Syndication::Mapper
#include <mapper.h>
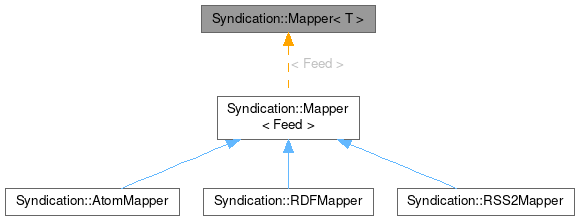
Public Member Functions | |
virtual | ~Mapper () |
virtual QSharedPointer< T > | map (SpecificDocumentPtr doc) const =0 |
Detailed Description
class Syndication::Mapper< T >
A mapper maps an SpecificDocument to something else.
The type of this "something else" is specified by the template parameter T. In the default implementation it is used with the Feed interface, but it is not limited to that. T can be an arbitrary class.
There are three (advanced and hopefully rare) use cases that require you to implement your own mapper. For more information on the possible uses, see TODO: link docs.
1) Add your own feed parser. In case you need support for another feed format (Okay! News, CDF, completely backward-incompatible Atom 5.0, you name it), you can implement AbstractParser and SpecificDocument for it and provide a Mapper<Feed>
- {public:};virtual QSharedPointer< T > map(SpecificDocumentPtr doc) const =0maps a format-specific document to abstraction of type T.ParserCollection< Feed > * parserCollection()The default ParserCollection instance parsing a DocumentSource into a Feed object.Definition global.cpp:41
2) Implement your own mapper for the Feed abstraction, for an existing parser. E.g. if you think Syndication does map Atom all wrong, you can implement your own Atom mapper and use that instead of the default one.
3) Use your own abstraction. In case the Feed interface does not fit your needs, you can use your own interface, let's say "MyFeed". Be aware you have to implement custom mappings for all feed formats then:
Constructor & Destructor Documentation
◆ ~Mapper()
|
inlinevirtual |
Member Function Documentation
◆ map()
|
pure virtual |
maps a format-specific document to abstraction of type T
.
- Note
- implementations may assume
doc
to have the type whose mapping they implement and may just statically cast to the subclass without further checking. If you register your own mapper, it's your responsibility to register the mapper only for the format it actually handles.
- Parameters
-
doc the document to map.
- Returns
- a newly created object implementing the abstraction
T
.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 31 2025 12:12:11 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.