Marble::GeoDataMultiGeometry
#include <GeoDataMultiGeometry.h>
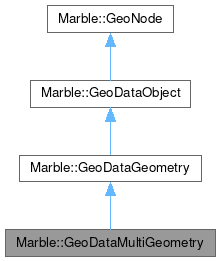
Additional Inherited Members | |
![]() | |
GeoDataGeometry (const GeoDataGeometry &other) | |
GeoDataGeometry (GeoDataGeometryPrivate *priv) | |
bool | equals (const GeoDataGeometry &other) const |
virtual bool | equals (const GeoDataObject &other) const |
GeoDataGeometry & | operator= (const GeoDataGeometry &other) |
![]() | |
GeoDataGeometryPrivate * | d_ptr |
Detailed Description
A class that can contain other GeoDataGeometry objects.
GeoDataMultiGeometry is a collection of other GeoDataGeometry objects. As one can add GeoDataMultiGeometry to itself, you can make up a collection of different objects to form one Placemark.
Definition at line 26 of file GeoDataMultiGeometry.h.
Constructor & Destructor Documentation
◆ GeoDataMultiGeometry() [1/2]
Marble::GeoDataMultiGeometry::GeoDataMultiGeometry | ( | ) |
Definition at line 22 of file GeoDataMultiGeometry.cpp.
◆ GeoDataMultiGeometry() [2/2]
|
explicit |
Definition at line 27 of file GeoDataMultiGeometry.cpp.
Member Function Documentation
◆ append()
void Marble::GeoDataMultiGeometry::append | ( | GeoDataGeometry * | other | ) |
add an element
Definition at line 219 of file GeoDataMultiGeometry.cpp.
◆ at() [1/2]
GeoDataGeometry & Marble::GeoDataMultiGeometry::at | ( | int | pos | ) |
Definition at line 100 of file GeoDataMultiGeometry.cpp.
◆ at() [2/2]
const GeoDataGeometry & Marble::GeoDataMultiGeometry::at | ( | int | pos | ) | const |
Definition at line 109 of file GeoDataMultiGeometry.cpp.
◆ begin()
QList< GeoDataGeometry * >::Iterator Marble::GeoDataMultiGeometry::begin | ( | ) |
Definition at line 157 of file GeoDataMultiGeometry.cpp.
◆ child() [1/2]
GeoDataGeometry * Marble::GeoDataMultiGeometry::child | ( | int | i | ) |
returns the requested child item
Definition at line 188 of file GeoDataMultiGeometry.cpp.
◆ child() [2/2]
const GeoDataGeometry * Marble::GeoDataMultiGeometry::child | ( | int | i | ) | const |
returns the requested child item
Definition at line 196 of file GeoDataMultiGeometry.cpp.
◆ childPosition()
int Marble::GeoDataMultiGeometry::childPosition | ( | const GeoDataGeometry * | child | ) | const |
returns the position of an item in the list
Definition at line 205 of file GeoDataMultiGeometry.cpp.
◆ clear()
void Marble::GeoDataMultiGeometry::clear | ( | ) |
Definition at line 239 of file GeoDataMultiGeometry.cpp.
◆ constBegin()
QList< GeoDataGeometry * >::ConstIterator Marble::GeoDataMultiGeometry::constBegin | ( | ) | const |
Definition at line 173 of file GeoDataMultiGeometry.cpp.
◆ constEnd()
QList< GeoDataGeometry * >::ConstIterator Marble::GeoDataMultiGeometry::constEnd | ( | ) | const |
Definition at line 179 of file GeoDataMultiGeometry.cpp.
◆ copy()
|
overridevirtual |
Implements Marble::GeoDataGeometry.
Definition at line 44 of file GeoDataMultiGeometry.cpp.
◆ end()
QList< GeoDataGeometry * >::Iterator Marble::GeoDataMultiGeometry::end | ( | ) |
Definition at line 165 of file GeoDataMultiGeometry.cpp.
◆ first() [1/2]
GeoDataGeometry & Marble::GeoDataMultiGeometry::first | ( | ) |
Definition at line 137 of file GeoDataMultiGeometry.cpp.
◆ first() [2/2]
const GeoDataGeometry & Marble::GeoDataMultiGeometry::first | ( | ) | const |
Definition at line 151 of file GeoDataMultiGeometry.cpp.
◆ geometryId()
|
overridevirtual |
Implements Marble::GeoDataGeometry.
Definition at line 39 of file GeoDataMultiGeometry.cpp.
◆ last() [1/2]
GeoDataGeometry & Marble::GeoDataMultiGeometry::last | ( | ) |
Definition at line 129 of file GeoDataMultiGeometry.cpp.
◆ last() [2/2]
const GeoDataGeometry & Marble::GeoDataMultiGeometry::last | ( | ) | const |
Definition at line 145 of file GeoDataMultiGeometry.cpp.
◆ latLonAltBox()
|
overridevirtual |
Reimplemented from Marble::GeoDataGeometry.
Definition at line 67 of file GeoDataMultiGeometry.cpp.
◆ nodeType()
|
overridevirtual |
Provides type information for downcasting a GeoNode.
Implements Marble::GeoNode.
Definition at line 34 of file GeoDataMultiGeometry.cpp.
◆ operator!=()
|
inline |
Definition at line 41 of file GeoDataMultiGeometry.h.
◆ operator<<()
GeoDataMultiGeometry & Marble::GeoDataMultiGeometry::operator<< | ( | const GeoDataGeometry & | value | ) |
Definition at line 228 of file GeoDataMultiGeometry.cpp.
◆ operator==()
bool Marble::GeoDataMultiGeometry::operator== | ( | const GeoDataMultiGeometry & | other | ) | const |
Definition at line 49 of file GeoDataMultiGeometry.cpp.
◆ operator[]() [1/2]
GeoDataGeometry & Marble::GeoDataMultiGeometry::operator[] | ( | int | pos | ) |
Definition at line 115 of file GeoDataMultiGeometry.cpp.
◆ operator[]() [2/2]
const GeoDataGeometry & Marble::GeoDataMultiGeometry::operator[] | ( | int | pos | ) | const |
Definition at line 123 of file GeoDataMultiGeometry.cpp.
◆ pack()
|
override |
Definition at line 248 of file GeoDataMultiGeometry.cpp.
◆ size()
int Marble::GeoDataMultiGeometry::size | ( | ) | const |
Definition at line 87 of file GeoDataMultiGeometry.cpp.
◆ unpack()
|
override |
Definition at line 263 of file GeoDataMultiGeometry.cpp.
◆ vector()
QList< GeoDataGeometry * > Marble::GeoDataMultiGeometry::vector | ( | ) |
Definition at line 93 of file GeoDataMultiGeometry.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.