Marble::StereographicProjection
#include <StereographicProjection.h>
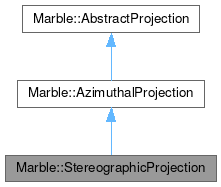
Public Member Functions | |
StereographicProjection () | |
qreal | clippingRadius () const override |
QString | description () const override |
bool | geoCoordinates (const int x, const int y, const ViewportParams *params, qreal &lon, qreal &lat, GeoDataCoordinates::Unit unit=GeoDataCoordinates::Degree) const override |
QIcon | icon () const override |
QString | name () const override |
bool | screenCoordinates (const GeoDataCoordinates &coordinates, const ViewportParams *params, qreal &x, qreal &y, bool &globeHidesPoint) const override |
bool | screenCoordinates (const GeoDataCoordinates &coordinates, const ViewportParams *viewport, qreal *x, qreal &y, int &pointRepeatNum, const QSizeF &size, bool &globeHidesPoint) const override |
bool | screenCoordinates (const GeoDataCoordinates &geopoint, const ViewportParams *viewport, qreal &x, qreal &y) const |
virtual bool | screenCoordinates (const GeoDataLineString &lineString, const ViewportParams *viewport, QList< QPolygonF * > &polygons) const=0 |
bool | screenCoordinates (const qreal lon, const qreal lat, const ViewportParams *viewport, qreal &x, qreal &y) const |
![]() | |
bool | isClippedToSphere () const override |
GeoDataLatLonAltBox | latLonAltBox (const QRect &screenRect, const ViewportParams *viewport) const override |
bool | mapCoversViewport (const ViewportParams *viewport) const override |
QPainterPath | mapShape (const ViewportParams *viewport) const override |
PreservationType | preservationType () const override |
bool | repeatableX () const override |
bool | screenCoordinates (const GeoDataCoordinates &geopoint, const ViewportParams *viewport, qreal &x, qreal &y) const |
bool | screenCoordinates (const qreal lon, const qreal lat, const ViewportParams *viewport, qreal &x, qreal &y) const |
SurfaceType | surfaceType () const override |
bool | traversableDateLine () const override |
bool | traversablePoles () const override |
![]() | |
AbstractProjection () | |
virtual bool | isOrientedNormal () const |
QRegion | mapRegion (const ViewportParams *viewport) const |
qreal | maxLat () const |
virtual qreal | maxValidLat () const |
qreal | minLat () const |
virtual qreal | minValidLat () const |
bool | screenCoordinates (const GeoDataCoordinates &geopoint, const ViewportParams *viewport, qreal &x, qreal &y) const |
bool | screenCoordinates (const qreal lon, const qreal lat, const ViewportParams *viewport, qreal &x, qreal &y) const |
void | setMaxLat (qreal maxLat) |
void | setMinLat (qreal minLat) |
Protected Member Functions | |
StereographicProjection (StereographicProjectionPrivate *dd) | |
![]() | |
AzimuthalProjection (AzimuthalProjectionPrivate *dd) | |
![]() | |
AbstractProjection (AbstractProjectionPrivate *dd) | |
Additional Inherited Members | |
![]() | |
enum | PreservationType { NoPreservation , Conformal , EqualArea } |
enum | SurfaceType { Cylindrical , Pseudocylindrical , Hybrid , Conical , Pseudoconical , Azimuthal } |
![]() | |
const QScopedPointer< AbstractProjectionPrivate > | d_ptr |
Detailed Description
A class to implement the spherical projection used by the "Globe" view.
Definition at line 21 of file StereographicProjection.h.
Constructor & Destructor Documentation
◆ StereographicProjection() [1/2]
Marble::StereographicProjection::StereographicProjection | ( | ) |
Construct a new StereographicProjection.
Definition at line 32 of file StereographicProjection.cpp.
◆ StereographicProjection() [2/2]
|
explicitprotected |
Definition at line 39 of file StereographicProjection.cpp.
Member Function Documentation
◆ clippingRadius()
|
overridevirtual |
Reimplemented from Marble::AzimuthalProjection.
Definition at line 69 of file StereographicProjection.cpp.
◆ description()
|
overridevirtual |
Returns a short user description of the projection that can be used in tooltips or dialogs.
Implements Marble::AbstractProjection.
Definition at line 58 of file StereographicProjection.cpp.
◆ geoCoordinates()
|
overridevirtual |
Get the earth coordinates corresponding to a pixel in the map.
- Parameters
-
x the x coordinate of the pixel y the y coordinate of the pixel params the viewport parameters lon the longitude angle is returned through this parameter lat the latitude angle is returned through this parameter unit the unit
- Returns
true
if the pixel (x, y) is within the globefalse
if the pixel (x, y) is outside the globe, i.e. in space.
Implements Marble::AbstractProjection.
Definition at line 142 of file StereographicProjection.cpp.
◆ icon()
|
overridevirtual |
Returns an icon for the projection.
Implements Marble::AbstractProjection.
Definition at line 64 of file StereographicProjection.cpp.
◆ name()
|
overridevirtual |
Returns the user-visible name of the projection.
Implements Marble::AbstractProjection.
Definition at line 53 of file StereographicProjection.cpp.
◆ screenCoordinates() [1/5]
|
overridevirtual |
Get the screen coordinates corresponding to geographical coordinates in the map.
- Parameters
-
coordinates the coordinates of the requested pixel position params the viewport parameters x the x coordinate of the pixel is returned through this parameter y the y coordinate of the pixel is returned through this parameter globeHidesPoint whether the globe hides the point
- Returns
true
if the geographical coordinates are visible on the screenfalse
if the geographical coordinates are not visible on the screen
Reimplemented from Marble::AzimuthalProjection.
Definition at line 74 of file StereographicProjection.cpp.
◆ screenCoordinates() [2/5]
|
overridevirtual |
Get the coordinates of screen points for geographical coordinates in the map.
- Parameters
-
coordinates the point on earth, including altitude, that we want the coordinates for. viewport the viewport parameters x the x coordinates of the pixels are returned through this parameter y the y coordinate of the pixel is returned through this parameter pointRepeatNum the amount of times that a single geographical point gets represented on the map size the size globeHidesPoint whether the point gets hidden on the far side of the earth
- Returns
true
if the geographical coordinates are visible on the screenfalse
if the geographical coordinates are not visible on the screen
- See also
- ViewportParams
Reimplemented from Marble::AzimuthalProjection.
Definition at line 117 of file StereographicProjection.cpp.
◆ screenCoordinates() [3/5]
bool AbstractProjection::screenCoordinates | ( | const GeoDataCoordinates & | geopoint, |
const ViewportParams * | viewport, | ||
qreal & | x, | ||
qreal & | y ) const |
Definition at line 187 of file AbstractProjection.cpp.
◆ screenCoordinates() [4/5]
|
virtual |
Reimplemented from Marble::AzimuthalProjection.
◆ screenCoordinates() [5/5]
bool AbstractProjection::screenCoordinates | ( | const qreal | lon, |
const qreal | lat, | ||
const ViewportParams * | viewport, | ||
qreal & | x, | ||
qreal & | y ) const |
Get the screen coordinates corresponding to geographical coordinates in the map.
- Parameters
-
lon the lon coordinate of the requested pixel position in radians lat the lat coordinate of the requested pixel position in radians viewport the viewport parameters x the x coordinate of the pixel is returned through this parameter y the y coordinate of the pixel is returned through this parameter
- Returns
true
if the geographical coordinates are visible on the screenfalse
if the geographical coordinates are not visible on the screen
- See also
- ViewportParams
Definition at line 168 of file AbstractProjection.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.