KDECore
#include <k3clientsocketbase.h>
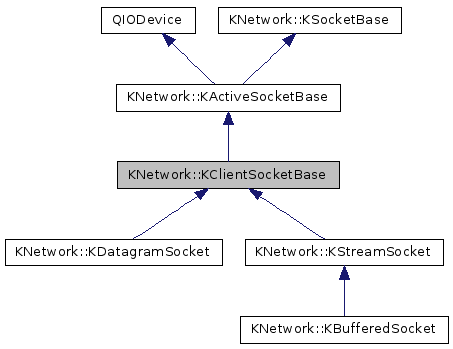
Public Types | |
enum | SocketState { Idle, HostLookup, HostFound, Bound, Connecting, Open, Closing, Unconnected = Bound, Connected = Open, Connection = Open } |
![]() | |
enum | SocketError { NoError = 0, LookupFailure, AddressInUse, AlreadyCreated, AlreadyBound, AlreadyConnected, NotConnected, NotBound, NotCreated, WouldBlock, ConnectionRefused, ConnectionTimedOut, InProgress, NetFailure, NotSupported, Timeout, UnknownError, RemotelyDisconnected } |
enum | SocketOptions { Blocking = 0x01, AddressReuseable = 0x02, IPv6Only = 0x04, Keepalive = 0x08, Broadcast = 0x10, NoDelay = 0x20 } |
Signals | |
void | aboutToConnect (const KNetwork::KResolverEntry &remote, bool &skip) |
void | bound (const KNetwork::KResolverEntry &local) |
void | closed () |
void | connected (const KNetwork::KResolverEntry &remote) |
void | gotError (int code) |
void | hostFound () |
void | readyWrite () |
void | stateChanged (int newstate) |
Public Member Functions | |
KClientSocketBase (QObject *parent) | |
virtual | ~KClientSocketBase () |
virtual bool | bind (const QString &node=QString(), const QString &service=QString())=0 |
virtual bool | bind (const KResolverEntry &address) |
virtual qint64 | bytesAvailable () const |
virtual void | close () |
virtual bool | connect (const QString &node=QString(), const QString &service=QString(), OpenMode mode=ReadWrite)=0 |
virtual bool | connect (const KResolverEntry &address, OpenMode mode=ReadWrite) |
virtual bool | disconnect () |
bool | emitsReadyRead () const |
bool | emitsReadyWrite () const |
virtual void | enableRead (bool enable) |
virtual void | enableWrite (bool enable) |
virtual bool | flush () |
virtual KSocketAddress | localAddress () const |
KResolver & | localResolver () const |
const KResolverResults & | localResults () const |
virtual bool | lookup () |
virtual bool | open (OpenMode mode) |
virtual KSocketAddress | peerAddress () const |
KResolver & | peerResolver () const |
const KResolverResults & | peerResults () const |
void | setFamily (int families) |
void | setResolutionEnabled (bool enable) |
SocketState | state () const |
virtual qint64 | waitForMore (int msecs, bool *timeout=0L) |
![]() | |
KActiveSocketBase (QObject *parent) | |
virtual | ~KActiveSocketBase () |
virtual bool | atEnd () const |
QString | errorString () const |
virtual bool | isSequential () const |
qint64 | peek (char *data, qint64 maxlen) |
qint64 | peek (char *data, qint64 maxlen, KSocketAddress &from) |
virtual qint64 | pos () const |
qint64 | read (char *data, qint64 maxlen) |
QByteArray | read (qint64 len) |
qint64 | read (char *data, qint64 maxlen, KSocketAddress &from) |
virtual bool | seek (qint64) |
virtual void | setSocketDevice (KSocketDevice *device) |
virtual qint64 | size () const |
void | ungetChar (char) |
qint64 | write (const char *data, qint64 len) |
qint64 | write (const QByteArray &data) |
qint64 | write (const char *data, qint64 len, const KSocketAddress &to) |
![]() | |
KSocketBase () | |
virtual | ~KSocketBase () |
bool | addressReuseable () const |
bool | blocking () const |
bool | broadcast () const |
SocketError | error () const |
QString | errorString () const |
bool | isIPv6Only () const |
QMutex * | mutex () const |
bool | noDelay () const |
virtual bool | setAddressReuseable (bool enable) |
virtual bool | setBlocking (bool enable) |
virtual bool | setBroadcast (bool enable) |
virtual bool | setIPv6Only (bool enable) |
virtual bool | setNoDelay (bool enable) |
int | setRequestedCapabilities (int add, int remove=0) |
KSocketDevice * | socketDevice () const |
Protected Slots | |
virtual void | slotReadActivity () |
virtual void | slotWriteActivity () |
Protected Member Functions | |
void | copyError () |
virtual qint64 | peekData (char *data, qint64 maxlen, KSocketAddress *from) |
virtual qint64 | readData (char *data, qint64 maxlen, KSocketAddress *from) |
virtual bool | setSocketOptions (int opts) |
void | setState (SocketState state) |
virtual void | stateChanging (SocketState newState) |
virtual qint64 | writeData (const char *data, qint64 len, const KSocketAddress *to) |
![]() | |
virtual qint64 | readData (char *data, qint64 len) |
void | resetError () |
void | setError (SocketError error) |
virtual qint64 | writeData (const char *data, qint64 len) |
![]() | |
bool | hasDevice () const |
void | resetError () |
void | setError (SocketError error) |
virtual int | socketOptions () const |
Additional Inherited Members | |
![]() | |
static QString | errorString (SocketError code) |
static bool | isFatalError (int code) |
Detailed Description
Abstract client socket class.
This class provides the base functionality for client sockets, such as, and especially, name resolution and signals.
- Note
- This class is abstract. If you're looking for a normal, client socket class, see KStreamSocket and KBufferedSocket
- Deprecated:
- Use KSocketFactory or KLocalSocket instead
Definition at line 50 of file k3clientsocketbase.h.
Member Enumeration Documentation
Socket states.
These are the possible states for a KClientSocketBase:
- Idle: socket is not connected
- HostLookup: socket is doing host lookup prior to connecting
- HostFound: name lookup is complete
- Bound: the socket is locally bound
- Connecting: socket is attempting connection
- Open: socket is open
- Connected (=Open): socket is connected
- Connection (=Open): yet another name for a connected socket
- Closing: socket is shutting down
Whenever the socket state changes, the stateChanged(int) signal will be emitted.
Enumerator | |
---|---|
Idle | |
HostLookup | |
HostFound | |
Bound | |
Connecting | |
Open | |
Closing | |
Unconnected | |
Connected | |
Connection |
Definition at line 72 of file k3clientsocketbase.h.
Constructor & Destructor Documentation
KClientSocketBase::KClientSocketBase | ( | QObject * | parent | ) |
Default constructor.
- Parameters
-
parent the parent QObject object
Definition at line 52 of file k3clientsocketbase.cpp.
|
virtual |
Destructor.
Definition at line 60 of file k3clientsocketbase.cpp.
Member Function Documentation
|
signal |
This signal is emitted when the socket is about to connect to an address (but before doing so).
The skip
parameter can be used to make the loop skip this address. Its value is initially false: change it to true if you want to skip the current address (as given by remote
).
This function is also useful if one wants to reset the timeout.
- Parameters
-
remote the address we're about to connect to skip set to true if you want to skip this address
- Note
- if the connection is successful, the connected() signal will be emitted.
|
pure virtual |
Binds this socket to the given nodename and service, or use the default ones if none are given.
Upon successful binding, the bound() signal will be emitted. If an error is found, the gotError() signal will be emitted.
- Note
- Due to the internals of the name lookup and binding mechanism, some (if not most) implementations of this function do not actually bind the socket until the connection is requested (see connect()). They only set the values for future reference.
This function returns true on success.
- Parameters
-
node the nodename service the service
Implemented in KNetwork::KDatagramSocket, and KNetwork::KStreamSocket.
|
virtual |
Reimplemented from KSocketBase.
Connect this socket to this specific address.
Unlike bind(const QString&, const QString&) above, this function really does bind the socket. No lookup is performed. The bound() signal will be emitted.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KDatagramSocket, and KNetwork::KStreamSocket.
Definition at line 193 of file k3clientsocketbase.cpp.
|
signal |
This signal is emitted when the socket successfully binds to an address.
- Parameters
-
local the local address we bound to
|
virtual |
Returns the number of bytes available on this socket.
Reimplemented from KSocketBase.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 296 of file k3clientsocketbase.cpp.
|
virtual |
Closes the socket.
Reimplemented from QIODevice.
The closing of the socket causes the emission of the signal closed().
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 270 of file k3clientsocketbase.cpp.
|
signal |
This signal is emitted when the socket completes the closing/shut down process.
|
pure virtual |
Attempts to connect to a given hostname and service, or use the default ones if none are given.
If a connection attempt is already in progress, check on its state and set the error status (NoError or InProgress).
If the blocking mode for this object is on, this function will only return when all the resolved peer addresses have been tried or when a connection is established.
Upon successfully connecting, the connected() signal will be emitted. If an error is found, the gotError() signal will be emitted.
- Note for derived classes:
- Derived classes must implement this function. The implementation will set the parameters for the lookup (using the peer KResolver object) and call lookup() to start it.
- The implementation should use the hostFound() signal to be notified of the completion of the lookup process and then proceed to start the connection itself. Care should be taken regarding the value of blocking() flag.
- Parameters
-
node the nodename (host to connect to) service the service to connect to mode the mode to open the connection in
Implemented in KNetwork::KDatagramSocket, and KNetwork::KStreamSocket.
|
virtual |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KDatagramSocket, and KNetwork::KStreamSocket.
Definition at line 214 of file k3clientsocketbase.cpp.
|
signal |
This socket is emitted when the socket successfully connects to a remote address.
- Parameters
-
remote the remote address we did connect to
|
protected |
Convenience function to set this object's error code to match that of the socket device.
Definition at line 453 of file k3clientsocketbase.cpp.
|
virtual |
Disconnects the socket.
Note that not all socket types can disconnect.
Implements KNetwork::KActiveSocketBase.
Definition at line 248 of file k3clientsocketbase.cpp.
bool KClientSocketBase::emitsReadyRead | ( | ) | const |
Returns true if the readyRead signal is set to be emitted.
Definition at line 364 of file k3clientsocketbase.cpp.
bool KClientSocketBase::emitsReadyWrite | ( | ) | const |
Returns true if the readyWrite signal is set to be emitted.
Definition at line 379 of file k3clientsocketbase.cpp.
|
virtual |
Enables the emission of the readyRead signal.
By default, this signal is enabled.
- Parameters
-
enable whether to enable the signal
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 369 of file k3clientsocketbase.cpp.
|
virtual |
Enables the emission of the readyWrite signal.
By default, this signal is disabled.
- Parameters
-
enable whether to enable the signal
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 384 of file k3clientsocketbase.cpp.
|
virtual |
This call is not supported on unbuffered sockets.
Reimplemented from QIODevice.
Definition at line 290 of file k3clientsocketbase.cpp.
|
signal |
This signal is emitted when this object finds an error.
The code
parameter contains the error code that can also be found by calling error().
|
signal |
This signal is emitted when the lookup is successfully completed.
|
virtual |
Returns the local socket address.
Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Definition at line 354 of file k3clientsocketbase.cpp.
KResolver & KClientSocketBase::localResolver | ( | ) | const |
Returns the internal KResolver object used for looking up the local host name and service.
This can be used to set extra options to the lookup process other than the default values, as well as obtaining the error codes in case of lookup failure.
Definition at line 103 of file k3clientsocketbase.cpp.
const KResolverResults & KClientSocketBase::localResults | ( | ) | const |
Returns the internal list of resolved results for the local address.
Definition at line 108 of file k3clientsocketbase.cpp.
|
virtual |
Starts the lookup for peer and local hostnames as well as their services.
If the blocking mode for this object is on, this function will wait for the lookup results to be available (by calling the KResolver::wait() method on the resolver objects).
When the lookup is done, the signal hostFound() will be emitted (only once, even if we're doing a double lookup). If the lookup failed (for any of the two lookups) the gotError() signal will be emitted with the appropriate error condition (see KSocketBase::SocketError).
This function returns true on success and false on error. Note that this is not the lookup result!
Definition at line 133 of file k3clientsocketbase.cpp.
|
virtual |
Opens the socket.
Reimplemented from QIODevice.
You should not call this function; instead, use connect()
Reimplemented from KNetwork::KActiveSocketBase.
Definition at line 265 of file k3clientsocketbase.cpp.
|
protectedvirtual |
Peeks data from the socket.
Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 328 of file k3clientsocketbase.cpp.
|
virtual |
Returns the peer socket address.
Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Definition at line 359 of file k3clientsocketbase.cpp.
KResolver & KClientSocketBase::peerResolver | ( | ) | const |
Returns the internal KResolver object used for looking up the peer host name and service.
This can be used to set extra options to the lookup process other than the default values, as well as obtaining the error codes in case of lookup failure.
Definition at line 93 of file k3clientsocketbase.cpp.
const KResolverResults & KClientSocketBase::peerResults | ( | ) | const |
Returns the internal list of resolved results for the peer address.
Definition at line 98 of file k3clientsocketbase.cpp.
|
protectedvirtual |
Reads data from a socket.
Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 316 of file k3clientsocketbase.cpp.
|
signal |
This signal is emitted whenever the socket is ready for writing – i.e., whenever there's space available in the buffers to receive more data.
The subsequent write operation is guaranteed to be non-blocking.
You can toggle the emission of this signal with the enableWrite() function. This signal is by default disabled. You will want to disable this signal after the first reception, since it'll probably fire at every event loop.
void KClientSocketBase::setFamily | ( | int | families | ) |
Sets the allowed families for the resolutions.
- Parameters
-
families the families that we want/accept
- See also
- KResolver::SocketFamilies for possible values
Definition at line 127 of file k3clientsocketbase.cpp.
void KClientSocketBase::setResolutionEnabled | ( | bool | enable | ) |
Enables or disables name resolution.
If this flag is set to true, bind() and connect() operations will trigger name lookup operations (i.e., converting a hostname into its binary form). If the flag is set to false, those operations will instead try to convert a string representation of an address without attempting name resolution.
This is useful, for instance, when IP addresses are in their string representation (such as "1.2.3.4") or come from other sources like KSocketAddress.
- Parameters
-
enable whether to enable
Definition at line 113 of file k3clientsocketbase.cpp.
|
protectedvirtual |
Sets the socket options.
Reimplemented from KSocketBase.
Reimplemented from KNetwork::KSocketBase.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 77 of file k3clientsocketbase.cpp.
|
protected |
Sets the socket state to state
.
This function does not emit the stateChanged() signal.
Definition at line 71 of file k3clientsocketbase.cpp.
|
protectedvirtualslot |
This slot is connected to the read notifier's signal meaning the socket can read more data.
The default implementation only emits the readyRead signal.
Override if your class requires processing of incoming data.
Definition at line 394 of file k3clientsocketbase.cpp.
|
protectedvirtualslot |
This slot is connected to the write notifier's signal meaning the socket can write more data.
The default implementation only emits the readyWrite signal.
Override if your class writes data from another source (like a buffer).
Definition at line 400 of file k3clientsocketbase.cpp.
KClientSocketBase::SocketState KClientSocketBase::state | ( | ) | const |
Returns the current state for this socket.
- See also
- SocketState
Definition at line 66 of file k3clientsocketbase.cpp.
|
signal |
This signal is emitted whenever the socket state changes.
Note: do not delete this object inside the slot called by this signal.
- Parameters
-
newstate the new state of the socket object
|
protectedvirtual |
This function is called by setState() whenever the state changes.
You should override it if you need to specify any actions to be done when the state changes.
The default implementation acts for these states only:
- Connected: it sets up the socket notifiers to fire readyRead and readyWrite signals.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 429 of file k3clientsocketbase.cpp.
Waits for more data.
Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 304 of file k3clientsocketbase.cpp.
|
protectedvirtual |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Writes data to the socket.
Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KDatagramSocket, and KNetwork::KBufferedSocket.
Definition at line 340 of file k3clientsocketbase.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:12 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.