marble
#include <GeoDataCoordinates.h>
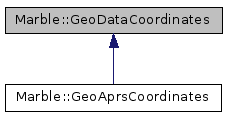
Public Types | |
enum | BearingType { InitialBearing, FinalBearing } |
enum | Notation { Decimal, DMS, DM, UTM, Astro } |
typedef QVector < GeoDataCoordinates * > | PtrVector |
enum | Unit { Radian, Degree } |
typedef QVector < GeoDataCoordinates > | Vector |
Static Public Member Functions | |
static GeoDataCoordinates::Notation | defaultNotation () |
static GeoDataCoordinates | fromString (const QString &string, bool &successful) |
static QString | latToString (qreal lat, GeoDataCoordinates::Notation notation, GeoDataCoordinates::Unit unit=Radian, int precision=-1, char format= 'f') |
static QString | lonToString (qreal lon, GeoDataCoordinates::Notation notation, GeoDataCoordinates::Unit unit=Radian, int precision=-1, char format= 'f') |
static qreal | normalizeLat (qreal lat, GeoDataCoordinates::Unit=GeoDataCoordinates::Radian) |
static qreal | normalizeLon (qreal lon, GeoDataCoordinates::Unit=GeoDataCoordinates::Radian) |
static void | normalizeLonLat (qreal &lon, qreal &lat, GeoDataCoordinates::Unit=GeoDataCoordinates::Radian) |
static void | setDefaultNotation (GeoDataCoordinates::Notation notation) |
Protected Attributes | |
GeoDataCoordinatesPrivate * | d |
Detailed Description
A 3d point representation.
GeoDataCoordinates is the simple representation of a single three dimensional point. It can be used all through out marble as the data type for three dimensional objects. it comprises of a Quaternion for speed issues. This class was introduced to reflect the difference between a simple 3d point and the GeoDataGeometry object containing such a point. The latter is a GeoDataPoint and is simply derived from GeoDataCoordinates.
- See also
- GeoDataPoint
Definition at line 52 of file GeoDataCoordinates.h.
Member Typedef Documentation
typedef QVector<GeoDataCoordinates*> Marble::GeoDataCoordinates::PtrVector |
Definition at line 103 of file GeoDataCoordinates.h.
typedef QVector<GeoDataCoordinates> Marble::GeoDataCoordinates::Vector |
Definition at line 102 of file GeoDataCoordinates.h.
Member Enumeration Documentation
The BearingType enum specifies where to measure the bearing along great circle arcs.
When traveling along a great circle arc defined by the two points A and B, the bearing varies along the arc. The "InitialBearing" bearing corresponds to the bearing value at A, the "FinalBearing" bearing to that at B.
Enumerator | |
---|---|
InitialBearing | |
FinalBearing |
Definition at line 96 of file GeoDataCoordinates.h.
enum used to specify the notation / numerical system
For degrees there exist two notations: "Decimal" (base-10) and the "Sexagesimal DMS" (base-60) which is traditionally used in cartography. Decimal notation uses floating point numbers to specify parts of a degree. The Sexagesimal DMS notation uses integer based Degrees-(Arc)Minutes-(Arc)Seconds to describe parts of a degree.
Definition at line 79 of file GeoDataCoordinates.h.
enum used constructor to specify the units used
Internally we always use radian for mathematical convenience. However the Marble's interfaces to the outside should default to degrees.
Enumerator | |
---|---|
Radian | |
Degree |
Definition at line 64 of file GeoDataCoordinates.h.
Constructor & Destructor Documentation
Marble::GeoDataCoordinates::GeoDataCoordinates | ( | const GeoDataCoordinates & | other | ) |
Definition at line 596 of file GeoDataCoordinates.cpp.
Marble::GeoDataCoordinates::GeoDataCoordinates | ( | ) |
constructs an invalid instance
Constructs an invalid instance such that calling isValid() on it will return
.
Definition at line 605 of file GeoDataCoordinates.cpp.
Marble::GeoDataCoordinates::GeoDataCoordinates | ( | qreal | lon, |
qreal | lat, | ||
qreal | alt = 0 , |
||
GeoDataCoordinates::Unit | unit = GeoDataCoordinates::Radian , |
||
int | detail = 0 |
||
) |
create a geocoordinate from longitude and latitude
- Parameters
-
_lon longitude _lat latitude alt altitude in meters (default: 0) _unit units that lon and lat get measured in (default for Radian: north pole at pi/2, southpole at -pi/2) _detail detail (default: 0)
Definition at line 587 of file GeoDataCoordinates.cpp.
|
virtual |
Definition at line 615 of file GeoDataCoordinates.cpp.
Member Function Documentation
qreal Marble::GeoDataCoordinates::altitude | ( | ) | const |
return the altitude of the Point in meters
Definition at line 1197 of file GeoDataCoordinates.cpp.
qreal Marble::GeoDataCoordinates::bearing | ( | const GeoDataCoordinates & | other, |
Unit | unit = Radian , |
||
BearingType | type = InitialBearing |
||
) | const |
Returns the bearing (true bearing, the angle between the line defined by this point and the other and the prime meridian)
- Parameters
-
other The second point that, together with this point, defines a line unit Unit of the result
- Returns
- The true bearing in the requested unit, not range normalized, in clockwise direction, with the value 0 corresponding to north
Definition at line 1213 of file GeoDataCoordinates.cpp.
|
static |
return Notation of string representation
Definition at line 764 of file GeoDataCoordinates.cpp.
|
virtual |
Definition at line 636 of file GeoDataCoordinates.cpp.
int Marble::GeoDataCoordinates::detail | ( | ) | const |
return the detail flag detail range: 0 for most important points, 5 for least important
Definition at line 1202 of file GeoDataCoordinates.cpp.
|
static |
try to parse the string into a coordinate pair
- Parameters
-
successful becomes true if the conversion succeeds
- Returns
- the geodatacoordinates
Definition at line 909 of file GeoDataCoordinates.cpp.
void Marble::GeoDataCoordinates::geoCoordinates | ( | qreal & | lon, |
qreal & | lat, | ||
GeoDataCoordinates::Unit | unit = GeoDataCoordinates::Radian |
||
) | const |
use this function to get the longitude and latitude with one call - use the unit parameter to switch between Radian and DMS
- Parameters
-
lon longitude lat latitude unit units that lon and lat get measured in (default for Radian: north pole at pi/2, southpole at -pi/2)
Definition at line 715 of file GeoDataCoordinates.cpp.
void Marble::GeoDataCoordinates::geoCoordinates | ( | qreal & | lon, |
qreal & | lat, | ||
qreal & | alt, | ||
GeoDataCoordinates::Unit | unit = GeoDataCoordinates::Radian |
||
) | const |
use this function to get the longitude, latitude and altitude with one call - use the unit parameter to switch between Radian and DMS
- Parameters
-
lon longitude lat latitude alt altitude in meters unit units that lon and lat get measured in (default for Radian: north pole at pi/2, southpole at -pi/2)
Definition at line 732 of file GeoDataCoordinates.cpp.
return whether our coordinates represent a pole This method can be used to check whether the coordinate equals one of the poles.
Definition at line 1231 of file GeoDataCoordinates.cpp.
bool Marble::GeoDataCoordinates::isValid | ( | ) | const |
Returns.
if the coordinate is valid,
otherwise.
- Returns
- whether the coordinate is valid
A coordinate is valid, if at least one component has been set and the last assignment was not an invalid GeoDataCoordinates object.
Definition at line 624 of file GeoDataCoordinates.cpp.
qreal Marble::GeoDataCoordinates::latitude | ( | GeoDataCoordinates::Unit | unit = GeoDataCoordinates::Radian | ) | const |
retrieves the latitude of the GeoDataCoordinates object use the unit parameter to switch between Radian and DMS
- Parameters
-
unit units that lon and lat get measured in (default for Radian: north pole at pi/2, southpole at -pi/2)
- Returns
- latitude
Definition at line 751 of file GeoDataCoordinates.cpp.
|
static |
Definition at line 1080 of file GeoDataCoordinates.cpp.
QString Marble::GeoDataCoordinates::latToString | ( | ) | const |
return a string representation of latitude of the coordinate convenience function that uses the default notation
Definition at line 1176 of file GeoDataCoordinates.cpp.
qreal Marble::GeoDataCoordinates::longitude | ( | GeoDataCoordinates::Unit | unit = GeoDataCoordinates::Radian | ) | const |
retrieves the longitude of the GeoDataCoordinates object use the unit parameter to switch between Radian and DMS
- Parameters
-
unit units that lon and lat get measured in (default for Radian: north pole at pi/2, southpole at -pi/2)
- Returns
- longitude
Definition at line 739 of file GeoDataCoordinates.cpp.
|
static |
Definition at line 933 of file GeoDataCoordinates.cpp.
QString Marble::GeoDataCoordinates::lonToString | ( | ) | const |
return a string representation of longitude of the coordinate convenience function that uses the default notation
Definition at line 1075 of file GeoDataCoordinates.cpp.
|
static |
normalize latitude to always be in -M_PI / 2.
<= lat <= +M_PI / 2 (Radian).
- Parameters
-
lat latitude
Definition at line 799 of file GeoDataCoordinates.cpp.
|
static |
normalize the longitude to always be -M_PI <= lon <= +M_PI (Radian).
- Parameters
-
lon longitude
Definition at line 776 of file GeoDataCoordinates.cpp.
|
static |
normalize both longitude and latitude at the same time This method normalizes both latitude and longitude, so that the latitude and the longitude stay within the "usual" range.
NOTE: If the latitude exceeds M_PI/2 (+90.0 deg) or -M_PI/2 (-90.0 deg) then this will be interpreted as a pole traversion where the point will end up on the opposite side of the globe. Therefore the longitude will change by M_PI (180 deg). If you don't want this behaviour use both normalizeLat() and normalizeLon() instead.
- Parameters
-
lon the longitude value lat the latitude value
Definition at line 845 of file GeoDataCoordinates.cpp.
|
virtual |
Definition at line 1186 of file GeoDataCoordinates.cpp.
GeoDataCoordinates & Marble::GeoDataCoordinates::operator= | ( | const GeoDataCoordinates & | other | ) |
Definition at line 1287 of file GeoDataCoordinates.cpp.
|
virtual |
Definition at line 1181 of file GeoDataCoordinates.cpp.
|
virtual |
Serialize the contents of the feature to stream
.
Definition at line 1293 of file GeoDataCoordinates.cpp.
const Quaternion & Marble::GeoDataCoordinates::quaternion | ( | ) | const |
return a Quaternion with the used coordinates
Definition at line 1226 of file GeoDataCoordinates.cpp.
void Marble::GeoDataCoordinates::set | ( | qreal | lon, |
qreal | lat, | ||
qreal | alt = 0 , |
||
GeoDataCoordinates::Unit | unit = GeoDataCoordinates::Radian |
||
) |
(re)set the coordinates in a GeoDataCoordinates object
- Parameters
-
_lon longitude _lat latitude alt altitude in meters (default: 0) _unit units that lon and lat get measured in (default for Radian: north pole at pi/2, southpole at -pi/2)
Definition at line 657 of file GeoDataCoordinates.cpp.
void Marble::GeoDataCoordinates::setAltitude | ( | const qreal | altitude | ) |
set the altitude of the Point in meters
- Parameters
-
altitude altitude
Definition at line 1191 of file GeoDataCoordinates.cpp.
|
static |
set the Notation of the string representation
- Parameters
-
notation Notation
Definition at line 770 of file GeoDataCoordinates.cpp.
void Marble::GeoDataCoordinates::setDetail | ( | const int | det | ) |
void Marble::GeoDataCoordinates::setLatitude | ( | qreal | lat, |
GeoDataCoordinates::Unit | unit = GeoDataCoordinates::Radian |
||
) |
set the longitude in a GeoDataCoordinates object
- Parameters
-
_lat longitude _unit units that lon and lat get measured in (default for Radian: north pole at pi/2, southpole at -pi/2)
Definition at line 699 of file GeoDataCoordinates.cpp.
void Marble::GeoDataCoordinates::setLongitude | ( | qreal | lon, |
GeoDataCoordinates::Unit | unit = GeoDataCoordinates::Radian |
||
) |
set the longitude in a GeoDataCoordinates object
- Parameters
-
_lon longitude _unit units that lon and lat get measured in (default for Radian: north pole at pi/2, southpole at -pi/2)
Definition at line 679 of file GeoDataCoordinates.cpp.
QString Marble::GeoDataCoordinates::toString | ( | ) | const |
return a string representation of the coordinate this is a convenience function which uses the default notation
Definition at line 921 of file GeoDataCoordinates.cpp.
QString Marble::GeoDataCoordinates::toString | ( | GeoDataCoordinates::Notation | notation, |
int | precision = -1 |
||
) | const |
return a string with the notation given by notation
- Parameters
-
notation set a notation different from the default one precision set the number of digits below degrees. The precision depends on the current notation: For Decimal representation the precision is the number of digits after the decimal point. In DMS a precision of 1 or 2 shows the arc minutes; a precision of 3 or 4 will show arc seconds. A precision beyond that will increase the number of digits after the arc second decimal point.
Definition at line 926 of file GeoDataCoordinates.cpp.
|
virtual |
Unserialize the contents of the feature from stream
.
Definition at line 1300 of file GeoDataCoordinates.cpp.
Member Data Documentation
|
protected |
Definition at line 356 of file GeoDataCoordinates.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:38:55 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.