kstars
#include <skymapcomposite.h>
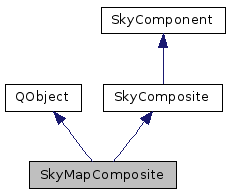
Signals | |
void | progressText (const QString &message) |
Additional Inherited Members | |
![]() | |
void | removeFromNames (const SkyObject *obj) |
Detailed Description
SkyMapComposite is the root object in the object hierarchy of the sky map.
All requests to update, init, draw etc. will be done with this class. The requests will be delegated to it's children. The object hierarchy will created by adding new objects via addComponent().
- Version
- 0.1
Definition at line 65 of file skymapcomposite.h.
Constructor & Destructor Documentation
|
explicit |
Constructor parent
pointer to the parent SkyComponent.
Definition at line 58 of file skymapcomposite.cpp.
SkyMapComposite::~SkyMapComposite | ( | ) |
Definition at line 109 of file skymapcomposite.cpp.
Member Function Documentation
void SkyMapComposite::addCustomCatalog | ( | const QString & | filename, |
int | index | ||
) |
Definition at line 468 of file skymapcomposite.cpp.
bool SkyMapComposite::addNameLabel | ( | SkyObject * | o | ) |
Definition at line 395 of file skymapcomposite.cpp.
Definition at line 568 of file skymapcomposite.cpp.
Definition at line 572 of file skymapcomposite.cpp.
Definition at line 558 of file skymapcomposite.cpp.
QString SkyMapComposite::currentCulture | ( | ) |
Definition at line 601 of file skymapcomposite.cpp.
QList< SkyComponent * > SkyMapComposite::customCatalogs | ( | ) |
Definition at line 585 of file skymapcomposite.cpp.
const QList< DeepSkyObject * > & SkyMapComposite::deepSkyObjects | ( | ) | const |
Definition at line 554 of file skymapcomposite.cpp.
|
virtual |
Delegate draw requests to all sub components psky
Reference to the QPainter on which to paint.
Reimplemented from SkyComposite.
Definition at line 170 of file skymapcomposite.cpp.
KSPlanet * SkyMapComposite::earth | ( | ) |
Definition at line 581 of file skymapcomposite.cpp.
|
virtual |
Emit signal about progress.
Reimplemented from SkyComponent.
Definition at line 548 of file skymapcomposite.cpp.
|
virtual |
Search the children of this SkyMapComposite for a SkyObject whose name matches the argument.
The objects' primary, secondary and long-form names will all be checked for a match.
- Note
- Overloaded from SkyComposite. In this version, we search the most likely object classes first to be more efficient.
name
the name to be matched
- Returns
- a pointer to the SkyObject whose name matches the argument, or a NULL pointer if no match was found.
Reimplemented from SkyComposite.
Definition at line 426 of file skymapcomposite.cpp.
QList< SkyObject * > SkyMapComposite::findObjectsInArea | ( | const SkyPoint & | p1, |
const SkyPoint & | p2 | ||
) |
- Returns
- the list of objects in the region defined by skypoints
- Parameters
-
p1 first sky point (top-left vertex of rectangular region) p2 second sky point (bottom-right vertex of rectangular region)
Definition at line 413 of file skymapcomposite.cpp.
SkyObject * SkyMapComposite::findStarByGenetiveName | ( | const QString | name | ) |
Definition at line 449 of file skymapcomposite.cpp.
FlagComponent * SkyMapComposite::flags | ( | ) |
Definition at line 605 of file skymapcomposite.cpp.
|
inline |
Definition at line 194 of file skymapcomposite.h.
QString SkyMapComposite::getCultureName | ( | int | index | ) |
Definition at line 593 of file skymapcomposite.cpp.
QStringList SkyMapComposite::getCultureNames | ( | ) |
Definition at line 589 of file skymapcomposite.cpp.
|
inline |
Definition at line 195 of file skymapcomposite.h.
bool SkyMapComposite::isLocalCNames | ( | ) |
Definition at line 544 of file skymapcomposite.cpp.
Definition at line 175 of file skymapcomposite.h.
- Returns
- the object nearest a given point in the sky.
- Parameters
-
p The point to find an object near maxrad The maximum search radius, in Degrees
- Note
- the angular separation to the matched object is returned through the maxrad variable.
Reimplemented from SkyComposite.
Definition at line 309 of file skymapcomposite.cpp.
KSPlanetBase * SkyMapComposite::planet | ( | int | n | ) |
Definition at line 453 of file skymapcomposite.cpp.
|
signal |
void SkyMapComposite::reloadAsteroids | ( | ) |
void SkyMapComposite::reloadCLines | ( | ) |
Definition at line 490 of file skymapcomposite.cpp.
void SkyMapComposite::reloadCNames | ( | ) |
Definition at line 499 of file skymapcomposite.cpp.
void SkyMapComposite::reloadComets | ( | ) |
void SkyMapComposite::reloadDeepSky | ( | ) |
Definition at line 512 of file skymapcomposite.cpp.
void SkyMapComposite::removeCustomCatalog | ( | const QString & | name | ) |
Definition at line 477 of file skymapcomposite.cpp.
bool SkyMapComposite::removeNameLabel | ( | SkyObject * | o | ) |
Definition at line 401 of file skymapcomposite.cpp.
SatellitesComponent * SkyMapComposite::satellites | ( | ) |
Definition at line 609 of file skymapcomposite.cpp.
void SkyMapComposite::setCurrentCulture | ( | QString | culture | ) |
Definition at line 597 of file skymapcomposite.cpp.
SolarSystemComposite * SkyMapComposite::solarSystemComposite | ( | ) |
Definition at line 613 of file skymapcomposite.cpp.
- Returns
- the star nearest a given point in the sky.
- Parameters
-
p The point to find a star near maxrad The maximum search radius, in Degrees
- Note
- the angular separation to the matched star is returned through the maxrad variable.
Definition at line 379 of file skymapcomposite.cpp.
Definition at line 563 of file skymapcomposite.cpp.
Definition at line 576 of file skymapcomposite.cpp.
SupernovaeComponent * SkyMapComposite::supernovaeComponent | ( | ) |
Definition at line 618 of file skymapcomposite.cpp.
|
virtual |
Delegate update-position requests to all sub components.
This function usually just updates the Horizontal (Azimuth/Altitude) coordinates. However, the precession and nutation must also be recomputed periodically. Requests to do so are sent through the doPrecess parameter. num
Pointer to the KSNumbers object
- See also
- updatePlanets()
- updateMoons()
- Note
- By default, the num parameter is NULL, indicating that Precession/Nutation computation should be skipped; this computation is only occasionally required.
Reimplemented from SkyComposite.
Definition at line 117 of file skymapcomposite.cpp.
|
virtual |
Delegate moon position updates to the SolarSystemComposite.
Planet positions change over time, so they need to be recomputed periodically, but not on every call to update(). This function will recompute the positions of the Earth's Moon and Jupiter's four Galilean moons. These objects are done separately from the other solar system bodies, because their positions change more rapidly, and so updateMoons() must be called more often than updatePlanets(). num
Pointer to the KSNumbers object
Reimplemented from SkyComponent.
Definition at line 159 of file skymapcomposite.cpp.
|
virtual |
Delegate planet position updates to the SolarSystemComposite.
Planet positions change over time, so they need to be recomputed periodically, but not on every call to update(). This function will recompute the positions of all solar system bodies except the Earth's Moon, Jupiter's Moons AND Saturn Moons (because these objects' positions change on a much more rapid timescale). num
Pointer to the KSNumbers object
Reimplemented from SkyComponent.
Definition at line 154 of file skymapcomposite.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:36:23 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.