kviewshell
IW44Image Class Reference
IW44 encoded gray-level and color images. More...
#include <IW44Image.h>
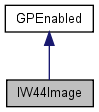
Public Types | |
enum | CRCBMode { CRCBnone, CRCBhalf, CRCBnormal, CRCBfull } |
enum | ImageType { GRAY = false, COLOR = true } |
Public Member Functions | |
virtual void | close_codec (void)=0 |
virtual int | decode_chunk (GP< ByteStream > gbs)=0 |
virtual void | decode_iff (IFFByteStream &iff, int maxchunks=999)=0 |
virtual int | encode_chunk (GP< ByteStream > gbs, const IWEncoderParms &parms) |
virtual void | encode_iff (IFFByteStream &iff, int nchunks, const IWEncoderParms *parms) |
virtual GP< GBitmap > | get_bitmap (int subsample, const GRect &rect) |
virtual GP< GBitmap > | get_bitmap (void) |
int | get_height (void) const |
virtual unsigned int | get_memory_usage (void) const =0 |
virtual int | get_percent_memory (void) const =0 |
virtual GP< GPixmap > | get_pixmap (int subsample, const GRect &rect) |
virtual GP< GPixmap > | get_pixmap (void) |
virtual int | get_serial (void)=0 |
int | get_width (void) const |
virtual int | parm_crcbdelay (const int parm) |
virtual void | parm_dbfrac (float frac)=0 |
virtual | ~IW44Image () |
Static Public Member Functions | |
static GP< IW44Image > | create_decode (const ImageType itype=COLOR) |
static GP< IW44Image > | create_encode (const GPixmap &bm, const GP< GBitmap > mask=0, CRCBMode crcbmode=CRCBnormal) |
static GP< IW44Image > | create_encode (const GBitmap &bm, const GP< GBitmap > mask=0) |
static GP< IW44Image > | create_encode (const ImageType itype=COLOR) |
Protected Member Functions | |
IW44Image (void) | |
Protected Attributes | |
Map * | cbmap |
int | cbytes |
Map * | crmap |
int | cserial |
int | cslice |
float | db_frac |
Map * | ymap |
Detailed Description
IW44 encoded gray-level and color images.This class acts as a base for images represented as a collection of IW44 wavelet coefficients. The coefficients are stored in a memory efficient data structure. Member function {get_bitmap} renders an arbitrary segment of the image into a {GBitmap}. Member functions {decode_iff} and {encode_iff} read and write DjVu IW44 files (see {IW44Image.h}). Both the copy constructor and the copy operator are declared as private members. It is therefore not possible to make multiple copies of instances of this class.
Definition at line 226 of file IW44Image.h.
Member Enumeration Documentation
enum IW44Image::CRCBMode |
Chrominance processing selector.
The following constants may be used as argument to the following {IWPixmap} constructor to indicate how the chrominance information should be processed. There are four possible values: {description} [CRCBnone:] The wavelet transform will discard the chrominance information and only keep the luminance. The image will show in shades of gray. [CRCBhalf:] The wavelet transform will process the chrominance at only half the image resolution. This option creates smaller files but may create artifacts in highly colored images. [CRCBnormal:] The wavelet transform will process the chrominance at full resolution. This is the default. [CRCBfull:] The wavelet transform will process the chrominance at full resolution. This option also disables the chrominance encoding delay (see {parm_crcbdelay}) which usually reduces the bitrate associated with the chrominance information. {description}
Definition at line 245 of file IW44Image.h.
enum IW44Image::ImageType |
Constructor & Destructor Documentation
IW44Image::IW44Image | ( | void | ) | [protected] |
Definition at line 1327 of file IW44Image.cpp.
IW44Image::~IW44Image | ( | ) | [virtual] |
Definition at line 1333 of file IW44Image.cpp.
Member Function Documentation
virtual void IW44Image::close_codec | ( | void | ) | [pure virtual] |
Resets the encoder/decoder state.
The first call to decode_chunk# or encode_chunk# initializes the coder for encoding or decoding. Function close_codec# must be called after processing the last chunk in order to reset the coder and release the associated memory.
Null constructor.
Constructs an empty IW44Image object. This object does not contain anything meaningful. You must call function {init}, {decode_iff} or {decode_chunk} to populate the wavelet coefficient data structure. You may not use {encode_iff} or {encode_chunk}.
Definition at line 1341 of file IW44Image.cpp.
GP< IW44Image > IW44Image::create_encode | ( | const GPixmap & | bm, | |
const GP< GBitmap > | mask = 0 , |
|||
CRCBMode | crcbmode = CRCBnormal | |||
) | [static] |
Initializes an IWPixmap with color image bm#.
This constructor performs the wavelet decomposition of image bm# and records the corresponding wavelet coefficient. Argument mask# is an optional bilevel image specifying the masked pixels (see {IW44Image.h}). Argument crcbmode# specifies how the chrominance information should be encoded (see {CRCBMode}).
Definition at line 1558 of file IW44EncodeCodec.cpp.
GP< IW44Image > IW44Image::create_encode | ( | const GBitmap & | bm, | |
const GP< GBitmap > | mask = 0 | |||
) | [static] |
Initializes an IWBitmap with image bm#.
This constructor performs the wavelet decomposition of image bm# and records the corresponding wavelet coefficient. Argument mask# is an optional bilevel image specifying the masked pixels (see {IW44Image.h}).
Definition at line 1396 of file IW44EncodeCodec.cpp.
Null constructor.
Constructs an empty IW44Image object. This object does not contain anything meaningful. You must call function {init}, {decode_iff} or {decode_chunk} to populate the wavelet coefficient data structure. You may then use {encode_iff} and {encode_chunk}.
Definition at line 1382 of file IW44EncodeCodec.cpp.
virtual int IW44Image::decode_chunk | ( | GP< ByteStream > | gbs | ) | [pure virtual] |
Decodes one data chunk from ByteStream bs#.
Successive calls to decode_chunk# decode successive chunks. You must call close_codec# after decoding the last chunk of a file. Note that function get_bitmap# and decode_chunk# may be called simultaneously from two execution threads.
virtual void IW44Image::decode_iff | ( | IFFByteStream & | iff, | |
int | maxchunks = 999 | |||
) | [pure virtual] |
This function enters a composite chunk (identifier FORM:BM44#, or FORM:PM44#), and decodes a maximum of maxchunks# data chunks (identifier BM44#).
Data for each chunk is processed using the function decode_chunk#.
int IW44Image::encode_chunk | ( | GP< ByteStream > | gbs, | |
const IWEncoderParms & | parms | |||
) | [virtual] |
Encodes one data chunk into ByteStream bs#.
Parameter parms# controls how much data is generated. The chunk data is written to ByteStream bs# with no IFF header. Successive calls to encode_chunk# encode successive chunks. You must call close_codec# after encoding the last chunk of a file.
Definition at line 1355 of file IW44Image.cpp.
void IW44Image::encode_iff | ( | IFFByteStream & | iff, | |
int | nchunks, | |||
const IWEncoderParms * | parms | |||
) | [virtual] |
Writes a gray level image into DjVu IW44 file.
This function creates a composite chunk (identifier FORM:BM44# or FORM:PM44#) composed of nchunks# chunks (identifier BM44# or PM44#). Data for each chunk is generated with encode_chunk# using the corresponding parameters in array parms#.
Definition at line 1362 of file IW44Image.cpp.
Reconstructs a segment of the image at a given scale.
The subsampling ratio subsample# must be a power of two between #1# and #32#. Argument rect# specifies which segment of the subsampled image should be reconstructed. The reconstructed image is returned as a GBitmap object whose size is equal to the size of the rectangle rect#.
Definition at line 303 of file IW44Image.h.
Reconstructs the complete image.
The reconstructed image is then returned as a GBitmap object.
Definition at line 297 of file IW44Image.h.
int IW44Image::get_height | ( | void | ) | const |
virtual unsigned int IW44Image::get_memory_usage | ( | void | ) | const [pure virtual] |
Returns the amount of memory used by the wavelet coefficients.
This amount of memory is expressed in bytes.
virtual int IW44Image::get_percent_memory | ( | void | ) | const [pure virtual] |
Returns the filling ratio of the internal data structure.
Wavelet coefficients are stored in a sparse array. This function tells what percentage of bins have been effectively allocated.
Reconstructs a segment of the image at a given scale.
The subsampling ratio subsample# must be a power of two between #1# and #32#. Argument rect# specifies which segment of the subsampled image should be reconstructed. The reconstructed image is returned as a GPixmap object whose size is equal to the size of the rectangle rect#.
Definition at line 312 of file IW44Image.h.
Reconstructs the complete image.
The reconstructed image is then returned as a GPixmap object.
Definition at line 306 of file IW44Image.h.
virtual int IW44Image::get_serial | ( | void | ) | [pure virtual] |
Returns the chunk serial number.
This function returns the serial number of the last chunk encoded with encode_chunk# or decoded with decode_chunk#. The first chunk always has serial number #1#. Successive chunks have increasing serial numbers. Value #0# is returned if this function is called before calling encode_chunk# or decode_chunk# or after calling close_codec#.
int IW44Image::get_width | ( | void | ) | const |
virtual int IW44Image::parm_crcbdelay | ( | const int | parm | ) | [inline, virtual] |
Sets the chrominance delay parameter.
This function can be called before encoding the first color IW44 data chunk. Parameter parm# is an encoding delay which reduces the bitrate associated with the chrominance information. The default chrominance encoding delay is 10.
Definition at line 362 of file IW44Image.h.
virtual void IW44Image::parm_dbfrac | ( | float | frac | ) | [pure virtual] |
Sets the dbfrac# parameter.
This function can be called before encoding the first IW44 data chunk. Parameter frac# modifies the decibel estimation algorithm in such a way that the decibel target only pertains to the average error of the fraction frac# of the most misrepresented 32x32 pixel blocks. Setting arguments frac# to #1.0# restores the normal behavior.
Member Data Documentation
Map * IW44Image::cbmap [protected] |
Definition at line 374 of file IW44Image.h.
int IW44Image::cbytes [protected] |
Definition at line 377 of file IW44Image.h.
Map * IW44Image::crmap [protected] |
Definition at line 374 of file IW44Image.h.
int IW44Image::cserial [protected] |
Definition at line 376 of file IW44Image.h.
int IW44Image::cslice [protected] |
Definition at line 375 of file IW44Image.h.
float IW44Image::db_frac [protected] |
Definition at line 372 of file IW44Image.h.
Map* IW44Image::ymap [protected] |
Definition at line 374 of file IW44Image.h.
The documentation for this class was generated from the following files: