kstars
#include <deepskyobject.h>
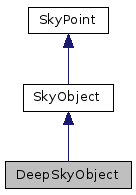
Public Types | |
enum | CATALOG { CAT_MESSIER =0, CAT_NGC =1, CAT_IC =2, CAT_UNKNOWN } |
![]() | |
enum | TYPE { STAR =0, CATALOG_STAR =1, PLANET =2, OPEN_CLUSTER =3, GLOBULAR_CLUSTER =4, GASEOUS_NEBULA =5, PLANETARY_NEBULA =6, SUPERNOVA_REMNANT =7, GALAXY =8, COMET =9, ASTEROID =10, CONSTELLATION =11, MOON =12, ASTERISM =13, GALAXY_CLUSTER =14, DARK_NEBULA =15, QUASAR =16, MULT_STAR =17, RADIO_SOURCE =18, SATELLITE =19, SUPERNOVA =20, TYPE_UNKNOWN } |
typedef qint64 | UID |
Public Member Functions | |
DeepSkyObject (int t=SkyObject::STAR, dms r=dms(0.0), dms d=dms(0.0), float m=0.0, const QString &n="unnamed", const QString &n2=QString(), const QString &lname=QString(), const QString &cat=QString(), float a=0.0, float b=0.0, double pa=0.0, int pgc=0, int ugc=0) | |
DeepSkyObject (const DeepSkyObject &o) | |
virtual | ~DeepSkyObject () |
float | a () const |
float | b () const |
QString | catalog (void) const |
virtual DeepSkyObject * | clone () const |
CatalogComponent * | customCatalog () |
float | e () const |
float | flux () const |
virtual SkyObject::UID | getUID () const |
const QImage & | image () const |
bool | isCatalogIC () const |
bool | isCatalogM () const |
bool | isCatalogNGC () const |
bool | isCatalogNone () const |
virtual double | labelOffset () const |
QString | labelString () const |
void | loadImage () |
virtual double | pa () const |
int | pgc () const |
void | setCatalog (const QString &s) |
void | setCustomCatalog (CatalogComponent *s) |
void | setFlux (const float &f) |
int | ugc () const |
![]() | |
SkyObject (int t=TYPE_UNKNOWN, dms r=dms(0.0), dms d=dms(0.0), float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
SkyObject (int t, double r, double d, float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
virtual | ~SkyObject () |
bool | hasAuxInfo () |
bool | hasLongName () const |
bool | hasName () const |
bool | hasName2 () const |
QStringList & | ImageList () |
QStringList & | ImageTitle () |
QStringList & | InfoList () |
QStringList & | InfoTitle () |
bool | isSolarSystem () const |
virtual QString | longname (void) const |
float | mag (void) const |
float | mag (const QString &band) const |
QString | messageFromTitle (const QString &imageTitle) |
virtual QString | name (void) const |
QString | name2 (void) const |
QString & | notes () |
SkyPoint | recomputeCoords (const KStarsDateTime &dt, const GeoLocation *geo=0) |
QTime | riseSetTime (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) |
dms | riseSetTimeAz (const KStarsDateTime &dt, const GeoLocation *geo, bool rst) |
QTime | riseSetTimeUT (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) |
void | saveUserLog (const QString &newLog) |
void | setLongName (const QString &longname=QString()) |
void | setNotes (QString _notes) |
void | setType (int t) |
void | showPopupMenu (KSPopupMenu *pmenu, const QPoint &pos) |
dms | transitAltitude (const KStarsDateTime &dt, const GeoLocation *geo) |
QTime | transitTime (const KStarsDateTime &dt, const GeoLocation *geo) |
QTime | transitTimeUT (const KStarsDateTime &dt, const GeoLocation *geo) |
QString | translatedLongName () const |
QString | translatedName () const |
QString | translatedName2 () const |
int | type (void) const |
QString | typeName () const |
QString & | userLog () |
![]() | |
SkyPoint (const dms &r, const dms &d) | |
SkyPoint (double r, double d) | |
SkyPoint () | |
virtual | ~SkyPoint () |
void | aberrate (const KSNumbers *num) |
void | addEterms (void) |
const dms & | alt () const |
dms | altRefracted () const |
dms | angularDistanceTo (const SkyPoint *sp, double *const positionAngle=0) const |
void | apparentCoord (long double jd0, long double jdf) |
const dms & | az () const |
void | B1950ToJ2000 (void) |
bool | bendlight () |
bool | checkBendLight () |
bool | checkCircumpolar (const dms *gLat) |
const dms & | dec () const |
const dms & | dec0 () const |
SkyPoint | deprecess (const KSNumbers *num, long double epoch=J2000) |
void | Equatorial1950ToGalactic (dms &galLong, dms &galLat) |
void | EquatorialToHorizontal (const dms *LST, const dms *lat) |
SkyPoint | Eterms (void) |
void | findEcliptic (const dms *Obliquity, dms &EcLong, dms &EcLat) |
void | GalacticToEquatorial1950 (const dms *galLong, const dms *galLat) |
void | HorizontalToEquatorial (const dms *LST, const dms *lat) |
void | J2000ToB1950 (void) |
SkyPoint | moveAway (const SkyPoint &from, double dist) |
void | nutate (const KSNumbers *num) |
bool | operator== (SkyPoint &p) |
void | precessFromAnyEpoch (long double jd0, long double jdf) |
const dms & | ra () const |
const dms & | ra0 () const |
void | set (const dms &r, const dms &d) |
void | setAlt (dms alt) |
void | setAlt (double alt) |
void | setAz (dms az) |
void | setAz (double az) |
void | setDec (dms d) |
void | setDec (double d) |
void | setDec0 (dms d) |
void | setDec0 (double d) |
void | setFromEcliptic (const dms *Obliquity, const dms &EcLong, const dms &EcLat) |
void | setRA (dms r) |
void | setRA (double r) |
void | setRA0 (dms r) |
void | setRA0 (double r) |
void | subtractEterms (void) |
virtual void | updateCoords (KSNumbers *num, bool includePlanets=true, const dms *lat=0, const dms *LST=0, bool forceRecompute=false) |
double | vGeocentric (double vhelio, long double jd) |
double | vGeoToVHelio (double vgeo, long double jd) |
double | vHeliocentric (double vlsr, long double jd) |
double | vHelioToVlsr (double vhelio, long double jd) |
double | vREarth (long double jd0) |
double | vRSite (double vsite[3]) |
double | vRSun (long double jd) |
double | vTopocentric (double vgeo, double vsite[3]) |
double | vTopoToVGeo (double vtopo, double vsite[3]) |
Public Attributes | |
quint64 | updateID |
quint64 | updateNumID |
Additional Inherited Members | |
![]() | |
static QString | typeName (const int t) |
![]() | |
static double | refract (const double alt) |
static dms | refract (const dms alt) |
static double | refractionCorr (double alt) |
static double | unrefract (const double alt) |
static dms | unrefract (const dms alt) |
![]() | |
static const UID | invalidUID = ~0 |
static const UID | UID_DEEPSKY = 2 |
static const UID | UID_GALAXY = 1 |
static const UID | UID_SOLARSYS = 3 |
static const UID | UID_STAR = 0 |
![]() | |
static const double | altCrit = -1.0 |
![]() | |
void | setMag (float m) |
void | setMag (const QString &band, const double mag) |
void | setName (const QString &name) |
void | setName2 (const QString &name2=QString()) |
![]() | |
void | precess (const KSNumbers *num) |
![]() | |
QSharedDataPointer< AuxInfo > | info |
QString | LongName |
QString | Name |
QString | Name2 |
![]() | |
long double | lastPrecessJD |
![]() | |
static QString | emptyString |
static QString | starString = QString("star") |
static QString | unnamedObjectString = QString(I18N_NOOP("unnamed object")) |
static QString | unnamedString = QString(I18N_NOOP("unnamed")) |
Detailed Description
Provides all necessary information about a deep-sky object: data inherited from SkyObject (coordinates, type, magnitude, 2 names, and URLs) and data specific to DeepSkyObjects (common name, angular size, position angle, Image, catalog)
Information about a "dep-sky" object; i.e., anything that's not a solar system body or a star.
- Version
- 1.0
Definition at line 43 of file deepskyobject.h.
Member Enumeration Documentation
The catalog ID of the DeepSkyObject.
Enumerator | |
---|---|
CAT_MESSIER | |
CAT_NGC | |
CAT_IC | |
CAT_UNKNOWN |
Definition at line 85 of file deepskyobject.h.
Constructor & Destructor Documentation
|
explicit |
Constructor.
Create DeepSkyObject with data according to arguments.
- Parameters
-
t Type of object r catalog Right Ascension d catalog Declination m magnitude (brightness) n Primary name n2 Secondary name lname Long name (common name) cat catalog ID a major axis (arcminutes) b minor axis (arcminutes) pa position angle (degrees) pgc PGC catalog number ugc UGC catalog number
Definition at line 54 of file deepskyobject.cpp.
DeepSkyObject::DeepSkyObject | ( | const DeepSkyObject & | o | ) |
Copy constructor.
- Parameters
-
o SkyObject from which to copy data
Definition at line 39 of file deepskyobject.cpp.
|
inlinevirtual |
*Destructor
Definition at line 79 of file deepskyobject.h.
Member Function Documentation
|
inline |
- Returns
- the object's major axis length, in arcminutes.
Definition at line 132 of file deepskyobject.h.
|
inline |
- Returns
- the object's minor axis length, in arcminutes.
Definition at line 137 of file deepskyobject.h.
QString DeepSkyObject::catalog | ( | void | ) | const |
- Returns
- a QString identifying the object's primary catalog. Possible catalog values are:
- "M" for Messier catalog
- "NGC" for NGC catalog
- "IC" for IC catalog
- empty string is presumed to be an object in a custom catalog
- See also
- setCatalog()
Definition at line 87 of file deepskyobject.cpp.
|
virtual |
Create copy of object.
This method is virtual copy constructor. It allows for safe copying of objects. In other words, KSPlanet object stored in SkyObject pointer will be copied as KSPlanet. Each subclass of SkyObject MUST implement clone method.
- Returns
- pointer to newly allocated object. Caller takes full responsibility for deallocating it.
Reimplemented from SkyObject.
Definition at line 72 of file deepskyobject.cpp.
|
inline |
- Returns
- a pointer to a custom catalog component
Definition at line 117 of file deepskyobject.h.
float DeepSkyObject::e | ( | ) | const |
- Returns
- the object's aspect ratio (MinorAxis/MajorAxis). Returns 1.0 if the object's MinorAxis=0.0.
Definition at line 81 of file deepskyobject.cpp.
|
inline |
- Returns
- the object's integrated flux, unit value is stored in the custom catalog component.
Definition at line 127 of file deepskyobject.h.
|
virtual |
Return UID for object.
This method should be reimplemented in all concrete subclasses. Implementation for SkyObject just returns invalidUID. It's required SkyObject is not an abstract class.
Reimplemented from SkyObject.
Definition at line 138 of file deepskyobject.cpp.
|
inline |
- Returns
- an object's image
Definition at line 161 of file deepskyobject.h.
|
inline |
- Returns
- true if the object is in the IC catalog
Definition at line 179 of file deepskyobject.h.
|
inline |
- Returns
- true if the object is in the Messier catalog
Definition at line 169 of file deepskyobject.h.
|
inline |
- Returns
- true if the object is in the NGC catalog
Definition at line 174 of file deepskyobject.h.
|
inline |
- Returns
- true if the object is not in a catalog
Definition at line 184 of file deepskyobject.h.
|
virtual |
- Returns
- the pixel distance for offseting the object's name label
Reimplemented from SkyObject.
Definition at line 107 of file deepskyobject.cpp.
|
virtual |
- Returns
- the string used to label the object on the map In the default implementation, this just returns translatedName() Overridden by StarObject.
Reimplemented from SkyObject.
Definition at line 119 of file deepskyobject.cpp.
void DeepSkyObject::loadImage | ( | ) |
Try to load the object's image.
Definition at line 101 of file deepskyobject.cpp.
|
inlinevirtual |
- Returns
- the object's position angle in degrees, measured clockwise from North.
Reimplemented from SkyObject.
Definition at line 148 of file deepskyobject.h.
|
inline |
- Returns
- the object's PGC catalog number. Return 0 if the object is not in PGC.
Definition at line 158 of file deepskyobject.h.
void DeepSkyObject::setCatalog | ( | const QString & | s | ) |
Set the internal Catalog value according to the QString argument: "M" : CAT_MESSIER "NGC" : CAT_NGC "IC" : CAT_IC.
- See also
- catalog()
Definition at line 94 of file deepskyobject.cpp.
|
inline |
Set the reference to the custom catalog component, if any.
- See also
- customCatalog()
Definition at line 112 of file deepskyobject.h.
|
inline |
Set the integrated flux value of the object.
Definition at line 122 of file deepskyobject.h.
|
inline |
- Returns
- the object's UGC catalog number. Return 0 if the object is not in UGC.
Definition at line 153 of file deepskyobject.h.
Member Data Documentation
quint64 DeepSkyObject::updateID |
Definition at line 191 of file deepskyobject.h.
quint64 DeepSkyObject::updateNumID |
Definition at line 192 of file deepskyobject.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:36:22 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.