step/stepcore
StepCore::Graph Class Reference
#include <tool.h>
Inheritance diagram for StepCore::Graph:
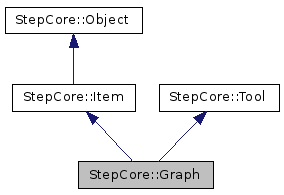
Public Member Functions | |
Graph (Vector2d position=Vector2d::Zero(), Vector2d size=Vector2d(400, 300)) | |
bool | autoLimitsX () const |
bool | autoLimitsY () const |
void | clearPoints () |
Vector2d | currentValue () const |
Vector2d | currentValue (bool *ok) const |
int | indexX () const |
int | indexY () const |
bool | isValid () const |
bool | isValidX () const |
bool | isValidY () const |
const Vector2d & | limitsX () const |
const Vector2d & | limitsY () const |
Object * | objectX () const |
Object * | objectY () const |
const Vector2dList & | points () const |
const Vector2d & | position () const |
QString | propertyX () const |
const MetaProperty * | propertyXPtr () const |
QString | propertyY () const |
const MetaProperty * | propertyYPtr () const |
Vector2d | recordPoint (bool *ok=0) |
void | setAutoLimitsX (bool autoLimitsX) |
void | setAutoLimitsY (bool autoLimitsY) |
void | setIndexX (int indexX) |
void | setIndexY (int indexY) |
void | setLimitsX (const Vector2d &limitsX) |
void | setLimitsY (const Vector2d &limitsY) |
void | setObjectX (Object *objectX) |
void | setObjectY (Object *objectY) |
void | setPoints (const Vector2dList &points) |
void | setPosition (const Vector2d &position) |
void | setPropertyX (const QString &propertyX) |
void | setPropertyY (const QString &propertyY) |
void | setShowLines (bool showLines) |
void | setShowPoints (bool showPoints) |
void | setSize (const Vector2d &size) |
bool | showLines () const |
bool | showPoints () const |
const Vector2d & | size () const |
QString | unitsX () const |
QString | unitsY () const |
![]() | |
Item (const QString &name=QString()) | |
Item (const Item &item) | |
virtual | ~Item () |
Color | color () const |
void | deleteObjectErrors () |
ItemGroup * | group () const |
ObjectErrors * | objectErrors () |
Item & | operator= (const Item &item) |
void | setColor (Color color) |
virtual void | setGroup (ItemGroup *group) |
virtual void | setWorld (World *world) |
ObjectErrors * | tryGetObjectErrors () const |
World * | world () const |
virtual void | worldItemRemoved (Item *item STEPCORE_UNUSED) |
![]() | |
Object (const QString &name=QString()) | |
virtual | ~Object () |
const QString & | name () const |
void | setName (const QString &name) |
![]() | |
virtual | ~Tool () |
Protected Attributes | |
bool | _autoLimitsX |
bool | _autoLimitsY |
int | _indexX |
int | _indexY |
Vector2d | _limitsX |
Vector2d | _limitsY |
Object * | _objectX |
Object * | _objectY |
Vector2dList | _points |
Vector2d | _position |
QString | _propertyX |
QString | _propertyY |
bool | _showLines |
bool | _showPoints |
Vector2d | _size |
![]() | |
QString | _name |
Additional Inherited Members | |
![]() | |
virtual ObjectErrors * | createObjectErrors () |
Detailed Description
Constructor & Destructor Documentation
Member Function Documentation
|
inline |
|
inline |
|
inline |
|
inline |
Vector2d StepCore::Graph::currentValue | ( | bool * | ok | ) | const |
|
inline |
|
inline |
|
inline |
bool StepCore::Graph::isValidX | ( | ) | const |
bool StepCore::Graph::isValidY | ( | ) | const |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
Vector2d StepCore::Graph::recordPoint | ( | bool * | ok = 0 | ) |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
|
inline |
QString StepCore::Graph::unitsX | ( | ) | const |
QString StepCore::Graph::unitsY | ( | ) | const |
Member Data Documentation
|
protected |
The documentation for this class was generated from the following files:
This file is part of the KDE documentation.
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:43:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:43:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.