KDECore
#include <k3bufferedsocket.h>
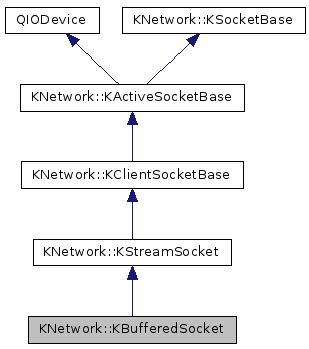
Public Member Functions | |
KBufferedSocket (const QString &node=QString(), const QString &service=QString(), QObject *parent=0L) | |
virtual | ~KBufferedSocket () |
virtual qint64 | bytesAvailable () const |
virtual qint64 | bytesToWrite () const |
virtual bool | canReadLine () const |
virtual void | close () |
virtual void | closeNow () |
virtual void | enableRead (bool enable) |
virtual void | enableWrite (bool enable) |
void | setInputBuffering (bool enable) |
void | setOutputBuffering (bool enable) |
virtual void | setSocketDevice (KSocketDevice *device) |
void | waitForConnect () |
virtual qint64 | waitForMore (int msecs, bool *timeout=0L) |
![]() | |
KStreamSocket (const QString &node=QString(), const QString &service=QString(), QObject *parent=0L) | |
virtual | ~KStreamSocket () |
virtual bool | bind (const QString &node=QString(), const QString &service=QString()) |
virtual bool | bind (const KResolverEntry &entry) |
virtual bool | connect (const QString &node=QString(), const QString &service=QString(), OpenMode mode=ReadWrite) |
virtual bool | connect (const KResolverEntry &entry, OpenMode mode=ReadWrite) |
int | remainingTimeout () const |
void | setTimeout (int msecs) |
int | timeout () const |
![]() | |
KClientSocketBase (QObject *parent) | |
virtual | ~KClientSocketBase () |
virtual bool | disconnect () |
bool | emitsReadyRead () const |
bool | emitsReadyWrite () const |
virtual bool | flush () |
virtual KSocketAddress | localAddress () const |
KResolver & | localResolver () const |
const KResolverResults & | localResults () const |
virtual bool | lookup () |
virtual bool | open (OpenMode mode) |
virtual KSocketAddress | peerAddress () const |
KResolver & | peerResolver () const |
const KResolverResults & | peerResults () const |
void | setFamily (int families) |
void | setResolutionEnabled (bool enable) |
SocketState | state () const |
![]() | |
KActiveSocketBase (QObject *parent) | |
virtual | ~KActiveSocketBase () |
virtual bool | atEnd () const |
QString | errorString () const |
virtual bool | isSequential () const |
qint64 | peek (char *data, qint64 maxlen) |
qint64 | peek (char *data, qint64 maxlen, KSocketAddress &from) |
virtual qint64 | pos () const |
qint64 | read (char *data, qint64 maxlen) |
QByteArray | read (qint64 len) |
qint64 | read (char *data, qint64 maxlen, KSocketAddress &from) |
virtual bool | seek (qint64) |
virtual qint64 | size () const |
void | ungetChar (char) |
qint64 | write (const char *data, qint64 len) |
qint64 | write (const QByteArray &data) |
qint64 | write (const char *data, qint64 len, const KSocketAddress &to) |
![]() | |
KSocketBase () | |
virtual | ~KSocketBase () |
bool | addressReuseable () const |
bool | blocking () const |
bool | broadcast () const |
SocketError | error () const |
QString | errorString () const |
bool | isIPv6Only () const |
QMutex * | mutex () const |
bool | noDelay () const |
virtual bool | setAddressReuseable (bool enable) |
virtual bool | setBlocking (bool enable) |
virtual bool | setBroadcast (bool enable) |
virtual bool | setIPv6Only (bool enable) |
virtual bool | setNoDelay (bool enable) |
int | setRequestedCapabilities (int add, int remove=0) |
KSocketDevice * | socketDevice () const |
Protected Slots | |
virtual void | slotReadActivity () |
virtual void | slotWriteActivity () |
![]() | |
virtual void | slotReadActivity () |
virtual void | slotWriteActivity () |
Protected Member Functions | |
virtual qint64 | peekData (char *data, qint64 maxlen, KSocketAddress *from) |
virtual qint64 | readData (char *data, qint64 maxlen, KSocketAddress *from) |
virtual qint64 | readLineData (char *data, qint64 maxSize) |
virtual bool | setSocketOptions (int opts) |
virtual void | stateChanging (SocketState newState) |
virtual qint64 | writeData (const char *data, qint64 len, const KSocketAddress *to) |
![]() | |
void | copyError () |
void | setState (SocketState state) |
![]() | |
virtual qint64 | readData (char *data, qint64 len) |
void | resetError () |
void | setError (SocketError error) |
virtual qint64 | writeData (const char *data, qint64 len) |
![]() | |
bool | hasDevice () const |
void | resetError () |
void | setError (SocketError error) |
virtual int | socketOptions () const |
Detailed Description
Buffered stream sockets.
This class allows the user to create and operate buffered stream sockets such as those used in most Internet connections. This class is also the one that resembles the most to the old QSocket implementation.
Objects of this type operate only in non-blocking mode. A call to setBlocking(true) will result in an error.
- Note
- Buffered sockets only make sense if you're using them from the main (event-loop) thread. This is actually a restriction imposed by Qt's QSocketNotifier. If you want to use a socket in an auxiliary thread, please use KStreamSocket.
- Deprecated:
- Use KSocketFactory or KLocalSocket instead
Definition at line 58 of file k3bufferedsocket.h.
Constructor & Destructor Documentation
|
explicit |
Default constructor.
- Parameters
-
node destination host service destination service to connect to parent the parent object for this object
Definition at line 52 of file k3bufferedsocket.cpp.
|
virtual |
Destructor.
Definition at line 61 of file k3bufferedsocket.cpp.
Member Function Documentation
|
virtual |
Make use of the buffers.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 99 of file k3bufferedsocket.cpp.
|
virtual |
Returns the length of the output buffer.
Definition at line 258 of file k3bufferedsocket.cpp.
|
virtual |
Returns true if a line can be read with readLine()
Definition at line 273 of file k3bufferedsocket.cpp.
|
virtual |
Closes the socket for new data, but allow data that had been buffered for output with writeData() to be still be written.
- See also
- closeNow
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 85 of file k3bufferedsocket.cpp.
|
virtual |
Closes the socket and discards any output data that had been buffered with writeData() but that had not yet been written.
- See also
- close
Definition at line 266 of file k3bufferedsocket.cpp.
|
virtual |
Catch changes.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 183 of file k3bufferedsocket.cpp.
|
virtual |
Catch changes.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 200 of file k3bufferedsocket.cpp.
|
protectedvirtual |
Peeks data from the socket.
The from
parameter is always set to peerAddress()
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 135 of file k3bufferedsocket.cpp.
|
protectedvirtual |
Reads data from a socket.
The from
parameter is always set to peerAddress()
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 119 of file k3bufferedsocket.cpp.
Improve the readLine performance.
Definition at line 281 of file k3bufferedsocket.cpp.
void KBufferedSocket::setInputBuffering | ( | bool | enable | ) |
Sets the use of input buffering.
Definition at line 230 of file k3bufferedsocket.cpp.
void KBufferedSocket::setOutputBuffering | ( | bool | enable | ) |
Sets the use of output buffering.
Definition at line 244 of file k3bufferedsocket.cpp.
|
virtual |
Be sure to catch new devices.
Reimplemented from KNetwork::KActiveSocketBase.
Definition at line 69 of file k3bufferedsocket.cpp.
|
protectedvirtual |
Buffered sockets can only operate in non-blocking mode.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 76 of file k3bufferedsocket.cpp.
|
protectedvirtualslot |
Slot called when there's read activity.
Definition at line 296 of file k3bufferedsocket.cpp.
|
protectedvirtualslot |
Slot called when there's write activity.
Definition at line 343 of file k3bufferedsocket.cpp.
|
protectedvirtual |
Catch connection to clear the buffers.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 212 of file k3bufferedsocket.cpp.
void KBufferedSocket::waitForConnect | ( | ) |
Blocks until the connection is either established, or completely failed.
Definition at line 286 of file k3bufferedsocket.cpp.
Make use of buffers.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 107 of file k3bufferedsocket.cpp.
|
protectedvirtual |
Writes data to the socket.
The to
parameter is discarded.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 151 of file k3bufferedsocket.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:12 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.