kviewshell
DjVuPort Class Reference
Base class for notification targets. More...
#include <DjVuPort.h>
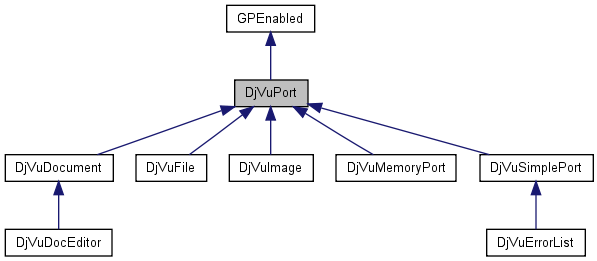
Public Member Functions | |
DjVuPort (const DjVuPort &port) | |
DjVuPort () | |
DjVuPort & | operator= (const DjVuPort &port) |
virtual | ~DjVuPort () |
DjVuPort.h | |
Files #"DjVuPort.h"# and #"DjVuPort.cpp"# implement a communication mechanism between different parties involved in decoding DjVu files. It should be pretty clear that the creator of {DjVuDocument} and {DjVuFile} would like to receive some information about the progress of decoding, errors occurred, etc. It may also want to provide source data for decoders (like it's done in the plugin where the real data is downloaded from the net and is fed into DjVu decoders). Normally this functionality is implemented by means of callbacks which are run when a given condition comes true. Unfortunately it's not quite easy to implement this strategy in our case. The reason is that there may be more than one "client" working with the same document, and the document should send the information to each of the clients. This could be done by means of callback {lists}, of course, but we want to achieve more bulletproof results: we want to be sure that the client that we're about to contact is still alive, and is not being destroyed by another thread. Besides, we are going to call these "callbacks" from many places, from many different classes. Maintaining multi-thread safe callback lists is very difficult. Finally, we want to provide some default implementation of these "callbacks" in the library, which should attempt to process the requests themselves if they can, and contact the client only if they're unable to do it (like in the case of {DjVuPort::request_data}() with local URL where {DjVuDocument} can get the data from the hard drive itself not disturbing the document's creator. Two classes implement a general communication mechanism: {DjVuPort} and {DjVuPortcaster}. Any sender and recipient of requests should be a subclass of {DjVuPort}. {DjVuPortcaster} maintains a map of routes between {DjVuPort}s, which should be configured by somebody else. Whenever a port wants to send a request, it calls the corresponding function of {DjVuPortcaster}, and the portcaster relays the request to all the destinations that it sees in the internal map. The {DjVuPortcaster} is responsible for keeping the map up to date by getting rid of destinations that have been destroyed. Map updates are performed from a single place and are serialized by a global monitor. DjVu decoder communication mechanism.
| |
virtual bool | inherits (const GUTF8String &class_name) const |
Static Public Member Functions | |
static DjVuPortcaster * | get_portcaster (void) |
static void | operator delete (void *addr) |
static void * | operator new (size_t sz) |
Notifications. | |
These virtual functions may be overridden by the subclasses of DjVuPort#.
They are called by the {DjVuPortcaster} when the port is alive and when there is a route between the source of the notification and this port. | |
enum | ErrorRecoveryAction { ABORT = 0, SKIP_PAGES = 1, SKIP_CHUNKS = 2, KEEP_ALL = 3 } |
virtual GP< DjVuFile > | id_to_file (const DjVuPort *source, const GUTF8String &id) |
virtual GURL | id_to_url (const DjVuPort *source, const GUTF8String &id) |
virtual void | notify_chunk_done (const DjVuPort *source, const GUTF8String &name) |
virtual void | notify_decode_progress (const DjVuPort *source, float done) |
virtual void | notify_doc_flags_changed (const class DjVuDocument *source, long set_mask, long clr_mask) |
virtual bool | notify_error (const DjVuPort *source, const GUTF8String &msg) |
virtual void | notify_file_flags_changed (const class DjVuFile *source, long set_mask, long clr_mask) |
virtual void | notify_redisplay (const class DjVuImage *source) |
virtual void | notify_relayout (const class DjVuImage *source) |
virtual bool | notify_status (const DjVuPort *source, const GUTF8String &msg) |
virtual GP< DataPool > | request_data (const DjVuPort *source, const GURL &url) |
Detailed Description
Base class for notification targets.DjVuPort# provides base functionality for classes willing to take part in sending and receiving messages generated during decoding process. You need to derive your class from DjVuPort# if you want it to be able to send or receive requests. In addition, for receiving requests you should override one or more virtual function.
{ Important remark} --- All ports should be allocated on the heap using operator new# and immediately secured using a {GP} smart pointer. Ports which are not secured by a smart-pointer are not considered ``alive'' and never receive notifications!
Definition at line 141 of file DjVuPort.h.
Member Enumeration Documentation
This is the standard types for defining what to do in case of errors.
This is only used by some of the subclasses, but it needs to be defined here to guarantee all subclasses use the same enum types. In general, many errors are non recoverable. Using a setting other than ABORT may just result in even more errors.
Definition at line 259 of file DjVuPort.h.
Constructor & Destructor Documentation
DjVuPort::DjVuPort | ( | ) |
Definition at line 207 of file DjVuPort.cpp.
DjVuPort::~DjVuPort | ( | void | ) | [virtual] |
Definition at line 234 of file DjVuPort.cpp.
DjVuPort::DjVuPort | ( | const DjVuPort & | port | ) |
Copy constructor.
When DjVuPort::s are copied, the portcaster copies all incoming and outgoing routes of the original.
Definition at line 216 of file DjVuPort.cpp.
Member Function Documentation
DjVuPortcaster * DjVuPort::get_portcaster | ( | void | ) | [static] |
Use this function to get a copy of the global {DjVuPortcaster}.
Definition at line 88 of file DjVuPort.cpp.
GP< DjVuFile > DjVuPort::id_to_file | ( | const DjVuPort * | source, | |
const GUTF8String & | id | |||
) | [virtual] |
This request is used to get a file corresponding to the given ID.
{DjVuDocument} is supposed to intercept it and either create a new instance of {DjVuFile} or reuse an existing one from the cache.
Reimplemented in DjVuDocument.
Definition at line 614 of file DjVuPort.cpp.
GURL DjVuPort::id_to_url | ( | const DjVuPort * | source, | |
const GUTF8String & | id | |||
) | [virtual] |
This request is issued to request translation of the ID, used in an DjVu INCL chunk to a URL, which may be used to request data associated with included file.
{DjVuDocument} usually intercepts all such requests, and the user doesn't have to worry about the translation
Reimplemented in DjVuDocument.
Definition at line 611 of file DjVuPort.cpp.
bool DjVuPort::inherits | ( | const GUTF8String & | class_name | ) | const [inline, virtual] |
Should return 1 if the called class inherits class class_name#.
When a destination receives a request, it can retrieve the pointer to the source DjVuPort#. This virtual function should be able to help to identify the source of the request. For example, {DjVuFile} is also derived from DjVuPort#. In order for the receiver to recognize the sender, the {DjVuFile} should override this function to return TRUE# when the class_name# is either DjVuPort# or DjVuFile#
Reimplemented in DjVuDocEditor, DjVuDocument, DjVuFile, DjVuSimplePort, and DjVuMemoryPort.
Definition at line 490 of file DjVuPort.h.
void DjVuPort::notify_chunk_done | ( | const DjVuPort * | source, | |
const GUTF8String & | name | |||
) | [virtual] |
This notification is sent when a new chunk has been decoded.
Reimplemented in DjVuFile, and DjVuImage.
Definition at line 632 of file DjVuPort.cpp.
void DjVuPort::notify_decode_progress | ( | const DjVuPort * | source, | |
float | done | |||
) | [virtual] |
This notification is sent from time to time while decoding is in progress.
The purpose is obvious: to provide a way to know how much is done and how long the decoding will continue. Argument done# is a number from 0 to 1 reflecting the progress.
Definition at line 641 of file DjVuPort.cpp.
virtual void DjVuPort::notify_doc_flags_changed | ( | const class DjVuDocument * | source, | |
long | set_mask, | |||
long | clr_mask | |||
) | [virtual] |
This notification is sent after the {DjVuDocument} flags have been changed.
This happens, for example, after it receives enough data and can determine its structure (BUNDLED#, OLD_INDEXED#, etc.).
- Parameters:
-
source {DjVuDocument}, which flags have been changed set_mask bits, which have been set clr_mask bits, which have been cleared
bool DjVuPort::notify_error | ( | const DjVuPort * | source, | |
const GUTF8String & | msg | |||
) | [virtual] |
This notification is sent when an error occurs and the error message should be shown to the user.
The receiver should return #0# if it is unable to process the request. Otherwise the receiver should return 1.
Reimplemented in DjVuErrorList, and DjVuSimplePort.
Definition at line 620 of file DjVuPort.cpp.
virtual void DjVuPort::notify_file_flags_changed | ( | const class DjVuFile * | source, | |
long | set_mask, | |||
long | clr_mask | |||
) | [virtual] |
This notification is sent after the {DjVuFile} flags have been changed.
This happens, for example, when: {itemize} Decoding succeeded, failed or just stopped All data has been received All included files have been created {itemize}
- Parameters:
-
source {DjVuFile}, which flags have been changed set_mask bits, which have been set clr_mask bits, which have been cleared
virtual void DjVuPort::notify_redisplay | ( | const class DjVuImage * | source | ) | [virtual] |
This notification is sent by {DjVuImage} when it should be redrawn.
It may be used to implement progressive redisplay.
- Parameters:
-
source The sender of the request
virtual void DjVuPort::notify_relayout | ( | const class DjVuImage * | source | ) | [virtual] |
This notification is sent by DjVuImage} when its geometry has been changed as a result of decoding.
It may be used to implement progressive redisplay.
bool DjVuPort::notify_status | ( | const DjVuPort * | source, | |
const GUTF8String & | msg | |||
) | [virtual] |
This notification is sent to update the decoding status.
The receiver should return #0# if it is unable to process the request. Otherwise the receiver should return 1.
Reimplemented in DjVuErrorList, and DjVuSimplePort.
Definition at line 623 of file DjVuPort.cpp.
void DjVuPort::operator delete | ( | void * | addr | ) | [static] |
Definition at line 178 of file DjVuPort.cpp.
void * DjVuPort::operator new | ( | size_t | sz | ) | [static] |
Definition at line 121 of file DjVuPort.cpp.
Copy operator.
Similarly to the copy constructor, the portcaster copies all incoming and outgoing coming routes of the original.
Definition at line 227 of file DjVuPort.cpp.
This request is issued when decoder needs additional data for decoding.
Both {DjVuFile} and {DjVuDocument} are initialized with a URL, not the document data. As soon as they need the data, they call this function, whose responsibility is to locate the source of the data basing on the URL# passed and return it back in the form of the {DataPool}. If this particular receiver is unable to fullfil the request, it should return #0#.
Reimplemented in DjVuDocEditor, DjVuDocument, DjVuErrorList, DjVuSimplePort, and DjVuMemoryPort.
Definition at line 617 of file DjVuPort.cpp.
The documentation for this class was generated from the following files: