertical ScrollBar and a horizontal ScrollBar. Wheel and KeyPress events (when keyNavigationEnabled
is true) are used to scroll the Flickable. When filterMouseEvents
is true, WheelHandler blocks mouse button input from reaching the Flickable and sets the interactive
property of the scrollbars to false when touch input is used.
Wheel event handling behavior:
- Pixel delta is ignored unless angle delta is not available because pixel delta scrolling is too slow. Qt Widgets doesn't use pixel delta either, so the default scroll speed should be consistent with Qt Widgets.
- When using angle delta, scroll using the step increments defined by
verticalStepSize
andhorizontalStepSize
. - When one of the keyboard modifiers in
pageScrollModifiers
is used, scroll by pages. - When using a device that doesn't use 120 angle delta unit increments such as a touchpad, the
verticalStepSize
,horizontalStepSize
and page increments (if using page scrolling) will be multiplied byangle delta / 120
to keep scrolling smooth. - If scrolling has happened in the last 400ms, use an internal QQuickItem stacked over the Flickable's contentItem to catch wheel events and use those wheel events to scroll, if possible. This prevents controls inside the Flickable's contentItem that allow scrolling to change the value (e.g., Sliders, SpinBoxes) from conflicting with scrolling the page.
Common usage with a Flickable:
Common usage inside of a ScrollView template:
Definition at line 170 of file wheelhandler.h.
Property Documentation
◆ blockTargetWheel
bool WheelHandler::blockTargetWheel |
This property holds whether the WheelHandler blocks all wheel events from reaching the Flickable.
When this property is false, scrolling the Flickable with WheelHandler will only block an event from reaching the Flickable if the Flickable is actually scrolled by WheelHandler.
NOTE: Wheel events created by touchpad gestures with pixel deltas will always be accepted no matter what. This is because they will cause the Flickable to jump back to where scrolling started unless the events are always accepted before they reach the Flickable.
The default value is true.
Definition at line 263 of file wheelhandler.h.
◆ filterMouseEvents
|
readwrite |
This property holds whether the WheelHandler filters mouse events like a Qt Quick Controls ScrollView would.
Touch events are allowed to flick the view and they make the scrollbars not interactive.
Mouse events are not allowed to flick the view and they make the scrollbars interactive.
Hover events on the scrollbars and wheel events on anything also make the scrollbars interactive when this property is set to true.
The default value is false
.
- Since
- KDE Frameworks 5.89
Definition at line 231 of file wheelhandler.h.
◆ horizontalStepSize
|
readwrite |
This property holds the horizontal step size.
The default value is equivalent to 20 * Qt.styleHints.wheelScrollLines
. This is consistent with the default increment for QScrollArea.
- See also
- verticalStepSize
- Since
- KDE Frameworks 5.89
Definition at line 203 of file wheelhandler.h.
◆ keyNavigationEnabled
|
readwrite |
This property holds whether the WheelHandler handles keyboard scrolling.
- Left arrow scrolls a step to the left.
- Right arrow scrolls a step to the right.
- Up arrow scrolls a step upwards.
- Down arrow scrolls a step downwards.
- PageUp scrolls to the previous page.
- PageDown scrolls to the next page.
- Home scrolls to the beginning.
- End scrolls to the end.
- When Alt is held, scroll horizontally when using PageUp, PageDown, Home or End.
The default value is false
.
- Since
- KDE Frameworks 5.89
Definition at line 251 of file wheelhandler.h.
◆ pageScrollModifiers
|
readwrite |
This property holds the keyboard modifiers that will be used to start page scrolling.
The default value is equivalent to Qt.ControlModifier | Qt.ShiftModifier
. This matches QScrollBar, which uses QAbstractSlider behavior.
- Since
- KDE Frameworks 5.89
Definition at line 214 of file wheelhandler.h.
◆ primaryOrientation
|
readwrite |
Definition at line 272 of file wheelhandler.h.
◆ scrollFlickableTarget
bool WheelHandler::scrollFlickableTarget |
This property holds whether the WheelHandler can use wheel events to scroll the Flickable.
The default value is true.
Definition at line 270 of file wheelhandler.h.
◆ target
|
readwrite |
This property holds the Qt Quick Flickable that the WheelHandler will control.
Definition at line 179 of file wheelhandler.h.
◆ verticalStepSize
|
readwrite |
This property holds the vertical step size.
The default value is equivalent to 20 * Qt.styleHints.wheelScrollLines
. This is consistent with the default increment for QScrollArea.
- See also
- horizontalStepSize
- Since
- KDE Frameworks 5.89
Definition at line 190 of file wheelhandler.h.
Constructor & Destructor Documentation
◆ WheelHandler()
|
explicit |
Definition at line 87 of file wheelhandler.cpp.
◆ ~WheelHandler()
|
override |
Definition at line 114 of file wheelhandler.cpp.
Member Function Documentation
◆ eventFilter()
Reimplemented from QObject.
Definition at line 567 of file wheelhandler.cpp.
◆ filterMouseEvents()
bool WheelHandler::filterMouseEvents | ( | ) | const |
Definition at line 356 of file wheelhandler.cpp.
◆ horizontalStepSize()
qreal WheelHandler::horizontalStepSize | ( | ) | const |
Definition at line 307 of file wheelhandler.cpp.
◆ keyNavigationEnabled()
bool WheelHandler::keyNavigationEnabled | ( | ) | const |
Definition at line 370 of file wheelhandler.cpp.
◆ pageScrollModifiers()
Qt::KeyboardModifiers WheelHandler::pageScrollModifiers | ( | ) | const |
Definition at line 337 of file wheelhandler.cpp.
◆ primaryOrientation()
Qt::Orientation WheelHandler::primaryOrientation | ( | ) | const |
Definition at line 411 of file wheelhandler.cpp.
◆ resetHorizontalStepSize()
void WheelHandler::resetHorizontalStepSize | ( | ) |
Definition at line 327 of file wheelhandler.cpp.
◆ resetPageScrollModifiers()
void WheelHandler::resetPageScrollModifiers | ( | ) |
Definition at line 351 of file wheelhandler.cpp.
◆ resetVerticalStepSize()
void WheelHandler::resetVerticalStepSize | ( | ) |
Definition at line 297 of file wheelhandler.cpp.
◆ scrollDown()
bool WheelHandler::scrollDown | ( | qreal | stepSize = -1 | ) |
Scroll down one step.
If the stepSize parameter is less than 0, the verticalStepSize will be used.
returns true if the contentItem was moved.
- Since
- KDE Frameworks 5.89
Definition at line 534 of file wheelhandler.cpp.
◆ scrollLeft()
bool WheelHandler::scrollLeft | ( | qreal | stepSize = -1 | ) |
Scroll left one step.
If the stepSize parameter is less than 0, the horizontalStepSize will be used.
returns true if the contentItem was moved.
- Since
- KDE Frameworks 5.89
Definition at line 545 of file wheelhandler.cpp.
◆ scrollRight()
bool WheelHandler::scrollRight | ( | qreal | stepSize = -1 | ) |
Scroll right one step.
If the stepSize parameter is less than 0, the horizontalStepSize will be used.
returns true if the contentItem was moved.
- Since
- KDE Frameworks 5.89
Definition at line 556 of file wheelhandler.cpp.
◆ scrollUp()
bool WheelHandler::scrollUp | ( | qreal | stepSize = -1 | ) |
Scroll up one step.
If the stepSize parameter is less than 0, the verticalStepSize will be used.
returns true if the contentItem was moved.
- Since
- KDE Frameworks 5.89
Definition at line 523 of file wheelhandler.cpp.
◆ setFilterMouseEvents()
void WheelHandler::setFilterMouseEvents | ( | bool | enabled | ) |
Definition at line 361 of file wheelhandler.cpp.
◆ setHorizontalStepSize()
void WheelHandler::setHorizontalStepSize | ( | qreal | stepSize | ) |
Definition at line 312 of file wheelhandler.cpp.
◆ setKeyNavigationEnabled()
void WheelHandler::setKeyNavigationEnabled | ( | bool | enabled | ) |
Definition at line 375 of file wheelhandler.cpp.
◆ setPageScrollModifiers()
void WheelHandler::setPageScrollModifiers | ( | Qt::KeyboardModifiers | modifiers | ) |
Definition at line 342 of file wheelhandler.cpp.
◆ setPrimaryOrientation()
void WheelHandler::setPrimaryOrientation | ( | Qt::Orientation | orientation | ) |
Definition at line 416 of file wheelhandler.cpp.
◆ setTarget()
void WheelHandler::setTarget | ( | QQuickItem * | target | ) |
Definition at line 124 of file wheelhandler.cpp.
◆ setVerticalStepSize()
void WheelHandler::setVerticalStepSize | ( | qreal | stepSize | ) |
Definition at line 282 of file wheelhandler.cpp.
◆ target()
QQuickItem * WheelHandler::target | ( | ) | const |
Definition at line 119 of file wheelhandler.cpp.
◆ verticalStepSize()
qreal WheelHandler::verticalStepSize | ( | ) | const |
Definition at line 277 of file wheelhandler.cpp.
◆ wheel
|
signal |
This signal is emitted when a wheel event reaches the event filter, just before scrolling is handled.
Accepting the wheel event in the onWheel
signal handler prevents scrolling from happening.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Apr 27 2024 22:10:06 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.