KWindowStateSaver
#include <kwindowstatesaver.h>
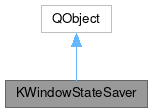
Public Member Functions | |
KWindowStateSaver (QWindow *window, const KConfigGroup &configGroup) | |
KWindowStateSaver (QWindow *window, const QString &configGroupName) | |
template<typename Widget> | |
KWindowStateSaver (Widget *widget, const KConfigGroup &configGroup) | |
template<typename Widget> | |
KWindowStateSaver (Widget *widget, const QString &configGroupName) | |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
Detailed Description
Saves and restores a window size and (when possible) position.
This is useful for retrofitting persisting window geometry on existing windows or dialogs, without having to modify those classes themselves, or having to inherit from them. For this, create a new instance of KWindowStateSaver for every window that should have it's state persisted, and pass it the window or widget as well as the config group the state should be stored in. The KWindowStateSaver will restore an existing state and then monitor the window for subsequent changes to persist. It will delete itself once the window is deleted.
Note that freshly created top-level QWidgets (such as the dialog in the above example) do not have an associated QWindow yet (ie. windowHandle() return nullptr
). KWindowStateSaver supports this with its QWidget constructors which will monitor the widget for having its associated QWindow created before continuing with that.
When implementing your own windows/dialogs, using KWindowConfig directly can be an alternative.
- See also
- KWindowConfig
- Since
- 5.92
Definition at line 46 of file kwindowstatesaver.h.
Constructor & Destructor Documentation
◆ KWindowStateSaver() [1/4]
|
explicit |
Create a new window state saver for window
.
- Parameters
-
configGroup A KConfigGroup that holds the window state.
Definition at line 65 of file kwindowstatesaver.cpp.
◆ KWindowStateSaver() [2/4]
Create a new window state saver for window
.
- Parameters
-
configGroupName The name of a KConfigGroup in the default state configuration (see KSharedConfig::openStateConfig) that holds the window state.
Definition at line 75 of file kwindowstatesaver.cpp.
◆ KWindowStateSaver() [3/4]
|
inlineexplicit |
Create a new window state saver for widget
.
Use this for widgets that aren't shown yet and would still return nullptr
from windowHandle().
- Parameters
-
configGroup A KConfigGroup that holds the window state.
Definition at line 94 of file kwindowstatesaver.h.
◆ KWindowStateSaver() [4/4]
|
inlineexplicit |
Create a new window state saver for widget
.
Use this for widgets that aren't shown yet and would still return nullptr
from windowHandle().
- Parameters
-
configGroupName The name of a KConfigGroup in the default state configuration (see KSharedConfig::openStateConfig) that holds the window state.
Definition at line 106 of file kwindowstatesaver.h.
◆ ~KWindowStateSaver()
KWindowStateSaver::~KWindowStateSaver | ( | ) |
Definition at line 85 of file kwindowstatesaver.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:04:35 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.