NetworkManager::VpnConnection
#include <vpnconnection.h>
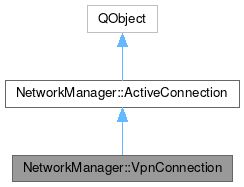
Public Types | |
typedef QList< Ptr > | List |
typedef QSharedPointer< VpnConnection > | Ptr |
enum | State { Unknown = 0 , Prepare , NeedAuth , Connecting , GettingIpConfig , Activated , Failed , Disconnected } |
enum | StateChangeReason { UnknownReason = 0 , NoneReason , UserDisconnectedReason , DeviceDisconnectedReason , ServiceStoppedReason , IpConfigInvalidReason , ConnectTimeoutReason , ServiceStartTimeoutReason , ServiceStartFailedReason , NoSecretsReason , LoginFailedReason , ConnectionRemovedReason } |
![]() | |
typedef QList< Ptr > | List |
typedef QSharedPointer< ActiveConnection > | Ptr |
enum | Reason { UknownReason = 0 , None , UserDisconnected , DeviceDisconnected , ServiceStopped , IpConfigInvalid , ConnectTimeout , ServiceStartTimeout , ServiceStartFailed , NoSecrets , LoginFailed , ConnectionRemoved , DependencyFailed , DeviceRealizeFailed , DeviceRemoved } |
enum | State { Unknown = 0 , Activating , Activated , Deactivating , Deactivated } |
Signals | |
void | bannerChanged (const QString &banner) |
void | stateChanged (NetworkManager::VpnConnection::State state, NetworkManager::VpnConnection::StateChangeReason reason) |
![]() | |
void | connectionChanged (const NetworkManager::Connection::Ptr &connection) |
void | default4Changed (bool isDefault) |
void | default6Changed (bool isDefault) |
void | devicesChanged () |
void | dhcp4ConfigChanged () |
void | dhcp6ConfigChanged () |
void | idChanged (const QString &id) |
void | ipV4ConfigChanged () |
void | ipV6ConfigChanged () |
void | masterChanged (const QString &uni) |
void | specificObjectChanged (const QString &path) |
void | stateChanged (NetworkManager::ActiveConnection::State state) |
void | stateChangedReason (NetworkManager::ActiveConnection::State state, NetworkManager::ActiveConnection::Reason reason) |
void | typeChanged (NetworkManager::ConnectionSettings::ConnectionType type) |
void | uuidChanged (const QString &uuid) |
void | vpnChanged (bool isVpn) |
Public Member Functions | |
VpnConnection (const QString &path, QObject *parent=nullptr) | |
~VpnConnection () override | |
QString | banner () const |
operator VpnConnection * () | |
NetworkManager::VpnConnection::State | state () const |
![]() | |
ActiveConnection (const QString &path, QObject *parent=nullptr) | |
~ActiveConnection () override | |
Connection::Ptr | connection () const |
bool | default4 () const |
bool | default6 () const |
QStringList | devices () const |
Dhcp4Config::Ptr | dhcp4Config () const |
Dhcp6Config::Ptr | dhcp6Config () const |
QString | id () const |
IpConfig | ipV4Config () const |
IpConfig | ipV6Config () const |
bool | isValid () const |
QString | master () const |
QString | path () const |
QString | specificObject () const |
NetworkManager::ActiveConnection::State | state () const |
NetworkManager::ConnectionSettings::ConnectionType | type () const |
QString | uuid () const |
bool | vpn () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
NETWORKMANAGERQT_NO_EXPORT | ActiveConnection (ActiveConnectionPrivate &dd, QObject *parent=nullptr) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
ActiveConnectionPrivate *const | d_ptr |
Detailed Description
An active VPN connection.
Definition at line 26 of file vpnconnection.h.
Member Typedef Documentation
◆ List
typedef QList<Ptr> NetworkManager::VpnConnection::List |
Definition at line 32 of file vpnconnection.h.
◆ Ptr
Definition at line 31 of file vpnconnection.h.
Member Enumeration Documentation
◆ State
Enum describing the possible VPN connection states.
Definition at line 36 of file vpnconnection.h.
◆ StateChangeReason
Definition at line 48 of file vpnconnection.h.
Constructor & Destructor Documentation
◆ VpnConnection()
|
explicit |
Creates a new VpnConnection object.
- Parameters
-
path the DBus path of the device
Definition at line 35 of file vpnconnection.cpp.
◆ ~VpnConnection()
|
override |
Destroys a VpnConnection object.
Definition at line 66 of file vpnconnection.cpp.
Member Function Documentation
◆ banner()
QString NetworkManager::VpnConnection::banner | ( | ) | const |
Return the current login banner.
Definition at line 70 of file vpnconnection.cpp.
◆ bannerChanged
|
signal |
This signal is emitted when the connection banner
has changed.
◆ operator VpnConnection *()
NetworkManager::VpnConnection::operator VpnConnection * | ( | ) |
operator for casting an ActiveConnection into a VpnConnection.
Returns 0 if this object is not a VPN connection. Introduced to make it possible to create a VpnConnection object for every active connection, without creating an ActiveConnection object, checking if it's a VPN connection, deleting the ActiveConnection and creating a VpnConnection
Definition at line 123 of file vpnconnection.cpp.
◆ state()
NetworkManager::VpnConnection::State NetworkManager::VpnConnection::state | ( | ) | const |
returns the current state
Definition at line 77 of file vpnconnection.cpp.
◆ stateChanged
|
signal |
This signal is emitted when the VPN connection state
has changed.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:52:50 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.