MessageViewer::Viewer
#include <viewer.h>
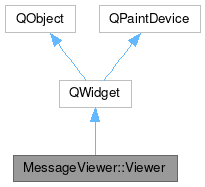
Public Types | |
enum | AttachmentAction { Open = 1 , OpenWith , View , Save , Properties , Delete , Copy , ScrollTo , ReplyMessageToAuthor , ReplyMessageToAll } |
enum | DisplayFormatMessage { UseGlobalSetting = 0 , Text = 1 , Html = 2 , Unknown = 3 , ICal = 4 } |
enum | ResourceOnlineMode { AllResources = 0 , SelectedResource = 1 } |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
Signals | |
void | deleteMessage (const Akonadi::Item &) |
void | displayPopupMenu (const Akonadi::Item &msg, const WebEngineViewer::WebHitTestResult &result, const QPoint &mousePos) |
void | itemRemoved () |
void | makeResourceOnline (MessageViewer::Viewer::ResourceOnlineMode mode) |
void | moveMessageToTrash () |
void | pageIsScrolledToBottom (bool) |
void | popupMenu (const Akonadi::Item &msg, const QUrl &url, const QUrl &imageUrl, const QPoint &mousePos) |
void | printingFinished () |
void | replyMessageTo (const KMime::Message::Ptr &message, bool replyToAll) |
void | requestConfigSync () |
void | sendResponse (MessageViewer::MDNWarningWidget::ResponseType type, KMime::MDN::SendingMode sendingMode) |
void | showMessage (const KMime::Message::Ptr &message, const QString &encoding) |
void | showNextMessage () |
void | showPreviousMessage () |
void | showReader (KMime::Content *aMsgPart, bool aHTML, const QString &encoding) |
void | showStatusBarMessage (const QString &message) |
void | urlClicked (const Akonadi::Item &, const QUrl &) |
void | zoomChanged (qreal zoomFactor) |
Public Slots | |
void | slotAttachmentSaveAll () |
void | slotAttachmentSaveAs () |
void | slotChangeDisplayMail (Viewer::DisplayFormatMessage, bool) |
void | slotFind () |
void | slotJumpDown () |
void | slotSaveMessage () |
void | slotScrollDown () |
void | slotScrollNext () |
void | slotScrollPrior () |
void | slotScrollUp () |
void | slotShowMessageSource () |
void | slotZoomIn () |
void | slotZoomOut () |
void | slotZoomReset () |
Public Member Functions | |
Viewer (QWidget *parent, QWidget *widget=nullptr, KActionCollection *actionCollection=nullptr) | |
void | addMessageLoadedHandler (AbstractMessageLoadedHandler *handler) |
void | atBottom () |
const AttachmentStrategy * | attachmentStrategy () const |
void | clear (MimeTreeParser::UpdateMode updateMode=MimeTreeParser::Delayed) |
QAction * | copyAction () const |
QAction * | copyImageLocation () const |
void | copySelectionToClipboard () |
QAction * | copyURLAction () const |
Akonadi::ItemFetchJob * | createFetchJob (const Akonadi::Item &item) |
CSSHelper * | cssHelper () const |
void | deleteMessage () |
QAction * | developmentToolsAction () const |
KToggleAction * | disableEmoticonAction () const |
Viewer::DisplayFormatMessage | displayFormatMessageOverwrite () const |
void | displaySplashPage (const QString &templateName, const QVariantHash &data, const QByteArray &domain=QByteArray()) |
MessageViewer::DKIMViewerMenu * | dkimViewerMenu () |
MessageViewer::DKIMWidgetInfo * | dkimWidgetInfo () |
void | enableMessageDisplay () |
void | exportToPdf (const QString &fileName) |
QAction * | findInMessageAction () const |
void | hasMultiMessages (bool messages) |
HeaderStylePlugin * | headerStylePlugin () const |
bool | htmlLoadExternal () const |
bool | htmlLoadExtOverride () const |
bool | htmlMail () const |
QUrl | imageUrlClicked () const |
QList< QAction * > | interceptorUrlActions (const WebEngineViewer::WebHitTestResult &result) const |
bool | isFixedFont () const |
QWidget * | mainWindow () |
void | mdnWarningAnimatedHide () |
KMime::Message::Ptr | message () const |
Akonadi::Item | messageItem () const |
QString | messagePath () const |
QAbstractItemModel * | messageTreeModel () const |
bool | mimePartTreeIsEmpty () const |
QString | overrideEncoding () const |
void | print () |
bool | printingMode () const |
void | printMessage (const Akonadi::Item &msg) |
void | printPreview () |
void | printPreviewMessage (const Akonadi::Item &message) |
void | readConfig () |
MessageViewer::RemoteContentMenu * | remoteContentMenu () const |
void | removeMessageLoadedHandler (AbstractMessageLoadedHandler *handler) |
QAction * | resetMessageDisplayFormatAction () const |
void | runJavaScript (const QString &code) |
QAction * | saveAsAction () const |
void | saveMainFrameScreenshotInFile (const QString &filename) |
QAction * | saveMessageDisplayFormatAction () const |
void | selectAll () |
QAction * | selectAllAction () const |
QString | selectedText () const |
void | setAttachmentStrategy (const AttachmentStrategy *strategy) |
void | setDecryptMessageOverwrite (bool overwrite=true) |
void | setDisplayFormatMessageOverwrite (Viewer::DisplayFormatMessage format) |
void | setFolderIdentity (uint folderIdentity) |
void | setHtmlLoadExtDefault (bool loadExtDefault) |
void | setHtmlLoadExtOverride (bool loadExtOverride) |
void | setIdentityManager (KIdentityManagementCore::IdentityManager *ident) |
void | setMessage (const KMime::Message::Ptr &message, MimeTreeParser::UpdateMode updateMode=MimeTreeParser::Delayed) |
void | setMessageItem (const Akonadi::Item &item, MimeTreeParser::UpdateMode updateMode=MimeTreeParser::Delayed) |
void | setMessagePart (KMime::Content *aMsgPart) |
void | setMessagePath (const QString &path) |
void | setOverrideEncoding (const QString &encoding) |
void | setPluginName (const QString &pluginName) |
void | setPrintElementBackground (bool printElementBackground) |
void | setPrinting (bool enable) |
void | setShowEncryptionDetails (bool showDetails) |
void | setShowSignatureDetails (bool showDetails) |
void | setUseFixedFont (bool useFixedFont) |
void | setWebViewZoomFactor (qreal factor) |
void | setZoomFactor (qreal zoomFactor) |
KActionMenu * | shareServiceUrlMenu () const |
QAction * | shareTextAction () const |
void | showDevelopmentTools () |
bool | showEncryptionDetails () const |
void | showMdnInformations (const QPair< QString, bool > &mdnInfo) |
void | showOpenAttachmentFolderWidget (const QList< QUrl > &urls) |
bool | showSignatureDetails () const |
QAction * | speakTextAction () const |
KToggleAction * | toggleFixFontAction () const |
KToggleAction * | toggleMimePartTreeAction () const |
void | update (MimeTreeParser::UpdateMode updateMode=MimeTreeParser::Delayed) |
void | updateShowMultiMessagesButton (bool enablePreviousButton, bool enableNextButton) |
QUrl | urlClicked () const |
QAction * | urlOpenAction () const |
QList< QAction * > | viewerPluginActionList (MessageViewer::ViewerPluginInterface::SpecificFeatureTypes features) |
QAction * | viewSourceAction () const |
qreal | webViewZoomFactor () const |
void | writeConfig (bool withSync=true) |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
virtual QSize | minimumSizeHint () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
virtual QSize | sizeHint () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
Protected Member Functions | |
void | changeEvent (QEvent *event) override |
void | closeEvent (QCloseEvent *) override |
bool | event (QEvent *e) override |
bool | eventFilter (QObject *obj, QEvent *event) override |
void | resizeEvent (QResizeEvent *) override |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEnterEvent *event) |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | initPainter (QPainter *painter) const const override |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | leaveEvent (QEvent *event) |
virtual int | metric (PaintDeviceMetric m) const const override |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | nativeEvent (const QByteArray &eventType, void *message, qintptr *result) |
virtual void | paintEvent (QPaintEvent *event) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus (Qt::InputMethodQuery query) |
virtual void | wheelEvent (QWheelEvent *event) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
ViewerPrivate *const | d_ptr |
Detailed Description
This is the main widget for the viewer.
See the documentation of ViewerPrivate for implementation details. See Mainpage.dox for an overview of the classes in the messageviewer library.
Member Enumeration Documentation
◆ AttachmentAction
◆ DisplayFormatMessage
◆ ResourceOnlineMode
Constructor & Destructor Documentation
◆ Viewer()
|
explicit |
Create a mail viewer widget.
- Parameters
-
parent parent widget widget the application's main widget actionCollection the action collection where the widget's actions will belong to
Definition at line 63 of file viewer.cpp.
Member Function Documentation
◆ addMessageLoadedHandler()
Adds a handler
for actions that will be executed when the message has been loaded into the view.
Definition at line 546 of file viewer.cpp.
◆ atBottom()
Returns true if the message view is scrolled to the bottom.
Definition at line 247 of file viewer.cpp.
◆ attachmentStrategy()
|
nodiscard |
Definition at line 374 of file viewer.cpp.
◆ changeEvent()
|
overrideprotectedvirtual |
Reimplemented from QWidget.
Definition at line 115 of file viewer.cpp.
◆ clear()
Convenience method to clear the reader and discard the current message.
Sets the internal message pointer returned by message() to 0.
- Parameters
-
updateMode - update the display immediately or not. See UpdateMode.
Definition at line 512 of file viewer.cpp.
◆ closeEvent()
|
overrideprotectedvirtual |
Some necessary event handling.
Reimplemented from QWidget.
Definition at line 210 of file viewer.cpp.
◆ copyAction()
|
nodiscard |
Definition at line 440 of file viewer.cpp.
◆ copyImageLocation()
|
nodiscard |
Definition at line 452 of file viewer.cpp.
◆ copySelectionToClipboard()
Definition at line 577 of file viewer.cpp.
◆ copyURLAction()
|
nodiscard |
Definition at line 434 of file viewer.cpp.
◆ createFetchJob()
Create an item fetch job that is suitable for using to fetch the message item that will be displayed on this viewer.
It will set the correct fetch scope. You still need to connect to the job's result signal.
Definition at line 534 of file viewer.cpp.
◆ cssHelper()
|
nodiscard |
Definition at line 398 of file viewer.cpp.
◆ deleteMessage()
Initiates a delete, by sending a signal to delete the message item.
Definition at line 565 of file viewer.cpp.
◆ developmentToolsAction()
|
nodiscard |
Definition at line 640 of file viewer.cpp.
◆ disableEmoticonAction()
|
nodiscard |
Definition at line 646 of file viewer.cpp.
◆ displayFormatMessageOverwrite()
|
nodiscard |
Get the html override setting.
Definition at line 277 of file viewer.cpp.
◆ displaySplashPage()
Display a generic HTML splash page instead of a message.
- Parameters
-
templateName - the template to be loaded data - data for the template domain the domain.
Definition at line 162 of file viewer.cpp.
◆ dkimViewerMenu()
|
nodiscard |
Definition at line 670 of file viewer.cpp.
◆ dkimWidgetInfo()
|
nodiscard |
Definition at line 778 of file viewer.cpp.
◆ enableMessageDisplay()
Enable the displaying of messages again after an splash (or other) page was displayed.
Definition at line 168 of file viewer.cpp.
◆ event()
|
nodiscardoverrideprotectedvirtual |
◆ eventFilter()
|
nodiscardoverrideprotectedvirtual |
Reimplemented from QObject.
Definition at line 100 of file viewer.cpp.
◆ exportToPdf()
Definition at line 784 of file viewer.cpp.
◆ findInMessageAction()
|
nodiscard |
Definition at line 607 of file viewer.cpp.
◆ hasMultiMessages()
Definition at line 748 of file viewer.cpp.
◆ headerStylePlugin()
|
nodiscard |
Definition at line 664 of file viewer.cpp.
◆ htmlLoadExternal()
|
nodiscard |
Is loading ext.
references to be supported? Takes into account override
Definition at line 313 of file viewer.cpp.
◆ htmlLoadExtOverride()
|
nodiscard |
Get the load external references override setting.
Definition at line 301 of file viewer.cpp.
◆ htmlMail()
|
nodiscard |
Is html mail to be supported?
Takes into account override
Definition at line 307 of file viewer.cpp.
◆ imageUrlClicked()
|
nodiscard |
Definition at line 494 of file viewer.cpp.
◆ interceptorUrlActions()
|
nodiscard |
Definition at line 712 of file viewer.cpp.
◆ isFixedFont()
|
nodiscard |
Definition at line 319 of file viewer.cpp.
◆ itemRemoved
|
signal |
Emitted when the item, previously set with setMessageItem, has been removed.
◆ mainWindow()
|
nodiscard |
Definition at line 331 of file viewer.cpp.
◆ mdnWarningAnimatedHide()
Definition at line 688 of file viewer.cpp.
◆ message()
|
nodiscard |
Returns the current message displayed in the viewer.
Definition at line 343 of file viewer.cpp.
◆ messageItem()
|
nodiscard |
Returns the current message item displayed in the viewer.
Definition at line 349 of file viewer.cpp.
◆ messagePath()
|
nodiscard |
The path to the message in terms of Akonadi collection hierarchy.
Definition at line 150 of file viewer.cpp.
◆ messageTreeModel()
A QAIM tree model of the message structure.
Definition at line 529 of file viewer.cpp.
◆ mimePartTreeIsEmpty()
|
nodiscard |
Definition at line 410 of file viewer.cpp.
◆ overrideEncoding()
|
nodiscard |
Definition at line 386 of file viewer.cpp.
◆ popupMenu
|
signal |
The user presses the right mouse button.
'url' may be 0.
◆ print()
Print the currently displayed message.
Definition at line 192 of file viewer.cpp.
◆ printingMode()
|
nodiscard |
Definition at line 470 of file viewer.cpp.
◆ printMessage()
Sets a message as the current one and print it immediately.
- Parameters
-
msg the message to display and print
Definition at line 174 of file viewer.cpp.
◆ printPreview()
Definition at line 186 of file viewer.cpp.
◆ printPreviewMessage()
Definition at line 180 of file viewer.cpp.
◆ readConfig()
Definition at line 523 of file viewer.cpp.
◆ remoteContentMenu()
|
nodiscard |
Definition at line 676 of file viewer.cpp.
◆ removeMessageLoadedHandler()
Removes the handler
for actions that will be executed when the message has been loaded into the view.
Definition at line 558 of file viewer.cpp.
◆ resetMessageDisplayFormatAction()
|
nodiscard |
Definition at line 634 of file viewer.cpp.
◆ resizeEvent()
|
overrideprotectedvirtual |
Reimplemented from QWidget.
Definition at line 198 of file viewer.cpp.
◆ runJavaScript()
Definition at line 718 of file viewer.cpp.
◆ saveAsAction()
|
nodiscard |
Definition at line 458 of file viewer.cpp.
◆ saveMainFrameScreenshotInFile()
Definition at line 652 of file viewer.cpp.
◆ saveMessageDisplayFormatAction()
|
nodiscard |
Definition at line 628 of file viewer.cpp.
◆ selectAll()
Definition at line 571 of file viewer.cpp.
◆ selectAllAction()
|
nodiscard |
Definition at line 422 of file viewer.cpp.
◆ selectedText()
|
nodiscard |
Definition at line 271 of file viewer.cpp.
◆ setAttachmentStrategy()
Definition at line 380 of file viewer.cpp.
◆ setDecryptMessageOverwrite()
Enforce message decryption.
Definition at line 337 of file viewer.cpp.
◆ setDisplayFormatMessageOverwrite()
Override default html mail setting.
Definition at line 283 of file viewer.cpp.
◆ setFolderIdentity()
Definition at line 802 of file viewer.cpp.
◆ setHtmlLoadExtDefault()
Default behavior for loading external references.
Use this for specifying the external reference loading behavior as specified in the user settings.
- See also
- setHtmlLoadExtOverride
Definition at line 289 of file viewer.cpp.
◆ setHtmlLoadExtOverride()
Override default load external references setting.
- Warning
- This must only be called when the user has explicitly been asked to retrieve external references!
- See also
- setHtmlLoadExtDefault
Definition at line 295 of file viewer.cpp.
◆ setIdentityManager()
Definition at line 796 of file viewer.cpp.
◆ setMessage()
Set the message that shall be shown.
- Parameters
-
message - the message to be shown. If 0, an empty page is displayed. updateMode - update the display immediately or not. See UpdateMode.
Definition at line 124 of file viewer.cpp.
◆ setMessageItem()
Set the Akonadi item that will be displayed.
- Parameters
-
item - the Akonadi item to be displayed. If it doesn't hold a mail (KMime::Message::Ptr as payload data), an empty page is shown. updateMode - update the display immediately or not. See UpdateMode.
Definition at line 133 of file viewer.cpp.
◆ setMessagePart()
Instead of settings a message to be shown sets a message part to be shown.
Definition at line 506 of file viewer.cpp.
◆ setMessagePath()
Set the path to the message in terms of Akonadi collection hierarchy.
Definition at line 156 of file viewer.cpp.
◆ setOverrideEncoding()
Definition at line 392 of file viewer.cpp.
◆ setPluginName()
Definition at line 694 of file viewer.cpp.
◆ setPrintElementBackground()
Definition at line 724 of file viewer.cpp.
◆ setPrinting()
Definition at line 476 of file viewer.cpp.
◆ setShowEncryptionDetails()
Definition at line 760 of file viewer.cpp.
◆ setShowSignatureDetails()
Definition at line 736 of file viewer.cpp.
◆ setUseFixedFont()
Definition at line 325 of file viewer.cpp.
◆ setWebViewZoomFactor()
Definition at line 772 of file viewer.cpp.
◆ setZoomFactor()
Definition at line 583 of file viewer.cpp.
◆ shareServiceUrlMenu()
|
nodiscard |
Definition at line 658 of file viewer.cpp.
◆ shareTextAction()
|
nodiscard |
Definition at line 613 of file viewer.cpp.
◆ showDevelopmentTools()
Definition at line 790 of file viewer.cpp.
◆ showEncryptionDetails()
|
nodiscard |
Definition at line 742 of file viewer.cpp.
◆ showMdnInformations()
Definition at line 682 of file viewer.cpp.
◆ showMessage
|
signal |
Emitted when the message should be shown in a separate window.
◆ showOpenAttachmentFolderWidget()
Definition at line 700 of file viewer.cpp.
◆ showReader
|
signal |
Emitted when the content should be shown in a separate window.
◆ showSignatureDetails()
|
nodiscard |
Definition at line 730 of file viewer.cpp.
◆ showStatusBarMessage
|
signal |
Emitted when a status bar message is shown.
Note that the status bar message is also set to KPIM::BroadcastStatus in addition.
◆ slotAttachmentSaveAll
|
slot |
Definition at line 223 of file viewer.cpp.
◆ slotAttachmentSaveAs
|
slot |
Definition at line 217 of file viewer.cpp.
◆ slotChangeDisplayMail
|
slot |
Definition at line 619 of file viewer.cpp.
◆ slotFind
|
slot |
Definition at line 368 of file viewer.cpp.
◆ slotJumpDown
|
slot |
Definition at line 253 of file viewer.cpp.
◆ slotSaveMessage
|
slot |
Definition at line 229 of file viewer.cpp.
◆ slotScrollDown
|
slot |
Definition at line 241 of file viewer.cpp.
◆ slotScrollNext
|
slot |
Definition at line 265 of file viewer.cpp.
◆ slotScrollPrior
|
slot |
Definition at line 259 of file viewer.cpp.
◆ slotScrollUp
|
slot |
HTML Widget scrollbar and layout handling.
Scrolling always happens in the direction of the slot that is called. I.e. the methods take the absolute value of
Definition at line 235 of file viewer.cpp.
◆ slotShowMessageSource
|
slot |
Definition at line 517 of file viewer.cpp.
◆ slotZoomIn
|
slot |
Definition at line 595 of file viewer.cpp.
◆ slotZoomOut
|
slot |
Definition at line 601 of file viewer.cpp.
◆ slotZoomReset
|
slot |
Definition at line 589 of file viewer.cpp.
◆ speakTextAction()
|
nodiscard |
Definition at line 446 of file viewer.cpp.
◆ toggleFixFontAction()
|
nodiscard |
Definition at line 404 of file viewer.cpp.
◆ toggleMimePartTreeAction()
|
nodiscard |
Definition at line 416 of file viewer.cpp.
◆ update()
Definition at line 500 of file viewer.cpp.
◆ updateShowMultiMessagesButton()
Definition at line 754 of file viewer.cpp.
◆ urlClicked() [1/2]
|
nodiscard |
Definition at line 488 of file viewer.cpp.
◆ urlClicked [2/2]
|
signal |
The message viewer handles some types of urls itself, most notably http(s) and ftp(s).
When it can't handle the url it will Q_EMIT this signal.
◆ urlOpenAction()
|
nodiscard |
Definition at line 464 of file viewer.cpp.
◆ viewerPluginActionList()
|
nodiscard |
Definition at line 706 of file viewer.cpp.
◆ viewSourceAction()
|
nodiscard |
Definition at line 428 of file viewer.cpp.
◆ webViewZoomFactor()
|
nodiscard |
Definition at line 766 of file viewer.cpp.
◆ writeConfig()
Definition at line 482 of file viewer.cpp.
Member Data Documentation
◆ d_ptr
|
protected |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:49:18 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.