Marble::AzimuthalProjection
#include <AzimuthalProjection.h>
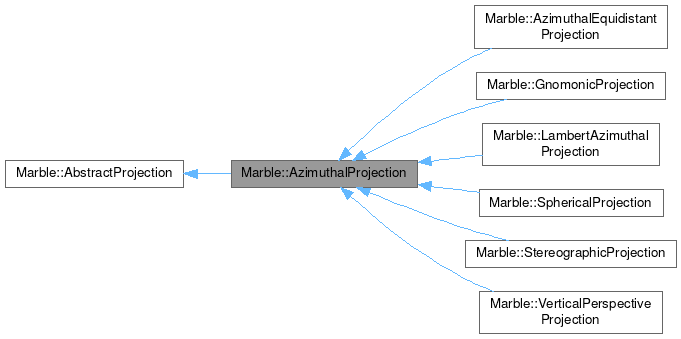
Public Member Functions | |
qreal | clippingRadius () const override |
bool | isClippedToSphere () const override |
GeoDataLatLonAltBox | latLonAltBox (const QRect &screenRect, const ViewportParams *viewport) const override |
bool | mapCoversViewport (const ViewportParams *viewport) const override |
QPainterPath | mapShape (const ViewportParams *viewport) const override |
PreservationType | preservationType () const override |
bool | repeatableX () const override |
virtual bool | screenCoordinates (const GeoDataCoordinates &coordinates, const ViewportParams *viewport, qreal *x, qreal &y, int &pointRepeatNum, const QSizeF &size, bool &globeHidesPoint) const=0 |
bool | screenCoordinates (const GeoDataCoordinates &geopoint, const ViewportParams *viewport, qreal &x, qreal &y) const |
virtual bool | screenCoordinates (const GeoDataCoordinates &geopoint, const ViewportParams *viewport, qreal &x, qreal &y, bool &globeHidesPoint) const=0 |
bool | screenCoordinates (const GeoDataLineString &lineString, const ViewportParams *viewport, QList< QPolygonF * > &polygons) const override |
bool | screenCoordinates (const qreal lon, const qreal lat, const ViewportParams *viewport, qreal &x, qreal &y) const |
SurfaceType | surfaceType () const override |
bool | traversableDateLine () const override |
bool | traversablePoles () const override |
![]() | |
AbstractProjection () | |
virtual QString | description () const =0 |
virtual bool | geoCoordinates (const int x, const int y, const ViewportParams *viewport, qreal &lon, qreal &lat, GeoDataCoordinates::Unit unit=GeoDataCoordinates::Degree) const =0 |
virtual QIcon | icon () const =0 |
virtual bool | isOrientedNormal () const |
QRegion | mapRegion (const ViewportParams *viewport) const |
qreal | maxLat () const |
virtual qreal | maxValidLat () const |
qreal | minLat () const |
virtual qreal | minValidLat () const |
virtual QString | name () const =0 |
bool | screenCoordinates (const GeoDataCoordinates &geopoint, const ViewportParams *viewport, qreal &x, qreal &y) const |
bool | screenCoordinates (const qreal lon, const qreal lat, const ViewportParams *viewport, qreal &x, qreal &y) const |
void | setMaxLat (qreal maxLat) |
void | setMinLat (qreal minLat) |
Protected Member Functions | |
AzimuthalProjection (AzimuthalProjectionPrivate *dd) | |
![]() | |
AbstractProjection (AbstractProjectionPrivate *dd) | |
Additional Inherited Members | |
![]() | |
enum | PreservationType { NoPreservation , Conformal , EqualArea } |
enum | SurfaceType { Cylindrical , Pseudocylindrical , Hybrid , Conical , Pseudoconical , Azimuthal } |
![]() | |
const QScopedPointer< AbstractProjectionPrivate > | d_ptr |
Detailed Description
A base class for the Gnomonic and Orthographic (Globe) projections in Marble.
Definition at line 25 of file AzimuthalProjection.h.
Constructor & Destructor Documentation
◆ AzimuthalProjection()
|
explicitprotected |
Definition at line 148 of file AzimuthalProjection.cpp.
Member Function Documentation
◆ clippingRadius()
|
overridevirtual |
Reimplemented from Marble::AbstractProjection.
Definition at line 28 of file AzimuthalProjection.cpp.
◆ isClippedToSphere()
|
overridevirtual |
Defines whether a projection is supposed to be clipped to a certain radius.
Example: The Gnomonic projection is clipped to a circle of a certain clipping radius (although it's mathematically defined beyond that radius).
Reimplemented from Marble::AbstractProjection.
Definition at line 23 of file AzimuthalProjection.cpp.
◆ latLonAltBox()
|
overridevirtual |
Returns a GeoDataLatLonAltBox bounding box of the given screenrect inside the given viewport.
Reimplemented from Marble::AbstractProjection.
Definition at line 66 of file AzimuthalProjection.cpp.
◆ mapCoversViewport()
|
overridevirtual |
Returns whether the projected data fully obstructs the current viewport.
In this case there are no black areas visible around the actual map. This case allows for performance optimizations.
Implements Marble::AbstractProjection.
Definition at line 47 of file AzimuthalProjection.cpp.
◆ mapShape()
|
overridevirtual |
Returns the shape/outline of a map projection.
This call allows e.g. to draw the default background color of the map itself.
Example: For an azimuthal projection a circle is returned at low zoom values.
Implements Marble::AbstractProjection.
Definition at line 126 of file AzimuthalProjection.cpp.
◆ preservationType()
|
inlineoverridevirtual |
Reimplemented from Marble::AbstractProjection.
Definition at line 52 of file AzimuthalProjection.h.
◆ repeatableX()
|
inlineoverridevirtual |
Returns whether the projection allows for wrapping in x direction (along the longitude scale).
Example: Cylindrical projections allow for repeating.
Reimplemented from Marble::AbstractProjection.
Definition at line 33 of file AzimuthalProjection.h.
◆ screenCoordinates() [1/5]
|
virtual |
Get the coordinates of screen points for geographical coordinates in the map.
- Parameters
-
coordinates the point on earth, including altitude, that we want the coordinates for. viewport the viewport parameters x the x coordinates of the pixels are returned through this parameter y the y coordinate of the pixel is returned through this parameter pointRepeatNum the amount of times that a single geographical point gets represented on the map size the size globeHidesPoint whether the point gets hidden on the far side of the earth
- Returns
true
if the geographical coordinates are visible on the screenfalse
if the geographical coordinates are not visible on the screen
- See also
- ViewportParams
Implements Marble::AbstractProjection.
Reimplemented in Marble::GnomonicProjection, Marble::LambertAzimuthalProjection, Marble::SphericalProjection, Marble::StereographicProjection, and Marble::VerticalPerspectiveProjection.
◆ screenCoordinates() [2/5]
Definition at line 187 of file AbstractProjection.cpp.
◆ screenCoordinates() [3/5]
|
virtual |
Get the screen coordinates corresponding to geographical coordinates in the map.
- Parameters
-
geopoint the point on earth, including altitude, that we want the coordinates for. viewport the viewport parameters x the x coordinate of the pixel is returned through this parameter y the y coordinate of the pixel is returned through this parameter globeHidesPoint whether the point gets hidden on the far side of the earth
- Returns
true
if the geographical coordinates are visible on the screenfalse
if the geographical coordinates are not visible on the screen
- See also
- ViewportParams
Implements Marble::AbstractProjection.
Reimplemented in Marble::GnomonicProjection, Marble::LambertAzimuthalProjection, Marble::SphericalProjection, Marble::StereographicProjection, and Marble::VerticalPerspectiveProjection.
◆ screenCoordinates() [4/5]
|
overridevirtual |
Implements Marble::AbstractProjection.
Definition at line 33 of file AzimuthalProjection.cpp.
◆ screenCoordinates() [5/5]
Get the screen coordinates corresponding to geographical coordinates in the map.
- Parameters
-
lon the lon coordinate of the requested pixel position in radians lat the lat coordinate of the requested pixel position in radians viewport the viewport parameters x the x coordinate of the pixel is returned through this parameter y the y coordinate of the pixel is returned through this parameter
- Returns
true
if the geographical coordinates are visible on the screenfalse
if the geographical coordinates are not visible on the screen
- See also
- ViewportParams
Definition at line 168 of file AbstractProjection.cpp.
◆ surfaceType()
|
inlineoverridevirtual |
Implements Marble::AbstractProjection.
Definition at line 47 of file AzimuthalProjection.h.
◆ traversableDateLine()
|
inlineoverridevirtual |
Reimplemented from Marble::AbstractProjection.
Definition at line 42 of file AzimuthalProjection.h.
◆ traversablePoles()
|
inlineoverridevirtual |
Returns whether the projection allows to navigate seamlessly "over" the pole.
Example: Azimuthal projections.
Reimplemented from Marble::AbstractProjection.
Definition at line 38 of file AzimuthalProjection.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:48:22 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.