KDECore
#include <k3streamsocket.h>
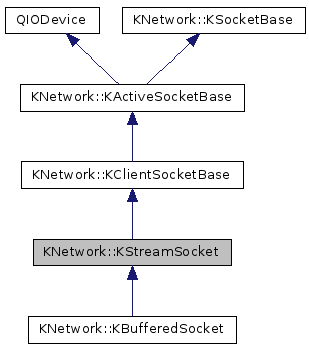
Signals | |
void | timedOut () |
![]() | |
void | aboutToConnect (const KNetwork::KResolverEntry &remote, bool &skip) |
void | bound (const KNetwork::KResolverEntry &local) |
void | closed () |
void | connected (const KNetwork::KResolverEntry &remote) |
void | gotError (int code) |
void | hostFound () |
void | readyWrite () |
void | stateChanged (int newstate) |
Public Member Functions | |
KStreamSocket (const QString &node=QString(), const QString &service=QString(), QObject *parent=0L) | |
virtual | ~KStreamSocket () |
virtual bool | bind (const QString &node=QString(), const QString &service=QString()) |
virtual bool | bind (const KResolverEntry &entry) |
virtual bool | connect (const QString &node=QString(), const QString &service=QString(), OpenMode mode=ReadWrite) |
virtual bool | connect (const KResolverEntry &entry, OpenMode mode=ReadWrite) |
int | remainingTimeout () const |
void | setTimeout (int msecs) |
int | timeout () const |
![]() | |
KClientSocketBase (QObject *parent) | |
virtual | ~KClientSocketBase () |
virtual qint64 | bytesAvailable () const |
virtual void | close () |
virtual bool | disconnect () |
bool | emitsReadyRead () const |
bool | emitsReadyWrite () const |
virtual void | enableRead (bool enable) |
virtual void | enableWrite (bool enable) |
virtual bool | flush () |
virtual KSocketAddress | localAddress () const |
KResolver & | localResolver () const |
const KResolverResults & | localResults () const |
virtual bool | lookup () |
virtual bool | open (OpenMode mode) |
virtual KSocketAddress | peerAddress () const |
KResolver & | peerResolver () const |
const KResolverResults & | peerResults () const |
void | setFamily (int families) |
void | setResolutionEnabled (bool enable) |
SocketState | state () const |
virtual qint64 | waitForMore (int msecs, bool *timeout=0L) |
![]() | |
KActiveSocketBase (QObject *parent) | |
virtual | ~KActiveSocketBase () |
virtual bool | atEnd () const |
QString | errorString () const |
virtual bool | isSequential () const |
qint64 | peek (char *data, qint64 maxlen) |
qint64 | peek (char *data, qint64 maxlen, KSocketAddress &from) |
virtual qint64 | pos () const |
qint64 | read (char *data, qint64 maxlen) |
QByteArray | read (qint64 len) |
qint64 | read (char *data, qint64 maxlen, KSocketAddress &from) |
virtual bool | seek (qint64) |
virtual void | setSocketDevice (KSocketDevice *device) |
virtual qint64 | size () const |
void | ungetChar (char) |
qint64 | write (const char *data, qint64 len) |
qint64 | write (const QByteArray &data) |
qint64 | write (const char *data, qint64 len, const KSocketAddress &to) |
![]() | |
KSocketBase () | |
virtual | ~KSocketBase () |
bool | addressReuseable () const |
bool | blocking () const |
bool | broadcast () const |
SocketError | error () const |
QString | errorString () const |
bool | isIPv6Only () const |
QMutex * | mutex () const |
bool | noDelay () const |
virtual bool | setAddressReuseable (bool enable) |
virtual bool | setBlocking (bool enable) |
virtual bool | setBroadcast (bool enable) |
virtual bool | setIPv6Only (bool enable) |
virtual bool | setNoDelay (bool enable) |
int | setRequestedCapabilities (int add, int remove=0) |
KSocketDevice * | socketDevice () const |
Detailed Description
Simple stream socket.
This class provides functionality to creating unbuffered, stream sockets. In the case of Internet (IP) sockets, this class creates and uses TCP/IP sockets.
Objects of this class start, by default, on non-blocking mode. Call setBlocking if you wish to change that.
KStreamSocket objects are thread-safe and can be used in auxiliary threads (i.e., not the thread in which the Qt event loop runs in). Note that KBufferedSocket cannot be used reliably in an auxiliary thread.
Sample usage:
Here's another sample, showing asynchronous operation:
- Version
- 0.9
- Deprecated:
- Use KSocketFactory or KLocalSocket instead
Definition at line 98 of file k3streamsocket.h.
Constructor & Destructor Documentation
|
explicit |
Default constructor.
- Parameters
-
node destination host service destination service to connect to parent the parent QObject object
Definition at line 55 of file k3streamsocket.cpp.
|
virtual |
Member Function Documentation
|
virtual |
Binds this socket to the given nodename and service, or use the default ones if none are given.
In order to bind to a service and allow the operating system to choose the interface, set node
to QString().
Reimplemented from KClientSocketBase.
Upon successful binding, the bound() signal will be emitted. If an error is found, the gotError() signal will be emitted.
- Note
- Due to the internals of the name lookup and binding mechanism, some (if not most) implementations of this function do not actually bind the socket until the connection is requested (see connect()). They only set the values for future reference.
This function returns true on success.
- Parameters
-
node the nodename service the service
Implements KNetwork::KClientSocketBase.
Definition at line 98 of file k3streamsocket.cpp.
|
virtual |
Reimplemented from KClientSocketBase.
Connect this socket to this specific address.
Unlike bind(const QString&, const QString&) above, this function really does bind the socket. No lookup is performed. The bound() signal will be emitted.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 110 of file k3streamsocket.cpp.
|
virtual |
Reimplemented from KClientSocketBase.
Attempts to connect to the these hostname and service, or use the default ones if none are given. If a connection attempt is already in progress, check on its state and set the error status (NoError, meaning the connection is completed, or InProgress).
If the blocking mode for this object is on, this function will only return when all the resolved peer addresses have been tried or when a connection is established.
Upon successfully connecting, the connected() signal will be emitted. If an error is found, the gotError() signal will be emitted.
This function also implements timeout handling.
- Parameters
-
node the remote node to connect to service the service on the remote node to connect to mode mode to operate this socket in
Implements KNetwork::KClientSocketBase.
Definition at line 115 of file k3streamsocket.cpp.
|
virtual |
Unshadowing from KClientSocketBase.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 204 of file k3streamsocket.cpp.
int KStreamSocket::remainingTimeout | ( | ) | const |
Retrieves the remaining timeout time (in milliseconds).
This value equals timeout() if there's no connection in progress.
Definition at line 80 of file k3streamsocket.cpp.
void KStreamSocket::setTimeout | ( | int | msecs | ) |
Sets the timeout value.
Setting this value while a connection attempt is in progress will reset the timer.
Please note that the timeout value is valid for the connection attempt only. No other operations are timed against this value – including the name lookup associated.
- Parameters
-
msecs the timeout value in milliseconds
Definition at line 90 of file k3streamsocket.cpp.
|
signal |
This signal is emitted when a connection timeout occurs.
int KStreamSocket::timeout | ( | ) | const |
Retrieves the timeout value (in milliseconds).
Definition at line 75 of file k3streamsocket.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:12 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.