Konsole
#include <ViewManager.h>
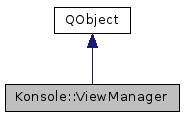
Public Types | |
enum | NavigationMethod { TabbedNavigation, NoNavigation } |
enum | NewTabBehavior { PutNewTabAtTheEnd = 0, PutNewTabAfterCurrentTab = 1 } |
Public Slots | |
Q_SCRIPTABLE int | currentSession () |
Q_SCRIPTABLE QString | defaultProfile () |
Q_SCRIPTABLE void | moveSessionLeft () |
Q_SCRIPTABLE void | moveSessionRight () |
Q_SCRIPTABLE int | newSession (QString profile, QString directory) |
Q_SCRIPTABLE int | newSession () |
Q_SCRIPTABLE void | nextSession () |
Q_SCRIPTABLE void | prevSession () |
Q_SCRIPTABLE QStringList | profileList () |
Q_SCRIPTABLE int | sessionCount () |
Q_SCRIPTABLE void | setTabWidthToText (bool) |
Signals | |
void | activeViewChanged (SessionController *controller) |
void | empty () |
void | newViewRequest () |
void | newViewRequest (Profile::Ptr) |
void | setMenuBarVisibleRequest (bool) |
void | setSaveGeometryOnExitRequest (bool) |
void | splitViewToggle (bool multipleViews) |
void | unplugController (SessionController *controller) |
void | updateWindowIcon () |
void | viewDetached (Session *session) |
void | viewPropertiesChanged (const QList< ViewProperties * > &propertiesList) |
Public Member Functions | |
ViewManager (QObject *parent, KActionCollection *collection) | |
~ViewManager () | |
QWidget * | activeView () const |
SessionController * | activeViewController () const |
void | applyProfileToView (TerminalDisplay *view, const Profile::Ptr profile) |
void | createView (Session *session) |
int | managerId () const |
NavigationMethod | navigationMethod () const |
void | restoreSessions (const KConfigGroup &group) |
void | saveSessions (KConfigGroup &group) |
IncrementalSearchBar * | searchBar () const |
QList< Session * > | sessions () |
void | setNavigationBehavior (int behavior) |
void | setNavigationMethod (NavigationMethod method) |
void | setNavigationPosition (int position) |
void | setNavigationStyleSheet (const QString &styleSheet) |
void | setNavigationVisibility (int visibility) |
void | setShowQuickButtons (bool show) |
QList< ViewProperties * > | viewProperties () const |
QWidget * | widget () const |
Detailed Description
Manages the terminal display widgets in a Konsole window or part.
When a view manager is created, it constructs a splitter widget ( accessed via widget() ) to hold one or more view containers. Each view container holds one or more terminal displays and a navigation widget ( eg. tabs or a list ) to allow the user to navigate between the displays in that container.
The view manager provides menu actions ( defined in the 'konsoleui.rc' XML file ) to manipulate the views and view containers - for example, actions to split the view left/right or top/bottom, detach a view from the current window and navigate between views and containers. These actions are added to the collection specified in the ViewManager's constructor.
The view manager provides facilities to construct display widgets for a terminal session and also to construct the SessionController which provides the menus and other user interface elements specific to each display/session pair.
Definition at line 66 of file ViewManager.h.
Member Enumeration Documentation
This enum describes the available types of navigation widget which newly created containers can provide to allow navigation between open sessions.
Enumerator | |
---|---|
TabbedNavigation |
Each container has a row of tabs (one per session) which the user can click on to navigate between open sessions. |
NoNavigation |
The container has no navigation widget. |
Definition at line 117 of file ViewManager.h.
This enum describes where newly created tab should be placed.
Enumerator | |
---|---|
PutNewTabAtTheEnd |
Put newly created tab at the end. |
PutNewTabAfterCurrentTab |
Put newly created tab right after current tab. |
Definition at line 130 of file ViewManager.h.
Constructor & Destructor Documentation
ViewManager::ViewManager | ( | QObject * | parent, |
KActionCollection * | collection | ||
) |
Constructs a new view manager with the specified parent
.
View-related actions defined in 'konsoleui.rc' are created and added to the specified collection
.
Definition at line 57 of file ViewManager.cpp.
ViewManager::~ViewManager | ( | ) |
Definition at line 109 of file ViewManager.cpp.
Member Function Documentation
QWidget * ViewManager::activeView | ( | ) | const |
Returns the view manager's active view.
Definition at line 118 of file ViewManager.cpp.
|
signal |
Emitted when the active view changes.
- Parameters
-
controller The controller associated with the active view
SessionController * ViewManager::activeViewController | ( | ) | const |
Returns the controller for the active view.
activeViewChanged() is emitted when this changes.
Definition at line 533 of file ViewManager.cpp.
void ViewManager::applyProfileToView | ( | TerminalDisplay * | view, |
const Profile::Ptr | profile | ||
) |
Applies the view-specific settings associated with specified profile
to the terminal display view
.
Definition at line 789 of file ViewManager.cpp.
void ViewManager::createView | ( | Session * | session | ) |
Creates a new view to display the output from and deliver input to session
.
Constructs a new container to hold the views if no container has yet been created.
Definition at line 577 of file ViewManager.cpp.
|
slot |
DBus slot that returns the current (active) session window.
Definition at line 999 of file ViewManager.cpp.
|
slot |
Definition at line 1040 of file ViewManager.cpp.
|
signal |
Emitted when the last view is removed from the view manager.
int ViewManager::managerId | ( | ) | const |
Definition at line 113 of file ViewManager.cpp.
|
slot |
DBus slot that switches the current session (as returned by currentSession()) with the left (or previous) one in the navigation tab.
Definition at line 1060 of file ViewManager.cpp.
|
slot |
DBus slot that Switches the current session (as returned by currentSession()) with the right (or next) one in the navigation tab.
Definition at line 1065 of file ViewManager.cpp.
ViewManager::NavigationMethod ViewManager::navigationMethod | ( | ) | const |
Returns the type of navigation widget created in new containers.
Definition at line 730 of file ViewManager.cpp.
|
slot |
DBus slot that creates a new session in the current view.
- Parameters
-
profile the name of the profile to be used directory the working directory where the session is started.
Definition at line 1019 of file ViewManager.cpp.
|
slot |
DBus slot that creates a new session in the current view with the associated default profile and the default working directory.
Definition at line 1008 of file ViewManager.cpp.
|
signal |
Requests creation of a new view with the default profile.
|
signal |
Requests creation of a new view, with the selected profile.
|
slot |
DBus slot that changes the view port to the next session.
Definition at line 1050 of file ViewManager.cpp.
|
slot |
DBus slot that changes the view port to the previous session.
Definition at line 1055 of file ViewManager.cpp.
|
slot |
Definition at line 1045 of file ViewManager.cpp.
void ViewManager::restoreSessions | ( | const KConfigGroup & | group | ) |
Definition at line 959 of file ViewManager.cpp.
void ViewManager::saveSessions | ( | KConfigGroup & | group | ) |
Session management.
Definition at line 924 of file ViewManager.cpp.
IncrementalSearchBar * ViewManager::searchBar | ( | ) | const |
Returns the search bar.
Definition at line 538 of file ViewManager.cpp.
|
slot |
DBus slot that returns the number of sessions in the current view.
Definition at line 994 of file ViewManager.cpp.
Returns a list of sessions in this ViewManager.
Definition at line 178 of file ViewManager.h.
|
signal |
Emitted when menu bar visibility changes because a profile that requires so is activated.
void ViewManager::setNavigationBehavior | ( | int | behavior | ) |
Definition at line 1133 of file ViewManager.cpp.
void ViewManager::setNavigationMethod | ( | NavigationMethod | method | ) |
Sets the type of widget provided to navigate between open sessions in a container.
The changes will only apply to newly created containers.
The default method is TabbedNavigation. To disable navigation widgets, call setNavigationMethod(ViewManager::NoNavigation) before creating any sessions.
Definition at line 685 of file ViewManager.cpp.
void ViewManager::setNavigationPosition | ( | int | position | ) |
Definition at line 1095 of file ViewManager.cpp.
void ViewManager::setNavigationStyleSheet | ( | const QString & | styleSheet | ) |
Definition at line 1106 of file ViewManager.cpp.
void ViewManager::setNavigationVisibility | ( | int | visibility | ) |
Definition at line 1085 of file ViewManager.cpp.
|
signal |
void ViewManager::setShowQuickButtons | ( | bool | show | ) |
Definition at line 1115 of file ViewManager.cpp.
|
slot |
DBus slot that sets ALL tabs' width to match their text.
Definition at line 1070 of file ViewManager.cpp.
|
signal |
Emitted when the number of views containers changes.
This is used to disable or enable menu items which can only be used when there are one or multiple containers visible.
- Parameters
-
multipleViews True if there are multiple view containers open or false if there is just a single view.
|
signal |
Emitted when the current session needs unplugged from factory().
- Parameters
-
controller The controller associated with the active view
|
signal |
|
signal |
Emitted when a session is detached from a view owned by this ViewManager.
QList< ViewProperties * > ViewManager::viewProperties | ( | ) | const |
Returns the list of view properties for views in the active container.
Each view widget is associated with a ViewProperties instance which provides access to basic information about the session being displayed in the view, such as title, current directory and associated icon.
Definition at line 907 of file ViewManager.cpp.
|
signal |
Emitted when the list of view properties ( as returned by viewProperties() ) changes.
This occurs when views are added to or removed from the active container, or if the active container is changed.
QWidget * ViewManager::widget | ( | ) | const |
Return the main widget for the view manager which holds all of the views managed by this ViewManager instance.
Definition at line 128 of file ViewManager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:25 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.