kstars
#include <printingwizard.h>
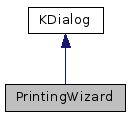
Public Types | |
enum | FOV_TYPE { FT_MANUAL, FT_STARHOPPER, FT_UNDEFINED } |
enum | WIZARD_STEPS { PW_WELCOME = 0, PW_OBJECT_SELECTION = 1, PW_CHART_CONFIG = 2, PW_FOV_TYPE = 3, PW_FOV_CONFIG = 4, PW_FOV_MANUAL = 5, PW_FOV_SH = 6, PW_FOV_BROWSE = 7, PW_CHART_CONTENTS = 8, PW_CHART_PRINT = 9 } |
Public Member Functions | |
PrintingWizard (QWidget *parent=0) | |
~PrintingWizard () | |
void | beginFovCapture () |
void | beginFovCapture (SkyPoint *center, FOV *fov=0) |
void | beginPointing () |
void | beginShBeginPointing () |
void | beginShFovCapture () |
void | captureFov () |
void | fovCaptureDone () |
FinderChart * | getFinderChart () |
SimpleFovExporter * | getFovExporter () |
QSize | getFovImageSize () |
QList< FovSnapshot * > * | getFovSnapshotList () |
FOV_TYPE | getFovType () |
QPrinter * | getPrinter () |
SkyObject * | getShBeginObject () |
SkyObject * | getSkyObject () |
void | pointingDone (SkyObject *obj) |
void | recaptureFov (int idx) |
void | setShBeginObject (SkyObject *obj) |
void | setSkyObject (SkyObject *obj) |
void | updateStepButtons () |
Detailed Description
Class representing Printing Wizard for KStars printed documents (currently only finder charts).
Definition at line 66 of file printingwizard.h.
Member Enumeration Documentation
FOV export method type enumeration.
Enumerator | |
---|---|
FT_MANUAL | |
FT_STARHOPPER | |
FT_UNDEFINED |
Definition at line 90 of file printingwizard.h.
Wizard steps enumeration.
Enumerator | |
---|---|
PW_WELCOME | |
PW_OBJECT_SELECTION | |
PW_CHART_CONFIG | |
PW_FOV_TYPE | |
PW_FOV_CONFIG | |
PW_FOV_MANUAL | |
PW_FOV_SH | |
PW_FOV_BROWSE | |
PW_CHART_CONTENTS | |
PW_CHART_PRINT |
Definition at line 73 of file printingwizard.h.
Constructor & Destructor Documentation
|
explicit |
Constructor.
Definition at line 48 of file printingwizard.cpp.
PrintingWizard::~PrintingWizard | ( | ) |
Destructor.
Definition at line 60 of file printingwizard.cpp.
Member Function Documentation
void PrintingWizard::beginFovCapture | ( | ) |
Hide Printing Wizard and put SkyMap in FOV capture mode.
Definition at line 125 of file printingwizard.cpp.
Hide Printing Wizard and put SkyMap in FOV capture mode.
- Parameters
-
center Point at which SkyMap should be centered. fov Field of view symbol, used to calculate zoom factor.
Definition at line 133 of file printingwizard.cpp.
void PrintingWizard::beginPointing | ( | ) |
Set SkyMap to pointing mode and hide Printing Wizard.
Definition at line 84 of file printingwizard.cpp.
void PrintingWizard::beginShBeginPointing | ( | ) |
Enter star hopping begin pointing mode.
Definition at line 97 of file printingwizard.cpp.
void PrintingWizard::beginShFovCapture | ( | ) |
Capture FOV snapshots using star hopper-based method.
Definition at line 198 of file printingwizard.cpp.
void PrintingWizard::captureFov | ( | ) |
Capture current contents of FOV symbol.
Definition at line 138 of file printingwizard.cpp.
void PrintingWizard::fovCaptureDone | ( | ) |
Disable FOV capture mode.
Definition at line 179 of file printingwizard.cpp.
|
inline |
Get used FinderChart document.
- Returns
- Used FinderChart document.
Definition at line 123 of file printingwizard.h.
|
inline |
Get pointer to the SimpleFovExporter class instance.
- Returns
- Pointer to the SimpleFovExporter instance used by Printing Wizard.
Definition at line 147 of file printingwizard.h.
|
inline |
Get FOV snapshot image size.
- Returns
- Size of the FOV snapshot image.
Definition at line 141 of file printingwizard.h.
|
inline |
Get FovSnapshot list.
- Returns
- Used FovSnapshot list.
Definition at line 135 of file printingwizard.h.
|
inline |
Get used FOV export method.
- Returns
- Selected FOV export method.
Definition at line 111 of file printingwizard.h.
|
inline |
Get printer used by Printing Wizard.
- Returns
- Used printer.
Definition at line 117 of file printingwizard.h.
|
inline |
Get object at which star hopping will begin.
- Returns
- Source object for star hopper.
Definition at line 153 of file printingwizard.h.
|
inline |
Get selected SkyObject, for which FinderChart is created.
- Returns
- Selected SkyObject.
Definition at line 129 of file printingwizard.h.
void PrintingWizard::pointingDone | ( | SkyObject * | obj | ) |
Quit object pointing mode and set the pointed object.
Definition at line 111 of file printingwizard.cpp.
void PrintingWizard::recaptureFov | ( | int | idx | ) |
Recapture FOV snapshot of passed index.
- Parameters
-
idx Index of the element to be recaptured.
Definition at line 271 of file printingwizard.cpp.
|
inline |
Set SkyObject at which star hopper will begin.
- Returns
- SkyObject at which star hopper will begin.
Definition at line 165 of file printingwizard.h.
|
inline |
Set SkyObject for which FinderChart is created.
- Returns
- SkyObject for which finder chart is created.
Definition at line 159 of file printingwizard.h.
void PrintingWizard::updateStepButtons | ( | ) |
Update Next/Previous step buttons.
Definition at line 72 of file printingwizard.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:36:23 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.