Marble::GeoDataPoint
#include <GeoDataPoint.h>
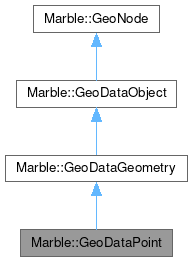
Public Types | |
using | Notation = GeoDataCoordinates::Notation |
using | Unit = GeoDataCoordinates::Unit |
using | Vector = QList<GeoDataPoint> |
Public Member Functions | |
GeoDataPoint (const GeoDataCoordinates &other) | |
GeoDataPoint (const GeoDataPoint &other) | |
GeoDataPoint (qreal lon, qreal lat, qreal alt=0, GeoDataPoint::Unit _unit=GeoDataCoordinates::Radian) | |
const GeoDataCoordinates & | coordinates () const |
GeoDataGeometry * | copy () const override |
virtual void | detach () |
EnumGeometryId | geometryId () const override |
const char * | nodeType () const override |
bool | operator!= (const GeoDataPoint &other) const |
bool | operator== (const GeoDataPoint &other) const |
void | pack (QDataStream &stream) const override |
void | setCoordinates (const GeoDataCoordinates &coordinates) |
void | unpack (QDataStream &stream) override |
![]() | |
AltitudeMode | altitudeMode () const |
void | detach () |
bool | extrude () const |
virtual const GeoDataLatLonAltBox & | latLonAltBox () const |
bool | operator!= (const GeoDataGeometry &other) const |
bool | operator== (const GeoDataGeometry &other) const |
void | pack (QDataStream &stream) const override |
void | setAltitudeMode (const AltitudeMode altitudeMode) |
void | setExtrude (bool extrude) |
void | unpack (QDataStream &stream) override |
![]() | |
GeoDataObject (const GeoDataObject &) | |
QString | id () const |
GeoDataObject & | operator= (const GeoDataObject &) |
void | pack (QDataStream &stream) const override |
GeoDataObject * | parent () |
const GeoDataObject * | parent () const |
QString | resolvePath (const QString &relativePath) const |
void | setId (const QString &value) |
void | setParent (GeoDataObject *parent) |
void | setTargetId (const QString &value) |
QString | targetId () const |
void | unpack (QDataStream &steam) override |
Additional Inherited Members | |
![]() | |
GeoDataGeometry (const GeoDataGeometry &other) | |
GeoDataGeometry (GeoDataGeometryPrivate *priv) | |
bool | equals (const GeoDataGeometry &other) const |
virtual bool | equals (const GeoDataObject &other) const |
GeoDataGeometry & | operator= (const GeoDataGeometry &other) |
![]() | |
GeoDataGeometryPrivate * | d_ptr |
Detailed Description
A Geometry object representing a 3d point.
GeoDataPoint is the GeoDataGeometry class representing a single three dimensional point. It reflects the Point tag of KML spec and can be contained in objects holding GeoDataGeometry objects. Nevertheless GeoDataPoint shouldn't be used if you just want to store 3d coordinates of a point that doesn't need to be inherited from GeoDataGeometry In that case use GeoDataCoordinates instead which has nearly the same features and is much more light weight. Please consider this especially if you expect to have a high amount of points e.g. for line strings, linear rings and polygons.
- See also
- GeoDataCoordinates
- GeoDataGeometry
Definition at line 41 of file GeoDataPoint.h.
Member Typedef Documentation
◆ Notation
Definition at line 44 of file GeoDataPoint.h.
◆ Unit
Definition at line 45 of file GeoDataPoint.h.
◆ Vector
Definition at line 78 of file GeoDataPoint.h.
Constructor & Destructor Documentation
◆ GeoDataPoint() [1/4]
Marble::GeoDataPoint::GeoDataPoint | ( | const GeoDataPoint & | other | ) |
Definition at line 28 of file GeoDataPoint.cpp.
◆ GeoDataPoint() [2/4]
|
explicit |
Definition at line 38 of file GeoDataPoint.cpp.
◆ GeoDataPoint() [3/4]
Marble::GeoDataPoint::GeoDataPoint | ( | ) |
Definition at line 46 of file GeoDataPoint.cpp.
◆ GeoDataPoint() [4/4]
Marble::GeoDataPoint::GeoDataPoint | ( | qreal | lon, |
qreal | lat, | ||
qreal | alt = 0, | ||
GeoDataPoint::Unit | _unit = GeoDataCoordinates::Radian ) |
create a geopoint from longitude and latitude
- Parameters
-
lon longitude lat latitude alt altitude (default: 0) _unit units that lon and lat get measured in (default for Radian: north pole at pi/2, southpole at -pi/2)
Definition at line 20 of file GeoDataPoint.cpp.
◆ ~GeoDataPoint()
|
override |
Definition at line 52 of file GeoDataPoint.cpp.
Member Function Documentation
◆ coordinates()
const GeoDataCoordinates & Marble::GeoDataPoint::coordinates | ( | ) | const |
Definition at line 86 of file GeoDataPoint.cpp.
◆ copy()
|
overridevirtual |
Implements Marble::GeoDataGeometry.
Definition at line 62 of file GeoDataPoint.cpp.
◆ detach()
|
virtual |
Definition at line 97 of file GeoDataPoint.cpp.
◆ geometryId()
|
overridevirtual |
Implements Marble::GeoDataGeometry.
Definition at line 57 of file GeoDataPoint.cpp.
◆ nodeType()
|
overridevirtual |
Provides type information for downcasting a GeoData.
Implements Marble::GeoNode.
Definition at line 92 of file GeoDataPoint.cpp.
◆ operator!=()
bool Marble::GeoDataPoint::operator!= | ( | const GeoDataPoint & | other | ) | const |
Definition at line 72 of file GeoDataPoint.cpp.
◆ operator==()
bool Marble::GeoDataPoint::operator== | ( | const GeoDataPoint & | other | ) | const |
Definition at line 67 of file GeoDataPoint.cpp.
◆ pack()
|
override |
Definition at line 102 of file GeoDataPoint.cpp.
◆ setCoordinates()
void Marble::GeoDataPoint::setCoordinates | ( | const GeoDataCoordinates & | coordinates | ) |
Definition at line 77 of file GeoDataPoint.cpp.
◆ unpack()
|
override |
Definition at line 109 of file GeoDataPoint.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.