KIO
#include <previewjob.h>
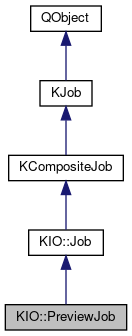
Public Types | |
enum | ScaleType { Unscaled, Scaled, ScaledAndCached } |
![]() | |
enum | Capability |
enum | KillVerbosity |
enum | Unit |
Signals | |
void | failed (const KFileItem &item) |
void | gotPreview (const KFileItem &item, const QPixmap &preview) |
![]() | |
void | canceled (KJob *job) |
void | connected (KIO::Job *job) |
![]() | |
void | description (KJob *job, const QString &title, const QPair< QString, QString > &field1=qMakePair(QString(), QString()), const QPair< QString, QString > &field2=qMakePair(QString(), QString())) |
void | finished (KJob *job) |
void | infoMessage (KJob *job, const QString &plain, const QString &rich=QString()) |
void | percent (KJob *job, unsigned long percent) |
void | processedAmount (KJob *job, KJob::Unit unit, qulonglong amount) |
void | processedSize (KJob *job, qulonglong size) |
void | result (KJob *job) |
void | resumed (KJob *job) |
void | speed (KJob *job, unsigned long speed) |
void | suspended (KJob *job) |
void | totalAmount (KJob *job, KJob::Unit unit, qulonglong amount) |
void | totalSize (KJob *job, qulonglong size) |
void | warning (KJob *job, const QString &plain, const QString &rich=QString()) |
Public Member Functions | |
PreviewJob (const KFileItemList &items, int width, int height, int iconSize, int iconAlpha, bool scale, bool save, const QStringList *enabledPlugins) | |
PreviewJob (const KFileItemList &items, const QSize &size, const QStringList *enabledPlugins=0) | |
virtual | ~PreviewJob () |
int | overlayIconAlpha () const |
int | overlayIconSize () const |
void | removeItem (const KUrl &url) |
ScaleType | scaleType () const |
int | sequenceIndex () const |
void | setIgnoreMaximumSize (bool ignoreSize=true) |
void | setOverlayIconAlpha (int alpha) |
void | setOverlayIconSize (int size) |
void | setScaleType (ScaleType type) |
void | setSequenceIndex (int index) |
![]() | |
virtual | ~Job () |
void | addMetaData (const QString &key, const QString &value) |
void | addMetaData (const QMap< QString, QString > &values) |
QStringList | detailedErrorStrings (const KUrl *reqUrl=0L, int method=-1) const |
QString | errorString () const |
bool | isInteractive () const |
void | mergeMetaData (const QMap< QString, QString > &values) |
MetaData | metaData () const |
MetaData | outgoingMetaData () const |
Job * | parentJob () const |
QString | queryMetaData (const QString &key) |
void | setMetaData (const KIO::MetaData &metaData) |
void | setParentJob (Job *parentJob) |
void | showErrorDialog (QWidget *parent=0) |
void | start () |
JobUiDelegate * | ui () const |
![]() | |
KCompositeJob (QObject *parent=0) | |
virtual | ~KCompositeJob () |
virtual | ~KJob () |
Capabilities | capabilities () const |
int | error () const |
QString | errorText () const |
bool | exec () |
bool | isAutoDelete () const |
bool | isSuspended () const |
KJob (QObject *parent=0) | |
unsigned long | percent () const |
qulonglong | processedAmount (Unit unit) const |
void | setAutoDelete (bool autodelete) |
void | setUiDelegate (KJobUiDelegate *delegate) |
qulonglong | totalAmount (Unit unit) const |
KJobUiDelegate * | uiDelegate () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static QStringList | availablePlugins () |
static KIO::filesize_t | maximumFileSize () |
static QStringList | supportedMimeTypes () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Protected Slots | |
virtual void | slotResult (KJob *job) |
![]() | |
virtual void | slotInfoMessage (KJob *job, const QString &plain, const QString &rich) |
virtual void | slotResult (KJob *job) |
Additional Inherited Members | |
![]() | |
bool | kill (KillVerbosity verbosity=Quietly) |
bool | resume () |
bool | suspend () |
![]() | |
Job () | |
Job (JobPrivate &dd) | |
virtual bool | addSubjob (KJob *job) |
virtual bool | doKill () |
virtual bool | doResume () |
virtual bool | doSuspend () |
virtual bool | removeSubjob (KJob *job) |
![]() | |
KCompositeJob (KCompositeJobPrivate &dd, QObject *parent) | |
void | clearSubjobs () |
void | emitPercent (qulonglong processedAmount, qulonglong totalAmount) |
void | emitResult () |
void | emitSpeed (unsigned long speed) |
bool | hasSubjobs () |
KJob (KJobPrivate &dd, QObject *parent) | |
void | setCapabilities (Capabilities capabilities) |
void | setError (int errorCode) |
void | setErrorText (const QString &errorText) |
void | setPercent (unsigned long percentage) |
void | setProcessedAmount (Unit unit, qulonglong amount) |
void | setTotalAmount (Unit unit, qulonglong amount) |
const QList< KJob * > & | subjobs () const |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
KJobPrivate *const | d_ptr |
![]() | |
objectName | |
Detailed Description
KIO Job to get a thumbnail picture.
This class catches a preview (thumbnail) for files.
Definition at line 38 of file previewjob.h.
Member Enumeration Documentation
Specifies the type of scaling that is applied to the generated preview.
- Since
- 4.7
Enumerator | |
---|---|
Unscaled |
The original size of the preview will be returned. Most previews will return a size of 256 x 256 pixels. |
Scaled |
The preview will be scaled to the size specified when constructing the PreviewJob. The aspect ratio will be kept. |
ScaledAndCached |
The preview will be scaled to the size specified when constructing the PreviewJob. The result will be cached for later use. Per default ScaledAndCached is set. |
Definition at line 46 of file previewjob.h.
Constructor & Destructor Documentation
PreviewJob::PreviewJob | ( | const KFileItemList & | items, |
int | width, | ||
int | height, | ||
int | iconSize, | ||
int | iconAlpha, | ||
bool | scale, | ||
bool | save, | ||
const QStringList * | enabledPlugins | ||
) |
Creates a new PreviewJob.
- Parameters
-
items a list of files to create previews for width the desired width height the desired height, 0 to use the width
iconSize the size of the mimetype icon to overlay over the preview or zero to not overlay an icon. This has no effect if the preview plugin that will be used doesn't use icon overlays. iconAlpha transparency to use for the icon overlay scale if the image is to be scaled to the requested size or returned in its original size save if the image should be cached for later use enabledPlugins If non-zero, this points to a list containing the names of the plugins that may be used. If enabledPlugins is zero all available plugins are used.
- Deprecated:
- Use PreviewJob(KFileItemList, QSize, QStringList) in combination with the setter-methods instead. Note that the semantics of
enabledPlugins
has been slightly changed.
Definition at line 134 of file previewjob.cpp.
PreviewJob::PreviewJob | ( | const KFileItemList & | items, |
const QSize & | size, | ||
const QStringList * | enabledPlugins = 0 |
||
) |
- Parameters
-
items List of files to create previews for. size Desired size of the preview. enabledPlugins If non-zero it defines the list of plugins that are considered for generating the preview. If enabledPlugins is zero the plugins specified in the KConfigGroup "PreviewSettings" are used.
- Since
- 4.7
Definition at line 165 of file previewjob.cpp.
|
virtual |
Definition at line 203 of file previewjob.cpp.
Member Function Documentation
|
static |
Returns a list of all available preview plugins.
The list contains the basenames of the plugins' .desktop files (no path, no .desktop).
- Returns
- the list of plugins
Definition at line 735 of file previewjob.cpp.
|
signal |
Emitted when a thumbnail for item
could not be created, either because a ThumbCreator for its MIME type does not exist, or because something went wrong.
- Parameters
-
item the file that failed
Emitted when a thumbnail picture for item
has been successfully retrieved.
- Parameters
-
item the file of the preview preview the preview image
|
static |
Returns the default "maximum file size", in bytes, used by PreviewJob.
This is useful for applications providing a GUI for letting the user change the size.
- Since
- 4.1
- Deprecated:
- PreviewJob uses different maximum file sizes dependent on the URL since 4.5. The returned file size is only valid for local URLs.
Definition at line 783 of file previewjob.cpp.
int PreviewJob::overlayIconAlpha | ( | ) | const |
- Returns
- The alpha-value for the MIME-type icon which overlays the preview. Per default 70 is returned.
- Since
- 4.7
Definition at line 232 of file previewjob.cpp.
int PreviewJob::overlayIconSize | ( | ) | const |
- Returns
- The size of the MIME-type icon which overlays the preview.
- See also
- PreviewJob::setOverlayIconSize()
- Since
- 4.7
Definition at line 220 of file previewjob.cpp.
void PreviewJob::removeItem | ( | const KUrl & | url | ) |
Removes an item from preview processing.
Use this if you passed an item to filePreview and want to delete it now.
- Parameters
-
url the url of the item that should be removed from the preview queue
Definition at line 381 of file previewjob.cpp.
PreviewJob::ScaleType PreviewJob::scaleType | ( | ) | const |
- Returns
- The scale type for the generated preview.
- See also
- PreviewJob::ScaleType
- Since
- 4.7
Definition at line 259 of file previewjob.cpp.
int KIO::PreviewJob::sequenceIndex | ( | ) | const |
Returns the currently set sequence index.
- Since
- KDE 4.3
Definition at line 404 of file previewjob.cpp.
void PreviewJob::setIgnoreMaximumSize | ( | bool | ignoreSize = true | ) |
If ignoreSize
is true, then the preview is always generated regardless of the settings.
Definition at line 408 of file previewjob.cpp.
void PreviewJob::setOverlayIconAlpha | ( | int | alpha | ) |
Sets the alpha-value for the MIME-type icon which overlays the preview.
The alpha-value may range from 0 (= fully transparent) to 255 (= opaque). Per default the value is set to 70.
- See also
- PreviewJob::setOverlayIconSize()
- Since
- 4.7
Definition at line 226 of file previewjob.cpp.
void PreviewJob::setOverlayIconSize | ( | int | size | ) |
Sets the size of the MIME-type icon which overlays the preview.
If zero is passed no overlay will be shown at all. The setting has no effect if the preview plugin that will be used does not use icon overlays. Per default the size is set to 0.
- Since
- 4.7
Definition at line 214 of file previewjob.cpp.
void PreviewJob::setScaleType | ( | ScaleType | type | ) |
Sets the scale type for the generated preview.
Per default PreviewJob::ScaledAndCached is set.
- See also
- PreviewJob::ScaleType
- Since
- 4.7
Definition at line 238 of file previewjob.cpp.
void KIO::PreviewJob::setSequenceIndex | ( | int | index | ) |
Sets the sequence index given to the thumb creators.
Use the sequence index, it is possible to create alternative icons for the same item. For example it may allow iterating through the items of a directory, or the frames of a video.
- Since
- KDE 4.3
Definition at line 400 of file previewjob.cpp.
|
protectedvirtualslot |
Definition at line 440 of file previewjob.cpp.
|
static |
Returns a list of all supported MIME types.
The list can contain entries like text/ * (without the space).
- Returns
- the list of mime types
Definition at line 745 of file previewjob.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:55 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.