kviewshell
DjVuFile Class Reference
DjVuFile# plays the central role in decoding {DjVuImage}s. More...
#include <DjVuFile.h>
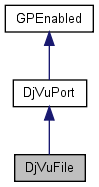
Public Types | |
enum | { DECODING = 1, DECODE_OK = 2, DECODE_FAILED = 4, DECODE_STOPPED = 8, DATA_PRESENT = 16, ALL_DATA_PRESENT = 32, INCL_FILES_CREATED = 64, MODIFIED = 128, DONT_START_DECODE = 256, STOPPED = 512, BLOCKED_STOPPED = 1024, CAN_COMPRESS = 2048, NEEDS_COMPRESSION = 4096 } |
enum | { STARTED = 1, FINISHED = 2 } |
Public Member Functions | |
void | change_info (GP< DjVuInfo > info, const bool do_reset=false) |
void | change_meta (const GUTF8String &meta, const bool do_reset=false) |
void | change_text (GP< DjVuTXT > txt, const bool do_reset=false) |
bool | contains_anno (void) |
bool | contains_chunk (const GUTF8String &chunk_name) |
bool | contains_meta (void) |
bool | contains_text (void) |
GP< DjVuNavDir > | find_ndir (void) |
GP< ByteStream > | get_anno (void) |
GUTF8String | get_chunk_name (int chunk_num) |
int | get_chunks_number (void) |
GPList< DjVuFile > | get_included_files (bool only_created=true) |
GP< DataPool > | get_init_data_pool (void) const |
unsigned int | get_memory_usage (void) const |
GP< ByteStream > | get_merged_anno (const GList< GURL > &ignore_list, int *max_level_ptr) |
GP< ByteStream > | get_merged_anno (int *max_level_ptr=0) |
void | get_meta (ByteStream &out) |
GP< ByteStream > | get_meta (void) |
void | get_text (ByteStream &out) |
GP< ByteStream > | get_text (void) |
void | init (const GURL &url, GP< DjVuPort > port=0) |
void | init (const GP< ByteStream > &str) |
void | insert_file (const GUTF8String &id, int chunk_num=1) |
void | merge_anno (ByteStream &out) |
void | move (const GURL &dir_url) |
virtual void | notify_chunk_done (const DjVuPort *source, const GUTF8String &name) |
virtual void | notify_file_flags_changed (const DjVuFile *source, long set_mask, long clr_mask) |
void | rebuild_data_pool (void) |
void | remove_anno (void) |
void | remove_meta (void) |
void | remove_text (void) |
virtual void | report_error (const GException &ex, const bool=true) |
void | set_name (const GUTF8String &name) |
void | unlink_file (const GUTF8String &id) |
virtual | ~DjVuFile (void) |
#DjVuFile# flags query functions | |
bool | are_incl_files_created (void) const |
bool | can_compress (void) const |
long | get_flags (void) const |
bool | is_all_data_present (void) const |
bool | is_data_present (void) const |
bool | is_decode_failed (void) const |
bool | is_decode_ok (void) const |
bool | is_decode_stopped (void) const |
bool | is_decoding (void) const |
bool | is_modified (void) const |
bool | needs_compression (void) const |
void | set_can_compress (bool m) |
void | set_modified (bool m) |
void | set_needs_compression (bool m) |
Decode control routines | |
GP< DjVuNavDir > | decode_ndir (void) |
void | process_incl_chunks (void) |
void | reset (void) |
bool | resume_decode (const bool sync=false) |
void | start_decode (void) |
void | stop (bool only_blocked) |
void | stop_decode (bool sync) |
void | wait_for_finish (void) |
DjVuFile.h | |
Files #"DjVuFile.h"# and #"DjVuFile.cpp"# contain implementation of the {DjVuFile} class, which takes the leading role in decoding of {DjVuImage}s. In the previous releases of the library the work of decoding has been entirely done in {DjVuImage}. Now, due to the introduction of multipage documents, the decoding procedure became significantly more complex and has been moved out from {DjVuImage} into {DjVuFile}. There is not much point though in creating just {DjVuFile} alone. The maximum power of the decoder is achieved when you create the {DjVuDocument} and work with { it} when decoding the image. Classes representing DjVu files.
| |
void | disable_standard_port (void) |
GSafeFlags & | get_safe_flags (void) |
GURL | get_url (void) const |
virtual bool | inherits (const GUTF8String &class_name) const |
virtual void | set_recover_errors (const ErrorRecoveryAction=ABORT) |
virtual void | set_verbose_eof (const bool verbose_eof=true) |
Encoding routines | |
GP< ByteStream > | get_djvu_bytestream (const bool included_too, const bool no_ndir=true) |
GP< DataPool > | get_djvu_data (const bool included_too, const bool no_ndir=true) |
Static Public Member Functions | |
static GP< DjVuFile > | create (const GURL &url, GP< DjVuPort > port=0, const ErrorRecoveryAction recover_action=ABORT, const bool verbose_eof=true) |
static GP< DjVuFile > | create (const GP< ByteStream > &str, const ErrorRecoveryAction recover_action=ABORT, const bool verbose_eof=true) |
static void | set_decode_codec (GP< GPixmap >(*codec)(ByteStream &bs)) |
static GP< DataPool > | unlink_file (const GP< DataPool > &data, const GUTF8String &name) |
Public Attributes | |
Decoded file contents | |
GP< ByteStream > | anno |
GP< IW44Image > | bg44 |
GP< GPixmap > | bgpm |
GUTF8String | description |
GP< DjVuNavDir > | dir |
GP< DjVuPalette > | fgbc |
GP< JB2Image > | fgjb |
GP< JB2Dict > | fgjd |
GP< GPixmap > | fgpm |
int | file_size |
GP< DjVuInfo > | info |
GP< ByteStream > | meta |
GUTF8String | mimetype |
GP< ByteStream > | text |
Protected Member Functions | |
DjVuFile (void) | |
Protected Attributes | |
GCriticalSection | anno_lock |
int | chunks_number |
GP< DataPool > | data_pool |
GPList< DjVuFile > | inc_files_list |
GCriticalSection | inc_files_lock |
GCriticalSection | meta_lock |
ErrorRecoveryAction | recover_errors |
GCriticalSection | text_lock |
GURL | url |
bool | verbose_eof |
Detailed Description
DjVuFile# plays the central role in decoding {DjVuImage}s.First of all, it represents a DjVu file whether it's part of a multipage all-in-one-file DjVu document, or part of a multipage DjVu document where every page is in a separate file, or the whole single page document. DjVuFile# can read its contents from a file and store it back when necessary.
Second, DjVuFile# does the greatest part of decoding work. In the past this was the responsibility of {DjVuImage}. Now, with the introduction of the multipage DjVu formats, the decoding routines have been extracted from the {DjVuImage} and put into this separate class DjVuFile#.
As {DjVuImage} before, DjVuFile# now contains public class variables corresponding to every component, that can ever be decoded from a DjVu file (such as INFO# chunk, BG44# chunk, SJBZ# chunk, etc.).
As before, the decoding is initiated by a single function ({start_decode}() in this case, and {DjVuImage::decode}() before). The difference is that DjVuFile# now handles threads creation itself. When you call the {start_decode}() function, it creates the decoding thread, which starts decoding, and which can create additional threads: one per each file included into this one.
{ Inclusion} is also a new feature specifically designed for a multipage document. Indeed, inside a given document there can be a lot of things shared between its pages. Examples can be the document annotation ({DjVuAnno}) and other things like shared shapes and dictionary (to be implemented). To avoid putting these chunks into every page, we have invented new chunk called INCL# which purpose is to make the decoder open the specified file and decode it.
{ Source of data.} The DjVuFile# can be initialized in two ways: {itemize} With URL# and {DjVuPort}. In this case DjVuFile# will request its data thru the communication mechanism provided by {DjVuPort} in the constructor. If this file references (includes) any other file, data for them will also be requested in the same way. With {ByteStream}. In this case the DjVuFile# will read its data directly from the passed stream. This constructor has been added to simplify creation of DjVuFile::s, which do no include anything else. In this case the {ByteStream} is enough for the DjVuFile# to initialize. {itemize}
{ Progress information.} DjVuFile# does not do decoding silently. Instead, it sends a whole set of notifications through the mechanism provided by {DjVuPort} and {DjVuPortcaster}. It tells the user of the class about the progress of the decoding, about possible errors, chunk being decoded, etc. The data is requested using this mechanism too.
{ Creating.} Depending on where you have data of the DjVu file, the DjVuFile# can be initialized in two ways: {itemize} By providing URL# and pointer to {DjVuPort}. In this case DjVuFile# will request data using communication mechanism provided by {DjVuPort}. This is useful when the data is on the web or when this file includes other files. By providing a {ByteStream} with the data for the file. Use it only when the file doesn't include other files. {itemize} There is also a bunch of functions provided for composing the desired {DjVuDocument} and modifying DjVuFile# structure. The examples are {delete_chunks}(), {insert_chunk}(), {include_file}() and {unlink_file}().
{ Caching.} In the case of plugin it's important to do the caching of decoded images or files. DjVuFile# appears to be the best candidate for caching, and that's why it supports this procedure. Whenever a DjVuFile# is successfully decoded, it's added to the cache by {DjVuDocument}. Next time somebody needs it, it will be extracted from the cache directly by {DjVuDocument} and won't be decoded again.
{ URLs.} Historically the biggest strain is put on making the decoder available for Netscape and IE plugins where the original files reside somewhere in the net. That is why DjVuFile# uses { URLs} to identify itself and other files. If you're working with files on the hard disk, you have to use the local URLs instead of file names. A good way to do two way conversion is the {GOS} class. Sometimes it happens that a given file does not reside anywhere but the memory. No problem in this case either. There is a special port {DjVuMemoryPort}, which can associate any URL with the corresponding data in the memory. All you need to do is to invent your own URL prefix for this case. "#memory:#" will do. The usage of absolute URLs has many advantages among which is the capability to cache files with their URL being the cache key.
Please note, that the DjVuFile# class has been designed to work closely with {DjVuDocument}. So please review the documentation on this class too.
Definition at line 201 of file DjVuFile.h.
Member Enumeration Documentation
anonymous enum |
- Enumerator:
-
DECODING DECODE_OK DECODE_FAILED DECODE_STOPPED DATA_PRESENT ALL_DATA_PRESENT INCL_FILES_CREATED MODIFIED DONT_START_DECODE STOPPED BLOCKED_STOPPED CAN_COMPRESS NEEDS_COMPRESSION
Definition at line 204 of file DjVuFile.h.
anonymous enum |
Constructor & Destructor Documentation
DjVuFile::DjVuFile | ( | void | ) | [protected] |
DjVuFile::~DjVuFile | ( | void | ) | [virtual] |
Definition at line 255 of file DjVuFile.cpp.
Member Function Documentation
bool DjVuFile::are_incl_files_created | ( | void | ) | const [inline] |
Returns TRUE# if all included files have been created.
Only when this function returns 1, the {get_included_files}() returns the correct information.
Definition at line 744 of file DjVuFile.h.
bool DjVuFile::can_compress | ( | void | ) | const [inline] |
Definition at line 775 of file DjVuFile.h.
void DjVuFile::change_meta | ( | const GUTF8String & | meta, | |
const bool | do_reset = false | |||
) |
bool DjVuFile::contains_anno | ( | void | ) |
Returns TRUE# if the file contains annotation chunks.
Known annotation chunks at the time of writing this help are: { ANTa}, { ANTz}, { FORM:ANNO}.
Definition at line 2132 of file DjVuFile.cpp.
bool DjVuFile::contains_chunk | ( | const GUTF8String & | chunk_name | ) |
Returns 1 if this file contains chunk with name chunk_name#.
Definition at line 2091 of file DjVuFile.cpp.
bool DjVuFile::contains_meta | ( | void | ) |
Returns TRUE# if the file contains metadata chunks.
Known metadata chunks at the time of writing this help are: { METa}, and { METz}.
Definition at line 2176 of file DjVuFile.cpp.
bool DjVuFile::contains_text | ( | void | ) |
Returns TRUE# if the file contains hiddentext chunks.
Known hiddentext chunks at the time of writing this help are: { TXTa}, and { TXTz}.
Definition at line 2154 of file DjVuFile.cpp.
GP< DjVuFile > DjVuFile::create | ( | const GURL & | url, | |
GP< DjVuPort > | port = 0 , |
|||
const ErrorRecoveryAction | recover_action = ABORT , |
|||
const bool | verbose_eof = true | |||
) | [static] |
Creator, does the init(const GURL &url, GP<DjVuPort> port=0).
Definition at line 209 of file DjVuFile.cpp.
GP< DjVuFile > DjVuFile::create | ( | const GP< ByteStream > & | str, | |
const ErrorRecoveryAction | recover_action = ABORT , |
|||
const bool | verbose_eof = true | |||
) | [static] |
GP< DjVuNavDir > DjVuFile::decode_ndir | ( | void | ) |
Looks for NDIR# chunk (navigation directory), and decodes its contents.
If the NDIR# chunk has not been found in {this} file, but this file includes others, the procedure will continue recursively. This function is useful to obtain the document navigation directory before any page has been decoded. After it returns the directory can be obtained by calling {find_ndir}() function.
{ Warning.} Contrary to {start_decode}(), this function does not return before it completely decodes the directory. Make sure, that this file and all included files have enough data.
Definition at line 1560 of file DjVuFile.cpp.
void DjVuFile::disable_standard_port | ( | void | ) | [inline] |
Disables the built-in port for accessing local files, which may have been created in the case when the port# argument to the {DjVuFile::DjVuFile}() constructor is ZERO#.
Definition at line 797 of file DjVuFile.h.
GP< DjVuNavDir > DjVuFile::find_ndir | ( | void | ) |
Looks for decoded# navigation directory ({DjVuNavDir}) in this or included files.
Returns ZERO# if nothing could be found.
{ Note.} This function does { not} attempt to decode NDIR# chunks. It is looking for predecoded components. NDIR# can be decoded either during regular decoding (initiated by {start_decode}() function) or by {decode_ndir}() function, which processes this and included files recursively in search of NDIR# chunks and decodes them.
Definition at line 1483 of file DjVuFile.cpp.
GP< ByteStream > DjVuFile::get_anno | ( | void | ) |
Returns the annotation chunks (#"ANTa"# and #"ANTz"#).
This function may be used even when the DjVuFile# has not been decoded yet. If all data has been received for this DjVuFile#, it will gather hidden text and return the result. If no hidden text has been found, ZERO# will be returned.
{ Summary:} This function will return complete annotations only when the {is_all_data_present}() returns TRUE#.
Definition at line 1847 of file DjVuFile.cpp.
GUTF8String DjVuFile::get_chunk_name | ( | int | chunk_num | ) |
int DjVuFile::get_chunks_number | ( | void | ) |
GP< ByteStream > DjVuFile::get_djvu_bytestream | ( | const bool | included_too, | |
const bool | no_ndir = true | |||
) |
The main function that encodes data back into binary stream.
The data returned will reflect possible changes made into the chunk structure, annotation chunks and navigation directory chunk NDIR#.
{ Note:} The file stream will not have the magic #0x41,0x54,0x26,0x54# at the beginning.
- Parameters:
-
included_too Process included files too.
Definition at line 2351 of file DjVuFile.cpp.
Same as {get_djvu_bytestream}(), returning a DataPool.
- Parameters:
-
included_too Process included files too.
Definition at line 2367 of file DjVuFile.cpp.
long DjVuFile::get_flags | ( | void | ) | const [inline] |
Returns the DjVuFile# flags.
The value returned is the result of ORing one or more of the following constants: {itemize} DECODING# The decoding is in progress DECODE_OK# The decoding has finished successfully DECODE_FAILED# The decoding has failed DECODE_STOPPED# The decoding has been stopped by {stop_decode}() function DATA_PRESENT# All data for this file has been received. It's especially important in the case of Netscape or IE plugins when the data is being received while the decoding is done. ALL_DATA_PRESENT# Not only data for this file, but also for all included file has been received. INCL_FILES_CREATED# All INCL# and INCF# chunks have been processed and the corresponding DjVuFile::s created. This is important to know to be sure that the list returned by {get_included_files}() is OK. {itemize}
Definition at line 696 of file DjVuFile.h.
Returns the list of included DjVuFiles.
{ Warning.} Included files are normally created during decoding. Before that they do not exist. If you call this function at that time and set only_created# to FALSE# then it will have to read all the data from this file in order to find INCL# chunks, which may block your application, if not all data is available.
- Parameters:
-
only_created If TRUE#, the file will not try to process INCL# chunks and load referenced files. It will return just those files, which have already been created during the decoding procedure.
Definition at line 311 of file DjVuFile.cpp.
Definition at line 585 of file DjVuFile.h.
unsigned int DjVuFile::get_memory_usage | ( | void | ) | const |
Definition at line 296 of file DjVuFile.cpp.
GP< ByteStream > DjVuFile::get_merged_anno | ( | const GList< GURL > & | ignore_list, | |
int * | max_level_ptr | |||
) |
Goes down the hierarchy of DjVuFile::s and merges their annotations.
(shouldn't this one be private?).
- Parameters:
-
max_level_ptr If this pointer is not ZERO, the function will use it to store the maximum level at which annotations were found. Top-level page files have ZERO level#. ignore_list The function will not process included DjVuFile::s with URLs matching those mentioned in this ignore_list#.
Definition at line 1648 of file DjVuFile.cpp.
GP< ByteStream > DjVuFile::get_merged_anno | ( | int * | max_level_ptr = 0 |
) |
Processes the included files hierarchy and returns merged annotations.
This function may be used even when the DjVuFile# has not been decoded yet. If all data has been received for this DjVuFile# and all included DjVuFile::s, it will will gather annotations from them and will return the result. If no annotations have been found, ZERO# will be returned. If either this DjVuFile# or any of the included files do not have all the data, the function will use the results of decoding, which may have been started with the {start_decode}() function. Otherwise ZERO# will be returned as well.
If max_level_ptr# pointer is not zero, the function will use it to store the maximum level number from which annotations have been obtained. ZERO# level corresponds to the top-level page file.
{ Summary:} This function will return complete annotations only when the {is_all_data_present}() returns TRUE#.
Definition at line 1672 of file DjVuFile.cpp.
void DjVuFile::get_meta | ( | ByteStream & | out | ) |
Definition at line 2411 of file DjVuFile.cpp.
GP< ByteStream > DjVuFile::get_meta | ( | void | ) |
Returns the meta chunks (#"METa"# and #"METz"#).
This function may be used even when the DjVuFile# has not been decoded yet. If all data has been received for this DjVuFile#, it will gather metadata and return the result. If no hidden text has been found, ZERO# will be returned.
{ Summary:} This function will return complete meta data only when the {is_all_data_present}() returns TRUE#.
Definition at line 1881 of file DjVuFile.cpp.
GSafeFlags & DjVuFile::get_safe_flags | ( | void | ) | [inline] |
Definition at line 702 of file DjVuFile.h.
void DjVuFile::get_text | ( | ByteStream & | out | ) |
Definition at line 2396 of file DjVuFile.cpp.
GP< ByteStream > DjVuFile::get_text | ( | void | ) |
Returns the text chunks (#"TXTa"# and #"TXTz"#).
This function may be used even when the DjVuFile# has not been decoded yet. If all data has been received for this DjVuFile#, it will gather hidden text and return the result. If no hidden text has been found, ZERO# will be returned.
{ Summary:} This function will return complete hidden text layers only when the {is_all_data_present}() returns TRUE#.
Definition at line 1864 of file DjVuFile.cpp.
GURL DjVuFile::get_url | ( | void | ) | const [inline] |
bool DjVuFile::inherits | ( | const GUTF8String & | class_name | ) | const [inline, virtual] |
Should return 1 if the called class inherits class class_name#.
When a destination receives a request, it can retrieve the pointer to the source DjVuPort#. This virtual function should be able to help to identify the source of the request. For example, {DjVuFile} is also derived from DjVuPort#. In order for the receiver to recognize the sender, the {DjVuFile} should override this function to return TRUE# when the class_name# is either DjVuPort# or DjVuFile#
Reimplemented from DjVuPort.
Definition at line 803 of file DjVuFile.h.
Initializes a DjVuFile# object.
As you can notice, the data is not directly passed to this function. The DjVuFile# will ask for it through the {DjVuPort} mechanism before the constructor finishes. If the data is stored locally on the hard disk then the pointer to {DjVuPort} may be set to ZERO#, which will make DjVuFile# read all data from the hard disk and report all errors to stderr#.
{ Note}. If the file includes (by means of INCL# chunks) other files then you should be ready to {enumerate} Reply to requests {DjVuPort::id_to_url}() issued to translate IDs (used in INCL# chunks) to absolute URLs. Usually, when the file is created by {DjVuDocument} this job is done by it. If you construct such a file manually, be prepared to do the ID to URL translation Provide data for all included files. {enumerate}
- Parameters:
-
url The URL assigned to this file. It will be used when the DjVuFile# asks for data. port All communication between DjVuFile::s and {DjVuDocument}s is done through the {DjVuPort} mechanism. If the {url} is not local or the data does not reside on the hard disk, the {port} parameter must not be ZERO#. If the {port} is ZERO# then DjVuFile# will create an internal instance of {DjVuSimplePort} for accessing local files and reporting errors. It can later be disabled by means of {disable_standard_port}() function.
Definition at line 222 of file DjVuFile.cpp.
void DjVuFile::init | ( | const GP< ByteStream > & | str | ) |
Initializes a DjVuFile# object.
This is a simplified initializer, which is not supposed to be used for decoding or creating DjVuFile::s, which include other files.
If the file is stored on the hard drive, you may also use the other constructor and pass it the file's URL and ZERO# port#. The DjVuFile# will read the data itself.
If you want to receive error messages and notifications, you may connect the DjVuFile# to your own {DjVuPort} after it has been constructed.
- Parameters:
-
str The stream containing data for the file.
Definition at line 179 of file DjVuFile.cpp.
void DjVuFile::insert_file | ( | const GUTF8String & | id, | |
int | chunk_num = 1 | |||
) |
Includes a DjVuFile# with the specified id# into this one.
This function will also insert an INCL# chunk at position chunk_num#. The function will request data for the included file and will create it before returning.
Definition at line 2630 of file DjVuFile.cpp.
bool DjVuFile::is_all_data_present | ( | void | ) | const [inline] |
Returns TRUE# if this file { and} all included files have received all data.
Definition at line 738 of file DjVuFile.h.
bool DjVuFile::is_data_present | ( | void | ) | const [inline] |
bool DjVuFile::is_decode_failed | ( | void | ) | const [inline] |
bool DjVuFile::is_decode_ok | ( | void | ) | const [inline] |
Returns TRUE# if decoding of the file has finished successfully.
Definition at line 714 of file DjVuFile.h.
bool DjVuFile::is_decode_stopped | ( | void | ) | const [inline] |
Returns TRUE# if decoding of the file has been stopped by {stop_decode}() function.
Definition at line 726 of file DjVuFile.h.
bool DjVuFile::is_decoding | ( | void | ) | const [inline] |
bool DjVuFile::is_modified | ( | void | ) | const [inline] |
Definition at line 750 of file DjVuFile.h.
void DjVuFile::merge_anno | ( | ByteStream & | out | ) |
Definition at line 2374 of file DjVuFile.cpp.
void DjVuFile::move | ( | const GURL & | dir_url | ) |
Definition at line 1984 of file DjVuFile.cpp.
bool DjVuFile::needs_compression | ( | void | ) | const [inline] |
Definition at line 762 of file DjVuFile.h.
void DjVuFile::notify_chunk_done | ( | const DjVuPort * | source, | |
const GUTF8String & | name | |||
) | [virtual] |
This notification is sent when a new chunk has been decoded.
Reimplemented from DjVuPort.
Definition at line 391 of file DjVuFile.cpp.
void DjVuFile::notify_file_flags_changed | ( | const DjVuFile * | source, | |
long | set_mask, | |||
long | clr_mask | |||
) | [virtual] |
Definition at line 400 of file DjVuFile.cpp.
void DjVuFile::process_incl_chunks | ( | void | ) |
Processes INCL# chunks and creates included files.
Normally you won't need to call this function because included files are created automatically when the file is being decoded. But if due to some reason you'd like to obtain the list of included files without decoding this file, this is an ideal function to call.
{ Warning.} This function does not return before it reads the whole file, which may block your application under some circumstances if not all data is available.
Definition at line 691 of file DjVuFile.cpp.
void DjVuFile::rebuild_data_pool | ( | void | ) |
Definition at line 2552 of file DjVuFile.cpp.
void DjVuFile::remove_anno | ( | void | ) |
void DjVuFile::remove_meta | ( | void | ) |
void DjVuFile::remove_text | ( | void | ) |
void DjVuFile::report_error | ( | const GException & | ex, | |
const | bool = true | |||
) | [virtual] |
Definition at line 660 of file DjVuFile.cpp.
void DjVuFile::reset | ( | void | ) |
bool DjVuFile::resume_decode | ( | const bool | sync = false |
) |
Start the decode iff not already decoded.
If sync is true, wait wait for decode to complete. Returns true of start_decode is called.
Definition at line 1368 of file DjVuFile.cpp.
void DjVuFile::set_can_compress | ( | bool | m | ) | [inline] |
Definition at line 781 of file DjVuFile.h.
void DjVuFile::set_decode_codec | ( | GP< GPixmap >(*)(ByteStream &bs) | codec | ) | [static] |
Definition at line 1220 of file DjVuFile.cpp.
void DjVuFile::set_modified | ( | bool | m | ) | [inline] |
Definition at line 756 of file DjVuFile.h.
void DjVuFile::set_name | ( | const GUTF8String & | name | ) |
Internal.
Used by DjVuDocument. The name# should { not} be encoded with {GOS::encode_reserved}().
Definition at line 1996 of file DjVuFile.cpp.
void DjVuFile::set_needs_compression | ( | bool | m | ) | [inline] |
Definition at line 768 of file DjVuFile.h.
void DjVuFile::set_recover_errors | ( | const ErrorRecoveryAction | action = ABORT |
) | [inline, virtual] |
Definition at line 834 of file DjVuFile.h.
void DjVuFile::set_verbose_eof | ( | const bool | verbose_eof = true |
) | [inline, virtual] |
Definition at line 827 of file DjVuFile.h.
void DjVuFile::start_decode | ( | void | ) |
Starts decode.
If threads are enabled, the decoding will be done in another thread. Be sure to use {wait_for_finish}() or listen for notifications sent through the {DjVuPortcaster} to remain in sync.
Definition at line 1325 of file DjVuFile.cpp.
void DjVuFile::stop | ( | bool | only_blocked | ) |
Recursively stops all data-related operations.
Depending on the value of only_blocked# flag this works as follows: {itemize} If only_blocked# is TRUE#, the function will make sure, that any further access to the file's data will result in a STOP# exception if the desired data is not available (and the thread would normally block). If only_blocked# is FALSE#, then { any} further access to the file's data will result in immediate STOP# exception. {itemize}
The action of this function is recursive, meaning that any DjVuFile# included into this one will also be stopped.
Use this function when you don't need the DjVuFile# anymore. The results cannot be undone, and the whole idea is to make all threads working with this file exit with the STOP# exception.
Definition at line 1444 of file DjVuFile.cpp.
void DjVuFile::stop_decode | ( | bool | sync | ) |
Stops decode.
If sync# is 1 then the function will not return until the decoding thread actually dies. Otherwise it will just signal the thread to stop and will return immediately. Decoding of all included files will be stopped too.
Definition at line 1387 of file DjVuFile.cpp.
GP< DataPool > DjVuFile::unlink_file | ( | const GP< DataPool > & | data, | |
const GUTF8String & | name | |||
) | [static] |
Will find an INCL# chunk containing name# in input data# and will remove it.
Definition at line 2563 of file DjVuFile.cpp.
void DjVuFile::unlink_file | ( | const GUTF8String & | id | ) |
void DjVuFile::wait_for_finish | ( | void | ) | [inline] |
Wait for the decoding to finish.
This will wait for the termination of included files too.
Definition at line 813 of file DjVuFile.h.
Member Data Documentation
GCriticalSection DjVuFile::anno_lock [protected] |
Definition at line 625 of file DjVuFile.h.
Pointer to the background component of DjVu image (IW44 encoded).
Definition at line 215 of file DjVuFile.h.
int DjVuFile::chunks_number [protected] |
Definition at line 630 of file DjVuFile.h.
GP<DataPool> DjVuFile::data_pool [protected] |
Definition at line 621 of file DjVuFile.h.
Pointer to the *old* navigation directory contained in this file.
Definition at line 233 of file DjVuFile.h.
Pointer to a colors vector for the foreground component of DjVu image.
Definition at line 225 of file DjVuFile.h.
Pointer to the mask of foreground component of DjVu image (JB2 encoded).
Definition at line 219 of file DjVuFile.h.
Pointer to the optional shape dictionary for the mask (JB2 encoded).
Definition at line 221 of file DjVuFile.h.
Pointer to a colors layer for the foreground component of DjVu image.
Definition at line 223 of file DjVuFile.h.
GPList<DjVuFile> DjVuFile::inc_files_list [protected] |
Definition at line 623 of file DjVuFile.h.
GCriticalSection DjVuFile::inc_files_lock [protected] |
Definition at line 624 of file DjVuFile.h.
GCriticalSection DjVuFile::meta_lock [protected] |
Definition at line 627 of file DjVuFile.h.
ErrorRecoveryAction DjVuFile::recover_errors [protected] |
Definition at line 628 of file DjVuFile.h.
GCriticalSection DjVuFile::text_lock [protected] |
Definition at line 626 of file DjVuFile.h.
GURL DjVuFile::url [protected] |
Definition at line 620 of file DjVuFile.h.
bool DjVuFile::verbose_eof [protected] |
Definition at line 629 of file DjVuFile.h.
The documentation for this class was generated from the following files: