KDEUI
#include <krichtextwidget.h>
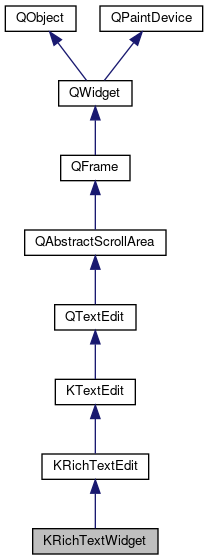
Public Types | |
enum | RichTextSupportValues { DisableRichText = 0x00, SupportBold = 0x01, SupportItalic = 0x02, SupportUnderline = 0x04, SupportStrikeOut = 0x08, SupportFontFamily = 0x10, SupportFontSize = 0x20, SupportTextForegroundColor = 0x40, SupportTextBackgroundColor = 0x80, FullTextFormattingSupport = 0xff, SupportChangeListStyle = 0x100, SupportIndentLists = 0x200, SupportDedentLists = 0x400, FullListSupport = 0xf00, SupportAlignment = 0x100000, SupportRuleLine = 0x400000, SupportHyperlinks = 0x800000, SupportFormatPainting = 0x1000000, SupportToPlainText = 0x2000000, SupportSuperScriptAndSubScript = 0x4000000, SupportDirection = 0x8000000, FullSupport = 0xffffffff } |
![]() | |
enum | Mode { Plain, Rich } |
Public Member Functions | |
KRichTextWidget (QWidget *parent) | |
KRichTextWidget (const QString &text, QWidget *parent=0) | |
~KRichTextWidget () | |
virtual void | createActions (KActionCollection *actionCollection) |
RichTextSupport | richTextSupport () const |
void | setRichTextSupport (const KRichTextWidget::RichTextSupport &support) |
void | updateActionStates () |
![]() | |
KRichTextEdit (const QString &text, QWidget *parent=0) | |
KRichTextEdit (QWidget *parent=0) | |
virtual | ~KRichTextEdit () |
bool | canDedentList () const |
bool | canIndentList () const |
QString | currentLinkText () const |
QString | currentLinkUrl () const |
void | enableRichTextMode () |
void | selectLinkText (QTextCursor *cursor) const |
void | selectLinkText () const |
void | setTextOrHtml (const QString &text) |
Mode | textMode () const |
QString | textOrHtml () const |
void | updateLink (const QString &linkUrl, const QString &linkText) |
![]() | |
KTextEdit (const QString &text, QWidget *parent=0) | |
KTextEdit (QWidget *parent=0) | |
~KTextEdit () | |
bool | checkSpellingEnabled () const |
QString | clickMessage () const |
virtual void | createHighlighter () |
void | enableFindReplace (bool enabled) |
void | forceSpellChecking () |
Sonnet::Highlighter * | highlighter () const |
void | highlightWord (int length, int pos) |
QMenu * | mousePopupMenu () |
void | setCheckSpellingEnabled (bool check) |
void | setClickMessage (const QString &msg) |
void | setHighlighter (Sonnet::Highlighter *_highLighter) |
virtual void | setReadOnly (bool readOnly) |
void | setSpellCheckingConfigFileName (const QString &fileName) |
void | setSpellInterface (KTextEditSpellInterface *spellInterface) |
void | showAutoCorrectButton (bool show) |
void | showTabAction (bool show) |
const QString & | spellCheckingLanguage () const |
![]() | |
QTextEdit (QWidget *parent) | |
QTextEdit (const QString &text, QWidget *parent) | |
QTextEdit (QWidget *parent, const char *name) | |
virtual | ~QTextEdit () |
bool | acceptRichText () const |
Qt::Alignment | alignment () const |
QString | anchorAt (const QPoint &pos) const |
void | append (const QString &text) |
AutoFormatting | autoFormatting () const |
bool | bold () const |
bool | canPaste () const |
void | clear () |
QColor | color () const |
void | copy () |
void | copyAvailable (bool yes) |
QMenu * | createStandardContextMenu () |
QMenu * | createStandardContextMenu (const QPoint &position) |
QTextCharFormat | currentCharFormat () const |
void | currentCharFormatChanged (const QTextCharFormat &f) |
void | currentColorChanged (const QColor &color) |
QFont | currentFont () const |
void | currentFontChanged (const QFont &font) |
QTextCursor | cursorForPosition (const QPoint &pos) const |
void | cursorPositionChanged () |
QRect | cursorRect (const QTextCursor &cursor) const |
QRect | cursorRect () const |
int | cursorWidth () const |
void | cut () |
QTextDocument * | document () const |
QString | documentTitle () const |
void | doKeyboardAction (KeyboardAction action) |
void | ensureCursorVisible () |
QList< ExtraSelection > | extraSelections () const |
QString | family () const |
bool | find (const QString &exp, QFlags< QTextDocument::FindFlag > options) |
bool | find (const QString &exp, bool cs, bool wo) |
QString | fontFamily () const |
bool | fontItalic () const |
qreal | fontPointSize () const |
bool | fontUnderline () const |
int | fontWeight () const |
bool | hasSelectedText () const |
void | insert (const QString &text) |
void | insertHtml (const QString &text) |
void | insertPlainText (const QString &text) |
bool | isModified () const |
bool | isReadOnly () const |
bool | isRedoAvailable () const |
bool | isUndoAvailable () const |
bool | isUndoRedoEnabled () const |
bool | italic () const |
int | lineWrapColumnOrWidth () const |
LineWrapMode | lineWrapMode () const |
virtual QVariant | loadResource (int type, const QUrl &name) |
void | mergeCurrentCharFormat (const QTextCharFormat &modifier) |
void | moveCursor (CursorAction action, QTextCursor::MoveMode mode) |
void | moveCursor (CursorAction action, bool select) |
void | moveCursor (QTextCursor::MoveOperation operation, QTextCursor::MoveMode mode) |
bool | overwriteMode () const |
void | paste () |
int | pointSize () const |
void | print (QPrinter *printer) const |
void | redo () |
void | redo () const |
void | redoAvailable (bool available) |
void | scrollToAnchor (const QString &name) |
void | selectAll () |
QString | selectedText () const |
void | selectionChanged () |
void | setAcceptRichText (bool accept) |
void | setAlignment (QFlags< Qt::AlignmentFlag > a) |
void | setAutoFormatting (QFlags< QTextEdit::AutoFormattingFlag > features) |
void | setBold (bool b) |
void | setColor (const QColor &color) |
void | setCurrentCharFormat (const QTextCharFormat &format) |
void | setCurrentFont (const QFont &f) |
void | setCursorWidth (int width) |
void | setDocument (QTextDocument *document) |
void | setDocumentTitle (const QString &title) |
void | setExtraSelections (const QList< ExtraSelection > &selections) |
void | setFamily (const QString &family) |
void | setFontFamily (const QString &fontFamily) |
void | setFontItalic (bool italic) |
void | setFontPointSize (qreal s) |
void | setFontUnderline (bool underline) |
void | setFontWeight (int weight) |
void | setHtml (const QString &text) |
void | setItalic (bool i) |
void | setLineWrapColumnOrWidth (int w) |
void | setLineWrapMode (LineWrapMode mode) |
void | setModified (bool m) |
void | setOverwriteMode (bool overwrite) |
void | setPlainText (const QString &text) |
void | setPointSize (int size) |
void | setReadOnly (bool ro) |
void | setTabChangesFocus (bool b) |
void | setTabStopWidth (int width) |
void | setText (const QString &text) |
void | setTextBackgroundColor (const QColor &c) |
void | setTextColor (const QColor &c) |
void | setTextCursor (const QTextCursor &cursor) |
void | setTextFormat (Qt::TextFormat f) |
void | setTextInteractionFlags (QFlags< Qt::TextInteractionFlag > flags) |
void | setUnderline (bool b) |
void | setUndoRedoEnabled (bool enable) |
void | setWordWrapMode (QTextOption::WrapMode policy) |
void | sync () |
bool | tabChangesFocus () const |
int | tabStopWidth () const |
QString | text () const |
QColor | textBackgroundColor () const |
void | textChanged () |
QColor | textColor () const |
QTextCursor | textCursor () const |
Qt::TextFormat | textFormat () const |
Qt::TextInteractionFlags | textInteractionFlags () const |
QString | toHtml () const |
QString | toPlainText () const |
bool | underline () const |
void | undo () const |
void | undo () |
void | undoAvailable (bool available) |
QTextOption::WrapMode | wordWrapMode () const |
void | zoomIn (int range) |
void | zoomOut (int range) |
![]() | |
QAbstractScrollArea (QWidget *parent) | |
~QAbstractScrollArea () | |
void | addScrollBarWidget (QWidget *widget, QFlags< Qt::AlignmentFlag > alignment) |
QWidget * | cornerWidget () const |
QScrollBar * | horizontalScrollBar () const |
Qt::ScrollBarPolicy | horizontalScrollBarPolicy () const |
QSize | maximumViewportSize () const |
virtual QSize | minimumSizeHint () const |
QWidgetList | scrollBarWidgets (QFlags< Qt::AlignmentFlag > alignment) |
void | setCornerWidget (QWidget *widget) |
void | setHorizontalScrollBar (QScrollBar *scrollBar) |
void | setHorizontalScrollBarPolicy (Qt::ScrollBarPolicy) |
void | setVerticalScrollBar (QScrollBar *scrollBar) |
void | setVerticalScrollBarPolicy (Qt::ScrollBarPolicy) |
void | setViewport (QWidget *widget) |
virtual QSize | sizeHint () const |
QScrollBar * | verticalScrollBar () const |
Qt::ScrollBarPolicy | verticalScrollBarPolicy () const |
QWidget * | viewport () const |
![]() | |
QFrame (QWidget *parent, QFlags< Qt::WindowType > f) | |
QFrame (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QFrame () | |
QRect | frameRect () const |
Shadow | frameShadow () const |
Shape | frameShape () const |
int | frameStyle () const |
int | frameWidth () const |
int | lineWidth () const |
int | midLineWidth () const |
void | setFrameRect (const QRect &) |
void | setFrameShadow (Shadow) |
void | setFrameShape (Shape) |
void | setFrameStyle (int style) |
void | setLineWidth (int) |
void | setMidLineWidth (int) |
![]() | |
QWidget (QWidget *parent, QFlags< Qt::WindowType > f) | |
QWidget (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QWidget () | |
bool | acceptDrops () const |
QString | accessibleDescription () const |
QString | accessibleName () const |
QList< QAction * > | actions () const |
void | activateWindow () |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
Qt::BackgroundMode | backgroundMode () const |
QPoint | backgroundOffset () const |
BackgroundOrigin | backgroundOrigin () const |
QPalette::ColorRole | backgroundRole () const |
QSize | baseSize () const |
QString | caption () const |
QWidget * | childAt (int x, int y, bool includeThis) const |
QWidget * | childAt (const QPoint &p, bool includeThis) const |
QWidget * | childAt (int x, int y) const |
QWidget * | childAt (const QPoint &p) const |
QRect | childrenRect () const |
QRegion | childrenRegion () const |
void | clearFocus () |
void | clearMask () |
bool | close (bool alsoDelete) |
bool | close () |
QColorGroup | colorGroup () const |
void | constPolish () const |
QMargins | contentsMargins () const |
QRect | contentsRect () const |
Qt::ContextMenuPolicy | contextMenuPolicy () const |
QCursor | cursor () const |
void | customContextMenuRequested (const QPoint &pos) |
void | drawText (const QPoint &p, const QString &s) |
void | drawText (int x, int y, const QString &s) |
WId | effectiveWinId () const |
void | ensurePolished () const |
void | erase () |
void | erase (const QRect &rect) |
void | erase (const QRegion &rgn) |
void | erase (int x, int y, int w, int h) |
Qt::FocusPolicy | focusPolicy () const |
QWidget * | focusProxy () const |
QWidget * | focusWidget () const |
const QFont & | font () const |
QFontInfo | fontInfo () const |
QFontMetrics | fontMetrics () const |
QPalette::ColorRole | foregroundRole () const |
QRect | frameGeometry () const |
QSize | frameSize () const |
const QRect & | geometry () const |
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
virtual HDC | getDC () const |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsProxyWidget * | graphicsProxyWidget () const |
bool | hasEditFocus () const |
bool | hasFocus () const |
bool | hasMouse () const |
bool | hasMouseTracking () const |
int | height () const |
virtual int | heightForWidth (int w) const |
void | hide () |
const QPixmap * | icon () const |
void | iconify () |
QString | iconText () const |
QInputContext * | inputContext () |
Qt::InputMethodHints | inputMethodHints () const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
bool | isAncestorOf (const QWidget *child) const |
bool | isDesktop () const |
bool | isDialog () const |
bool | isEnabled () const |
bool | isEnabledTo (QWidget *ancestor) const |
bool | isEnabledToTLW () const |
bool | isFullScreen () const |
bool | isHidden () const |
bool | isInputMethodEnabled () const |
bool | isMaximized () const |
bool | isMinimized () const |
bool | isModal () const |
bool | isPopup () const |
bool | isShown () const |
bool | isTopLevel () const |
bool | isUpdatesEnabled () const |
bool | isVisible () const |
bool | isVisibleTo (QWidget *ancestor) const |
bool | isVisibleToTLW () const |
bool | isWindow () const |
bool | isWindowModified () const |
QLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
QLocale | locale () const |
void | lower () |
Qt::HANDLE | macCGHandle () const |
Qt::HANDLE | macQDHandle () const |
QPoint | mapFrom (QWidget *parent, const QPoint &pos) const |
QPoint | mapFromGlobal (const QPoint &pos) const |
QPoint | mapFromParent (const QPoint &pos) const |
QPoint | mapTo (QWidget *parent, const QPoint &pos) const |
QPoint | mapToGlobal (const QPoint &pos) const |
QPoint | mapToParent (const QPoint &pos) const |
QRegion | mask () const |
int | maximumHeight () const |
QSize | maximumSize () const |
int | maximumWidth () const |
int | minimumHeight () const |
QSize | minimumSize () const |
int | minimumWidth () const |
void | move (int x, int y) |
void | move (const QPoint &) |
QWidget * | nativeParentWidget () const |
QWidget * | nextInFocusChain () const |
QRect | normalGeometry () const |
void | overrideWindowFlags (QFlags< Qt::WindowType > flags) |
bool | ownCursor () const |
bool | ownFont () const |
bool | ownPalette () const |
virtual QPaintEngine * | paintEngine () const |
const QPalette & | palette () const |
QWidget * | parentWidget (bool sameWindow) const |
QWidget * | parentWidget () const |
QPlatformWindow * | platformWindow () const |
QPlatformWindowFormat | platformWindowFormat () const |
void | polish () |
QPoint | pos () const |
QWidget * | previousInFocusChain () const |
void | raise () |
void | recreate (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
QRect | rect () const |
virtual void | releaseDC (HDC hdc) const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | repaint (int x, int y, int w, int h, bool b) |
void | repaint (const QRegion &rgn, bool b) |
void | repaint () |
void | repaint (int x, int y, int w, int h) |
void | repaint (const QRegion &rgn) |
void | repaint (bool b) |
void | repaint (const QRect &rect) |
void | repaint (const QRect &r, bool b) |
void | reparent (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
void | reparent (QWidget *parent, const QPoint &p, bool showIt) |
void | resize (int w, int h) |
void | resize (const QSize &) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setActiveWindow () |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setBackgroundMode (Qt::BackgroundMode widgetBackground, Qt::BackgroundMode paletteBackground) |
void | setBackgroundOrigin (BackgroundOrigin background) |
void | setBackgroundPixmap (const QPixmap &pixmap) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setCaption (const QString &c) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContentsMargins (const QMargins &margins) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setEraseColor (const QColor &color) |
void | setErasePixmap (const QPixmap &pixmap) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus (Qt::FocusReason reason) |
void | setFocus () |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setFont (const QFont &f, bool b) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (int x, int y, int w, int h) |
void | setGeometry (const QRect &) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setIcon (const QPixmap &i) |
void | setIconText (const QString &it) |
void | setInputContext (QInputContext *context) |
void | setInputMethodEnabled (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setKeyCompression (bool b) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setPalette (const QPalette &p, bool b) |
void | setPaletteBackgroundColor (const QColor &color) |
void | setPaletteBackgroundPixmap (const QPixmap &pixmap) |
void | setPaletteForegroundColor (const QColor &color) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, QFlags< Qt::WindowType > f) |
void | setPlatformWindow (QPlatformWindow *window) |
void | setPlatformWindowFormat (const QPlatformWindowFormat &format) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setShown (bool shown) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy::Policy hor, QSizePolicy::Policy ver, bool hfw) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setSizePolicy (QSizePolicy) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
QStyle * | setStyle (const QString &style) |
void | setStyleSheet (const QString &styleSheet) |
void | setToolTip (const QString &) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlags (QFlags< Qt::WindowType > type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (QFlags< Qt::WindowState > windowState) |
void | setWindowSurface (QWindowSurface *surface) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const |
QSize | sizeIncrement () const |
QSizePolicy | sizePolicy () const |
void | stackUnder (QWidget *w) |
QString | statusTip () const |
QStyle * | style () const |
QString | styleSheet () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
QString | toolTip () const |
QWidget * | topLevelWidget () const |
bool | underMouse () const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetFont () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | unsetPalette () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | update () |
void | updateGeometry () |
bool | updatesEnabled () const |
QRect | visibleRect () const |
QRegion | visibleRegion () const |
QString | whatsThis () const |
int | width () const |
QWidget * | window () const |
QString | windowFilePath () const |
Qt::WindowFlags | windowFlags () const |
QIcon | windowIcon () const |
QString | windowIconText () const |
Qt::WindowModality | windowModality () const |
qreal | windowOpacity () const |
QString | windowRole () const |
Qt::WindowStates | windowState () const |
QWindowSurface * | windowSurface () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
WId | winId () const |
int | x () const |
const QX11Info & | x11Info () const |
Qt::HANDLE | x11PictureHandle () const |
int | y () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
Protected Member Functions | |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
![]() | |
virtual void | keyPressEvent (QKeyEvent *event) |
![]() | |
bool | checkSpellingEnabledInternal () const |
virtual void | contextMenuEvent (QContextMenuEvent *) |
virtual void | deleteWordBack () |
virtual void | deleteWordForward () |
virtual bool | event (QEvent *) |
virtual void | focusInEvent (QFocusEvent *) |
virtual void | focusOutEvent (QFocusEvent *) |
virtual void | paintEvent (QPaintEvent *) |
void | setCheckSpellingEnabledInternal (bool check) |
virtual void | wheelEvent (QWheelEvent *) |
![]() | |
virtual bool | canInsertFromMimeData (const QMimeData *source) const |
virtual void | changeEvent (QEvent *e) |
virtual QMimeData * | createMimeDataFromSelection () const |
virtual void | dragEnterEvent (QDragEnterEvent *e) |
virtual void | dragLeaveEvent (QDragLeaveEvent *e) |
virtual void | dragMoveEvent (QDragMoveEvent *e) |
virtual void | dropEvent (QDropEvent *e) |
virtual bool | focusNextPrevChild (bool next) |
virtual void | inputMethodEvent (QInputMethodEvent *e) |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery property) const |
virtual void | insertFromMimeData (const QMimeData *source) |
virtual void | keyReleaseEvent (QKeyEvent *e) |
virtual void | mouseDoubleClickEvent (QMouseEvent *e) |
virtual void | mouseMoveEvent (QMouseEvent *e) |
virtual void | mousePressEvent (QMouseEvent *e) |
virtual void | resizeEvent (QResizeEvent *e) |
virtual void | scrollContentsBy (int dx, int dy) |
virtual void | showEvent (QShowEvent *) |
![]() | |
void | setupViewport (QWidget *viewport) |
void | setViewportMargins (const QMargins &margins) |
void | setViewportMargins (int left, int top, int right, int bottom) |
virtual bool | viewportEvent (QEvent *event) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | enterEvent (QEvent *event) |
bool | focusNextChild () |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | languageChange () |
virtual void | leaveEvent (QEvent *event) |
virtual bool | macEvent (EventHandlerCallRef caller, EventRef event) |
virtual int | metric (PaintDeviceMetric m) const |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | qwsEvent (QWSEvent *event) |
void | resetInputContext () |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus () |
virtual bool | winEvent (MSG *message, long *result) |
virtual bool | x11Event (XEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QPaintDevice () | |
Additional Inherited Members | |
![]() | |
void | selectionFinished () |
void | textModeChanged (KRichTextEdit::Mode mode) |
![]() | |
void | aboutToShowContextMenu (QMenu *menu) |
void | checkSpellingChanged (bool) |
void | languageChanged (const QString &language) |
void | spellCheckerAutoCorrect (const QString ¤tWord, const QString &autoCorrectWord) |
void | spellCheckingCanceled () |
void | spellCheckingFinished () |
void | spellCheckStatus (const QString &) |
![]() | |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
QWidgetMapper * | wmapper () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
int | x11AppCells (int screen) |
Qt::HANDLE | x11AppColormap (int screen) |
bool | x11AppDefaultColormap (int screen) |
bool | x11AppDefaultVisual (int screen) |
int | x11AppDepth (int screen) |
Display * | x11AppDisplay () |
int | x11AppDpiX (int screen) |
int | x11AppDpiY (int screen) |
Qt::HANDLE | x11AppRootWindow (int screen) |
int | x11AppScreen () |
void * | x11AppVisual (int screen) |
void | x11SetAppDpiX (int dpi, int screen) |
void | x11SetAppDpiY (int dpi, int screen) |
![]() | |
typedef | AutoFormatting |
![]() | |
typedef | RenderFlags |
![]() | |
void | slotDoFind () |
void | slotDoReplace () |
void | slotFind () |
void | slotFindNext () |
void | slotFindPrevious () |
void | slotReplace () |
void | slotReplaceNext () |
void | slotSpeakText () |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
A KRichTextEdit with common actions.
This class implements common actions which are often used with KRichTextEdit. All you need to do is to call createActions(), and the actions will be added to your KXMLGUIWindow. Remember to also add the chosen actions to your application ui.rc file.
See the KRichTextWidget::RichTextSupportValues enum for an overview of supported actions.
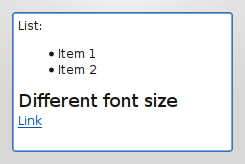
- Since
- 4.1
Definition at line 45 of file krichtextwidget.h.
Member Enumeration Documentation
These flags describe what actions will be created by createActions() after passing a combination of these flags to setRichTextSupport().
Enumerator | |
---|---|
DisableRichText |
No rich text support at all, no actions will be created. Do not use in combination with other flags. |
SupportBold |
Action to format the selected text as bold. If no text is selected, the word under the cursor is formatted bold. This is a KToggleAction. The status is automatically updated when the text cursor is moved. |
SupportItalic |
Action to format the selected text as italic. If no text is selected, the word under the cursor is formatted italic. This is a KToggleAction. The status is automatically updated when the text cursor is moved. |
SupportUnderline |
Action to underline the selected text. If no text is selected, the word under the cursor is underlined. This is a KToggleAction. The status is automatically updated when the text cursor is moved. |
SupportStrikeOut |
Action to strike out the selected text. If no text is selected, the word under the cursor is struck out. This is a KToggleAction. The status is automatically updated when the text cursor is moved. |
SupportFontFamily |
Action to change the font family of the currently selected text. If no text is selected, the font family of the word under the cursor is changed. Displayed as a combobox when inserted into the toolbar. This is a KFontAction. The status is automatically updated when the text cursor is moved. |
SupportFontSize |
Action to change the font size of the currently selected text. If no text is selected, the font size of the word under the cursor is changed. Displayed as a combobox when inserted into the toolbar. This is a KFontSizeAction. The status is automatically updated when the text cursor is moved. |
SupportTextForegroundColor |
Action to change the text color of the currently selected text. If no text is selected, the text color of the word under the cursor is changed. Opens a KColorDialog to select the color. |
SupportTextBackgroundColor |
Action to change the background color of the currently selected text. If no text is selected, the backgound color of the word under the cursor is changed. Opens a KColorDialog to select the color. |
FullTextFormattingSupport |
A combination of all the flags above. Includes all actions that change the format of the text. |
SupportChangeListStyle |
Action to make the current line a list element, change the list style or remove list formatting. Displayed as a combobox when inserted into a toolbar. This is a KSelectAction. The status is automatically updated when the text cursor is moved. |
SupportIndentLists |
Action to increase the current list nesting level. This makes it possible to create nested lists. |
SupportDedentLists |
Action to decrease the current list nesting level. |
FullListSupport |
All of the three list actions above. Includes all list-related actions. |
SupportAlignment |
Actions to align the current paragraph left, righ, center or justify. These actions are KToogleActions. The status is automatically updated when the text cursor is moved. |
SupportRuleLine |
Action to insert a horizontal line. |
SupportHyperlinks |
Action to convert the current text to a hyperlink. If no text is selected, the word under the cursor is converted. This action opens a dialog where the user can enter the link target. |
SupportFormatPainting |
Action to make the mouse cursor a format painter. The user can select text with that painter. The selected text gets the same format as the text that was previously selected. |
SupportToPlainText |
Action to change the text of the whole text edit to plain text. All rich text formatting will get lost. |
SupportSuperScriptAndSubScript |
Actions to format text as superscript or subscript. If no text is selected, the word under the cursor is formatted as selected. This is a KToggleAction. The status is automatically updated when the text cursor is moved. |
SupportDirection |
Action to change direction of text to Right-To-Left or Left-To-Right. |
FullSupport |
Includes all above actions for full rich text support. |
Definition at line 56 of file krichtextwidget.h.
Constructor & Destructor Documentation
|
explicit |
Constructor.
- Parameters
-
parent the parent widget
Constructs a KRichTextWidget object.
- Parameters
-
text The initial text of the text edit, which is interpreted as HTML. parent The parent widget
KRichTextWidget::~KRichTextWidget | ( | ) |
Destructor.
Member Function Documentation
|
virtual |
Creates the actions and adds them to the given action collection.
Call this before calling setupGUI() in your application, but after calling setRichTextSupport().
The XML file of your KXmlGuiWindow needs to have the action names in them, so that the actions actually appear in the menu and in the toolbars.
Below is a list of actions that are created,depending on the supported rich text subset set by setRichTextSupport(). The list contains action names. Those names need to be the same in your XML file.
See the KRichTextWidget::RichTextSupportValues enum documentation for a detailed explaination of each action.
XML Name | RichTextSupportValues flag |
format_text_foreground_color | SupportTextForegroundColor |
format_text_background_color | SupportTextBackgroundColor |
format_font_family | SupportFontFamily |
format_font_size | SupportFontSize |
format_text_bold | SupportBold |
format_text_italic | SupportItalic |
format_text_underline | SupportUnderline |
format_text_strikeout | SupportStrikeOut |
format_align_left | SupportAlignment |
format_align_center | SupportAlignment |
format_align_right | SupportAlignment |
format_align_justify | SupportAlignment |
direction_ltr | SupportDirection |
direction_rtl | SupportDirection |
format_list_style | SupportChangeListStyle |
format_list_indent_more | SupportIndentLists |
format_list_indent_less | SupportDedentLists |
insert_horizontal_rule | SupportRuleLine |
manage_link | SupportHyperlinks |
format_painter | SupportFormatPainting |
action_to_plain_text | SupportToPlainText |
format_text_subscript & format_text_superscript | SupportSuperScriptAndSubScript |
- Parameters
-
actionCollection the actions will be added to this action collection
|
protectedvirtual |
Reimplemented.
Catches mouse release events. Used to know when a selection has been completed.
Reimplemented from QTextEdit.
RichTextSupport KRichTextWidget::richTextSupport | ( | ) | const |
Returns the supported rich text subset available.
- Returns
- The supported subset.
|
slot |
Disables or enables all of the actions created by createActions().
This may be useful in cases where rich text mode may be set on or off.
- Parameters
-
enabled Whether to enable or disable the actions.
void KRichTextWidget::setRichTextSupport | ( | const KRichTextWidget::RichTextSupport & | support | ) |
Sets the supported rich text subset available.
The default is KRichTextWidget::FullSupport and will be set in the constructor.
You need to call createActions() afterwards.
- Parameters
-
support The supported subset.
void KRichTextWidget::updateActionStates | ( | ) |
Tells KRichTextWidget to update the state of the actions created by createActions().
This is normally automatically done, but there might be a few cases where you'll need to manually call this function.
Call this function only after calling createActions().
Property Documentation
|
readwrite |
Definition at line 49 of file krichtextwidget.h.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:03 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.