step/stepcore
#include <softbody.h>
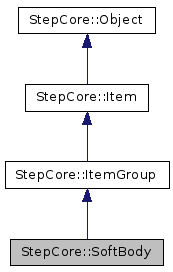
Public Member Functions | |
SoftBody () | |
Vector2d | acceleration () const |
void | addItems (const ItemList &items) |
double | angularAcceleration () const |
double | angularMomentum () const |
double | angularVelocity () const |
QString | borderParticleNames () const |
const SoftBodyParticleList & | borderParticles () |
ItemList | createSoftBodyItems (const Vector2d &position, const Vector2d &size, const Vector2i &split, double bodyMass, double youngModulus, double bodyDamping) |
Vector2d | force () const |
double | inertia () const |
double | mass () const |
Vector2d | position () const |
void | setAngularMomentum (double angularMomentum) |
void | setAngularVelocity (double angularVelocity) |
void | setBorderParticleNames (const QString &borderParticleNames) |
void | setPosition (const Vector2d position) |
void | setShowInternalItems (bool showInternalItems) |
void | setVelocity (const Vector2d velocity) |
void | setWorld (World *world) |
bool | showInternalItems () const |
double | torque () const |
Vector2d | velocity () const |
void | worldItemRemoved (Item *item) |
![]() | |
ItemGroup (const QString &name=QString()) | |
ItemGroup (const ItemGroup &group) | |
~ItemGroup () | |
virtual void | addItem (Item *item) |
ItemList | allItems () const |
void | allItems (ItemList *items) const |
Item * | childItem (int index) const |
Item * | childItem (const QString &name) const |
int | childItemCount () const |
int | childItemIndex (const Item *item) const |
void | clear () |
virtual void | deleteItem (Item *item) |
Item * | item (const QString &name) const |
const ItemList & | items () const |
ItemGroup & | operator= (const ItemGroup &group) |
virtual void | removeItem (Item *item) |
void | setWorld (World *world) |
void | worldItemRemoved (Item *item) |
![]() | |
Item (const QString &name=QString()) | |
Item (const Item &item) | |
virtual | ~Item () |
Color | color () const |
void | deleteObjectErrors () |
ItemGroup * | group () const |
ObjectErrors * | objectErrors () |
Item & | operator= (const Item &item) |
void | setColor (Color color) |
virtual void | setGroup (ItemGroup *group) |
ObjectErrors * | tryGetObjectErrors () const |
World * | world () const |
virtual void | worldItemRemoved (Item *item STEPCORE_UNUSED) |
![]() | |
Object (const QString &name=QString()) | |
virtual | ~Object () |
const QString & | name () const |
void | setName (const QString &name) |
Protected Attributes | |
QString | _borderParticleNames |
SoftBodyParticleList | _borderParticles |
bool | _showInternalItems |
![]() | |
QString | _name |
Additional Inherited Members | |
![]() | |
virtual ObjectErrors * | createObjectErrors () |
Detailed Description
SoftBody - a group of several SoftBodyParticle and SoftBodySprings.
Definition at line 65 of file softbody.h.
Constructor & Destructor Documentation
|
inline |
Constructs a SoftBody.
Definition at line 71 of file softbody.h.
Member Function Documentation
|
inline |
Get acceleration of the center of mass.
Definition at line 110 of file softbody.h.
void StepCore::SoftBody::addItems | ( | const ItemList & | items | ) |
Adds all items to ItemGroup.
Definition at line 149 of file softbody.cc.
|
inline |
Get angular acceleration of the body.
Definition at line 113 of file softbody.h.
double StepCore::SoftBody::angularMomentum | ( | ) | const |
Get the angular momentum of the body.
Definition at line 239 of file softbody.cc.
double StepCore::SoftBody::angularVelocity | ( | ) | const |
Get the angular velicity of the body.
Definition at line 256 of file softbody.cc.
QString StepCore::SoftBody::borderParticleNames | ( | ) | const |
Definition at line 327 of file softbody.cc.
const SoftBodyParticleList & StepCore::SoftBody::borderParticles | ( | ) |
Get ordered list of particles on the border of the body.
Definition at line 311 of file softbody.cc.
ItemList StepCore::SoftBody::createSoftBodyItems | ( | const Vector2d & | position, |
const Vector2d & | size, | ||
const Vector2i & | split, | ||
double | bodyMass, | ||
double | youngModulus, | ||
double | bodyDamping | ||
) |
Creates paricles and springs inside soft body.
- Parameters
-
position Position of the center of the body size Size of the edge of the body split Split count of the edge of the body bodyMass Total mass of the body youngModulus Young's modulus of the body bodyDamping Damping of the body
- Returns
- List of particles and springs
Vector2d StepCore::SoftBody::force | ( | ) | const |
Get the force acting on the body.
Definition at line 282 of file softbody.cc.
double StepCore::SoftBody::inertia | ( | ) | const |
Get the inrtia of the body.
Definition at line 224 of file softbody.cc.
double StepCore::SoftBody::mass | ( | ) | const |
Get total body mass.
Definition at line 157 of file softbody.cc.
Vector2d StepCore::SoftBody::position | ( | ) | const |
Get the position of the center of mass.
Definition at line 171 of file softbody.cc.
void StepCore::SoftBody::setAngularMomentum | ( | double | angularMomentum | ) |
Set the angular momentum of the body.
Definition at line 277 of file softbody.cc.
void StepCore::SoftBody::setAngularVelocity | ( | double | angularVelocity | ) |
Set the angular velicity of the body.
Definition at line 261 of file softbody.cc.
void StepCore::SoftBody::setBorderParticleNames | ( | const QString & | borderParticleNames | ) |
Definition at line 338 of file softbody.cc.
void StepCore::SoftBody::setPosition | ( | const Vector2d | position | ) |
Set the position of the center of mass.
Definition at line 185 of file softbody.cc.
|
inline |
Set status of drawing of internal items.
Definition at line 129 of file softbody.h.
void StepCore::SoftBody::setVelocity | ( | const Vector2d | velocity | ) |
Set the velocity of the center of mass.
Definition at line 212 of file softbody.cc.
|
virtual |
Set/change pointer to World in which this object lives.
Reimplemented from StepCore::Item.
Definition at line 356 of file softbody.cc.
|
inline |
Get status of drawing of internal items.
Definition at line 127 of file softbody.h.
double StepCore::SoftBody::torque | ( | ) | const |
Get the torque acting on the body.
Definition at line 296 of file softbody.cc.
Vector2d StepCore::SoftBody::velocity | ( | ) | const |
Get the velocity of the center of mass.
Definition at line 197 of file softbody.cc.
void StepCore::SoftBody::worldItemRemoved | ( | Item * | item | ) |
Definition at line 344 of file softbody.cc.
Member Data Documentation
|
protected |
Definition at line 143 of file softbody.h.
|
protected |
Definition at line 142 of file softbody.h.
|
protected |
Definition at line 144 of file softbody.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:43:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.