KDECore
#include <k3socketdevice.h>
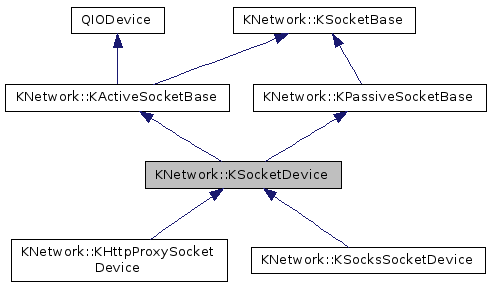
Public Types | |
enum | Capabilities { CanConnectString = 0x01, CanBindString = 0x02, CanNotBind = 0x04, CanNotListen = 0x08, CanMulticast = 0x10, CanNotUseDatagrams = 0x20 } |
![]() | |
enum | SocketError { NoError = 0, LookupFailure, AddressInUse, AlreadyCreated, AlreadyBound, AlreadyConnected, NotConnected, NotBound, NotCreated, WouldBlock, ConnectionRefused, ConnectionTimedOut, InProgress, NetFailure, NotSupported, Timeout, UnknownError, RemotelyDisconnected } |
enum | SocketOptions { Blocking = 0x01, AddressReuseable = 0x02, IPv6Only = 0x04, Keepalive = 0x08, Broadcast = 0x10, NoDelay = 0x20 } |
Public Member Functions | |
KSocketDevice (const KSocketBase *=0L, QObject *objparent=0L) | |
KSocketDevice (int fd, OpenMode mode=ReadWrite) | |
KSocketDevice (QObject *parent) | |
virtual | ~KSocketDevice () |
virtual KSocketDevice * | accept () |
virtual bool | bind (const KResolverEntry &address) |
virtual qint64 | bytesAvailable () const |
virtual int | capabilities () const |
virtual void | close () |
virtual bool | connect (const KResolverEntry &address, OpenMode mode=ReadWrite) |
virtual bool | create (int family, int type, int protocol) |
bool | create (const KResolverEntry &address) |
virtual bool | disconnect () |
QSocketNotifier * | exceptionNotifier () const |
virtual KSocketAddress | externalAddress () const |
virtual bool | flush () |
virtual bool | listen (int backlog=5) |
virtual KSocketAddress | localAddress () const |
virtual qint64 | peekData (char *data, qint64 maxlen, KSocketAddress *from=0L) |
virtual KSocketAddress | peerAddress () const |
virtual bool | poll (bool *input, bool *output, bool *exception=0L, int timeout=-1, bool *timedout=0L) |
bool | poll (int timeout=-1, bool *timedout=0L) |
virtual qint64 | readData (char *data, qint64 maxlen, KSocketAddress *from=0L) |
QSocketNotifier * | readNotifier () const |
virtual bool | setSocketOptions (int opts) |
int | socket () const |
virtual qint64 | waitForMore (int msecs, bool *timeout=0L) |
virtual qint64 | writeData (const char *data, qint64 len, const KSocketAddress *to=0L) |
QSocketNotifier * | writeNotifier () const |
![]() | |
KActiveSocketBase (QObject *parent) | |
virtual | ~KActiveSocketBase () |
virtual bool | atEnd () const |
QString | errorString () const |
virtual bool | isSequential () const |
virtual bool | open (OpenMode mode) |
qint64 | peek (char *data, qint64 maxlen) |
qint64 | peek (char *data, qint64 maxlen, KSocketAddress &from) |
virtual qint64 | pos () const |
qint64 | read (char *data, qint64 maxlen) |
QByteArray | read (qint64 len) |
qint64 | read (char *data, qint64 maxlen, KSocketAddress &from) |
virtual bool | seek (qint64) |
virtual void | setSocketDevice (KSocketDevice *device) |
virtual qint64 | size () const |
void | ungetChar (char) |
qint64 | write (const char *data, qint64 len) |
qint64 | write (const QByteArray &data) |
qint64 | write (const char *data, qint64 len, const KSocketAddress &to) |
![]() | |
KSocketBase () | |
virtual | ~KSocketBase () |
bool | addressReuseable () const |
bool | blocking () const |
bool | broadcast () const |
SocketError | error () const |
QString | errorString () const |
bool | isIPv6Only () const |
QMutex * | mutex () const |
bool | noDelay () const |
virtual bool | setAddressReuseable (bool enable) |
virtual bool | setBlocking (bool enable) |
virtual bool | setBroadcast (bool enable) |
virtual bool | setIPv6Only (bool enable) |
virtual bool | setNoDelay (bool enable) |
int | setRequestedCapabilities (int add, int remove=0) |
KSocketDevice * | socketDevice () const |
![]() | |
KPassiveSocketBase () | |
virtual | ~KPassiveSocketBase () |
Static Public Member Functions | |
static void | addNewImpl (KSocketDeviceFactoryBase *factory, int capabilities) |
static KSocketDevice * | createDefault (KSocketBase *parent) |
static KSocketDevice * | createDefault (KSocketBase *parent, int capabilities) |
static KSocketDeviceFactoryBase * | setDefaultImpl (KSocketDeviceFactoryBase *factory) |
![]() | |
static QString | errorString (SocketError code) |
static bool | isFatalError (int code) |
Protected Member Functions | |
KSocketDevice (bool, const KSocketBase *parent=0L) | |
virtual QSocketNotifier * | createNotifier (QSocketNotifier::Type type) const |
![]() | |
virtual qint64 | readData (char *data, qint64 len) |
void | resetError () |
void | setError (SocketError error) |
virtual qint64 | writeData (const char *data, qint64 len) |
![]() | |
bool | hasDevice () const |
void | resetError () |
void | setError (SocketError error) |
virtual int | socketOptions () const |
Protected Attributes | |
int | m_sockfd |
Detailed Description
Low-level socket functionality.
This class provides low-level socket functionality.
Most users will prefer "cooked" interfaces like those of KStreamSocket or KServerSocket.
Descended classes from this one provide some other kinds of socket functionality, like proxying or specific socket types.
- Deprecated:
- Use KSocketFactory or KLocalSocket instead
Definition at line 51 of file k3socketdevice.h.
Member Enumeration Documentation
Capabilities for the socket implementation.
KSocketDevice-derived classes can implement certain capabilities that are not available in the default class. These capabilities are described by these flags. The default KSocketDevice class has none of these capabilities.
For the negative capabilities (inabilities, the CanNot* forms), when a capability is not present, the implementation will default to the original behaviour.
Enumerator | |
---|---|
CanConnectString |
Can connect to hostnames. If this flag is present, the string form of connect() can be used. |
CanBindString |
Can bind to hostnames. If this flag is present, the string form of bind() can be used |
CanNotBind |
Can not bind. If this flag is present, this implementation cannot bind |
CanNotListen |
Can not listen. If this flag is present, this implementation cannot listen |
CanMulticast |
Can send multicast as well as join/leave multicast groups. |
CanNotUseDatagrams |
Can not use datagrams. Note that this implies multicast capability not being available either. |
Definition at line 64 of file k3socketdevice.h.
Constructor & Destructor Documentation
|
explicit |
Default constructor.
The parameter is used to specify which socket this object is used as a device for.
Definition at line 87 of file k3socketdevice.cpp.
|
explicit |
Constructs a new object around an already-open socket.
Note: you should write programs that create sockets through the classes whenever possible.
Definition at line 96 of file k3socketdevice.cpp.
KSocketDevice::KSocketDevice | ( | QObject * | parent | ) |
QObject constructor.
Definition at line 106 of file k3socketdevice.cpp.
|
virtual |
|
explicitprotected |
Special constructor.
This constructor will cause the internal socket device NOT to be set. Use this if your socket device class takes another underlying socket device.
- Parameters
-
parent the parent, if any
Definition at line 112 of file k3socketdevice.cpp.
Member Function Documentation
|
virtual |
Accepts a new incoming connection.
Note: this function returns a socket of type KSocketDevice.
Implements KNetwork::KPassiveSocketBase.
Reimplemented in KNetwork::KSocksSocketDevice.
Definition at line 382 of file k3socketdevice.cpp.
|
static |
Adds a factory of KSocketDevice objects to the list, along with its capabilities flag.
Definition at line 915 of file k3socketdevice.cpp.
|
virtual |
Binds this socket to the given address.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KSocksSocketDevice.
Definition at line 293 of file k3socketdevice.cpp.
|
virtual |
Returns the number of bytes available for reading without blocking.
Definition at line 439 of file k3socketdevice.cpp.
|
virtual |
Returns the set of capabilities this socket class implements.
The set of capabilities is defined as an OR-ed mask of Capabilities bits.
The default implementation is guaranteed to always return 0. That is, derived implementations always return bits where they differ from the system standard sockets.
Reimplemented in KNetwork::KHttpProxySocketDevice, and KNetwork::KSocksSocketDevice.
Definition at line 132 of file k3socketdevice.cpp.
|
virtual |
Closes the socket.
Reimplemented from QIODevice.
Use this function to close the socket this object is holding open.
Implements KNetwork::KPassiveSocketBase.
Reimplemented in KNetwork::KHttpProxySocketDevice.
Definition at line 232 of file k3socketdevice.cpp.
|
virtual |
Connect to a remote host.
Implements KNetwork::KActiveSocketBase.
Definition at line 345 of file k3socketdevice.cpp.
|
virtual |
Creates a socket but don't connect or bind anywhere.
This function is the equivalent of the system call socket(2).
Definition at line 261 of file k3socketdevice.cpp.
bool KSocketDevice::create | ( | const KResolverEntry & | address | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Creates a socket but don't connect or bind anywhere.
Definition at line 288 of file k3socketdevice.cpp.
|
static |
Creates a new default KSocketDevice object given the parent object.
The capabilities flag indicates the desired capabilities the object being created should possess. Those capabilities are not guaranteed: if no factory can provide such an object, a default object will be created.
- Parameters
-
parent the KSocketBase parent
Definition at line 877 of file k3socketdevice.cpp.
|
static |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.This will create an object only if the requested capabilities match.
- Parameters
-
parent the parent capabilities the requested capabilities
Definition at line 890 of file k3socketdevice.cpp.
|
protectedvirtual |
Creates a socket notifier of the given type.
This function is called by readNotifier(), writeNotifier() and exceptionNotifier() when they need to create a socket notifier (i.e., the first call to those functions after the socket is open). After that call, those functions cache the socket notifier and will not need to call this function again.
Reimplement this function in your derived class if your socket type requires a different kind of QSocketNotifier. The return value should be deleteable with delete. (close() deletes them).
- Parameters
-
type the socket notifier type
Definition at line 829 of file k3socketdevice.cpp.
|
virtual |
Disconnects this socket.
Implements KNetwork::KActiveSocketBase.
Definition at line 406 of file k3socketdevice.cpp.
QSocketNotifier * KSocketDevice::exceptionNotifier | ( | ) | const |
Returns a socket notifier for exceptional events on this socket.
The is created only when requested.
This function might return NULL.
Definition at line 683 of file k3socketdevice.cpp.
|
virtual |
Returns this socket's externally visible local address.
If this socket has a local address visible externally different from the normal local address (as returned by localAddress()), then return it.
Certain implementations will use proxies and thus have externally visible addresses different from the local socket values. The default implementation returns the same value as localAddress().
- Note
- This function may return an empty KSocketAddress. In that case, the externally visible address could/can not be determined.
Implements KNetwork::KPassiveSocketBase.
Reimplemented in KNetwork::KSocksSocketDevice, and KNetwork::KHttpProxySocketDevice.
Definition at line 640 of file k3socketdevice.cpp.
|
virtual |
This call is not supported on sockets.
Reimplemented from QIODevice.
Definition at line 256 of file k3socketdevice.cpp.
|
virtual |
Puts this socket into listening mode.
Implements KNetwork::KPassiveSocketBase.
Reimplemented in KNetwork::KSocksSocketDevice.
Definition at line 324 of file k3socketdevice.cpp.
|
virtual |
Returns this socket's local address.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KSocksSocketDevice.
Definition at line 568 of file k3socketdevice.cpp.
|
virtual |
Peeks the data in the socket and the source address.
Implements KNetwork::KActiveSocketBase.
Definition at line 515 of file k3socketdevice.cpp.
|
virtual |
Returns this socket's peer address.
If this implementation does proxying of some sort, this is the real external address, not the proxy's address.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KSocksSocketDevice, and KNetwork::KHttpProxySocketDevice.
Definition at line 604 of file k3socketdevice.cpp.
|
virtual |
Executes a poll in the socket, via select(2) or poll(2).
The events polled are returned in the parameters pointers. Set any of them to NULL to disable polling of that event.
On exit, input
, output
and exception
will contain true if an event of that kind is waiting on the socket or false if not. If a timeout occurred, set timedout
to true (all other parameters are necessarily set to false).
- Parameters
-
input if set, turns on polling for input events output if set, turns on polling for output events exception if set, turns on polling for exceptional events timeout the time in milliseconds to wait for an event; 0 for no wait and any negative value to wait forever timedout on exit, will contain true if the polling timed out
- Returns
- true if the poll call succeeded and false if an error occurred
Reimplemented in KNetwork::KSocksSocketDevice.
Definition at line 701 of file k3socketdevice.cpp.
Shorter version to poll for any events in a socket.
This call polls for input, output and exceptional events in a socket but does not return their states. This is useful if you need to wait for any event, but don't need to know which; or for timeouts.
- Parameters
-
timeout the time in milliseconds to wait for an event; 0 for no wait and any negative value to wait forever timedout on exit, will contain true if the polling timed out
- Returns
- true if the poll call succeeded and false if an error occurred
Definition at line 823 of file k3socketdevice.cpp.
|
virtual |
Reads data and the source address from this socket.
Implements KNetwork::KActiveSocketBase.
Definition at line 494 of file k3socketdevice.cpp.
QSocketNotifier * KSocketDevice::readNotifier | ( | ) | const |
Returns a socket notifier for input on this socket.
The notifier is created only when requested. Whether it is enabled or not depends on the implementation.
This function might return NULL.
Definition at line 647 of file k3socketdevice.cpp.
|
static |
Sets the default KSocketDevice implementation to use and return the old factory.
- Parameters
-
factory the factory object for the implementation
Definition at line 907 of file k3socketdevice.cpp.
|
virtual |
This implementation sets the options on the socket.
Reimplemented from KNetwork::KSocketBase.
Definition at line 137 of file k3socketdevice.cpp.
int KSocketDevice::socket | ( | ) | const |
Returns the file descriptor for this socket.
Definition at line 127 of file k3socketdevice.cpp.
Waits up to msecs
for more data to be available on this socket.
This function is a wrapper against poll(). This function will wait for any read events.
Implements KNetwork::KActiveSocketBase.
Definition at line 451 of file k3socketdevice.cpp.
|
virtual |
Writes the given data to the given destination address.
Implements KNetwork::KActiveSocketBase.
Definition at line 536 of file k3socketdevice.cpp.
QSocketNotifier * KSocketDevice::writeNotifier | ( | ) | const |
Returns a socket notifier for output on this socket.
The is created only when requested.
This function might return NULL.
Definition at line 665 of file k3socketdevice.cpp.
Member Data Documentation
|
protected |
The socket file descriptor.
It is used throughout the implementation and subclasses.
Definition at line 96 of file k3socketdevice.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:12 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.