KMyMoneyPlugin::Plugin
#include <kmymoneyplugin.h>
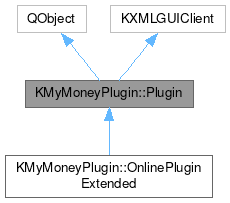
Public Slots | |
virtual void | plug (KXMLGUIFactory *guiFactory) |
virtual void | unplug () |
virtual void | updateActions (const SelectedObjects &selections) |
virtual void | updateConfiguration () |
Public Member Functions | |
Plugin (QObject *parent, const KPluginMetaData &metaData, const QVariantList &args) | |
QString | componentDisplayName () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
KXMLGUIClient (KXMLGUIClient *parent) | |
virtual QAction * | action (const QDomElement &element) const |
QAction * | action (const QString &name) const |
virtual KActionCollection * | actionCollection () const |
QList< KXMLGUIClient * > | childClients () |
KXMLGUIBuilder * | clientBuilder () const |
virtual QString | componentName () const |
virtual QDomDocument | domDocument () const |
KXMLGUIFactory * | factory () const |
void | insertChildClient (KXMLGUIClient *child) |
KXMLGUIClient * | parentClient () const |
void | plugActionList (const QString &name, const QList< QAction * > &actionList) |
void | reloadXML () |
void | removeChildClient (KXMLGUIClient *child) |
void | replaceXMLFile (const QString &xmlfile, const QString &localxmlfile, bool merge=false) |
void | setClientBuilder (KXMLGUIBuilder *builder) |
void | setFactory (KXMLGUIFactory *factory) |
void | setXMLGUIBuildDocument (const QDomDocument &doc) |
void | unplugActionList (const QString &name) |
virtual QString | xmlFile () const |
QDomDocument | xmlguiBuildDocument () const |
Protected Member Functions | |
AppInterface * | appInterface () const |
ImportInterface * | importInterface () const |
StatementInterface * | statementInterface () const |
KToggleAction * | toggleAction (const QString &name) const |
ViewInterface * | viewInterface () const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
void | loadStandardsXmlFile () |
virtual void | setComponentName (const QString &componentName, const QString &componentDisplayName) |
virtual void | setDOMDocument (const QDomDocument &document, bool merge=false) |
virtual void | setLocalXMLFile (const QString &file) |
virtual void | setXML (const QString &document, bool merge=false) |
virtual void | setXMLFile (const QString &file, bool merge=false, bool setXMLDoc=true) |
virtual void | stateChanged (const QString &newstate, ReverseStateChange reverse=StateNoReverse) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
static QString | findVersionNumber (const QString &xml) |
![]() | |
static QString | standardsXmlFileLocation () |
Detailed Description
This class describes the interface between KMyMoney and it's plugins.
The plugins are based on Qt 5's plugin system. So you must compile json information into the plugin. KMyMoney looks into the folder "${PLUGIN_INSTALL_DIR}/kmymoney/" and loads all plugins found there (if the user did not deactivate the plugin).
The json header of the plugin must comply with the requirements of KCoreAddon's KPluginMetaData class. To load the plugin at start up the service type "KMyMoney/Plugin" must be set.
- Warning
- The plugin system for KMyMoney 5 is still in development. Especially the loading of the on-demand plugins (mainly undocumented :( ) will change.
A basic json header is shown below.
This example assumes you are using
to replace the version variables using cmake.
- See also
- https://doc.qt.io/qt-5/plugins-howto.html
- https://api.kde.org/frameworks/kcoreaddons/html/classKPluginMetaData.html
Definition at line 106 of file kmymoneyplugin.h.
Constructor & Destructor Documentation
◆ Plugin()
|
explicit |
Definition at line 29 of file kmymoneyplugin.cpp.
◆ ~Plugin()
|
virtual |
Definition at line 41 of file kmymoneyplugin.cpp.
Member Function Documentation
◆ appInterface()
|
protected |
Definition at line 90 of file kmymoneyplugin.cpp.
◆ componentDisplayName()
QString KMyMoneyPlugin::Plugin::componentDisplayName | ( | ) | const |
Definition at line 45 of file kmymoneyplugin.cpp.
◆ importInterface()
|
protected |
Definition at line 108 of file kmymoneyplugin.cpp.
◆ plug
|
virtualslot |
Called during plug in process.
Reimplemented in KMyMoneyPlugin::OnlinePluginExtended.
Definition at line 50 of file kmymoneyplugin.cpp.
◆ statementInterface()
|
protected |
Definition at line 102 of file kmymoneyplugin.cpp.
◆ toggleAction()
|
protected |
See KMyMoneyApp::toggleAction() for a description.
Definition at line 68 of file kmymoneyplugin.cpp.
◆ unplug
|
virtualslot |
Called before unloading.
Reimplemented in KMyMoneyPlugin::OnlinePluginExtended.
Definition at line 55 of file kmymoneyplugin.cpp.
◆ updateActions
|
virtualslot |
This method is called by the application whenever a selection changes.
The default implementation does nothing.
Definition at line 59 of file kmymoneyplugin.cpp.
◆ updateConfiguration
|
virtualslot |
This method is called by the application whenever the configuration changes.
The default implementation does nothing.
Definition at line 64 of file kmymoneyplugin.cpp.
◆ viewInterface()
|
protected |
Definition at line 96 of file kmymoneyplugin.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:15:40 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.