QCPLayer
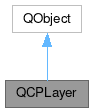
Public Types | |
enum | LayerMode { lmLogical , lmBuffered } |
![]() | |
typedef | QObjectList |
Public Member Functions | |
QCPLayer (QCustomPlot *parentPlot, const QString &layerName) | |
QList< QCPLayerable * > | children () const |
int | index () const |
LayerMode | mode () const |
QString | name () const |
QCustomPlot * | parentPlot () const |
void | replot () |
void | setMode (LayerMode mode) |
void | setVisible (bool visible) |
bool | visible () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
void | addChild (QCPLayerable *layerable, bool prepend) |
void | draw (QCPPainter *painter) |
void | drawToPaintBuffer () |
void | removeChild (QCPLayerable *layerable) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
QList< QCPLayerable * > | mChildren |
int | mIndex |
LayerMode | mMode |
QString | mName |
QWeakPointer< QCPAbstractPaintBuffer > | mPaintBuffer |
QCustomPlot * | mParentPlot |
bool | mVisible |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Detailed Description
A layer that may contain objects, to control the rendering order.
The Layering system of QCustomPlot is the mechanism to control the rendering order of the elements inside the plot.
It is based on the two classes QCPLayer and QCPLayerable. QCustomPlot holds an ordered list of one or more instances of QCPLayer (see QCustomPlot::addLayer, QCustomPlot::layer, QCustomPlot::moveLayer, etc.). When replotting, QCustomPlot goes through the list of layers bottom to top and successively draws the layerables of the layers into the paint buffer(s).
A QCPLayer contains an ordered list of QCPLayerable instances. QCPLayerable is an abstract base class from which almost all visible objects derive, like axes, grids, graphs, items, etc.
Default layers
Initially, QCustomPlot has six layers: "background", "grid", "main", "axes", "legend" and "overlay" (in that order). On top is the "overlay" layer, which only contains the QCustomPlot's selection rect (QCustomPlot::selectionRect). The next two layers "axes" and "legend" contain the default axes and legend, so they will be drawn above plottables. In the middle, there is the "main" layer. It is initially empty and set as the current layer (see QCustomPlot::setCurrentLayer). This means, all new plottables, items etc. are created on this layer by default. Then comes the "grid" layer which contains the QCPGrid instances (which belong tightly to QCPAxis, see QCPAxis::grid). The Axis rect background shall be drawn behind everything else, thus the default QCPAxisRect instance is placed on the "background" layer. Of course, the layer affiliation of the individual objects can be changed as required (QCPLayerable::setLayer).
Controlling the rendering order via layers
Controlling the ordering of layerables in the plot is easy: Create a new layer in the position you want the layerable to be in, e.g. above "main", with QCustomPlot::addLayer. Then set the current layer with QCustomPlot::setCurrentLayer to that new layer and finally create the objects normally. They will be placed on the new layer automatically, due to the current layer setting. Alternatively you could have also ignored the current layer setting and just moved the objects with QCPLayerable::setLayer to the desired layer after creating them.
It is also possible to move whole layers. For example, If you want the grid to be shown in front of all plottables/items on the "main" layer, just move it above "main" with QCustomPlot::moveLayer.
The rendering order within one layer is simply by order of creation or insertion. The item created last (or added last to the layer), is drawn on top of all other objects on that layer.
When a layer is deleted, the objects on it are not deleted with it, but fall on the layer below the deleted layer, see QCustomPlot::removeLayer.
Replotting only a specific layer
If the layer mode (setMode) is set to lmBuffered, you can replot only this specific layer by calling replot. In certain situations this can provide better replot performance, compared with a full replot of all layers. Upon creation of a new layer, the layer mode is initialized to lmLogical. The only layer that is set to lmBuffered in a new QCustomPlot instance is the "overlay" layer, containing the selection rect.
Definition at line 682 of file qcustomplot.h.
Member Enumeration Documentation
◆ LayerMode
enum QCPLayer::LayerMode |
Defines the different rendering modes of a layer. Depending on the mode, certain layers can be replotted individually, without the need to replot (possibly complex) layerables on other layers.
- See also
- setMode
Enumerator | |
---|---|
lmLogical | Layer is used only for rendering order, and shares paint buffer with all other adjacent logical layers. |
lmBuffered | Layer has its own paint buffer and may be replotted individually (see replot). |
Definition at line 702 of file qcustomplot.h.
Constructor & Destructor Documentation
◆ QCPLayer()
QCPLayer::QCPLayer | ( | QCustomPlot * | parentPlot, |
const QString & | layerName ) |
Creates a new QCPLayer instance.
Normally you shouldn't directly instantiate layers, use QCustomPlot::addLayer instead.
- Warning
- It is not checked that layerName is actually a unique layer name in parentPlot. This check is only performed by QCustomPlot::addLayer.
Definition at line 1067 of file qcustomplot.cpp.
◆ ~QCPLayer()
|
virtual |
Definition at line 1079 of file qcustomplot.cpp.
Member Function Documentation
◆ addChild()
|
protected |
Adds the layerable to the list of this layer. If prepend is set to true, the layerable will be prepended to the list, i.e. be drawn beneath the other layerables already in the list.
This function does not change the mLayer member of layerable to this layer. (Use QCPLayerable::setLayer to change the layer of an object, not this function.)
- See also
- removeChild
Definition at line 1224 of file qcustomplot.cpp.
◆ children()
|
inline |
Returns a list of all layerables on this layer. The order corresponds to the rendering order: layerables with higher indices are drawn above layerables with lower indices.
Definition at line 714 of file qcustomplot.h.
◆ draw()
|
protected |
Draws the contents of this layer with the provided painter.
- See also
- replot, drawToPaintBuffer
Definition at line 1143 of file qcustomplot.cpp.
◆ drawToPaintBuffer()
|
protected |
Draws the contents of this layer into the paint buffer which is associated with this layer. The association is established by the parent QCustomPlot, which manages all paint buffers (see QCustomPlot::setupPaintBuffers).
- See also
- draw
Definition at line 1166 of file qcustomplot.cpp.
◆ index()
|
inline |
Returns the index this layer has in the QCustomPlot. The index is the integer number by which this layer can be accessed via QCustomPlot::layer.
Layers with higher indices will be drawn above layers with lower indices.
Definition at line 713 of file qcustomplot.h.
◆ mode()
|
inline |
Definition at line 716 of file qcustomplot.h.
◆ name()
|
inline |
Definition at line 712 of file qcustomplot.h.
◆ parentPlot()
|
inline |
Definition at line 711 of file qcustomplot.h.
◆ removeChild()
|
protected |
Removes the layerable from the list of this layer.
This function does not change the mLayer member of layerable. (Use QCPLayerable::setLayer to change the layer of an object, not this function.)
- See also
- addChild
Definition at line 1247 of file qcustomplot.cpp.
◆ replot()
void QCPLayer::replot | ( | ) |
If the layer mode (setMode) is set to lmBuffered, this method allows replotting only the layerables on this specific layer, without the need to replot all other layers (as a call to QCustomPlot::replot would do).
QCustomPlot also makes sure to replot all layers instead of only this one, if the layer ordering or any layerable-layer-association has changed since the last full replot and any other paint buffers were thus invalidated.
If the layer mode is lmLogical however, this method simply calls QCustomPlot::replot on the parent QCustomPlot instance.
- See also
- draw
Definition at line 1198 of file qcustomplot.cpp.
◆ setMode()
void QCPLayer::setMode | ( | QCPLayer::LayerMode | mode | ) |
Sets the rendering mode of this layer.
If mode is set to lmBuffered for a layer, it will be given a dedicated paint buffer by the parent QCustomPlot instance. This means it may be replotted individually by calling QCPLayer::replot, without needing to replot all other layers.
Layers which are set to lmLogical (the default) are used only to define the rendering order and can't be replotted individually.
Note that each layer which is set to lmBuffered requires additional paint buffers for the layers below, above and for the layer itself. This increases the memory consumption and (slightly) decreases the repainting speed because multiple paint buffers need to be joined. So you should carefully choose which layers benefit from having their own paint buffer. A typical example would be a layer which contains certain layerables (e.g. items) that need to be changed and thus replotted regularly, while all other layerables on other layers stay static. By default, only the topmost layer called "overlay" is in mode lmBuffered, and contains the selection rect.
- See also
- replot
Definition at line 1127 of file qcustomplot.cpp.
◆ setVisible()
void QCPLayer::setVisible | ( | bool | visible | ) |
Sets whether this layer is visible or not. If visible is set to false, all layerables on this layer will be invisible.
This function doesn't change the visibility property of the layerables (QCPLayerable::setVisible), but the QCPLayerable::realVisibility of each layerable takes the visibility of the parent layer into account.
Definition at line 1101 of file qcustomplot.cpp.
◆ visible()
|
inline |
Definition at line 715 of file qcustomplot.h.
Member Data Documentation
◆ mChildren
|
protected |
Definition at line 730 of file qcustomplot.h.
◆ mIndex
|
protected |
Definition at line 729 of file qcustomplot.h.
◆ mMode
|
protected |
Definition at line 732 of file qcustomplot.h.
◆ mName
|
protected |
Definition at line 728 of file qcustomplot.h.
◆ mPaintBuffer
|
protected |
Definition at line 735 of file qcustomplot.h.
◆ mParentPlot
|
protected |
Definition at line 727 of file qcustomplot.h.
◆ mVisible
|
protected |
Definition at line 731 of file qcustomplot.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:16:42 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.