KKeySequenceRecorder
#include <KKeySequenceRecorder>
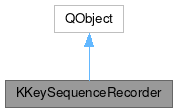
Properties | |
QKeySequence | currentKeySequence |
bool | isRecording |
bool | modifierlessAllowed |
bool | modifierOnlyAllowed |
bool | multiKeyShortcutsAllowed |
QWindow * | window |
![]() | |
objectName | |
Signals | |
void | currentKeySequenceChanged () |
void | gotKeySequence (const QKeySequence &keySequence) |
void | modifierlessAllowedChanged () |
void | modifierOnlyAllowedChanged () |
void | multiKeyShortcutsAllowedChanged () |
void | recordingChanged () |
void | windowChanged () |
Public Slots | |
void | cancelRecording () |
Public Member Functions | |
KKeySequenceRecorder (QWindow *window, QObject *parent=nullptr) | |
QKeySequence | currentKeySequence () const |
bool | isRecording () const |
bool | modifierlessAllowed () const |
bool | modifierOnlyAllowed () const |
bool | multiKeyShortcutsAllowed () const |
void | setCurrentKeySequence (const QKeySequence &sequence) |
void | setModifierlessAllowed (bool allowed) |
void | setModifierOnlyAllowed (bool allowed) |
void | setMultiKeyShortcutsAllowed (bool allowed) |
void | setWindow (QWindow *window) |
Q_INVOKABLE void | startRecording () |
QWindow * | window () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Record a QKeySequence by listening to key events in a window.
After calling startRecording key events in the set window will be captured until a valid QKeySequence has been recorded and gotKeySequence is emitted. See multiKeyShortcutsAllowed and modifierlessAllowed for what constitutes a valid key sequence.
During recording any shortcuts are inhibited and cannot be triggered. Either by using the keyboard-shortcuts-inhibit protocol on Wayland or grabbing the keyboard.
For graphical elements that record key sequences and can optionally perform conflict checking against existing shortcuts see KKeySequenceWidget and KeySequenceItem.
Porting from KF5 to KF6:
The class KeySequenceRecorder was renamed to KKeySequenceRecorder.
- See also
- KKeySequenceWidget, KeySequenceItem
- Since
- 6.0
Definition at line 45 of file kkeysequencerecorder.h.
Property Documentation
◆ currentKeySequence
|
readwrite |
The recorded key sequence.
After construction this is empty.
During recording it is continuously updated with the newest user input.
After recording it contains the last recorded QKeySequence
Definition at line 61 of file kkeysequencerecorder.h.
◆ isRecording
|
read |
Whether key events are currently recorded.
Definition at line 52 of file kkeysequencerecorder.h.
◆ modifierlessAllowed
|
readwrite |
If key presses of "plain" keys without a modifier are considered to be a valid finished key combination.
Plain keys include letter and symbol keys and text editing keys (Return, Space, Tab, Backspace, Delete). Other keys like F1, Cursor keys, Insert, PageDown will always work.
By default this is false
.
Definition at line 74 of file kkeysequencerecorder.h.
◆ modifierOnlyAllowed
|
readwrite |
It makes it acceptable for the key sequence to be just a modifier (e.g.
Shift or Control)
By default, if only a modifier is pressed and then released, the component will remain waiting for the sequence. When enabled, it will take the modifier key as the key sequence.
By default this is false
.
Definition at line 95 of file kkeysequencerecorder.h.
◆ multiKeyShortcutsAllowed
|
readwrite |
Controls the amount of key combinations that are captured until recording stops and gotKeySequence is emitted.
By default this is true
and "Emacs-style" key sequences are recorded. Recording does not stop until four valid key combination have been recorded. Afterwards currentKeySequence().count()
will be 4.
Otherwise only one key combination is recorded before gotKeySequence is emitted with a QKeySequence with a count()
of 1.
- See also
- QKeySequence
Definition at line 85 of file kkeysequencerecorder.h.
◆ window
|
readwrite |
The window in which the key events are happening that should be recorded.
Definition at line 65 of file kkeysequencerecorder.h.
Constructor & Destructor Documentation
◆ KKeySequenceRecorder()
|
explicit |
Constructor.
- window The window whose key events will be recorded.
- See also
- window
Definition at line 505 of file kkeysequencerecorder.cpp.
◆ ~KKeySequenceRecorder()
|
overridenoexcept |
Definition at line 517 of file kkeysequencerecorder.cpp.
Member Function Documentation
◆ cancelRecording
|
slot |
Stops the recording session.
Definition at line 548 of file kkeysequencerecorder.cpp.
◆ currentKeySequence()
QKeySequence KKeySequenceRecorder::currentKeySequence | ( | ) | const |
Definition at line 560 of file kkeysequencerecorder.cpp.
◆ gotKeySequence
|
signal |
This signal is emitted when a key sequence has been recorded.
Compared to currentKeySequenceChanged and currentKeySequence this is signal is not emitted continuously during recording but only after recording has finished.
◆ isRecording()
bool KKeySequenceRecorder::isRecording | ( | ) | const |
Definition at line 555 of file kkeysequencerecorder.cpp.
◆ modifierlessAllowed()
bool KKeySequenceRecorder::modifierlessAllowed | ( | ) | const |
Definition at line 627 of file kkeysequencerecorder.cpp.
◆ modifierOnlyAllowed()
bool KKeySequenceRecorder::modifierOnlyAllowed | ( | ) | const |
Definition at line 641 of file kkeysequencerecorder.cpp.
◆ multiKeyShortcutsAllowed()
bool KKeySequenceRecorder::multiKeyShortcutsAllowed | ( | ) | const |
Definition at line 613 of file kkeysequencerecorder.cpp.
◆ setCurrentKeySequence()
void KKeySequenceRecorder::setCurrentKeySequence | ( | const QKeySequence & | sequence | ) |
Definition at line 571 of file kkeysequencerecorder.cpp.
◆ setModifierlessAllowed()
void KKeySequenceRecorder::setModifierlessAllowed | ( | bool | allowed | ) |
Definition at line 632 of file kkeysequencerecorder.cpp.
◆ setModifierOnlyAllowed()
void KKeySequenceRecorder::setModifierOnlyAllowed | ( | bool | allowed | ) |
Definition at line 646 of file kkeysequencerecorder.cpp.
◆ setMultiKeyShortcutsAllowed()
void KKeySequenceRecorder::setMultiKeyShortcutsAllowed | ( | bool | allowed | ) |
Definition at line 618 of file kkeysequencerecorder.cpp.
◆ setWindow()
void KKeySequenceRecorder::setWindow | ( | QWindow * | window | ) |
Definition at line 585 of file kkeysequencerecorder.cpp.
◆ startRecording()
void KKeySequenceRecorder::startRecording | ( | ) |
Start recording.
Calling startRecording when window() is nullptr
has no effect.
Definition at line 524 of file kkeysequencerecorder.cpp.
◆ window()
QWindow * KKeySequenceRecorder::window | ( | ) | const |
Definition at line 580 of file kkeysequencerecorder.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:54:34 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.