kstars
#include <kstarsdatetime.h>
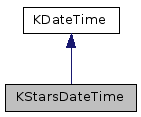
Public Member Functions | |
KStarsDateTime () | |
KStarsDateTime (long double djd) | |
KStarsDateTime (const KStarsDateTime &kdt) | |
KStarsDateTime (const KDateTime &kdt) | |
KStarsDateTime (const QDateTime &qdt) | |
KStarsDateTime (const QDate &_d, const QTime &_t) | |
KStarsDateTime | addDays (int nd) const |
KStarsDateTime | addSecs (double s) const |
long double | djd () const |
double | epoch () const |
dms | gst () const |
QTime | GSTtoUT (dms GST) const |
bool | operator!= (const KStarsDateTime &d) const |
bool | operator< (const KStarsDateTime &d) const |
bool | operator<= (const KStarsDateTime &d) const |
bool | operator== (const KStarsDateTime &d) const |
bool | operator> (const KStarsDateTime &d) const |
bool | operator>= (const KStarsDateTime &d) const |
void | setDate (const QDate &d) |
void | setDJD (long double jd) |
bool | setFromEpoch (double e) |
bool | setFromEpoch (const QString &e) |
void | setTime (const QTime &t) |
Static Public Member Functions | |
static KStarsDateTime | currentDateTime (KDateTime::Spec ts=KDateTime::Spec::ClockTime()) |
static KStarsDateTime | fromString (const QString &s) |
Detailed Description
Extension of KDateTime for KStars KStarsDateTime can represent the date/time as a Julian Day, using a long double, in which the fractional portion encodes the time of day to a precision of a less than a second.
Also adds Greenwich Sidereal Time and "epoch", which is just the date expressed as a floating point number representing the year, with the fractional part representing the date and time (with poor time resolution; typically the Epoch is only taken to the hundredths place, which is a few days).
- Note
- Local time and Local sideral time are not handled here. Because they depend on the geographic location, they are part of the GeoLocation class.
- Version
- 1.0
Definition at line 45 of file kstarsdatetime.h.
Constructor & Destructor Documentation
KStarsDateTime::KStarsDateTime | ( | ) |
Default constructor Creates a date/time at J2000 (noon on Jan 1, 200)
Definition at line 26 of file kstarsdatetime.cpp.
KStarsDateTime::KStarsDateTime | ( | long double | djd | ) |
Constructor Creates a date/time at the specified Julian Day.
jd
The Julian Day
Definition at line 63 of file kstarsdatetime.cpp.
KStarsDateTime::KStarsDateTime | ( | const KStarsDateTime & | kdt | ) |
Copy constructor kdt
The KStarsDateTime object to copy.
Definition at line 31 of file kstarsdatetime.cpp.
KStarsDateTime::KStarsDateTime | ( | const KDateTime & | kdt | ) |
Copy constructor kdt
The KDateTime object to copy.
Definition at line 36 of file kstarsdatetime.cpp.
KStarsDateTime::KStarsDateTime | ( | const QDateTime & | qdt | ) |
Copy constructor qdt
The QDateTime object to copy.
Definition at line 46 of file kstarsdatetime.cpp.
KStarsDateTime::KStarsDateTime | ( | const QDate & | _d, |
const QTime & | _t | ||
) |
Constructor Create a KStarsDateTimne based on the specified Date and Time.
_d
The QDate to assign _t
The QTime to assign
Definition at line 55 of file kstarsdatetime.cpp.
Member Function Documentation
|
inline |
Modify the Date/Time by adding a number of days.
nd
the number of days to add. The number can be negative.
Definition at line 117 of file kstarsdatetime.h.
KStarsDateTime KStarsDateTime::addSecs | ( | double | s | ) | const |
- Returns
- a KStarsDateTime that is the given number of seconds later than this KStarsDateTime.
s
the number of seconds to add. The number can be negative.
Definition at line 127 of file kstarsdatetime.cpp.
|
static |
- Returns
- the date and time according to the CPU clock
ts
a Qt::TimeSpec value that determines whether the date is computed from the Local Time or the Universal Time.
- See also
- Qt::TimeSpec
Definition at line 67 of file kstarsdatetime.cpp.
|
inline |
- Returns
- the julian day as a long double, including the time as the fractional portion.
Definition at line 145 of file kstarsdatetime.h.
|
inline |
- Returns
- the epoch value of the Date/Time.
- Note
- the epoch is shorthand for the date, expressed as a floating-point year value.
- See also
- setFromEpoch()
Definition at line 166 of file kstarsdatetime.h.
|
static |
- Returns
- a KStarsDateTime object parsed from the given string.
- Note
- This function is format-agnostic; it will try several formats when parsing the string.
- Parameters
-
s the string expressing the date/time to be parsed.
Definition at line 75 of file kstarsdatetime.cpp.
dms KStarsDateTime::gst | ( | ) | const |
- Returns
- The Greenwich Sidereal Time The Greenwich sidereal time is the Right Ascension coordinate that is currently transiting the Prime Meridian at the Royal Observatory in Greenwich, UK (longitude=0.0)
Definition at line 138 of file kstarsdatetime.cpp.
QTime KStarsDateTime::GSTtoUT | ( | dms | GST | ) | const |
Convert a given Greenwich Sidereal Time to Universal Time (=Greenwich Mean Time).
GST
the Greenwich Sidereal Time to convert to Universal Time.
Definition at line 176 of file kstarsdatetime.cpp.
|
inline |
Definition at line 120 of file kstarsdatetime.h.
|
inline |
Definition at line 121 of file kstarsdatetime.h.
|
inline |
Definition at line 122 of file kstarsdatetime.h.
|
inline |
Definition at line 119 of file kstarsdatetime.h.
|
inline |
Definition at line 123 of file kstarsdatetime.h.
|
inline |
Definition at line 124 of file kstarsdatetime.h.
void KStarsDateTime::setDate | ( | const QDate & | d | ) |
Assign the Date according to a QDate object.
d
the QDate to assign
Definition at line 119 of file kstarsdatetime.cpp.
void KStarsDateTime::setDJD | ( | long double | jd | ) |
Assign the (long double) Julian Day value, which includes the time of day encoded in the fractional portion.
jd
the Julian Day value to assign.
Definition at line 99 of file kstarsdatetime.cpp.
bool KStarsDateTime::setFromEpoch | ( | double | e | ) |
Set the Date/Time from an epoch value, represented as a double.
e
the epoch value
- Returns
- true if date set successfully
- See also
- epoch()
Definition at line 195 of file kstarsdatetime.cpp.
bool KStarsDateTime::setFromEpoch | ( | const QString & | e | ) |
Set the Date/Time from an epoch value, represented as a string.
e
the epoch value
- Returns
- true if date set successfully
- See also
- epoch()
Definition at line 216 of file kstarsdatetime.cpp.
void KStarsDateTime::setTime | ( | const QTime & | t | ) |
Assign the Time according to a QTime object.
t
the QTime to assign
Definition at line 133 of file kstarsdatetime.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:36:22 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.