KTextEditor::Command
#include <KTextEditor/Command>
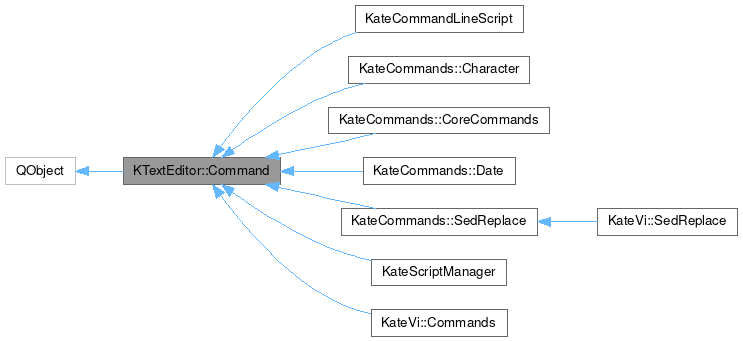
Public Member Functions | |
Command (const QStringList &cmds, QObject *parent=nullptr) | |
~Command () override | |
const QStringList & | cmds () const |
virtual KCompletion * | completionObject (KTextEditor::View *view, const QString &cmdname) |
virtual bool | exec (KTextEditor::View *view, const QString &cmd, QString &msg, const KTextEditor::Range &range=KTextEditor::Range::invalid())=0 |
virtual bool | help (KTextEditor::View *view, const QString &cmd, QString &msg)=0 |
virtual void | processText (KTextEditor::View *view, const QString &text) |
virtual bool | supportsRange (const QString &cmd) |
virtual bool | wantsToProcessText (const QString &cmdname) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
An Editor command line command.
Introduction
The Command class represents a command for the editor command line. A command simply consists of a string, for example find. The command auto-registers itself at the Editor. The Editor itself queries the command for a list of accepted strings/commands by calling cmds(). If the command gets invoked the function exec() is called, i.e. you have to implement the reaction in exec(). Whenever the user needs help for a command help() is called.
Command Information
To provide reasonable information about a specific command there are the following accessor functions for a given command string:
- name() returns a label
- description() returns a descriptive text
- category() returns a category into which the command fits.
These getters allow KTextEditor implementations to plug commands into menus and toolbars, so that a user can assign shortcuts.
Command Completion
The Command optionally can show a completion popup to help the user select a valid entry as first parameter to the Command. To this end, return a valid completion item by reiplementing completionObject().
The returned completion object is deleted automatically once it is not needed anymore. Therefore neither delete the completion object yourself nor return the same completion object twice.
Interactive Commands
In case the Command needs to interactively process the text of the parameters, override wantsToProcessText() by returning true and reimplement processText().
A typical example of an iterative command would be the incremental search.
- See also
- KTextEditor::CommandInterface
Definition at line 70 of file include/ktexteditor/command.h.
Constructor & Destructor Documentation
◆ Command()
Command::Command | ( | const QStringList & | cmds, |
QObject * | parent = nullptr ) |
Constructor with parent
.
Will register this command for the commands names given in cmds
at the global editor instance.
Definition at line 347 of file ktexteditor.cpp.
◆ ~Command()
|
override |
Virtual destructor.
Will unregister this command at the global editor instance.
Member Function Documentation
◆ cmds()
|
inline |
Return a list of strings a command may begin with.
This is the same list the command was constructed with. A string is the start part of a pure text which can be handled by this command, i.e. for the command s/sdl/sdf/g the corresponding string is simply s, and for char:1212 simply char.
- Returns
- list of supported commands
Definition at line 96 of file include/ktexteditor/command.h.
◆ completionObject()
|
virtual |
Return a KCompletion object that will substitute the command line default one while typing the first argument of the command cmdname
.
The text will be added to the command separated by one space character.
Override this method if your command can provide a completion object. The returned completion object is deleted automatically once it is not needed anymore. Therefore neither delete the completion object yourself nor return the same completion object twice.
The default implementation returns a null pointer (nullptr).
- Parameters
-
view the view the command will work on cmdname the command name associated with this request.
- Returns
- a valid completion object or nullptr, if a completion object is not supported
Reimplemented in KateCommands::CoreCommands, and KateVi::Commands.
Definition at line 369 of file ktexteditor.cpp.
◆ exec()
|
pure virtual |
Execute the command for the given view
and cmd
string.
Return the success value and a msg
for status. As example we consider a replace command. The replace command would return the number of replaced strings as msg
, like "16 replacements made." If an error occurred in the usage it would return false and set the msg
to something like "missing argument." or such.
If a non-invalid range is given, the command shall be executed on that range. supportsRange() tells if the command supports that.
- Returns
- true on success, otherwise false
Implemented in KateCommandLineScript, KateCommands::Character, KateCommands::CoreCommands, KateCommands::Date, KateCommands::SedReplace, KateScriptManager, and KateVi::Commands.
◆ help()
|
pure virtual |
Shows help for the given view
and cmd
string.
If your command has a help text for cmd
you have to return true and set the msg
to a meaningful text. The help text is embedded by the Editor in a Qt::RichText enabled widget, e.g. a QToolTip.
- Returns
- true if your command has a help text, otherwise false
Implemented in KateCommandLineScript, KateCommands::Character, KateCommands::CoreCommands, KateCommands::Date, KateCommands::SedReplace, KateScriptManager, and KateVi::Commands.
◆ processText()
|
virtual |
This is called by the command line each time the argument text for the command changed, if wantsToProcessText() returns true.
- Parameters
-
view the current view text the current command text typed by the user
- See also
- wantsToProcessText()
Definition at line 379 of file ktexteditor.cpp.
◆ supportsRange()
|
virtual |
Find out if a given command can act on a range.
This is used for checking if a command should be called when the user also gave a range or if an error should be raised.
- Returns
- true if command supports acting on a range of lines, false not. The default implementation returns false.
Reimplemented in KateCommandLineScript, KateCommands::CoreCommands, KateCommands::SedReplace, and KateVi::Commands.
Definition at line 364 of file ktexteditor.cpp.
◆ wantsToProcessText()
|
virtual |
Check, whether the command wants to process text interactively for the given command with name cmdname
.
If you return true, the command's processText() method is called whenever the text in the command line changed.
Reimplement this to return true, if your commands wants to process the text while typing.
- Parameters
-
cmdname the command name associated with this query.
- Returns
- true, if your command wants to process text interactively, otherwise false
- See also
- processText()
Definition at line 374 of file ktexteditor.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:56:22 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.