KItinerary::PdfDocument
#include <pdfdocument.h>
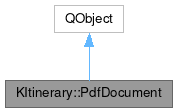
Properties | |
QString | author |
QDateTime | creationTime |
QString | creator |
QDateTime | modificationTime |
int | pageCount |
QVariantList | pages |
QString | producer |
QString | text |
QString | title |
![]() | |
objectName | |
Public Member Functions | |
PdfDocument (QObject *parent=nullptr) | |
QString | author () const |
QDateTime | creationTime () const |
QString | creator () const |
int | fileSize () const |
QDateTime | modificationTime () const |
PdfPage | page (int index) const |
int | pageCount () const |
QString | producer () const |
QString | text () const |
QString | title () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static PdfDocument * | fromData (const QByteArray &data, QObject *parent=nullptr) |
static bool | maybePdf (const QByteArray &data) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
PDF document for extraction.
This is used as input for ExtractorEngine and the JS extractor scripts.
Definition at line 91 of file pdfdocument.h.
Property Documentation
◆ author
|
read |
Definition at line 102 of file pdfdocument.h.
◆ creationTime
|
read |
Definition at line 97 of file pdfdocument.h.
◆ creator
|
read |
Definition at line 101 of file pdfdocument.h.
◆ modificationTime
|
read |
Definition at line 98 of file pdfdocument.h.
◆ pageCount
|
read |
Definition at line 95 of file pdfdocument.h.
◆ pages
|
read |
Definition at line 96 of file pdfdocument.h.
◆ producer
|
read |
Definition at line 100 of file pdfdocument.h.
◆ text
|
read |
Definition at line 94 of file pdfdocument.h.
◆ title
|
read |
Definition at line 99 of file pdfdocument.h.
Constructor & Destructor Documentation
◆ PdfDocument()
|
explicit |
Definition at line 234 of file pdfdocument.cpp.
Member Function Documentation
◆ author()
QString PdfDocument::author | ( | ) | const |
The document author.
Definition at line 355 of file pdfdocument.cpp.
◆ creationTime()
QDateTime PdfDocument::creationTime | ( | ) | const |
Creation time as specified in the PDF file.
Definition at line 295 of file pdfdocument.cpp.
◆ creator()
QString PdfDocument::creator | ( | ) | const |
The document creator.
Definition at line 350 of file pdfdocument.cpp.
◆ fileSize()
int PdfDocument::fileSize | ( | ) | const |
File size of the entire document in bytes.
Definition at line 259 of file pdfdocument.cpp.
◆ fromData()
|
static |
Creates a PdfDocument from the given raw data.
- Returns
nullptr
if loading fails or Poppler was not found.
Definition at line 368 of file pdfdocument.cpp.
◆ maybePdf()
|
static |
Fast check whether data
might be a PDF document.
Definition at line 394 of file pdfdocument.cpp.
◆ modificationTime()
QDateTime PdfDocument::modificationTime | ( | ) | const |
Modification time as specified in the PDF file.
Definition at line 304 of file pdfdocument.cpp.
◆ page()
PdfPage PdfDocument::page | ( | int | index | ) | const |
The n-thj page in this document.
Definition at line 254 of file pdfdocument.cpp.
◆ pageCount()
int PdfDocument::pageCount | ( | ) | const |
The number of pages in this document.
Definition at line 249 of file pdfdocument.cpp.
◆ producer()
QString PdfDocument::producer | ( | ) | const |
The document producer.
Definition at line 345 of file pdfdocument.cpp.
◆ text()
QString PdfDocument::text | ( | ) | const |
The entire text extracted from the PDF document.
Definition at line 242 of file pdfdocument.cpp.
◆ title()
QString PdfDocument::title | ( | ) | const |
The document title.
Definition at line 340 of file pdfdocument.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:54:59 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.