step/stepcore
StepCore::Controller Class Reference
#include <tool.h>
Inheritance diagram for StepCore::Controller:
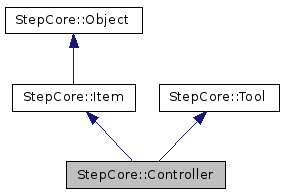
Public Member Functions | |
Controller (Vector2d position=Vector2d::Zero(), Vector2d size=Vector2d(200, 60)) | |
const QString & | decreaseShortcut () const |
const QString & | increaseShortcut () const |
double | increment () const |
int | index () const |
bool | isValid () const |
const Vector2d & | limits () const |
Object * | object () const |
const Vector2d & | position () const |
QString | property () const |
const MetaProperty * | propertyPtr () const |
void | setDecreaseShortcut (const QString &decreaseShortcut) |
void | setIncreaseShortcut (const QString &increaseShortcut) |
void | setIncrement (double increment) |
void | setIndex (int index) |
void | setLimits (const Vector2d &limits) |
void | setObject (Object *object) |
void | setPosition (const Vector2d &position) |
void | setProperty (const QString &property) |
void | setSize (const Vector2d &size) |
void | setValue (double value) |
void | setValue (double value, bool *ok) |
const Vector2d & | size () const |
QString | units () const |
double | value () const |
double | value (bool *ok) const |
![]() | |
Item (const QString &name=QString()) | |
Item (const Item &item) | |
virtual | ~Item () |
Color | color () const |
void | deleteObjectErrors () |
ItemGroup * | group () const |
ObjectErrors * | objectErrors () |
Item & | operator= (const Item &item) |
void | setColor (Color color) |
virtual void | setGroup (ItemGroup *group) |
virtual void | setWorld (World *world) |
ObjectErrors * | tryGetObjectErrors () const |
World * | world () const |
virtual void | worldItemRemoved (Item *item STEPCORE_UNUSED) |
![]() | |
Object (const QString &name=QString()) | |
virtual | ~Object () |
const QString & | name () const |
void | setName (const QString &name) |
![]() | |
virtual | ~Tool () |
Protected Attributes | |
QString | _decreaseShortcut |
QString | _increaseShortcut |
double | _increment |
int | _index |
Vector2d | _limits |
Object * | _object |
Vector2d | _position |
QString | _property |
Vector2d | _size |
![]() | |
QString | _name |
Additional Inherited Members | |
![]() | |
virtual ObjectErrors * | createObjectErrors () |
Detailed Description
Controller item to control properties of other objects.
Actual displaying of the Controller and its user interaction should be implemented by application
Constructor & Destructor Documentation
|
explicit |
Constructs Controller.
Member Function Documentation
|
inline |
|
inline |
|
inline |
|
inline |
bool StepCore::Controller::isValid | ( | ) | const |
|
inline |
|
inline |
|
inline |
Get position of the Controller.
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
Set position of the Controller.
|
inline |
|
inline |
Set size of the Controller.
|
inline |
void StepCore::Controller::setValue | ( | double | value, |
bool * | ok = 0 |
||
) |
|
inline |
Get size of the Controller.
QString StepCore::Controller::units | ( | ) | const |
|
inline |
double StepCore::Controller::value | ( | bool * | ok | ) | const |
Member Data Documentation
The documentation for this class was generated from the following files:
This file is part of the KDE documentation.
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:43:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:43:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.