kstars
#include <observinglist.h>
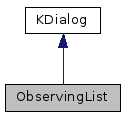
Public Slots | |
void | saveImage (KUrl url, QString filename) |
void | slotAddObject (SkyObject *o=NULL, bool session=false, bool update=false) |
void | slotAddToSession () |
void | slotAddVisibleObj () |
void | slotAVT () |
void | slotCenterObject () |
void | slotChangeTab (int index) |
void | slotDeleteAllImages () |
void | slotDeleteCurrentImage () |
void | slotDetails () |
void | slotDSS () |
void | slotFind () |
void | slotGetImage (bool _dss=false) |
void | slotGoogleImage () |
void | slotImageViewer () |
void | slotLoadWishList () |
void | slotLocation () |
void | slotNewSelection () |
void | slotOALExport () |
void | slotOpenList () |
void | slotRemoveObject (SkyObject *o=NULL, bool session=false, bool update=false) |
void | slotRemoveSelectedObjects () |
void | slotSaveAllImages () |
void | slotSaveImage () |
void | slotSaveList () |
void | slotSaveSession (bool nativeSave=true) |
void | slotSaveSessionAs (bool nativeSave=true) |
void | slotSetTime () |
void | slotSlewToObject () |
void | slotToggleSize () |
void | slotUpdate () |
void | slotWizard () |
void | slotWUT () |
Public Member Functions | |
ObservingList (KStars *_ks) | |
~ObservingList () | |
bool | contains (const SkyObject *o) |
SkyObject * | currentObject () const |
KStarsDateTime | dateTime () |
bool | eventFilter (QObject *obj, QEvent *event) |
double | findAltitude (SkyPoint *p, double hour=0) |
SkyObject * | findObjectByName (QString name) |
GeoLocation * | geoLocation () |
QString | getTime (const SkyObject *o) |
QList< QString > | imageList () |
bool | isLarge () const |
QList< SkyObject * > & | obsList () |
void | plot (SkyObject *o) |
void | saveCurrentList () |
void | saveCurrentUserLog () |
void | saveThumbImage () |
QTime | scheduledTime (SkyObject *o) |
void | selectObject (const SkyObject *o) |
QList< SkyObject * > & | sessionList () |
void | setCurrentImage (const SkyObject *o, bool temp=false) |
void | setDefaultImage () |
void | setSaveImagesButton () |
void | setTime (const SkyObject *o, QTime t) |
Protected Slots | |
void | downloadReady () |
void | slotClose () |
Detailed Description
Tool window for managing a custom list of objects.
The window displays the Name, RA, Dec, mag, and type of each object in the list.
By selecting an object in the list, you can perform a number of functions on that object:
- Center it in the display
- Examine its Details Window
- Point the telescope at it
- Attach a custom icon or name label (TBD)
- Attach a trail (solar system only) (TBD)
- Open the AltVsTime tool
The user can also save/load their observing lists, and can export list data (TBD: as HTML table? CSV format? plain text?)
The observing notes associated with the selected object are displayed below the list.
TODO:
- Implement a "shaded" state, in which the UI is compressed to make it easier to float on the KStars window. Displays only object names, and single-letter action buttons, and no user log.
- Implement an InfoBox version (the ultimate shaded state)
Tool for managing a custom list of objects
- Version
- 1.0
Definition at line 79 of file observinglist.h.
Constructor & Destructor Documentation
ObservingList::ObservingList | ( | KStars * | _ks | ) |
Constructor.
Definition at line 89 of file observinglist.cpp.
ObservingList::~ObservingList | ( | ) |
Destuctor (empty)
Definition at line 219 of file observinglist.cpp.
Member Function Documentation
|
inline |
- Returns
- true if the object is in the observing list
o
pointer to the object to test.
Definition at line 94 of file observinglist.h.
|
inline |
- Returns
- pointer to the currently-selected object in the observing list
- Note
- if more than one object is selected, this function returns 0.
Definition at line 111 of file observinglist.h.
|
inline |
Definition at line 166 of file observinglist.h.
|
protectedslot |
Definition at line 1078 of file observinglist.cpp.
bool ObservingList::eventFilter | ( | QObject * | obj, |
QEvent * | event | ||
) |
This is the declaration of the event filter function which is installed on the KImageFilePreview and the TabeView.
Definition at line 1221 of file observinglist.cpp.
double ObservingList::findAltitude | ( | SkyPoint * | p, |
double | hour = 0 |
||
) |
Return the altitude of the given SkyObject for the given hour.
p
pointer to the SkyObject hour
time at which altitude has to be found
Definition at line 939 of file observinglist.cpp.
SkyObject * ObservingList::findObjectByName | ( | QString | name | ) |
return the object with the name as the passed QString from the Session List, return null otherwise
Definition at line 1334 of file observinglist.cpp.
|
inline |
Definition at line 164 of file observinglist.h.
|
inline |
Definition at line 158 of file observinglist.h.
|
inline |
Return the list of downloaded images.
Definition at line 131 of file observinglist.h.
|
inline |
- Returns
- true if the window is in its default "large" state.
Definition at line 98 of file observinglist.h.
- Returns
- reference to the current observing list
Definition at line 102 of file observinglist.h.
void ObservingList::plot | ( | SkyObject * | o | ) |
Plot the SkyObject's Altitude vs Time in the AVTPlotWidget.
o
pointer to the object to be plotted
Definition at line 910 of file observinglist.cpp.
void ObservingList::saveCurrentList | ( | ) |
If the current list has unsaved changes, ask the user about saving it.
- Note
- also clears the list in preparation of opening a new one
Definition at line 799 of file observinglist.cpp.
void ObservingList::saveCurrentUserLog | ( | ) |
Save the user log text to a file.
- Note
- the log is attached to the current object in obsList.
Definition at line 735 of file observinglist.cpp.
|
slot |
saves the image syncronously from a given URL into a given file url
the url from which the image has to be downloaded filename
the file onto which the url has to be copied to NOTE: This is not a generic image saver, it is specific to the current object
Definition at line 1153 of file observinglist.cpp.
void ObservingList::saveThumbImage | ( | ) |
saves a thumbnail image for the details dialog from the downloaded image
Definition at line 1306 of file observinglist.cpp.
|
inline |
Definition at line 160 of file observinglist.h.
void ObservingList::selectObject | ( | const SkyObject * | o | ) |
make a selection in the session view
Definition at line 1341 of file observinglist.cpp.
- Returns
- reference to the current observing list
Definition at line 106 of file observinglist.h.
void ObservingList::setCurrentImage | ( | const SkyObject * | o, |
bool | temp = false |
||
) |
Sets the image parameters for the current object o
The passed object for setting the parameters temp
A flag to edit the name for temporary images.
Definition at line 1096 of file observinglist.cpp.
void ObservingList::setDefaultImage | ( | ) |
set the default image in the image preview.
Definition at line 1354 of file observinglist.cpp.
void ObservingList::setSaveImagesButton | ( | ) |
decides on whether to enable the SaveImages button or not
Definition at line 1210 of file observinglist.cpp.
|
inline |
Definition at line 162 of file observinglist.h.
|
slot |
add a new object to list o
pointer to the object to add to the list session
flag toggle adding the object to the session list update
flag to toggle the call of slotSaveList
Definition at line 226 of file observinglist.cpp.
|
slot |
Add the object to the Session List.
Definition at line 658 of file observinglist.cpp.
|
slot |
Definition at line 1318 of file observinglist.cpp.
|
slot |
Show the Altitude vs Time for selecteld objects.
Definition at line 681 of file observinglist.cpp.
|
slot |
center the selected object in the display
Definition at line 584 of file observinglist.cpp.
|
slot |
toggle the setEnabled flags according to current view set the m_currentItem to NULL and clear selections index
captures the integer value sent by the signal which is the currentIndex of the table
Definition at line 1001 of file observinglist.cpp.
|
protectedslot |
Definition at line 726 of file observinglist.cpp.
|
slot |
Removes all the save DSS/SDSS images from the disk.
Definition at line 1186 of file observinglist.cpp.
|
slot |
Remove the current image.
Definition at line 1300 of file observinglist.cpp.
|
slot |
Show the details window for the selected object.
Definition at line 642 of file observinglist.cpp.
|
inlineslot |
download the DSS image and show it
Definition at line 325 of file observinglist.h.
|
slot |
Open the Find Dialog.
Definition at line 670 of file observinglist.cpp.
|
slot |
Downloads the corresponding DSS or SDSS image from the web and displays it.
Definition at line 1061 of file observinglist.cpp.
|
slot |
Definition at line 1283 of file observinglist.cpp.
|
slot |
Shows the image in a ImageViewer window.
Definition at line 1175 of file observinglist.cpp.
|
slot |
Load the Wish list from disk.
Definition at line 848 of file observinglist.cpp.
|
slot |
Opens the Location dialog to set the GeoLocation for the sessionlist.
Definition at line 1026 of file observinglist.cpp.
|
slot |
Tasks needed when changing the selected object Save the user log of the previous selected object, find the new selected object in the obsList, and show the notes associated with the new selected object.
Definition at line 455 of file observinglist.cpp.
|
slot |
Export a target list to the oal compliant format.
Definition at line 1314 of file observinglist.cpp.
|
slot |
load an observing list from disk.
Definition at line 746 of file observinglist.cpp.
|
slot |
Remove skyobject from the observing list.
o
pointer to the SkyObject to be removed. session
flag to tell it whether to remove the object from the sessionlist or from the wishlist update
flag to toggle the call of slotSaveList Use SkyMap::clickedObject() if o is NULL (default)
Definition at line 334 of file observinglist.cpp.
|
slot |
Remove skyobjects which are highlighted in the observing list tool from the observing list.
Definition at line 393 of file observinglist.cpp.
|
slot |
Downloads the images of all the objects in the session list Note: This downloads the SDSS image, checks if the size is > default image and gets the DSS image if thats the case.
Definition at line 1129 of file observinglist.cpp.
|
slot |
saves the temporary image permanently
Definition at line 1168 of file observinglist.cpp.
|
slot |
save the current observing list to disk.
Definition at line 820 of file observinglist.cpp.
|
slot |
save the current session
Definition at line 879 of file observinglist.cpp.
|
slot |
save the current observing session plan to disk, specify filename.
Definition at line 812 of file observinglist.cpp.
|
slot |
Takes the time from the QTimeEdit box and sets it as the time parameter in the tableview of the SessionList.
Definition at line 1054 of file observinglist.cpp.
|
slot |
slew the telescope to the selected object
Definition at line 599 of file observinglist.cpp.
|
slot |
toggle between the large and small window states
Definition at line 949 of file observinglist.cpp.
|
slot |
Updates the tableviews for the new geolocation and date.
Definition at line 1035 of file observinglist.cpp.
|
slot |
construct a new observing list using the wizard.
Definition at line 900 of file observinglist.cpp.
|
slot |
Open the WUT dialog.
Definition at line 650 of file observinglist.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:36:23 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.