Okular::BookmarkManager
#include <bookmarkmanager.h>
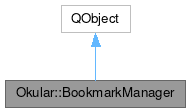
Signals | |
void | bookmarksChanged (const QUrl &url) |
void | openUrl (const QUrl &url) |
void | saved () |
Public Member Functions | |
QList< QAction * > | actionsForUrl (const QUrl &documentUrl) const |
void | addBookmark (const DocumentViewport &vp) |
bool | addBookmark (const QUrl &documentUrl, const Okular::DocumentViewport &vp, const QString &title=QString()) |
void | addBookmark (int page) |
KBookmark | bookmark (const DocumentViewport &viewport) const |
KBookmark | bookmark (int page) const |
KBookmark::List | bookmarks () const |
KBookmark::List | bookmarks (const QUrl &documentUrl) const |
KBookmark::List | bookmarks (int page) const |
QList< QUrl > | files () const |
bool | isBookmarked (const DocumentViewport &viewport) const |
bool | isBookmarked (int page) const |
KBookmark | nextBookmark (const DocumentViewport &viewport) const |
KBookmark | previousBookmark (const DocumentViewport &viewport) const |
void | removeBookmark (const DocumentViewport &vp) |
int | removeBookmark (const QUrl &documentUrl, const KBookmark &bm) |
void | removeBookmark (int page) |
void | removeBookmarks (const QUrl &documentUrl, const KBookmark::List &list) |
void | renameBookmark (const QUrl &documentUrl, const QString &newName) |
void | renameBookmark (KBookmark *bm, const QString &newName) |
void | save () const |
QString | titleForUrl (const QUrl &documentUrl) const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Bookmarks manager utility.
This class is responsible for loading and saving the bookmarks using the proper format, and for working with them (eg querying, adding, removing).
Definition at line 30 of file bookmarkmanager.h.
Constructor & Destructor Documentation
◆ ~BookmarkManager()
|
override |
Definition at line 176 of file bookmarkmanager.cpp.
Member Function Documentation
◆ actionsForUrl()
Returns a list of actions for the bookmarks of the specified url
.
- Note
- the actions will have no parents, so you have to delete them yourself
Definition at line 617 of file bookmarkmanager.cpp.
◆ addBookmark() [1/3]
void BookmarkManager::addBookmark | ( | const DocumentViewport & | vp | ) |
Adds a bookmark for the given viewport vp
.
- Since
- 0.15 (KDE 4.9)
Definition at line 411 of file bookmarkmanager.cpp.
◆ addBookmark() [2/3]
bool BookmarkManager::addBookmark | ( | const QUrl & | documentUrl, |
const Okular::DocumentViewport & | vp, | ||
const QString & | title = QString() ) |
Adds a new bookmark for the documentUrl
at the specified viewport vp
, with an optional title
.
If no title
is specified, then #n will be used.
Definition at line 416 of file bookmarkmanager.cpp.
◆ addBookmark() [3/3]
void BookmarkManager::addBookmark | ( | int | page | ) |
Adds a bookmark for the given page
.
Definition at line 400 of file bookmarkmanager.cpp.
◆ bookmark() [1/2]
KBookmark BookmarkManager::bookmark | ( | const DocumentViewport & | viewport | ) | const |
Returns the bookmark for the given viewport
of the document.
- Since
- 0.15 (KDE 4.9)
Definition at line 323 of file bookmarkmanager.cpp.
◆ bookmark() [2/2]
KBookmark BookmarkManager::bookmark | ( | int | page | ) | const |
Returns the bookmark for the given page of the document.
- Since
- 0.14 (KDE 4.8)
Definition at line 311 of file bookmarkmanager.cpp.
◆ bookmarks() [1/3]
KBookmark::List BookmarkManager::bookmarks | ( | ) | const |
Returns the list of bookmarks for document.
- Since
- 0.14 (KDE 4.8)
Definition at line 292 of file bookmarkmanager.cpp.
◆ bookmarks() [2/3]
KBookmark::List BookmarkManager::bookmarks | ( | const QUrl & | documentUrl | ) | const |
Returns the list of bookmarks for the specified documentUrl
.
Definition at line 268 of file bookmarkmanager.cpp.
◆ bookmarks() [3/3]
KBookmark::List BookmarkManager::bookmarks | ( | int | page | ) | const |
Returns the list of bookmarks for the given page of the document.
- Since
- 0.15 (KDE 4.9)
Definition at line 297 of file bookmarkmanager.cpp.
◆ bookmarksChanged
|
signal |
The bookmarks for specified url
were changed.
- Since
- 0.7 (KDE 4.1)
◆ files()
Returns the list of documents with bookmarks.
Definition at line 254 of file bookmarkmanager.cpp.
◆ isBookmarked() [1/2]
bool BookmarkManager::isBookmarked | ( | const DocumentViewport & | viewport | ) | const |
Return whether the given viewport
is bookmarked.
- Since
- 0.15 (KDE 4.9)
Definition at line 693 of file bookmarkmanager.cpp.
◆ isBookmarked() [2/2]
bool BookmarkManager::isBookmarked | ( | int | page | ) | const |
Returns whether the given page
is bookmarked.
Definition at line 688 of file bookmarkmanager.cpp.
◆ nextBookmark()
KBookmark BookmarkManager::nextBookmark | ( | const DocumentViewport & | viewport | ) | const |
Given a viewport
, returns the next bookmark.
- Since
- 0.15 (KDE 4.9)
Definition at line 700 of file bookmarkmanager.cpp.
◆ openUrl
|
signal |
The bookmark manager is requesting to open the specified url
.
◆ previousBookmark()
KBookmark BookmarkManager::previousBookmark | ( | const DocumentViewport & | viewport | ) | const |
Given a viewport
, returns the previous bookmark.
- Since
- 0.15 (KDE 4.9)
Definition at line 717 of file bookmarkmanager.cpp.
◆ removeBookmark() [1/3]
void BookmarkManager::removeBookmark | ( | const DocumentViewport & | vp | ) |
Remove a bookmark for the given viewport vp
.
- Since
- 0.15 (KDE 4.9)
Definition at line 493 of file bookmarkmanager.cpp.
◆ removeBookmark() [2/3]
Removes the bookmark bm
for the documentUrl
specified.
Definition at line 542 of file bookmarkmanager.cpp.
◆ removeBookmark() [3/3]
void BookmarkManager::removeBookmark | ( | int | page | ) |
Remove a bookmark for the given page
.
Definition at line 485 of file bookmarkmanager.cpp.
◆ removeBookmarks()
void BookmarkManager::removeBookmarks | ( | const QUrl & | documentUrl, |
const KBookmark::List & | list ) |
Removes the bookmarks in list
for the documentUrl
specified.
- Note
- it will remove only the bookmarks which belong to
documentUrl
- Since
- 0.11 (KDE 4.5)
Definition at line 572 of file bookmarkmanager.cpp.
◆ renameBookmark() [1/2]
Renames the top-level bookmark for the documentUrl
specified with the newName
specified.
- Since
- 0.15 (KDE 4.9)
Definition at line 514 of file bookmarkmanager.cpp.
◆ renameBookmark() [2/2]
Returns the bookmark given bookmark of the document.
- Since
- 0.14 (KDE 4.8)
Definition at line 501 of file bookmarkmanager.cpp.
◆ save()
void BookmarkManager::save | ( | ) | const |
Forces to save the list of bookmarks.
Definition at line 349 of file bookmarkmanager.cpp.
◆ saved
|
signal |
This signal is emitted whenever bookmarks have been saved.
◆ titleForUrl()
Returns title for the documentUrl
.
- Since
- 0.15 (KDE 4.9)
Definition at line 533 of file bookmarkmanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:13:35 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.