KGameProcessIO
#include <KGame/KGameIO>
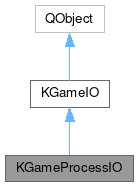
Signals | |
void | signalIOAdded (KGameIO *game, QDataStream &stream, KPlayer *p, bool *send) |
void | signalProcessQuery (QDataStream &stream, KGameProcessIO *me) |
void | signalReceivedStderr (const QString &msg) |
![]() | |
void | signalPrepareTurn (QDataStream &stream, bool turn, KGameIO *io, bool *send) |
Public Member Functions | |
KGameProcessIO (const QString &name) | |
~KGameProcessIO () override | |
void | initIO (KPlayer *p) override |
void | notifyTurn (bool turn) override |
int | rtti () const override |
void | sendMessage (QDataStream &stream, int msgid, quint32 receiver, quint32 sender) |
void | sendSystemMessage (QDataStream &stream, int msgid, quint32 receiver, quint32 sender) |
![]() | |
KGameIO () | |
KGameIO (KPlayer *) | |
void | Debug () |
KGame * | game () const |
KPlayer * | player () const |
bool | sendInput (QDataStream &stream, bool transmit=true, quint32 sender=0) |
void | setPlayer (KPlayer *p) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Slots | |
void | receivedMessage (const QByteArray &receiveBuffer) |
Protected Member Functions | |
void | sendAllMessages (QDataStream &stream, int msgid, quint32 receiver, quint32 sender, bool usermsg) |
![]() | |
KGameIO (KGameIOPrivate &dd, KPlayer *player=nullptr) | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
enum | IOMode { GenericIO = 1 , KeyIO = 2 , MouseIO = 4 , ProcessIO = 8 , ComputerIO = 16 } |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Detailed Description
The KGameProcessIO class.
It is used to create a computer player via a separate process and communicate transparently with it. Its counterpart is the KGameProcess class which needs to be used by the computer player. See its documentation for the definition of the computer player.
Constructor & Destructor Documentation
◆ KGameProcessIO()
|
explicit |
Creates a computer player via a separate process.
The process name is given as fully qualified filename. Example:
- Parameters
-
name the filename of the process to start
Definition at line 260 of file kgameio.cpp.
◆ ~KGameProcessIO()
|
override |
Deletes the process input devices.
Definition at line 288 of file kgameio.cpp.
Member Function Documentation
◆ initIO()
|
overridevirtual |
Init this device by setting the player and e.g.
sending an init message to the device. Calling this function will emit the IOAdded signal on which you can react and initilise the computer player. This function is called automatically when adding the IO to a player.
Reimplemented from KGameIO.
Definition at line 308 of file kgameio.cpp.
◆ notifyTurn()
|
overridevirtual |
Notifies the IO device that the player's setTurn had been called Called by KPlayer.
You can react on the signalPrepareTurn to prepare a message for the process, i.e. either update it on the changes made to the game since the last turn or the initIO has been called or transmit your gamestatus now.
- Parameters
-
turn is true/false
Reimplemented from KGameIO.
Definition at line 328 of file kgameio.cpp.
◆ receivedMessage
|
protectedslot |
Internal message handler to receive data from the process.
Definition at line 387 of file kgameio.cpp.
◆ rtti()
|
overridevirtual |
The identification of the IO.
- Returns
- ProcessIO
Implements KGameIO.
Definition at line 303 of file kgameio.cpp.
◆ sendAllMessages()
|
protected |
Internal combined function for all message handling.
Definition at line 356 of file kgameio.cpp.
◆ sendMessage()
void KGameProcessIO::sendMessage | ( | QDataStream & | stream, |
int | msgid, | ||
quint32 | receiver, | ||
quint32 | sender ) |
Send a message to the process.
This is analogous to the sendMessage commands of KGame. It will result in a signal of the computer player on which you can react in the process player.
- Parameters
-
stream - the actual data msgid - the id of the message receiver - not used sender - who send the message
Definition at line 351 of file kgameio.cpp.
◆ sendSystemMessage()
void KGameProcessIO::sendSystemMessage | ( | QDataStream & | stream, |
int | msgid, | ||
quint32 | receiver, | ||
quint32 | sender ) |
Send a system message to the process.
This is analogous to the sendMessage commands of KGame. It will result in a signal of the computer player on which you can react in the process player.
- Parameters
-
stream - the actual data msgid - the id of the message receiver - not used sender - who send the message
Definition at line 346 of file kgameio.cpp.
◆ signalIOAdded
|
signal |
Signal generated when the computer player is added.
You can use this to communicated with the process and e.g. send initialisation information to the process.
- Parameters
-
game the KGameIO object itself stream the stream into which the move will be written p the player itself send set this to false if no move should be generated
◆ signalProcessQuery
|
signal |
A computer query message is received.
This is a 'dummy' message sent by the process if it needs to communicate with us. It is not forwarded over the network. Reacting to this message allows you to 'answer' questions of the process, e.g. sending addition data which the process needs to calculate a move.
Example:
◆ signalReceivedStderr
|
signal |
Text is received by the process on STDERR.
This is usually a debug string.
- Parameters
-
msg The text
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:46:49 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.