KTextEditor::Editor
#include <KTextEditor/Editor>
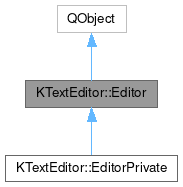
Public Types | |
using | ExpandFunction = QString (*)(const QStringView &text, KTextEditor::View *view) |
Signals | |
void | configChanged (KTextEditor::Editor *editor) |
void | documentCreated (KTextEditor::Editor *editor, KTextEditor::Document *document) |
void | repositoryReloaded (KTextEditor::Editor *editor) |
Public Member Functions | |
virtual const KAboutData & | aboutData () const =0 |
void | addVariableExpansion (const QList< QWidget * > &widgets, const QStringList &variables=QStringList()) const |
virtual KTextEditor::Application * | application () const =0 |
virtual QStringList | commandList () const =0 |
virtual QList< Command * > | commands () const =0 |
virtual void | configDialog (QWidget *parent)=0 |
virtual ConfigPage * | configPage (int number, QWidget *parent)=0 |
virtual int | configPages () const =0 |
virtual Document * | createDocument (QObject *parent)=0 |
QString | defaultEncoding () const |
virtual QList< Document * > | documents ()=0 |
QString | expandText (const QString &text, KTextEditor::View *view) const |
bool | expandVariable (const QString &variable, KTextEditor::View *view, QString &output) const |
QFont | font () const |
virtual Command * | queryCommand (const QString &cmd) const =0 |
bool | registerVariableMatch (const QString &name, const QString &description, ExpandFunction expansionFunc) |
bool | registerVariablePrefix (const QString &prefix, const QString &description, ExpandFunction expansionFunc) |
const KSyntaxHighlighting::Repository & | repository () const |
virtual void | setApplication (KTextEditor::Application *application)=0 |
KSyntaxHighlighting::Theme | theme () const |
bool | unregisterVariable (const QString &variableName) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static Editor * | instance () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
Editor (EditorPrivate *impl) | |
~Editor () override | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
Detailed Description
Accessor interface for the KTextEditor framework.
Topics:
Introduction
The Editor part can either be accessed through the static accessor Editor::instance() or through the KParts component model (see Using KTextEditor as KPart). The Editor singleton provides general information and configuration methods for the Editor, for example KAboutData by using aboutData().
The Editor has a list of all opened documents. Get this list with documents(). To create a new Document call createDocument(). The signal documentCreated() is emitted whenever the Editor created a new document.
Editor Configuration
The config dialog can be shown with configDialog(). Instead of using the config dialog, the config pages can also be embedded into the application's config dialog. To do this, configPages() returns the number of config pages that exist and configPage() returns the requested page. The configuration are saved automatically by the Editor.
- Note
- It is recommended to embed the config pages into the main application's config dialog instead of using a separate config dialog, if the config dialog does not look cluttered then. This way, all settings are grouped together in one place.
Command Line Commands
With Commands it is possible to add new commands to the command line. These Commands then are added to all document Views. Common use cases include commands like find or setting document variables. The list of all registered commands can be obtained either through commandList() or through commands(). Further, a specific command can be obtained through queryCommand(). For further information, read the Command API documentation.
Member Typedef Documentation
◆ ExpandFunction
using KTextEditor::Editor::ExpandFunction = QString (*)(const QStringView &text, KTextEditor::View *view) |
Constructor & Destructor Documentation
◆ Editor()
|
protected |
Constructor.
Create the Editor object and pass it the internal implementation to store a d-pointer.
- Parameters
-
impl d-pointer to use
Definition at line 81 of file ktexteditor.cpp.
◆ ~Editor()
|
overrideprotecteddefault |
Virtual destructor.
Member Function Documentation
◆ aboutData()
|
pure virtual |
Get the about data of this Editor part.
- Returns
- about data
Implemented in KTextEditor::EditorPrivate.
◆ addVariableExpansion()
void Editor::addVariableExpansion | ( | const QList< QWidget * > & | widgets, |
const QStringList & | variables = QStringList() ) const |
Adds a QAction to the widget in widgets
that whenever focus is gained.
When the action is invoked, a non-modal dialog is shown that lists all variables
. If variables
is non-empty, then only the variables in variables
are listed.
The supported QWidgets in the widgets
argument currently are:
- Since
- 5.63
Definition at line 128 of file ktexteditor.cpp.
◆ application()
|
pure virtual |
Current hosting application, if any set.
- Returns
- current application object or a dummy interface that allows you to call the functions will never return a nullptr
Implemented in KTextEditor::EditorPrivate.
◆ commandList()
|
pure virtual |
Get a list of available command line strings.
- Returns
- command line strings
- See also
- commands()
Implemented in KTextEditor::EditorPrivate.
◆ commands()
Get a list of all registered commands.
- Returns
- list of all commands
- See also
- queryCommand(), commandList()
Implemented in KTextEditor::EditorPrivate.
◆ configChanged
|
signal |
This signal is emitted whenever the editor configuration is changed.
- Parameters
-
editor the editor which's config has changed
- Since
- 5.79
◆ configDialog()
|
pure virtual |
Show the editor's config dialog, changes will be applied to the editor and the configuration changes are saved.
- Note
- Instead of using the config dialog, the config pages can be embedded into your own config dialog by using configPages() and configPage().
- Parameters
-
parent parent widget
Implemented in KTextEditor::EditorPrivate.
◆ configPage()
|
pure virtual |
Get the config page with the number
, config pages from 0 to configPages()-1 are available if configPages() > 0.
Configuration changes done over this widget are automatically saved.
- Parameters
-
number index of config page parent parent widget for config page
- Returns
- created config page or NULL, if the number is out of bounds
- See also
- configPages()
Implemented in KTextEditor::EditorPrivate.
◆ configPages()
|
pure virtual |
Get the number of available config pages.
If a number < 1 is returned, it does not support config pages.
- Returns
- number of config pages
- See also
- configPage()
Implemented in KTextEditor::EditorPrivate.
◆ createDocument()
Create a new document object with parent
.
For each created document, the signal documentCreated() is emitted.
- Parameters
-
parent parent object
- Returns
- new KTextEditor::Document object
- See also
- documents(), documentCreated()
Implemented in KTextEditor::EditorPrivate.
◆ defaultEncoding()
QString Editor::defaultEncoding | ( | ) | const |
Get the current default encoding for this Editor part.
- Returns
- default encoding
Definition at line 95 of file ktexteditor.cpp.
◆ documentCreated
|
signal |
The editor
emits this signal whenever a document
was successfully created.
- Parameters
-
editor pointer to the Editor singleton which created the new document document the newly created document instance
- See also
- createDocument()
◆ documents()
Get a list of all documents of this editor.
- Returns
- list of all existing documents
- See also
- createDocument()
Implemented in KTextEditor::EditorPrivate.
◆ expandText()
QString Editor::expandText | ( | const QString & | text, |
KTextEditor::View * | view ) const |
Expands arbitrary text
that may contain arbitrary many variables.
On success, the expanded text is written to output
.
- Since
- 6.0
Definition at line 123 of file ktexteditor.cpp.
◆ expandVariable()
bool Editor::expandVariable | ( | const QString & | variable, |
KTextEditor::View * | view, | ||
QString & | output ) const |
Expands a single variable
, writing the expanded value to output
.
- Returns
- true on success, otherwise false.
- Since
- 5.57
Definition at line 118 of file ktexteditor.cpp.
◆ font()
QFont Editor::font | ( | ) | const |
Get the current global editor font.
Might change during runtime, configChanged() will be emitted in that cases. Individual views might have set different fonts, can be queried with the "font" key via
- See also
- KTextEditor::ConfigInterface::configValue().
- Returns
- current global font for all views
- Since
- 5.80
Definition at line 133 of file ktexteditor.cpp.
◆ instance()
|
static |
Accessor to get the Editor instance.
- Note
- This object will stay alive until QCoreApplication terminates. You shall not delete it yourself. There is only ONE Editor instance of this per process.
- Returns
- Editor controller, after initial construction, will live until QCoreApplication is terminating.
Definition at line 89 of file ktexteditor.cpp.
◆ queryCommand()
Query for the command cmd
.
If the command cmd
does not exist the return value is NULL.
- Parameters
-
cmd name of command to query for
- Returns
- the found command or NULL if no such command exists
Implemented in KTextEditor::EditorPrivate.
◆ registerVariableMatch()
bool Editor::registerVariableMatch | ( | const QString & | name, |
const QString & | description, | ||
ExpandFunction | expansionFunc ) |
Registers a variable called name
for exact matches.
For instance, a variable called "CurrentDocument:Path" could be registered which then expands to the path the current document.
- Returns
- true on success, false if the variable could not be registered, e.g. because it already was registered previously.
- Since
- 5.57
Definition at line 101 of file ktexteditor.cpp.
◆ registerVariablePrefix()
bool Editor::registerVariablePrefix | ( | const QString & | prefix, |
const QString & | description, | ||
ExpandFunction | expansionFunc ) |
Registers a variable for arbitrary text that matches the specified prefix.
For instance, a variable called "ENV:" could be registered which then expands arbitrary environment variables, e.g. ENV:HOME would expand to the user's home directory.
- Note
- A colon ':' is used as separator for the prefix and the text after the colon that should be evaluated.
- Returns
- true on success, false if a prefix could not be registered, e.g. because it already was registered previously.
- Since
- 5.57
Definition at line 107 of file ktexteditor.cpp.
◆ repository()
const KSyntaxHighlighting::Repository & Editor::repository | ( | ) | const |
Get read-only access to the syntax highlighting repository the editor uses.
Might be reloaded during runtime, repositoryReloaded() will be emitted in that cases.
- Returns
- syntax repository used by the editor
- Since
- 5.79
Definition at line 143 of file ktexteditor.cpp.
◆ repositoryReloaded
|
signal |
This signal is emitted whenever the editor syntax repository is reloaded.
Can be used to e.g. re-instantiate syntax definitions that got invalidated by the repository reload.
- Parameters
-
editor the editor which's repository was reloaded
- Since
- 5.79
◆ setApplication()
|
pure virtual |
Set the global application object.
This will allow the editor component to access the hosting application.
- Parameters
-
application application object if the argument is a nullptr, this will reset the application back to a dummy interface
Implemented in KTextEditor::EditorPrivate.
◆ theme()
KSyntaxHighlighting::Theme Editor::theme | ( | ) | const |
Get the current global theme.
Might change during runtime, configChanged() will be emitted in that cases. Individual views might have set different themes,
- See also
- KTextEditor::View::theme().
- Returns
- current global theme for all views
- Since
- 5.79
Definition at line 138 of file ktexteditor.cpp.
◆ unregisterVariable()
bool Editor::unregisterVariable | ( | const QString & | variableName | ) |
Unregisters a variable that was previously registered with registerVariableMatch() or registerVariablePrefix().
- Returns
- true if the variable was successfully unregistered, and false if the variable did not exist.
- Since
- 6.0
Definition at line 113 of file ktexteditor.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:56:22 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.