KFileItemActions
#include <KFileItemActions>
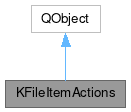
Public Types | |
enum class | MenuActionSource { Services = 0x1 , Plugins = 0x2 , All = Services | Plugins } |
typedef QFlags< MenuActionSource > | MenuActionSources |
Signals | |
void | error (const QString &errorMessage) |
void | openWithDialogAboutToBeShown () |
Public Slots | |
void | runPreferredApplications (const KFileItemList &fileOpenList) |
Public Member Functions | |
KFileItemActions (QObject *parent=nullptr) | |
~KFileItemActions () override | |
void | addActionsTo (QMenu *menu, MenuActionSources sources=MenuActionSource::All, const QList< QAction * > &additionalActions={}, const QStringList &excludeList={}) |
void | insertOpenWithActionsTo (QAction *before, QMenu *topMenu, const QStringList &excludedDesktopEntryNames) |
void | setItemListProperties (const KFileItemListProperties &itemList) |
void | setParentWidget (QWidget *widget) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static KService::List | associatedApplications (const QStringList &mimeTypeList) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class creates and handles the actions for a url (or urls) in a popupmenu.
This includes:
- "open with <application>" actions, but also
- user-defined actions for a .desktop file, defined in the file itself (see the desktop entry standard)
- servicemenus actions, defined in .desktop files and selected based on the MIME type of the url
KFileItemActions respects Kiosk-based restrictions (see the KAuthorized namespace in the KConfig framework). In particular, the "action/openwith" action is checked when determining actions for opening files (see addOpenWithActionsTo()) and service-specific actions are checked before adding service actions to a menu (see addServiceActionsTo()).
For user-defined actions in a .desktop file, the "X-KDE-AuthorizeAction" key can be used to determine which actions are checked before the user-defined action is allowed. The action is ignored if any of the listed actions are not authorized.
- Note
- : The builtin services like mount/unmount for old-style device desktop files (which mainly concerns CDROM and Floppy drives) have been deprecated since 5.82; those menu entries were hidden long before that, since the FSDevice .desktop template file hadn't been installed for quite a while.
Definition at line 49 of file kfileitemactions.h.
Member Typedef Documentation
◆ MenuActionSources
Definition at line 130 of file kfileitemactions.h.
Member Enumeration Documentation
◆ MenuActionSource
|
strong |
Enumerator | |
---|---|
Services | Add user defined actions and servicemenu actions (this used to include builtin actions, which have been deprecated since 5.82 see class API documentation) |
Plugins | Add actions implemented by plugins. See KAbstractFileItemActionPlugin base class. |
Definition at line 124 of file kfileitemactions.h.
Constructor & Destructor Documentation
◆ KFileItemActions()
KFileItemActions::KFileItemActions | ( | QObject * | parent = nullptr | ) |
Creates a KFileItemActions instance.
Note that this instance must stay alive for at least as long as the popupmenu; it has the slots for the actions created by addOpenWithActionsTo/addServiceActionsTo.
Definition at line 237 of file kfileitemactions.cpp.
◆ ~KFileItemActions()
|
overridedefault |
Destructor.
Member Function Documentation
◆ addActionsTo()
void KFileItemActions::addActionsTo | ( | QMenu * | menu, |
MenuActionSources | sources = MenuActionSource::All, | ||
const QList< QAction * > & | additionalActions = {}, | ||
const QStringList & | excludeList = {} ) |
This methods adds additional actions to the menu.
- Parameters
-
menu Menu to which the actions/submenus will be added. sources sources from which the actions should be fetched. By default all sources are used. additionalActions additional actions that should be added to the "Actions" submenu or top level menu if there are less than three entries in total. excludeList list of action names or plugin ids that should be excluded
- Since
- 5.77
Definition at line 258 of file kfileitemactions.cpp.
◆ associatedApplications()
|
static |
Returns the applications associated with all the given MIME types.
This is basically a KApplicationTrader::query, but it supports multiple MIME types, and also cleans up "apparent" duplicates, such as different versions of the same application installed in parallel.
The list is sorted according to the user preferences for the given MIME type(s). In case multiple MIME types appear in the URL list, the logic is: applications that on average appear earlier on the associated applications list for the given MIME types also appear earlier on the final applications list.
Note that for a single MIME type there is no need to use this, you should use KApplicationTrader instead, e.g. query() or preferredService().
This will return an empty list if the "openwith" Kiosk action is not authorized (see KAuthorized::authorize()
).
- Parameters
-
mimeTypeList the MIME types
- Returns
- the sorted list of services.
- Since
- 5.83
Definition at line 275 of file kfileitemactions.cpp.
◆ error
|
signal |
Forwards the errors from the KAbstractFileItemActionPlugin instances.
- Since
- 5.82
◆ insertOpenWithActionsTo()
void KFileItemActions::insertOpenWithActionsTo | ( | QAction * | before, |
QMenu * | topMenu, | ||
const QStringList & | excludedDesktopEntryNames ) |
Generates the "Open With <Application>" actions, and inserts them in menu
, before action before
.
If before
is nullptr or doesn't exist in the menu the actions will be appended to the menu.
All actions are created as children of the menu.
No actions will be added if the "openwith" Kiosk action is not authorized (see KAuthorized::authorize()).
- Parameters
-
before the "open with" actions will be inserted before this action; if this action is nullptr or isn't available in topMenu
, the "open with" actions will be appendedmenu the QMenu where the actions will be added excludedDesktopEntryNames list of desktop entry names that will not be shown
- Since
- 5.82
Definition at line 288 of file kfileitemactions.cpp.
◆ openWithDialogAboutToBeShown
|
signal |
Emitted before the "Open With" dialog is shown This is used e.g in folderview to close the folder peek popups on invoking the "Open With" menu action.
- Since
- 4.8.2
◆ runPreferredApplications
|
slot |
Slot used to execute a list of files in their respective preferred application.
- Parameters
-
fileOpenList the list of KFileItems to open.
- Since
- 5.83
Definition at line 322 of file kfileitemactions.cpp.
◆ setItemListProperties()
void KFileItemActions::setItemListProperties | ( | const KFileItemListProperties & | itemList | ) |
Sets all the data for the next instance of the popupmenu.
- See also
- KFileItemListProperties
Definition at line 245 of file kfileitemactions.cpp.
◆ setParentWidget()
void KFileItemActions::setParentWidget | ( | QWidget * | widget | ) |
Set the parent widget for any dialogs being shown.
This should normally be your mainwindow, not a popup menu, so that it still exists even after the popup is closed (e.g. error message from KRun) and so that QAction::setStatusTip can find a statusbar, too.
Definition at line 843 of file kfileitemactions.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:24 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.