kstars
Satellite Class Reference
#include <satellite.h>
Inheritance diagram for Satellite:
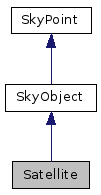
Public Member Functions | |
Satellite (QString name, QString line1, QString line2) | |
~Satellite () | |
double | altitude () |
QString | id () |
bool | isVisible () |
double | range () |
bool | selected () |
void | setSelected (bool selected) |
void | updatePos () |
double | velocity () |
![]() | |
SkyObject (int t=TYPE_UNKNOWN, dms r=dms(0.0), dms d=dms(0.0), float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
SkyObject (int t, double r, double d, float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
virtual | ~SkyObject () |
virtual SkyObject * | clone () const |
virtual UID | getUID () const |
bool | hasAuxInfo () |
bool | hasLongName () const |
bool | hasName () const |
bool | hasName2 () const |
QStringList & | ImageList () |
QStringList & | ImageTitle () |
QStringList & | InfoList () |
QStringList & | InfoTitle () |
bool | isSolarSystem () const |
virtual double | labelOffset () const |
virtual QString | labelString () const |
virtual QString | longname (void) const |
float | mag (void) const |
float | mag (const QString &band) const |
QString | messageFromTitle (const QString &imageTitle) |
virtual QString | name (void) const |
QString | name2 (void) const |
QString & | notes () |
virtual double | pa () const |
SkyPoint | recomputeCoords (const KStarsDateTime &dt, const GeoLocation *geo=0) |
QTime | riseSetTime (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) |
dms | riseSetTimeAz (const KStarsDateTime &dt, const GeoLocation *geo, bool rst) |
QTime | riseSetTimeUT (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) |
void | saveUserLog (const QString &newLog) |
void | setLongName (const QString &longname=QString()) |
void | setNotes (QString _notes) |
void | setType (int t) |
void | showPopupMenu (KSPopupMenu *pmenu, const QPoint &pos) |
dms | transitAltitude (const KStarsDateTime &dt, const GeoLocation *geo) |
QTime | transitTime (const KStarsDateTime &dt, const GeoLocation *geo) |
QTime | transitTimeUT (const KStarsDateTime &dt, const GeoLocation *geo) |
QString | translatedLongName () const |
QString | translatedName () const |
QString | translatedName2 () const |
int | type (void) const |
QString | typeName () const |
QString & | userLog () |
![]() | |
SkyPoint (const dms &r, const dms &d) | |
SkyPoint (double r, double d) | |
SkyPoint () | |
virtual | ~SkyPoint () |
void | aberrate (const KSNumbers *num) |
void | addEterms (void) |
const dms & | alt () const |
dms | altRefracted () const |
dms | angularDistanceTo (const SkyPoint *sp, double *const positionAngle=0) const |
void | apparentCoord (long double jd0, long double jdf) |
const dms & | az () const |
void | B1950ToJ2000 (void) |
bool | bendlight () |
bool | checkBendLight () |
bool | checkCircumpolar (const dms *gLat) |
const dms & | dec () const |
const dms & | dec0 () const |
SkyPoint | deprecess (const KSNumbers *num, long double epoch=J2000) |
void | Equatorial1950ToGalactic (dms &galLong, dms &galLat) |
void | EquatorialToHorizontal (const dms *LST, const dms *lat) |
SkyPoint | Eterms (void) |
void | findEcliptic (const dms *Obliquity, dms &EcLong, dms &EcLat) |
void | GalacticToEquatorial1950 (const dms *galLong, const dms *galLat) |
void | HorizontalToEquatorial (const dms *LST, const dms *lat) |
void | J2000ToB1950 (void) |
SkyPoint | moveAway (const SkyPoint &from, double dist) |
void | nutate (const KSNumbers *num) |
bool | operator== (SkyPoint &p) |
void | precessFromAnyEpoch (long double jd0, long double jdf) |
const dms & | ra () const |
const dms & | ra0 () const |
void | set (const dms &r, const dms &d) |
void | setAlt (dms alt) |
void | setAlt (double alt) |
void | setAz (dms az) |
void | setAz (double az) |
void | setDec (dms d) |
void | setDec (double d) |
void | setDec0 (dms d) |
void | setDec0 (double d) |
void | setFromEcliptic (const dms *Obliquity, const dms &EcLong, const dms &EcLat) |
void | setRA (dms r) |
void | setRA (double r) |
void | setRA0 (dms r) |
void | setRA0 (double r) |
void | subtractEterms (void) |
virtual void | updateCoords (KSNumbers *num, bool includePlanets=true, const dms *lat=0, const dms *LST=0, bool forceRecompute=false) |
double | vGeocentric (double vhelio, long double jd) |
double | vGeoToVHelio (double vgeo, long double jd) |
double | vHeliocentric (double vlsr, long double jd) |
double | vHelioToVlsr (double vhelio, long double jd) |
double | vREarth (long double jd0) |
double | vRSite (double vsite[3]) |
double | vRSun (long double jd) |
double | vTopocentric (double vgeo, double vsite[3]) |
double | vTopoToVGeo (double vtopo, double vsite[3]) |
Additional Inherited Members | |
![]() | |
enum | TYPE { STAR =0, CATALOG_STAR =1, PLANET =2, OPEN_CLUSTER =3, GLOBULAR_CLUSTER =4, GASEOUS_NEBULA =5, PLANETARY_NEBULA =6, SUPERNOVA_REMNANT =7, GALAXY =8, COMET =9, ASTEROID =10, CONSTELLATION =11, MOON =12, ASTERISM =13, GALAXY_CLUSTER =14, DARK_NEBULA =15, QUASAR =16, MULT_STAR =17, RADIO_SOURCE =18, SATELLITE =19, SUPERNOVA =20, TYPE_UNKNOWN } |
typedef qint64 | UID |
![]() | |
static QString | typeName (const int t) |
![]() | |
static double | refract (const double alt) |
static dms | refract (const dms alt) |
static double | refractionCorr (double alt) |
static double | unrefract (const double alt) |
static dms | unrefract (const dms alt) |
![]() | |
static const UID | invalidUID = ~0 |
static const UID | UID_DEEPSKY = 2 |
static const UID | UID_GALAXY = 1 |
static const UID | UID_SOLARSYS = 3 |
static const UID | UID_STAR = 0 |
![]() | |
static const double | altCrit = -1.0 |
![]() | |
void | setMag (float m) |
void | setMag (const QString &band, const double mag) |
void | setName (const QString &name) |
void | setName2 (const QString &name2=QString()) |
![]() | |
void | precess (const KSNumbers *num) |
![]() | |
QSharedDataPointer< AuxInfo > | info |
QString | LongName |
QString | Name |
QString | Name2 |
![]() | |
long double | lastPrecessJD |
![]() | |
static QString | emptyString |
static QString | starString = QString("star") |
static QString | unnamedObjectString = QString(I18N_NOOP("unnamed object")) |
static QString | unnamedString = QString(I18N_NOOP("unnamed")) |
Detailed Description
Constructor & Destructor Documentation
Satellite::Satellite | ( | QString | name, |
QString | line1, | ||
QString | line2 | ||
) |
Constructor.
Definition at line 62 of file satellite.cpp.
Satellite::~Satellite | ( | ) |
Destructor.
Definition at line 108 of file satellite.cpp.
Member Function Documentation
double Satellite::altitude | ( | ) |
- Returns
- Satellite altitude in km
Definition at line 1220 of file satellite.cpp.
QString Satellite::id | ( | ) |
- Returns
- Satellite international designator
Definition at line 1230 of file satellite.cpp.
bool Satellite::isVisible | ( | ) |
- Returns
- True if the satellite is visible (above horizon, in the sunlight and sun at least 12° under horizon)
Definition at line 1196 of file satellite.cpp.
double Satellite::range | ( | ) |
- Returns
- Satellite range from observer in km
Definition at line 1225 of file satellite.cpp.
bool Satellite::selected | ( | ) |
- Returns
- True if the satellite is selected
Definition at line 1201 of file satellite.cpp.
void Satellite::setSelected | ( | bool | selected | ) |
Select or not the satellite.
Definition at line 1206 of file satellite.cpp.
void Satellite::updatePos | ( | ) |
Update satellite position.
Definition at line 647 of file satellite.cpp.
double Satellite::velocity | ( | ) |
- Returns
- Satellite velocity in km/s
Definition at line 1215 of file satellite.cpp.
The documentation for this class was generated from the following files:
This file is part of the KDE documentation.
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:36:23 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:36:23 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.