Marble::AbstractDataPluginModel
#include <AbstractDataPluginModel.h>
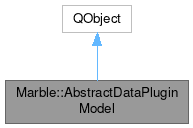
Properties | |
QObject * | favoritesModel |
![]() | |
objectName | |
Signals | |
void | favoriteItemsChanged (const QStringList &favoriteItems) |
void | favoriteItemsOnlyChanged () |
void | itemsUpdated () |
Public Slots | |
void | addItemsToList (const QList< AbstractDataPluginItem * > &items) |
void | addItemToList (AbstractDataPluginItem *item) |
void | clear () |
Public Member Functions | |
AbstractDataPluginModel (const QString &name, const MarbleModel *marbleModel, QObject *parent=nullptr) | |
QStringList | favoriteItems () const |
QObject * | favoritesModel () |
AbstractDataPluginItem * | findItem (const QString &id) const |
bool | isFavoriteItemsOnly () const |
bool | itemExists (const QString &id) const |
QList< AbstractDataPluginItem * > | items (const ViewportParams *viewport, qint32 number=10) |
const MarbleModel * | marbleModel () const |
virtual void | setFavoriteItems (const QStringList &list) |
void | setFavoriteItemsOnly (bool favoriteOnly) |
void | setItemSettings (const QHash< QString, QVariant > &itemSettings) |
QList< AbstractDataPluginItem * > | whichItemAt (const QPoint &curpos) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
void | downloadDescriptionFile (const QUrl &url) |
void | downloadItem (const QUrl &url, const QString &type, AbstractDataPluginItem *item) |
virtual void | getAdditionalItems (const GeoDataLatLonAltBox &box, qint32 number=10)=0 |
virtual void | getItem (const QString &id) |
virtual void | parseFile (const QByteArray &file) |
void | registerItemProperties (const QMetaObject &item) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
An abstract data model (not based on QAbstractModel) for a AbstractDataPlugin.
This class is an abstract model for a AbstractDataPlugin. It provides the storage and selection of added items and it is also responsible for downloading item data.
The functions getAdditionalItems() and parseFile() have to be reimplemented in a subclass.
Definition at line 39 of file AbstractDataPluginModel.h.
Property Documentation
◆ favoritesModel
|
read |
Definition at line 44 of file AbstractDataPluginModel.h.
Constructor & Destructor Documentation
◆ AbstractDataPluginModel()
|
explicit |
Definition at line 242 of file AbstractDataPluginModel.cpp.
◆ ~AbstractDataPluginModel()
|
override |
Definition at line 258 of file AbstractDataPluginModel.cpp.
Member Function Documentation
◆ addItemsToList
|
slot |
Adds the items
to the list of initialized items.
It checks if items with the same id are already in the list and ignores and deletes them in this case.
Definition at line 390 of file AbstractDataPluginModel.cpp.
◆ addItemToList
|
slot |
Convenience method to add one item to the list.
See addItemsToList
Definition at line 385 of file AbstractDataPluginModel.cpp.
◆ clear
|
slot |
Removes all items.
Definition at line 619 of file AbstractDataPluginModel.cpp.
◆ downloadDescriptionFile()
|
protected |
Download the description file from the url
.
Definition at line 374 of file AbstractDataPluginModel.cpp.
◆ downloadItem()
|
protected |
Downloads the file from url
.
item
-> addDownloadedFile() will be called when the download is finished.
- Parameters
-
url the file URL type The type of the download (to be specified by the subclasser) item the data plugin item
Definition at line 362 of file AbstractDataPluginModel.cpp.
◆ favoriteItems()
QStringList Marble::AbstractDataPluginModel::favoriteItems | ( | ) | const |
Definition at line 456 of file AbstractDataPluginModel.cpp.
◆ findItem()
AbstractDataPluginItem * Marble::AbstractDataPluginModel::findItem | ( | const QString & | id | ) | const |
Finds the item with id
in the list.
- Returns
- The pointer to the item or (if no item has been found) 0
Definition at line 520 of file AbstractDataPluginModel.cpp.
◆ getAdditionalItems()
|
protectedpure virtual |
Managing to get number
additional items in box
.
This includes generating a url and downloading the corresponding file. This method has to be implemented in a subclass.
◆ getItem()
|
protectedvirtual |
Retrieve data for a specific item.
- Parameters
-
id Item id of the item to retrieve
Definition at line 439 of file AbstractDataPluginModel.cpp.
◆ isFavoriteItemsOnly()
bool Marble::AbstractDataPluginModel::isFavoriteItemsOnly | ( | ) | const |
Definition at line 470 of file AbstractDataPluginModel.cpp.
◆ itemExists()
bool Marble::AbstractDataPluginModel::itemExists | ( | const QString & | id | ) | const |
Testing the existence of the item id
in the list.
Definition at line 531 of file AbstractDataPluginModel.cpp.
◆ items()
QList< AbstractDataPluginItem * > Marble::AbstractDataPluginModel::items | ( | const ViewportParams * | viewport, |
qint32 | number = 10 ) |
Get the items on the viewport Returns the currently downloaded images in the viewport
.
The maximum number of images can be specified with number
, 0 means no limit.
- Returns
- The list of item with most important item first.
Definition at line 268 of file AbstractDataPluginModel.cpp.
◆ marbleModel()
const MarbleModel * Marble::AbstractDataPluginModel::marbleModel | ( | ) | const |
Definition at line 263 of file AbstractDataPluginModel.cpp.
◆ parseFile()
|
protectedvirtual |
Parse the file
and generate items.
The items will be added to the list or the method starts additionally needed downloads. This method has to be implemented in a subclass.
Definition at line 357 of file AbstractDataPluginModel.cpp.
◆ registerItemProperties()
|
protected |
Definition at line 634 of file AbstractDataPluginModel.cpp.
◆ setFavoriteItems()
|
virtual |
Definition at line 444 of file AbstractDataPluginModel.cpp.
◆ setFavoriteItemsOnly()
void Marble::AbstractDataPluginModel::setFavoriteItemsOnly | ( | bool | favoriteOnly | ) |
Definition at line 461 of file AbstractDataPluginModel.cpp.
◆ setItemSettings()
void Marble::AbstractDataPluginModel::setItemSettings | ( | const QHash< QString, QVariant > & | itemSettings | ) |
Sets the settings for all items.
Sets the settings for all items before painting. This ensures that all items react on changed settings.
Definition at line 536 of file AbstractDataPluginModel.cpp.
◆ whichItemAt()
QList< AbstractDataPluginItem * > Marble::AbstractDataPluginModel::whichItemAt | ( | const QPoint & | curpos | ) |
Get all items that contain the given point Returns a list of all items that contain the point curpos
.
Definition at line 343 of file AbstractDataPluginModel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.