Okular::Annotation
#include <annotations.h>
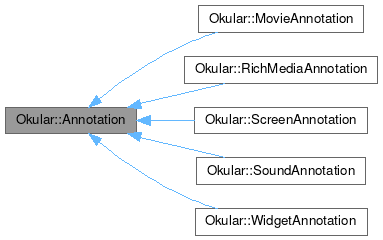
Classes | |
class | Revision |
class | Style |
class | Window |
Public Types | |
enum | AdditionalActionType { PageOpening , PageClosing , CursorEntering , CursorLeaving , MousePressed , MouseReleased , FocusIn , FocusOut } |
typedef void(* | DisposeDataFunction) (const Okular::Annotation *) |
enum | Flag { Hidden = 1 , FixedSize = 2 , FixedRotation = 4 , DenyPrint = 8 , DenyWrite = 16 , DenyDelete = 32 , ToggleHidingOnMouse = 64 , External = 128 , ExternallyDrawn = 256 , BeingMoved = 512 , BeingResized = 1024 } |
enum | LineEffect { NoEffect = 1 , Cloudy = 2 } |
enum | LineStyle { Solid = 1 , Dashed = 2 , Beveled = 4 , Inset = 8 , Underline = 16 } |
enum | RevisionScope { Reply = 1 , Group = 2 , Delete = 4 } |
enum | RevisionType { None = 1 , Marked = 2 , Unmarked = 4 , Accepted = 8 , Rejected = 16 , Cancelled = 32 , Completed = 64 } |
enum | SubType { AText = 1 , ALine = 2 , AGeom = 3 , AHighlight = 4 , AStamp = 5 , AInk = 6 , ACaret = 8 , AFileAttachment = 9 , ASound = 10 , AMovie = 11 , AScreen = 12 , AWidget = 13 , ARichMedia = 14 , A_BASE = 0 } |
Detailed Description
Annotation struct holds properties shared by all annotations.
An Annotation is an object (text note, highlight, sound, popup window, ..) contained by a Page in the document.
Definition at line 95 of file annotations.h.
Member Typedef Documentation
◆ DisposeDataFunction
typedef void(* Okular::Annotation::DisposeDataFunction) (const Okular::Annotation *) |
A function to be called when the annotation is destroyed.
- Warning
- the function must not call any virtual function, nor subcast.
- Since
- 0.7 (KDE 4.1)
Definition at line 209 of file annotations.h.
Member Enumeration Documentation
◆ AdditionalActionType
Describes the type of additional actions.
- Since
- 0.16 (KDE 4.10)
Definition at line 190 of file annotations.h.
◆ Flag
Describes additional properties of an annotation.
Definition at line 130 of file annotations.h.
◆ LineEffect
Describes possible line effects for.
- See also
- ALine annotation.
Enumerator | |
---|---|
NoEffect | No effect. |
Cloudy | The cloudy effect. |
Definition at line 158 of file annotations.h.
◆ LineStyle
Describes possible line styles for.
- See also
- ALine annotation.
Enumerator | |
---|---|
Solid | A solid line. |
Dashed | A dashed line. |
Beveled | A beveled line. |
Inset | An inset line. |
Underline | An underline. |
Definition at line 147 of file annotations.h.
◆ RevisionScope
Describes the scope of revision information.
Enumerator | |
---|---|
Reply | Belongs to a reply. |
Group | Belongs to a group. |
Delete | Belongs to a deleted paragraph. |
Definition at line 166 of file annotations.h.
◆ RevisionType
Describes the type of revision information.
Enumerator | |
---|---|
None | Not specified. |
Marked | Is marked. |
Unmarked | Is unmarked. |
Accepted | Has been accepted. |
Rejected | Was rejected. |
Cancelled | Has been cancelled. |
Completed | Has been completed. |
Definition at line 175 of file annotations.h.
◆ SubType
Describes the type of annotation as defined in PDF standard.
Definition at line 110 of file annotations.h.
Constructor & Destructor Documentation
◆ ~Annotation()
|
virtual |
Destroys the annotation.
Definition at line 583 of file annotations.cpp.
Member Function Documentation
◆ adjust()
void Annotation::adjust | ( | const NormalizedPoint & | deltaCoord1, |
const NormalizedPoint & | deltaCoord2 ) |
Adjust the annotation by the specified coordinates.
Adds coordinates of deltaCoord1
to annotations top left corner, and deltaCoord2
to the bottom right.
- See also
- canBeResized()
Definition at line 696 of file annotations.cpp.
◆ author()
QString Annotation::author | ( | ) | const |
Returns the author of the annotation.
Definition at line 598 of file annotations.cpp.
◆ boundingRectangle()
NormalizedRect Annotation::boundingRectangle | ( | ) | const |
Returns the bounding rectangle of the annotation.
Definition at line 674 of file annotations.cpp.
◆ canBeMoved()
bool Annotation::canBeMoved | ( | ) | const |
Returns whether the annotation can be moved.
- Since
- 0.7 (KDE 4.1)
Definition at line 766 of file annotations.cpp.
◆ canBeResized()
bool Annotation::canBeResized | ( | ) | const |
Returns whether the annotation can be resized.
Definition at line 783 of file annotations.cpp.
◆ contents()
QString Annotation::contents | ( | ) | const |
Returns the contents of the annotation.
Definition at line 610 of file annotations.cpp.
◆ creationDate()
QDateTime Annotation::creationDate | ( | ) | const |
Returns the creation date of the annotation.
Definition at line 646 of file annotations.cpp.
◆ flags()
int Annotation::flags | ( | ) | const |
◆ getAnnotationPropertiesDomNode()
QDomNode Annotation::getAnnotationPropertiesDomNode | ( | ) | const |
Retrieve the QDomNode representing this annotation's properties.
- Since
- 0.17 (KDE 4.11)
Definition at line 889 of file annotations.cpp.
◆ modificationDate()
QDateTime Annotation::modificationDate | ( | ) | const |
Returns the last modification date of the annotation.
Definition at line 634 of file annotations.cpp.
◆ nativeId()
QVariant Annotation::nativeId | ( | ) | const |
Returns the "native" id of the annotation.
- Since
- 0.7 (KDE 4.1)
Definition at line 754 of file annotations.cpp.
◆ openDialogAfterCreation()
bool Annotation::openDialogAfterCreation | ( | ) | const |
Returns whether the annotation dialog should be open after creation of the annotation or not.
- Since
- 0.13 (KDE 4.7)
Definition at line 706 of file annotations.cpp.
◆ revisions() [1/2]
QList< Annotation::Revision > & Annotation::revisions | ( | ) |
Returns a reference to the revision list of the annotation.
Definition at line 736 of file annotations.cpp.
◆ revisions() [2/2]
const QList< Annotation::Revision > & Annotation::revisions | ( | ) | const |
Returns a reference to the revision list of the annotation.
Definition at line 742 of file annotations.cpp.
◆ setAnnotationProperties()
void Annotation::setAnnotationProperties | ( | const QDomNode & | node | ) |
Sets annotations internal properties according to the contents of node
.
- Since
- 0.17 (KDE 4.11)
Definition at line 898 of file annotations.cpp.
◆ setAuthor()
void Annotation::setAuthor | ( | const QString & | author | ) |
Sets the author
of the annotation.
Definition at line 592 of file annotations.cpp.
◆ setBoundingRectangle()
void Annotation::setBoundingRectangle | ( | const NormalizedRect & | rectangle | ) |
Sets the bounding rectangle
of the annotation.
Definition at line 664 of file annotations.cpp.
◆ setContents()
void Annotation::setContents | ( | const QString & | contents | ) |
Sets the contents
of the annotation.
Definition at line 604 of file annotations.cpp.
◆ setCreationDate()
void Annotation::setCreationDate | ( | const QDateTime & | date | ) |
Sets the creation date
of the annotation.
The date must be before or equal to
- See also
- modificationDate()
Definition at line 640 of file annotations.cpp.
◆ setDisposeDataFunction()
void Annotation::setDisposeDataFunction | ( | DisposeDataFunction | func | ) |
Sets a function to be called when the annotation is destroyed.
- Warning
- the function must not call any virtual function, nor subcast.
- Since
- 0.7 (KDE 4.1)
Definition at line 760 of file annotations.cpp.
◆ setFlags()
void Annotation::setFlags | ( | int | flags | ) |
◆ setModificationDate()
void Annotation::setModificationDate | ( | const QDateTime & | date | ) |
Sets the last modification date
of the annotation.
The date must be before or equal to QDateTime::currentDateTime()
Definition at line 628 of file annotations.cpp.
◆ setNativeId()
void Annotation::setNativeId | ( | const QVariant & | id | ) |
Sets the "native" id
of the annotation.
This is for use of the Generator, that can optionally store an handle (a pointer, an identifier, etc) of the "native" annotation object, if any.
- Note
- Okular makes no use of this
- Since
- 0.7 (KDE 4.1)
Definition at line 748 of file annotations.cpp.
◆ setUniqueName()
void Annotation::setUniqueName | ( | const QString & | name | ) |
Sets the unique name
of the annotation.
Definition at line 616 of file annotations.cpp.
◆ store()
|
virtual |
Stores the annotation as xml in document
under the given parent node
.
Reimplemented in Okular::MovieAnnotation, Okular::RichMediaAnnotation, Okular::ScreenAnnotation, Okular::SoundAnnotation, and Okular::WidgetAnnotation.
Definition at line 795 of file annotations.cpp.
◆ style() [1/2]
Annotation::Style & Annotation::style | ( | ) |
Returns a reference to the style object of the annotation.
Definition at line 712 of file annotations.cpp.
◆ style() [2/2]
const Annotation::Style & Annotation::style | ( | ) | const |
Returns a const reference to the style object of the annotation.
Definition at line 718 of file annotations.cpp.
◆ subType()
|
pure virtual |
Returns the sub type of the annotation.
Implemented in Okular::MovieAnnotation, Okular::RichMediaAnnotation, Okular::ScreenAnnotation, Okular::SoundAnnotation, and Okular::WidgetAnnotation.
◆ transformedBoundingRectangle()
NormalizedRect Annotation::transformedBoundingRectangle | ( | ) | const |
Returns the transformed bounding rectangle of the annotation.
This rectangle must be used when showing annotations on screen to have them rotated correctly.
Definition at line 680 of file annotations.cpp.
◆ translate()
void Annotation::translate | ( | const NormalizedPoint & | coord | ) |
Move the annotation by the specified coordinates.
- See also
- canBeMoved()
Definition at line 686 of file annotations.cpp.
◆ uniqueName()
QString Annotation::uniqueName | ( | ) | const |
Returns the unique name of the annotation.
Definition at line 622 of file annotations.cpp.
◆ window() [1/2]
Annotation::Window & Annotation::window | ( | ) |
Returns a reference to the window object of the annotation.
Definition at line 724 of file annotations.cpp.
◆ window() [2/2]
const Annotation::Window & Annotation::window | ( | ) | const |
Returns a const reference to the window object of the annotation.
Definition at line 730 of file annotations.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:51:38 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.