KFileItemDelegate
#include <KFileItemDelegate>
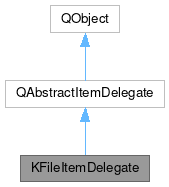
Public Types | |
enum | Information { NoInformation , Size , Permissions , OctalPermissions , Owner , OwnerAndGroup , CreationTime , ModificationTime , AccessTime , MimeType , FriendlyMimeType , LinkDest , LocalPathOrUrl , Comment } |
typedef QList< Information > | InformationList |
![]() | |
enum | EndEditHint |
![]() | |
typedef | QObjectList |
Properties | |
InformationList | information |
bool | jobTransfersVisible |
QSize | maximumSize |
qreal | shadowBlur |
QColor | shadowColor |
QPointF | shadowOffset |
bool | showToolTipWhenElided |
![]() | |
objectName | |
Public Slots | |
bool | helpEvent (QHelpEvent *event, QAbstractItemView *view, const QStyleOptionViewItem &option, const QModelIndex &index) override |
QRegion | shape (const QStyleOptionViewItem &option, const QModelIndex &index) |
Public Member Functions | |
KFileItemDelegate (QObject *parent=nullptr) | |
~KFileItemDelegate () override | |
QWidget * | createEditor (QWidget *parent, const QStyleOptionViewItem &option, const QModelIndex &index) const override |
bool | editorEvent (QEvent *event, QAbstractItemModel *model, const QStyleOptionViewItem &option, const QModelIndex &index) override |
bool | eventFilter (QObject *object, QEvent *event) override |
QRect | iconRect (const QStyleOptionViewItem &option, const QModelIndex &index) const |
bool | jobTransfersVisible () const |
QSize | maximumSize () const |
void | paint (QPainter *painter, const QStyleOptionViewItem &option, const QModelIndex &index) const override |
void | setEditorData (QWidget *editor, const QModelIndex &index) const override |
void | setJobTransfersVisible (bool jobTransfersVisible) |
void | setMaximumSize (const QSize &size) |
void | setModelData (QWidget *editor, QAbstractItemModel *model, const QModelIndex &index) const override |
void | setShadowBlur (qreal radius) |
void | setShadowColor (const QColor &color) |
void | setShadowOffset (const QPointF &offset) |
void | setShowInformation (const InformationList &list) |
void | setShowInformation (Information information) |
void | setShowToolTipWhenElided (bool showToolTip) |
void | setWrapMode (QTextOption::WrapMode wrapMode) |
qreal | shadowBlur () const |
QColor | shadowColor () const |
QPointF | shadowOffset () const |
InformationList | showInformation () const |
bool | showToolTipWhenElided () const |
QSize | sizeHint (const QStyleOptionViewItem &option, const QModelIndex &index) const override |
void | updateEditorGeometry (QWidget *editor, const QStyleOptionViewItem &option, const QModelIndex &index) const override |
QTextOption::WrapMode | wrapMode () const |
![]() | |
QAbstractItemDelegate (QObject *parent) | |
void | closeEditor (QWidget *editor, QAbstractItemDelegate::EndEditHint hint) |
void | commitData (QWidget *editor) |
virtual void | destroyEditor (QWidget *editor, const QModelIndex &index) const const |
virtual bool | helpEvent (QHelpEvent *event, QAbstractItemView *view, const QStyleOptionViewItem &option, const QModelIndex &index) |
void | sizeHintChanged (const QModelIndex &index) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
EditNextItem | |
EditPreviousItem | |
NoHint | |
RevertModelCache | |
SubmitModelCache | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
KFileItemDelegate is intended to be used to provide a KDE file system view, when using one of the standard item views in Qt with KDirModel.
While primarily intended to be used with KDirModel, it uses Qt::DecorationRole and Qt::DisplayRole for the icons and text labels, just like QItemDelegate, and can thus be used with any standard model.
When used with KDirModel however, KFileItemDelegate can change the way the display and/or decoration roles are drawn, based on properties of the file items. For example, if the file item is a symbolic link, it will use an italic font to draw the file name.
KFileItemDelegate also supports showing additional information about the file items below the icon labels.
Which information should be shown, if any, is controlled by the information property, which is a list that can be set by calling setShowInformation(), and read by calling showInformation(). By default this list is empty.
To use KFileItemDelegate, instantiate an object from the delegate, and call setItemDelegate() in one of the standard item views in Qt:
Definition at line 55 of file kfileitemdelegate.h.
Member Typedef Documentation
◆ InformationList
Definition at line 164 of file kfileitemdelegate.h.
Member Enumeration Documentation
◆ Information
This enum defines the additional information that can be displayed below item labels in icon views.
The information will only be shown for indexes for which the model provides a valid value for KDirModel::FileItemRole, and only when there's sufficient vertical space to display at least one line of the information, along with the display label.
For the number of items to be shown for folders, the model must provide a valid value for KDirMode::ChildCountRole, in addition to KDirModel::FileItemRole.
Note that KFileItemDelegate will not call KFileItem::determineMimeType() if KFileItem::isMimeTypeKnown() returns false, so if you want to display MIME types you should use a KMimeTypeResolver with the model and the view, to ensure that MIME types are resolved. If the MIME type isn't known, "Unknown" will be displayed until the MIME type has been successfully resolved.
Enumerator | |
---|---|
NoInformation | No additional information will be shown for items. |
Size | The file size for files, and the number of items for folders. |
Permissions | A UNIX permissions string, e.g. -rwxr-xr-x. |
OctalPermissions | The permissions as an octal value, e.g. 0644. |
Owner | The user name of the file owner, e.g. root. |
OwnerAndGroup | The user and group that owns the file, e.g. root:root. |
CreationTime | The date and time the file/folder was created. |
ModificationTime | The date and time the file/folder was last modified. |
AccessTime | The date and time the file/folder was last accessed. |
MimeType | The MIME type for the item, e.g. text/html. |
FriendlyMimeType | The descriptive name for the MIME type, e.g. HTML Document. |
LinkDest | The destination of a symbolic link.
|
LocalPathOrUrl | The local path to the file or the URL in case it is not a local file.
|
Comment | A simple comment that can be displayed to the user as is.
|
Definition at line 146 of file kfileitemdelegate.h.
Property Documentation
◆ information
|
readwrite |
This property holds which additional information (if any) should be shown below items in icon views.
Access functions:
- void setShownformation(InformationList information)
- InformationList showInformation() const
Definition at line 67 of file kfileitemdelegate.h.
◆ jobTransfersVisible
|
readwrite |
This property determines if there are KIO jobs on a destination URL visible, then they will have a small animation overlay displayed on them.
Definition at line 122 of file kfileitemdelegate.h.
◆ maximumSize
|
readwrite |
This property holds the maximum size that can be returned by KFileItemDelegate::sizeHint().
If the maximum size is empty, it will be ignored.
Definition at line 107 of file kfileitemdelegate.h.
◆ shadowBlur
|
readwrite |
This property holds the blur radius for the text shadow.
The default value for this property is 2.
Access functions:
- void setShadowBlur(qreal radius)
- qreal shadowBlur() const
Definition at line 100 of file kfileitemdelegate.h.
◆ shadowColor
|
readwrite |
This property holds the color used for the text shadow.
The alpha value in the color determines the opacity of the shadow. Shadows are only rendered when the alpha value is non-zero. The default value for this property is Qt::transparent.
Access functions:
Definition at line 80 of file kfileitemdelegate.h.
◆ shadowOffset
|
readwrite |
This property holds the horizontal and vertical offset for the text shadow.
The default value for this property is (1, 1).
Access functions:
Definition at line 90 of file kfileitemdelegate.h.
◆ showToolTipWhenElided
|
readwrite |
This property determines whether a tooltip will be shown by the delegate if the display role is elided.
This tooltip will contain the full display role information. The tooltip will only be shown if the Qt::ToolTipRole differs from Qt::DisplayRole, or if they match, showToolTipWhenElided flag is set and the display role information is elided.
Definition at line 116 of file kfileitemdelegate.h.
Constructor & Destructor Documentation
◆ KFileItemDelegate()
|
explicit |
Constructs a new KFileItemDelegate.
- Parameters
-
parent The parent object for the delegate.
Definition at line 808 of file kfileitemdelegate.cpp.
◆ ~KFileItemDelegate()
|
overridedefault |
Destroys the item delegate.
Member Function Documentation
◆ createEditor()
|
overridevirtual |
Reimplemented from QAbstractItemDelegate.
Reimplemented from QAbstractItemDelegate.
Definition at line 1318 of file kfileitemdelegate.cpp.
◆ editorEvent()
|
overridevirtual |
Reimplemented from QAbstractItemDelegate.
Reimplemented from QAbstractItemDelegate.
Definition at line 1332 of file kfileitemdelegate.cpp.
◆ eventFilter()
Reimplemented from QAbstractItemDelegate.
Reimplemented from QObject.
Definition at line 1500 of file kfileitemdelegate.cpp.
◆ helpEvent
|
overrideslot |
Reimplemented from QAbstractItemDelegate.
Definition at line 1416 of file kfileitemdelegate.cpp.
◆ iconRect()
QRect KFileItemDelegate::iconRect | ( | const QStyleOptionViewItem & | option, |
const QModelIndex & | index ) const |
Returns the rectangle of the icon that is aligned inside the decoration rectangle.
Definition at line 974 of file kfileitemdelegate.cpp.
◆ jobTransfersVisible()
bool KFileItemDelegate::jobTransfersVisible | ( | ) | const |
Returns whether or not the displaying of job transfers is enabled.
- See also
- setJobTransfersVisible()
Definition at line 990 of file kfileitemdelegate.cpp.
◆ maximumSize()
QSize KFileItemDelegate::maximumSize | ( | ) | const |
Returns the maximum size for KFileItemDelegate::sizeHint().
- See also
- setMaximumSize()
Definition at line 949 of file kfileitemdelegate.cpp.
◆ paint()
|
overridevirtual |
Paints the item indicated by index
, using painter
.
The item will be drawn in the rectangle specified by option.rect. The correct size for that rectangle can be obtained by calling sizeHint().
This function will use the following data values if the model provides them for the item, in place of the values in option:
- Qt::FontRole The font that should be used for the display role.
- Qt::TextAlignmentRole The alignment of the display role.
- Qt::ForegroundRole The text color for the display role.
- Qt::BackgroundRole The background color for the item.
This function is reimplemented from QAbstractItemDelegate.
- Parameters
-
painter The painter with which to draw the item. option The style options that should be used when painting the item. index The index to the item that should be painted.
Implements QAbstractItemDelegate.
Definition at line 1113 of file kfileitemdelegate.cpp.
◆ setEditorData()
|
overridevirtual |
Reimplemented from QAbstractItemDelegate.
Reimplemented from QAbstractItemDelegate.
Definition at line 1342 of file kfileitemdelegate.cpp.
◆ setJobTransfersVisible()
void KFileItemDelegate::setJobTransfersVisible | ( | bool | jobTransfersVisible | ) |
Enable/Disable the displaying of an animated overlay that is shown for any destination urls (in the view).
When enabled, the animations (if any) will be drawn automatically.
Only the files/folders that are visible and have jobs associated with them will display the animation. You would likely not want this enabled if you perform some kind of custom painting that takes up a whole item, and will just make this(and what you paint) look funky.
Default is disabled.
Note: The model (KDirModel) needs to have it's method called with the same value, when you make the call to this method.
Definition at line 984 of file kfileitemdelegate.cpp.
◆ setMaximumSize()
void KFileItemDelegate::setMaximumSize | ( | const QSize & | size | ) |
Sets the maximum size for KFileItemDelegate::sizeHint().
- See also
- maximumSize()
Definition at line 944 of file kfileitemdelegate.cpp.
◆ setModelData()
|
overridevirtual |
Reimplemented from QAbstractItemDelegate.
Reimplemented from QAbstractItemDelegate.
Definition at line 1373 of file kfileitemdelegate.cpp.
◆ setShadowBlur()
void KFileItemDelegate::setShadowBlur | ( | qreal | radius | ) |
Sets the blur radius for the text shadow.
- See also
- shadowBlur()
Definition at line 934 of file kfileitemdelegate.cpp.
◆ setShadowColor()
void KFileItemDelegate::setShadowColor | ( | const QColor & | color | ) |
Sets the color used for drawing the text shadow.
To enable text shadows, set the shadow color to a non-transparent color. To disable text shadows, set the color to Qt::transparent.
- See also
- shadowColor()
Definition at line 914 of file kfileitemdelegate.cpp.
◆ setShadowOffset()
void KFileItemDelegate::setShadowOffset | ( | const QPointF & | offset | ) |
Sets the horizontal and vertical offset for the text shadow.
- See also
- shadowOffset()
Definition at line 924 of file kfileitemdelegate.cpp.
◆ setShowInformation() [1/2]
void KFileItemDelegate::setShowInformation | ( | const InformationList & | list | ) |
Sets the list of information lines that are shown below the icon label in list views.
You will typically construct the list like this:
The information lines will be displayed in the list order. The delegate will first draw the item label, and then as many information lines as will fit in the available space.
- Parameters
-
list A list of information items that should be shown
Definition at line 895 of file kfileitemdelegate.cpp.
◆ setShowInformation() [2/2]
void KFileItemDelegate::setShowInformation | ( | Information | information | ) |
Sets a single information line that is shown below the icon label in list views.
This is a convenience function for when you only want to show a single line of information.
- Parameters
-
information The information that should be shown
Definition at line 900 of file kfileitemdelegate.cpp.
◆ setShowToolTipWhenElided()
void KFileItemDelegate::setShowToolTipWhenElided | ( | bool | showToolTip | ) |
Sets whether a tooltip should be shown if the display role is elided containing the full display role information.
- Note
- The tooltip will only be shown if the Qt::ToolTipRole differs from Qt::DisplayRole, or if they match, showToolTipWhenElided flag is set and the display role information is elided.
- See also
- showToolTipWhenElided()
Definition at line 954 of file kfileitemdelegate.cpp.
◆ setWrapMode()
void KFileItemDelegate::setWrapMode | ( | QTextOption::WrapMode | wrapMode | ) |
When the contents text needs to be wrapped, wrapMode
strategy will be followed.
Definition at line 964 of file kfileitemdelegate.cpp.
◆ shadowBlur()
qreal KFileItemDelegate::shadowBlur | ( | ) | const |
Returns the blur radius for the text shadow.
- See also
- setShadowBlur()
Definition at line 939 of file kfileitemdelegate.cpp.
◆ shadowColor()
QColor KFileItemDelegate::shadowColor | ( | ) | const |
Returns the color used for the text shadow.
- See also
- setShadowColor()
Definition at line 919 of file kfileitemdelegate.cpp.
◆ shadowOffset()
QPointF KFileItemDelegate::shadowOffset | ( | ) | const |
Returns the offset used for the text shadow.
- See also
- setShadowOffset()
Definition at line 929 of file kfileitemdelegate.cpp.
◆ shape
|
slot |
Returns the shape of the item as a region.
The returned region can be used for precise hit testing of the item.
Definition at line 1456 of file kfileitemdelegate.cpp.
◆ showInformation()
KFileItemDelegate::InformationList KFileItemDelegate::showInformation | ( | ) | const |
Returns the file item information that should be shown below item labels in list views.
Definition at line 909 of file kfileitemdelegate.cpp.
◆ showToolTipWhenElided()
bool KFileItemDelegate::showToolTipWhenElided | ( | ) | const |
Returns whether a tooltip should be shown if the display role is elided containing the full display role information.
- Note
- The tooltip will only be shown if the Qt::ToolTipRole differs from Qt::DisplayRole, or if they match, showToolTipWhenElided flag is set and the display role information is elided.
- See also
- setShowToolTipWhenElided()
Definition at line 959 of file kfileitemdelegate.cpp.
◆ sizeHint()
|
overridevirtual |
Returns the nominal size for the item referred to by index
, given the provided options.
If the model provides a valid Qt::FontRole and/or Qt::TextAlignmentRole for the item, those will be used instead of the ones specified in the style options.
This function is reimplemented from QAbstractItemDelegate.
- Parameters
-
option The style options that should be used when painting the item. index The index to the item for which to return the size hint.
Implements QAbstractItemDelegate.
Definition at line 836 of file kfileitemdelegate.cpp.
◆ updateEditorGeometry()
|
overridevirtual |
Reimplemented from QAbstractItemDelegate.
Reimplemented from QAbstractItemDelegate.
Definition at line 1381 of file kfileitemdelegate.cpp.
◆ wrapMode()
QTextOption::WrapMode KFileItemDelegate::wrapMode | ( | ) | const |
Returns the wrapping strategy followed to show text when it needs wrapping.
Definition at line 969 of file kfileitemdelegate.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:56:14 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.