kviewshell
DjVuDocEditor Class Reference
DjVuDocEditor# is an extension of {DjVuDocument} class with additional capabilities for editing the document contents. More...
#include <DjVuDocEditor.h>
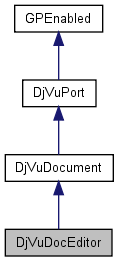
Public Member Functions | |
bool | can_be_saved (void) const |
void | create_shared_anno_file (void(*progress_cb)(float progress, void *)=0, void *cl_data=0) |
GURL | get_doc_url (void) const |
int | get_orig_doc_type (void) const |
int | get_save_doc_type (void) const |
GP< DjVuFile > | get_shared_anno_file (void) |
virtual bool | inherits (const GUTF8String &class_name) const |
GUTF8String | insert_file (const GURL &url, const GUTF8String &parent_id, int chunk_num=1, DjVuPort *source=0) |
void | insert_group (const GList< GURL > &furl_list, int page_num=-1, void(*refresh_cb)(void *)=0, void *cl_data=0) |
void | insert_page (GP< DataPool > &file_pool, const GURL &fname, int page_num=-1) |
void | insert_page (const GURL &fname, int page_num=-1) |
void | move_page (int page_num, int new_page_num) |
void | move_pages (const GList< int > &page_list, int shift) |
GUTF8String | page_to_id (int page_num) const |
void | remove_file (const GUTF8String &id, bool remove_unref=true) |
void | remove_page (int page_num, bool remove_unref=true) |
void | remove_pages (const GList< int > &page_list, bool remove_unref=true) |
virtual GP< DataPool > | request_data (const DjVuPort *source, const GURL &url) |
void | save (void) |
virtual void | save_as (const GURL &where, bool bundled) |
void | save_pages_as (const GP< ByteStream > &str, const GList< int > &page_list) |
void | set_djvm_nav (GP< DjVmNav > nav) |
void | set_file_name (const GUTF8String &id, const GUTF8String &name) |
void | set_file_title (const GUTF8String &id, const GUTF8String &title) |
void | set_page_name (int page_num, const GUTF8String &name) |
void | set_page_title (int page_num, const GUTF8String &title) |
void | simplify_anno (void(*progress_cb)(float progress, void *)=0, void *cl_data=0) |
virtual void | write (const GP< ByteStream > &str, const GMap< GUTF8String, void * > &reserved) |
virtual void | write (const GP< ByteStream > &str, bool force_djvm=false) |
virtual | ~DjVuDocEditor (void) |
Thumbnails | |
void | generate_thumbnails (int thumb_size, bool(*cb)(int page_num, void *)=0, void *cl_data=0) |
int | generate_thumbnails (int thumb_size, int page_num) |
int | get_thumbnails_num (void) const |
int | get_thumbnails_size (void) const |
void | remove_thumbnails (void) |
Static Public Member Functions | |
static GP< DjVuDocEditor > | create_wait (void) |
static GP< DjVuDocEditor > | create_wait (const GURL &url) |
Static Public Attributes | |
static int | thumbnails_per_file = 10 |
Protected Member Functions | |
DjVuDocEditor (void) | |
virtual GP< DataPool > | get_thumbnail (int page_num, bool dont_decode) |
void | init (const GURL &url) |
void | init (void) |
virtual GP< DjVuFile > | url_to_file (const GURL &url, bool dont_create) const |
Detailed Description
DjVuDocEditor# is an extension of {DjVuDocument} class with additional capabilities for editing the document contents.It can be used to: {enumerate} Create (compose) new multipage DjVu documents using single page DjVu documents. The class does { not} do compression. Insert and remove different pages of multipage DjVu documents. Change attributes ({names}, {IDs} and {titles}) of files composing the DjVu document. Generate thumbnail images and integrate them into the document. {enumerate}
Definition at line 104 of file DjVuDocEditor.h.
Constructor & Destructor Documentation
DjVuDocEditor::DjVuDocEditor | ( | void | ) | [protected] |
DjVuDocEditor::~DjVuDocEditor | ( | void | ) | [virtual] |
Member Function Documentation
bool DjVuDocEditor::can_be_saved | ( | void | ) | const |
Returns TRUE# if the document can be "saved" (sometimes the only possibility is to do a "save as").
The reason why we have this function is that DjVuDocEditor# can save documents in new formats only (BUNDLED# and INDIRECT#). At the same time it recognizes all DjVu formats (OLD_BUNDLED#, OLD_INDEXED#, BUNDLED#, and INDIRECT#).
OLD_BUNDLED# and BUNDLED# documents occupy only one file, so in this case "saving" involves the automatic conversion to BUNDLED# format and storing data into the same file.
OLD_INDEXED# documents, on the other hand, occupy more than one file. They could be converted to INDIRECT# format if these two formats had the same set of files. Unfortunately, these formats are too different, and the best thing to do is to use "save as" capability.
Definition at line 2158 of file DjVuDocEditor.cpp.
void DjVuDocEditor::create_shared_anno_file | ( | void(*)(float progress, void *) | progress_cb = 0 , |
|
void * | cl_data = 0 | |||
) |
Will create a file that will be included into every page and marked with the SHARED_ANNO# flag.
This file can be used to store global annotations (annotations applicable to every page).
{ Note:} There may be only one SHARED_ANNO# file in any DjVu multipage document.
Definition at line 1425 of file DjVuDocEditor.cpp.
GP< DjVuDocEditor > DjVuDocEditor::create_wait | ( | void | ) | [static] |
Creates a DjVuDocEditor class and initializes an empty document.
Definition at line 2128 of file DjVuDocEditor.cpp.
GP< DjVuDocEditor > DjVuDocEditor::create_wait | ( | const GURL & | url | ) | [static] |
Creates a DjVuDocEditor class and initializes with fname#.
Definition at line 2137 of file DjVuDocEditor.cpp.
void DjVuDocEditor::generate_thumbnails | ( | int | thumb_size, | |
bool(*)(int page_num, void *) | cb = 0 , |
|||
void * | cl_data = 0 | |||
) |
Generates thumbnails for those pages, which do not have them yet.
If you want to regenerate thumbnails for all pages, call {remove_thumbnails}() prior to calling this function.
- Parameters:
-
thumb_size The size of the thumbnails in pixels. DjVu viewer is able to rescale the thumbnail images if necessary, so this parameter affects thumbnails quality only. 128 is a good number. cb The callback, which will be called after thumbnail image for the next page has been generated. Regardless of if the document already has thumbnail images for some of its pages, the callback will be called pages_num# times, where pages_num# is the total number of pages in the document. The callback should return FALSE# if thumbnails generating should proceed. TRUE# will stop it.
Definition at line 1733 of file DjVuDocEditor.cpp.
int DjVuDocEditor::generate_thumbnails | ( | int | thumb_size, | |
int | page_num | |||
) |
Generates thumbnails for the specified page, if and only if it does not have a thumbnail yet.
If you want to regenerate thumbnails for all pages, call {remove_thumbnails}() prior to calling this function.
- Parameters:
-
thumb_size The size of the thumbnails in pixels. DjVu viewer is able to rescale the thumbnail images if necessary, so this parameter affects thumbnails quality only. 128 is a good number. page_num The page number to genate the thumbnail for.
Definition at line 1690 of file DjVuDocEditor.cpp.
GURL DjVuDocEditor::get_doc_url | ( | void | ) | const |
Definition at line 2181 of file DjVuDocEditor.cpp.
int DjVuDocEditor::get_orig_doc_type | ( | void | ) | const |
Returns type of open document.
DjVuDocEditor# silently converts any open DjVu document to BUNDLED# format (see {DjVuDocument}. Thus, {DjVuDocument::get_doc_type}() will always be returning BUNDLED#. Use this function to learn the original format of the document being edited.
Definition at line 2152 of file DjVuDocEditor.cpp.
int DjVuDocEditor::get_save_doc_type | ( | void | ) | const |
Returns type of the document, which can be created by {save}() function.
Can be INDIRECT#, BUNDLED#, SINGLE_PAGE#, or UNKNOWN_TYPE#. The latter indicates, that {save}() will fail, and that {save_as}() should be used instead
Definition at line 2165 of file DjVuDocEditor.cpp.
Returns a pointer to the file with SHARED_ANNO# flag on.
This file should be used for storing document-wide annotations.
{ Note:} There may be only one SHARED_ANNO# file in any DjVu multipage document.
Definition at line 1481 of file DjVuDocEditor.cpp.
Returns a {DataPool} containing one chunk TH44# with the encoded thumbnail for the specified page.
The function first looks for thumbnails enclosed into the document and if it fails to find one, it decodes the required page and creates the thumbnail on the fly (unless dont_decode# is true).
{ Note:} It may happen that the returned {DataPool} will not contain all the data you need. In this case you will need to install a trigger into the {DataPool} to learn when the data actually arrives.
Reimplemented from DjVuDocument.
Definition at line 1493 of file DjVuDocEditor.cpp.
int DjVuDocEditor::get_thumbnails_num | ( | void | ) | const |
Returns the number of thumbnails stored inside this document.
It may be ZERO#, which means, that there are no thumbnails at all.
It may be equal to the number of pages, which is what should normally be.
Finally, it may be greater than ZERO# and less than the number of pages, in which case thumbnails should be regenerated before the document can be saved.
Definition at line 1519 of file DjVuDocEditor.cpp.
int DjVuDocEditor::get_thumbnails_size | ( | void | ) | const |
Returns the size of the first encountered thumbnail image.
Since thumbnails can currently be generated by {generate_thumbnails}() only, all thumbnail images should be of the same size. Thus, the number returned is actually the size of {all} document thumbnails.
The function will return #-1# if there are no thumbnails.
Definition at line 1534 of file DjVuDocEditor.cpp.
bool DjVuDocEditor::inherits | ( | const GUTF8String & | class_name | ) | const [virtual] |
Returns TRUE if class_name# is #"DjVuDocEditor"#, #"DjVuDocument"# or #"DjVuPort"#.
Reimplemented from DjVuDocument.
Definition at line 2146 of file DjVuDocEditor.cpp.
void DjVuDocEditor::init | ( | const GURL & | url | ) | [protected] |
Initialization function.
Opens document with name filename#.
{ Note}: You must call either of the two available {init}() function before you start doing anything else with the DjVuDocEditor#.
Definition at line 171 of file DjVuDocEditor.cpp.
void DjVuDocEditor::init | ( | void | ) | [protected] |
Initialization function.
Initializes an empty document.
{ Note}: You must call either of the two available {init}() function before you start doing anything else with the DjVuDocEditor#.
Definition at line 146 of file DjVuDocEditor.cpp.
GUTF8String DjVuDocEditor::insert_file | ( | const GURL & | url, | |
const GUTF8String & | parent_id, | |||
int | chunk_num = 1 , |
|||
DjVuPort * | source = 0 | |||
) |
Definition at line 436 of file DjVuDocEditor.cpp.
void DjVuDocEditor::insert_group | ( | const GList< GURL > & | furl_list, | |
int | page_num = -1 , |
|||
void(*)(void *) | refresh_cb = 0 , |
|||
void * | cl_data = 0 | |||
) |
Inserts a group of pages into this DjVu document.
Like {insert_page}() it will insert every page into the document. The main advantage of calling this function once for the whole group instead of calling {insert_page}() for every page is the processing of included files:
The group of files may include one or more files, which are thus shared by them. If you call {insert_page}() for every page, this shared file will be inserted into the document more than once though under different names. This is how {insert_page}() works: whenever it inserts something, it checks for duplicate names with only one purpose: invent a new name if a given one is already in use.
On the other hand, if you call insert_group#(), it will insert shared included files only once. This is because it can analyze the group of files before inserting them and figure out what files are shared and thus should be inserted only once.
- Parameters:
-
fname_list List of top-level files for the pages to be inserted page_num Position where the new pages should be inserted at. Negative value means "append"
Definition at line 777 of file DjVuDocEditor.cpp.
void DjVuDocEditor::insert_page | ( | GP< DataPool > & | file_pool, | |
const GURL & | fname, | |||
int | page_num = -1 | |||
) |
Inserts a new page with data inside the data_pool# as page number page_num.
- Parameters:
-
data_pool {DataPool} with data for this page. file_name Name, which will be assigned to this page. If you try to save the document in INDIRECT# format, a file with this name will be created to hold the page's data. If there is already a file in the document with the same name, the function will derive a new unique name from file_name, which will be assigned to the page. page_num Describes where the page should be inserted. Negative number means "append".
Definition at line 895 of file DjVuDocEditor.cpp.
void DjVuDocEditor::insert_page | ( | const GURL & | fname, | |
int | page_num = -1 | |||
) |
Inserts the referenced file into this DjVu document.
- Parameters:
-
fname Name of the top-level file containing the image of the page to be inserted. This file must be a DjVu file and may include one or more other DjVu files.
When inserting a file, the function may modify its name to be unique in the DjVu document.
- Parameters:
-
page_num Position where the new page should be inserted at. Negative value means "append"
Definition at line 883 of file DjVuDocEditor.cpp.
void DjVuDocEditor::move_page | ( | int | page_num, | |
int | new_page_num | |||
) |
Makes page number page_num# to be new_page_num#.
If new_page_num# is negative or too big, the function will move page page_num# to the end of the document.
Definition at line 1161 of file DjVuDocEditor.cpp.
void DjVuDocEditor::move_pages | ( | const GList< int > & | page_list, | |
int | shift | |||
) |
Shifts all pags from the page_list# according to the shift#.
The shift# can be positive (shift toward the end of the document) or negative (shift toward the beginning of the document).
It is OK to make shift# too big in value. Pages will just be moved to the end (or to the beginning, depending on the shift# sign) of the document.
Definition at line 1220 of file DjVuDocEditor.cpp.
GUTF8String DjVuDocEditor::page_to_id | ( | int | page_num | ) | const |
Translates page number page_num# to ID.
If page_num# is invalid, an exception is thrown.
Reimplemented from DjVuDocument.
Definition at line 351 of file DjVuDocEditor.cpp.
void DjVuDocEditor::remove_file | ( | const GUTF8String & | id, | |
bool | remove_unref = true | |||
) |
Removes a DjVu file with the specified id#.
If some other files include this file, the corresponding INCL# chunks will be removed to avoid dead links.
If remove_unref# is TRUE#, the function will also remove every file, which will become unreferenced after the removal of this file.
Definition at line 1036 of file DjVuDocEditor.cpp.
void DjVuDocEditor::remove_page | ( | int | page_num, | |
bool | remove_unref = true | |||
) |
Removes the specified page from the document.
If remove_unref# is TRUE#, the function will also remove from the document any file, which became unreferenced due to the page's removal
Definition at line 1068 of file DjVuDocEditor.cpp.
void DjVuDocEditor::remove_pages | ( | const GList< int > & | page_list, | |
bool | remove_unref = true | |||
) |
Removes the specified pages from the document.
If remove_unref# is TRUE#, the function will also remove from the document any file, which became unreferenced due to the pages' removal
Definition at line 1083 of file DjVuDocEditor.cpp.
void DjVuDocEditor::remove_thumbnails | ( | void | ) |
This request is issued when decoder needs additional data for decoding.
Both {DjVuFile} and {DjVuDocument} are initialized with a URL, not the document data. As soon as they need the data, they call this function, whose responsibility is to locate the source of the data basing on the URL# passed and return it back in the form of the {DataPool}. If this particular receiver is unable to fullfil the request, it should return #0#.
Reimplemented from DjVuDocument.
Definition at line 230 of file DjVuDocEditor.cpp.
void DjVuDocEditor::save | ( | void | ) |
Saves the document.
May generate exception if the document can not be saved, and {save_as}() should be used. See {can_be_saved}() for details.
Definition at line 1861 of file DjVuDocEditor.cpp.
void DjVuDocEditor::save_as | ( | const GURL & | where, | |
bool | bundled | |||
) | [virtual] |
Saves the document.
Reimplemented from DjVuDocument.
Definition at line 1905 of file DjVuDocEditor.cpp.
void DjVuDocEditor::save_pages_as | ( | const GP< ByteStream > & | str, | |
const GList< int > & | page_list | |||
) |
Saves the specified pages in DjVu BUNDLED# multipage document.
Definition at line 1771 of file DjVuDocEditor.cpp.
void DjVuDocEditor::set_file_name | ( | const GUTF8String & | id, | |
const GUTF8String & | name | |||
) |
Changes the name of the file with ID id# to name#.
Refer to {DjVmDir} for the explanation of {IDs}, {names} and {titles}.
Definition at line 1280 of file DjVuDocEditor.cpp.
void DjVuDocEditor::set_file_title | ( | const GUTF8String & | id, | |
const GUTF8String & | title | |||
) |
Changes the title of the file with ID id# to title#.
Refer to {DjVmDir} for the explanation of {IDs}, {names} and {titles}.
Definition at line 1317 of file DjVuDocEditor.cpp.
void DjVuDocEditor::set_page_name | ( | int | page_num, | |
const GUTF8String & | name | |||
) |
Changes the name of the page page_num# to name#.
Refer to {DjVmDir} for the explanation of {IDs}, {names} and {titles}.
Definition at line 1305 of file DjVuDocEditor.cpp.
void DjVuDocEditor::set_page_title | ( | int | page_num, | |
const GUTF8String & | title | |||
) |
Changes the title of the page page_num# to title#.
Refer to {DjVmDir} for the explanation of {IDs}, {names} and {titles}.
Definition at line 1327 of file DjVuDocEditor.cpp.
void DjVuDocEditor::simplify_anno | ( | void(*)(float progress, void *) | progress_cb = 0 , |
|
void * | cl_data = 0 | |||
) |
Use this function to simplify annotations in the document.
The "simplified" format is when annotations are only allowed either in top-level page files or in a special file with SHARED_ANNO# flag on. This file is supposed to be included into every page.
Definition at line 1343 of file DjVuDocEditor.cpp.
void DjVuDocEditor::write | ( | const GP< ByteStream > & | str, | |
const GMap< GUTF8String, void * > & | reserved | |||
) | [virtual] |
Always save as bundled, renaming any files conflicting with the the names in the supplied GMap.
Reimplemented from DjVuDocument.
Definition at line 1888 of file DjVuDocEditor.cpp.
void DjVuDocEditor::write | ( | const GP< ByteStream > & | str, | |
bool | force_djvm = false | |||
) | [virtual] |
Saves the document in the {new bundled} format.
All the data is "bundled" into one file and this file is written into the passed stream.
If force_djvm# is TRUE# then even one page documents will be saved in the DJVM BUNDLED# format (inside a FORM:DJVM#);
{ Plugin Warning}. This function will read contents of the whole document. Thus, if you call it from the main thread (the thread, which transfers data from Netscape), the plugin will block.
Reimplemented from DjVuDocument.
Definition at line 1872 of file DjVuDocEditor.cpp.
Member Data Documentation
int DjVuDocEditor::thumbnails_per_file = 10 [static] |
Definition at line 107 of file DjVuDocEditor.h.
The documentation for this class was generated from the following files: