kstars
#include <kstars.h>
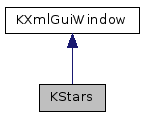
Public Slots | |
Q_SCRIPTABLE Q_NOREPLY void | addLabel (const QString &name) |
Q_SCRIPTABLE Q_NOREPLY void | addTrail (const QString &name) |
Q_SCRIPTABLE Q_NOREPLY void | changeViewOption (const QString &option, const QString &value) |
void | clearCachedFindDialog () |
Q_SCRIPTABLE Q_NOREPLY void | defaultZoom () |
Q_SCRIPTABLE Q_NOREPLY void | drawLine (int x1, int y1, int x2, int y2, int speed) |
Q_SCRIPTABLE Q_NOREPLY void | exportImage (const QString &filename, int width=-1, int height=-1, bool includeLegend=false) |
Q_SCRIPTABLE QString | getDSSURL (const QString &objectName) |
Q_SCRIPTABLE QString | getDSSURL (double RA_J2000, double Dec_J2000, float width=15, float height=15) |
Q_SCRIPTABLE QString | getObjectDataXML (const QString &objectName) |
Q_SCRIPTABLE QString | getObservingSessionPlanObjectNames () |
Q_SCRIPTABLE QString | getObservingWishListObjectNames () |
QString | getOption (const QString &name) |
Q_SCRIPTABLE QString | getSkyMapDimensions () |
Q_SCRIPTABLE Q_NOREPLY void | loadColorScheme (const QString &name) |
Q_SCRIPTABLE Q_NOREPLY void | lookTowards (const QString &direction) |
Q_SCRIPTABLE Q_NOREPLY void | popupMessage (int x, int y, const QString &message) |
Q_SCRIPTABLE Q_NOREPLY void | printImage (bool usePrintDialog, bool useChartColors) |
Q_SCRIPTABLE Q_NOREPLY void | readConfig () |
Q_SCRIPTABLE Q_NOREPLY void | removeLabel (const QString &name) |
Q_SCRIPTABLE Q_NOREPLY void | removeTrail (const QString &name) |
Q_SCRIPTABLE Q_NOREPLY void | setAltAz (double alt, double az) |
Q_SCRIPTABLE Q_NOREPLY void | setApproxFOV (double FOV_Degrees) |
Q_SCRIPTABLE Q_NOREPLY void | setColor (const QString &colorName, const QString &value) |
Q_SCRIPTABLE Q_NOREPLY void | setGeoLocation (const QString &city, const QString &province, const QString &country) |
Q_SCRIPTABLE Q_NOREPLY void | setLocalTime (int yr, int mth, int day, int hr, int min, int sec) |
Q_SCRIPTABLE Q_NOREPLY void | setRaDec (double ra, double dec) |
Q_SCRIPTABLE Q_NOREPLY void | setTracking (bool track) |
void | slotApplyConfigChanges () |
void | slotApplyWIConfigChanges () |
void | slotClearAllTrails () |
void | slotFlagManager () |
void | slotGeoLocator () |
void | slotSetTimeToNow () |
void | slotSetZoom () |
void | slotShowPositionBar (SkyPoint *) |
void | slotTrack () |
void | slotZoomChanged () |
void | updateTime (const bool automaticDSTchange=true) |
Q_SCRIPTABLE Q_NOREPLY void | waitFor (double t) |
Q_SCRIPTABLE Q_NOREPLY void | waitForKey (const QString &k) |
Q_SCRIPTABLE Q_NOREPLY void | writeConfig () |
Q_SCRIPTABLE Q_NOREPLY void | zoom (double z) |
Q_SCRIPTABLE Q_NOREPLY void | zoomIn () |
Q_SCRIPTABLE Q_NOREPLY void | zoomOut () |
Public Member Functions | |
virtual | ~KStars () |
void | addColorMenuItem (const QString &name, const QString &actionName) |
void | applyConfig (bool doApplyFocus=true) |
KStarsData * | data () const |
EkosManager * | ekosManager () const |
Execute * | getExecute () |
FlagManager * | getFlagManager () const |
PrintingWizard * | getPrintingWizard () const |
void | hideAllFovExceptFirst () |
SkyMap * | map () const |
ObservingList * | observingList () const |
void | removeColorMenuItem (const QString &actionName) |
void | selectNextFov () |
void | selectPreviousFov () |
void | showImgExportDialog () |
void | showWI (ObsConditions *obs) |
void | showWISettingsUI () |
void | syncFOVActions () |
Static Public Member Functions | |
static KStars * | createInstance (bool doSplash, bool clockrunning=true, const QString &startDateString=QString()) |
static KStars * | Instance () |
Detailed Description
This is the main window for KStars.
In addition to the GUI elements, the class contains the program clock, KStarsData, and SkyMap objects. It also contains functions for the D-Bus interface.
- Version
- 1.0
Constructor & Destructor Documentation
|
virtual |
Member Function Documentation
void KStars::addColorMenuItem | ( | const QString & | name, |
const QString & | actionName | ||
) |
Add an item to the color-scheme action manu.
- Parameters
-
name The name to use in the menu actionName The internal name for the action (derived from filename)
Definition at line 1154 of file kstarsactions.cpp.
|
slot |
DBUS interface function.
Add a name label to the named object
- Parameters
-
name the name of the object to which the label will be attached
Definition at line 115 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Add a trail to the named solar system body
- Parameters
-
name the name of the body to which the trail will be attached
Definition at line 131 of file kstarsdcop.cpp.
void KStars::applyConfig | ( | bool | doApplyFocus = true | ) |
Apply config options throughout the program.
In most cases, options are set in the "Options" object directly, but for some things we have to manually react to config changes.
- Parameters
-
doApplyFocus If true, then focus posiiton will be set from config file
Definition at line 165 of file kstars.cpp.
|
slot |
DBUS interface function.
modify a view option.
- Parameters
-
option the name of the option to be modified value the option's new value
Definition at line 278 of file kstarsdcop.cpp.
|
slot |
Delete FindDialog because ObjNames list has changed in KStarsData due to reloading star data.
So list in FindDialog must be new filled with current data.
Delete findDialog only if it is not opened
Definition at line 152 of file kstars.cpp.
|
static |
Create an instance of this class.
Destroy any previous instance
- Parameters
-
doSplash clockrunning startDateString
- Note
- See KStars::KStars for details on parameters
- Returns
- a pointer to the instance
Definition at line 129 of file kstars.cpp.
|
inline |
- Returns
- pointer to KStarsData object which contains application data.
|
slot |
DBUS interface function.
reset to the default zoom level.
Definition at line 159 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Draw a line on the sky map.
- Note
- Not Yet Implemented
- Parameters
-
x1 starting x-coordinate of line y1 starting y-coordinate of line x2 ending x-coordinate of line y2 ending y-coordinate of line speed speed at which line should appear from start to end points (in pixels per second)
Definition at line 192 of file kstarsdcop.cpp.
|
inline |
|
slot |
DBUS interface function.
Export the sky image to a file.
- Parameters
-
filename the filename for the exported image width the width for the exported image. Map's width will be used if nothing or an invalid value is supplied. height the height for the exported image. Map's height will be used if nothing or an invalid value is supplied. includeLegend should we include a legend?
Definition at line 429 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Return a URL to retrieve Digitized Sky Survey image.
- Parameters
-
objectName name of the object.
- Note
- If the object is note found, the string "ERROR" is returned.
Definition at line 441 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Return a URL to retrieve Digitized Sky Survey image.
- Parameters
-
RA_J2000 J2000.0 RA Dec_J2000 J2000.0 Declination width width of the image, in arcminutes (default = 15) height height of the image, in arcminutes (default = 15)
Definition at line 451 of file kstarsdcop.cpp.
Execute * KStars::getExecute | ( | ) |
Definition at line 363 of file kstars.cpp.
|
inline |
|
slot |
DBUS interface function.
Return XML containing information about a sky object
- Parameters
-
objectName name of the object.
- Note
- If the object was not found, the XML is empty.
Definition at line 456 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Return a newline-separated list of objects in the observing session plan.
- Note
- Unfortunately, unnamed objects are troublesome. Hopefully, we don't have them on the observing list.
Definition at line 513 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Return a newline-separated list of objects in the observing wishlist.
- Note
- Unfortunately, unnamed objects are troublesome. Hopefully, we don't have them on the observing list.
Definition at line 505 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
- Parameters
-
name the name of the option to query
- Returns
- the current value of the named option
Definition at line 267 of file kstarsdcop.cpp.
|
inline |
|
slot |
DBUS interface function.
Get the dimensions of the Sky Map.
- Returns
- a string containing widthxheight in pixels.
Definition at line 525 of file kstarsdcop.cpp.
void KStars::hideAllFovExceptFirst | ( | ) |
Definition at line 249 of file kstars.cpp.
|
inlinestatic |
|
slot |
DBUS interface function.
Load a color scheme.
- Parameters
-
name the name of the color scheme to load (e.g., "Moonless Night")
Definition at line 406 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Point in the direction described by the string argument.
- Parameters
-
direction either an object name, a compass direction (e.g., "north"), or "zenith"
Definition at line 73 of file kstarsdcop.cpp.
|
inline |
|
inline |
|
slot |
DBUS interface function.
Show text message in a popup window.
- Note
- Not Yet Implemented
- Parameters
-
x x-coordinate for message window y y-coordinate for message window message the text to display in the message window
Definition at line 188 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Print the sky image.
- Parameters
-
usePrintDialog if true, the KDE print dialog will be shown; otherwise, default parameters will be used useChartColors if true, the "Star Chart" color scheme will be used for the printout, which will save ink.
Definition at line 528 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Read config file. This function is useful for restoring the user settings from the config file, after having modified the settings in memory.
- See also
- writeConfig()
Definition at line 245 of file kstarsdcop.cpp.
void KStars::removeColorMenuItem | ( | const QString & | actionName | ) |
Remove an item from the color-scheme action manu.
- Parameters
-
actionName The internal name of the action (derived from filename)
Definition at line 1168 of file kstarsactions.cpp.
|
slot |
DBUS interface function.
Remove a name label from the named object
- Parameters
-
name the name of the object from which the label will be removed
Definition at line 123 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Remove a trail from the named solar system body
- Parameters
-
name the name of the object from which the trail will be removed
Definition at line 139 of file kstarsdcop.cpp.
void KStars::selectNextFov | ( | ) |
Definition at line 272 of file kstars.cpp.
void KStars::selectPreviousFov | ( | ) |
Definition at line 301 of file kstars.cpp.
|
slot |
DBUS interface function.
Set focus to given Alt/Az coordinates.
- Parameters
-
alt the Altitude coordinate for the focus (in Degrees) az the Azimuth coordinate for the focus (in Degrees)
Definition at line 69 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Set the approx field-of-view
- Parameters
-
FOV_Degrees field of view in degrees
Definition at line 521 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Modify a color.
- Parameters
-
colorName the name of the color to be modified (e.g., "SkyColor") value the new color to use
Definition at line 398 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Set the geographic location.
- Parameters
-
city the city name of the location province the province name of the location country the country name of the location
Definition at line 196 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Set local time and date.
- Parameters
-
yr year of date mth month of date day day of date hr hour of time min minute of time sec second of time
Definition at line 163 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Set focus to given Ra/Dec coordinates
- Parameters
-
ra the Right Ascension coordinate for the focus (in Hours) dec the Declination coordinate for the focus (in Degrees)
Definition at line 64 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Toggle tracking.
- Parameters
-
track engage tracking if true; else disengage tracking
Definition at line 184 of file kstarsdcop.cpp.
void KStars::showImgExportDialog | ( | ) |
Definition at line 230 of file kstars.cpp.
void KStars::showWI | ( | ObsConditions * | obs | ) |
void KStars::showWISettingsUI | ( | ) |
Definition at line 329 of file kstars.cpp.
|
slot |
Apply new settings and redraw skymap.
Definition at line 548 of file kstarsactions.cpp.
|
slot |
Apply new settings for WI.
Definition at line 568 of file kstarsactions.cpp.
|
slot |
Remove all trails which may have been added to solar system bodies.
Definition at line 1121 of file kstarsactions.cpp.
|
slot |
action slot: open Flag Manager
Definition at line 397 of file kstarsactions.cpp.
|
slot |
action slot: open dialog for selecting a new geographic location
Definition at line 461 of file kstarsactions.cpp.
|
slot |
action slot: sync kstars clock to system time
Definition at line 600 of file kstarsactions.cpp.
|
slot |
action slot: Allow user to specify a field-of-view angle for the display window in degrees, and set the zoom level accordingly.
Definition at line 992 of file kstarsactions.cpp.
|
slot |
Display position in the status bar.
Definition at line 1208 of file kstarsactions.cpp.
|
slot |
action slot: Toggle whether kstars is tracking current position
Definition at line 892 of file kstarsactions.cpp.
|
slot |
Called when zoom level is changed.
Enables/disables zoom actions and updates status bar.
Definition at line 972 of file kstarsactions.cpp.
void KStars::syncFOVActions | ( | ) |
Definition at line 235 of file kstars.cpp.
|
slot |
Update time-dependent data and (possibly) repaint the sky map.
- Parameters
-
automaticDSTchange change DST status automatically?
Definition at line 334 of file kstars.cpp.
|
slot |
DBUS interface function.
Delay further execution of DBUS commands.
- Parameters
-
t number of seconds to delay
Definition at line 167 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Pause further DBUS execution until a key is pressed.
- Parameters
-
k the key which will resume DBUS execution
Definition at line 173 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Write current settings to config file. This function is useful for storing user settings before modifying them with a DBUS script. The original settings can be restored with readConfig().
- See also
- readConfig()
Definition at line 260 of file kstarsdcop.cpp.
|
slot |
DBUS interface function.
Set zoom level to specified value.
- Parameters
-
z the zoom level. Units are pixels per radian.
Definition at line 147 of file kstarsdcop.cpp.
|
slot |
|
slot |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:36:22 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.