Marble::OsmPlacemarkData
#include <OsmPlacemarkData.h>
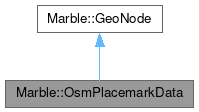
Public Member Functions | |
QString | action () const |
void | addMemberReference (int key, const OsmPlacemarkData &value) |
void | addNodeReference (const GeoDataCoordinates &key, const OsmPlacemarkData &value) |
void | addRelation (qint64 id, OsmType type, const QString &role) |
void | addTag (const QString &key, const QString &value) |
void | changeNodeReference (const GeoDataCoordinates &oldKey, const GeoDataCoordinates &newKey) |
QString | changeset () const |
bool | containsMemberReference (int key) const |
bool | containsNodeReference (const GeoDataCoordinates &key) const |
bool | containsRelation (qint64 id) const |
bool | containsTag (const QString &key, const QString &value) const |
bool | containsTagKey (const QString &key) const |
QHash< QString, QString >::const_iterator | findTag (const QString &key) const |
OsmPlacemarkDataHashRef * | hRef () const |
qint64 | id () const |
bool | isEmpty () const |
bool | isNull () const |
QString | isVisible () const |
OsmPlacemarkData & | memberReference (int key) |
OsmPlacemarkData | memberReference (int key) const |
OsmPlacemarkData & | nodeReference (const GeoDataCoordinates &coordinates) |
OsmPlacemarkData | nodeReference (const GeoDataCoordinates &coordinates) const |
const char * | nodeType () const override |
qint64 | oid () const |
QHash< OsmIdentifier, QString >::const_iterator | relationReferencesBegin () const |
QHash< OsmIdentifier, QString >::const_iterator | relationReferencesEnd () const |
void | removeMemberReference (int key) |
void | removeNodeReference (const GeoDataCoordinates &key) |
void | removeRelation (qint64 id) |
void | removeTag (const QString &key) |
void | setAction (const QString &action) |
void | setChangeset (const QString &changeset) |
void | setId (qint64 id) |
void | setTimestamp (const QString ×tamp) |
void | setUid (const QString &uid) |
void | setUser (const QString &user) |
void | setVersion (const QString &version) |
void | setVisible (const QString &visible) |
QHash< QString, QString >::const_iterator | tagsBegin () const |
QHash< QString, QString >::const_iterator | tagsEnd () const |
QString | tagValue (const QString &key) const |
QString | timestamp () const |
QString | uid () const |
QString | user () const |
QString | version () const |
Static Public Member Functions | |
static OsmPlacemarkData | fromParserAttributes (const QXmlStreamAttributes &attributes) |
Detailed Description
This class is used to encapsulate the osm data fields kept within a placemark's extendedData.
It stores OSM server generated data: id, version, changeset, uid, visible, user, timestamp; It also stores a hash map of <tags> ( key-value mappings ) and a hash map of component osm placemarks
- See also
- m_nodeReferences
- m_memberReferences
The usual workflow with osmData goes as follows:
Parsing stage: The OsmParser parses tags (they have server-generated attributes), creates new placemarks and assigns them new OsmPlacemarkData objects with all the needed information.
Editing stage: While editing placemarks that have OsmPlacemarkData, all relevant changes reflect on the OsmPlacemarkData object as well, so as not to uncorrelate data from the actual placemarks.
Writing stage: The OsmObjectManager assigns OsmPlacemarkData objects to placemarks that do not have it ( these are usually newly created placemarks within the editor, or placemarks loaded from ".kml" files ). Placemarks that already have it, are simply written as-is.
Definition at line 76 of file OsmPlacemarkData.h.
Constructor & Destructor Documentation
◆ OsmPlacemarkData()
Marble::OsmPlacemarkData::OsmPlacemarkData | ( | ) |
Definition at line 22 of file OsmPlacemarkData.cpp.
Member Function Documentation
◆ action()
QString Marble::OsmPlacemarkData::action | ( | ) | const |
Definition at line 77 of file OsmPlacemarkData.cpp.
◆ addMemberReference()
void Marble::OsmPlacemarkData::addMemberReference | ( | int | key, |
const OsmPlacemarkData & | value ) |
addRef this function inserts a int = OsmplacemarkData mapping into the reference hash, equivalent to the osm <nd ref="@p boundary of index @key" > core data element
- See also
- m_memberReferences
Definition at line 204 of file OsmPlacemarkData.cpp.
◆ addNodeReference()
void Marble::OsmPlacemarkData::addNodeReference | ( | const GeoDataCoordinates & | key, |
const OsmPlacemarkData & | value ) |
addRef this function inserts a GeoDataCoordinates = OsmPlacemarkData mapping into the reference hash, equivalent to the <member ref="@p key" > osm core data element
Definition at line 173 of file OsmPlacemarkData.cpp.
◆ addRelation()
addRelation calling this makes the osm placemark a member of the relation with id
as id, while having the role role
Definition at line 232 of file OsmPlacemarkData.cpp.
◆ addTag()
addTag this function inserts a string key=value mapping, equivalent to the <tag k="@p key" v="@p value"> osm core data element
Definition at line 127 of file OsmPlacemarkData.cpp.
◆ changeNodeReference()
void Marble::OsmPlacemarkData::changeNodeReference | ( | const GeoDataCoordinates & | oldKey, |
const GeoDataCoordinates & | newKey ) |
changeNodeReference is a convenience function that allows the quick change of a node hash entry.
This is generally used to update the osm data in case nodes are being moved in the editor.
Definition at line 188 of file OsmPlacemarkData.cpp.
◆ changeset()
QString Marble::OsmPlacemarkData::changeset | ( | ) | const |
Definition at line 47 of file OsmPlacemarkData.cpp.
◆ containsMemberReference()
bool Marble::OsmPlacemarkData::containsMemberReference | ( | int | key | ) | const |
Definition at line 227 of file OsmPlacemarkData.cpp.
◆ containsNodeReference()
bool Marble::OsmPlacemarkData::containsNodeReference | ( | const GeoDataCoordinates & | key | ) | const |
Definition at line 183 of file OsmPlacemarkData.cpp.
◆ containsRelation()
bool Marble::OsmPlacemarkData::containsRelation | ( | qint64 | id | ) | const |
this is wrong and just done this way for backward behavior compatible
this method should probably take type as an additional argument
Definition at line 246 of file OsmPlacemarkData.cpp.
◆ containsTag()
containsTag returns true if the tag hash contains an entry with the key
as key and value
as value
Definition at line 137 of file OsmPlacemarkData.cpp.
◆ containsTagKey()
bool Marble::OsmPlacemarkData::containsTagKey | ( | const QString & | key | ) | const |
containsTagKey returns true if the tag hash contains an entry with the key
as key
Definition at line 143 of file OsmPlacemarkData.cpp.
◆ findTag()
QHash< QString, QString >::const_iterator Marble::OsmPlacemarkData::findTag | ( | const QString & | key | ) | const |
tagValue returns a pointer to the tag that has key
as key or the end iterator if there is no such tag
Definition at line 148 of file OsmPlacemarkData.cpp.
◆ fromParserAttributes()
|
static |
fromParserAttributes is a convenience function that parses all osm-related arguments of a tag
- Returns
- an OsmPlacemarkData object containing all the necessary data
Definition at line 274 of file OsmPlacemarkData.cpp.
◆ hRef()
OsmPlacemarkDataHashRef * Marble::OsmPlacemarkData::hRef | ( | ) | const |
Return the insternal instance of the hash-table functions container.
Definition at line 31 of file OsmPlacemarkData.cpp.
◆ id()
qint64 Marble::OsmPlacemarkData::id | ( | ) | const |
Definition at line 36 of file OsmPlacemarkData.cpp.
◆ isEmpty()
bool Marble::OsmPlacemarkData::isEmpty | ( | ) | const |
isEmpty returns true if no attribute other than the id has been set
Definition at line 269 of file OsmPlacemarkData.cpp.
◆ isNull()
bool Marble::OsmPlacemarkData::isNull | ( | ) | const |
isNull returns false if the osmData is loaded from a source or true if its just default constructed
Definition at line 264 of file OsmPlacemarkData.cpp.
◆ isVisible()
QString Marble::OsmPlacemarkData::isVisible | ( | ) | const |
Definition at line 62 of file OsmPlacemarkData.cpp.
◆ memberReference() [1/2]
OsmPlacemarkData & Marble::OsmPlacemarkData::memberReference | ( | int | key | ) |
this function returns the osmData associated with a member boundary's index -1 represents the outer boundary of a polygon, and 0,1,2... the inner boundaries, in the order provided by polygon->innerBoundaries();
Definition at line 194 of file OsmPlacemarkData.cpp.
◆ memberReference() [2/2]
OsmPlacemarkData Marble::OsmPlacemarkData::memberReference | ( | int | key | ) | const |
Definition at line 199 of file OsmPlacemarkData.cpp.
◆ nodeReference() [1/2]
OsmPlacemarkData & Marble::OsmPlacemarkData::nodeReference | ( | const GeoDataCoordinates & | coordinates | ) |
this function returns the osmData associated with a nd
Definition at line 163 of file OsmPlacemarkData.cpp.
◆ nodeReference() [2/2]
OsmPlacemarkData Marble::OsmPlacemarkData::nodeReference | ( | const GeoDataCoordinates & | coordinates | ) | const |
Definition at line 168 of file OsmPlacemarkData.cpp.
◆ nodeType()
|
overridevirtual |
Provides type information for downcasting a GeoNode.
Implements Marble::GeoNode.
Definition at line 302 of file OsmPlacemarkData.cpp.
◆ oid()
qint64 Marble::OsmPlacemarkData::oid | ( | ) | const |
Definition at line 41 of file OsmPlacemarkData.cpp.
◆ relationReferencesBegin()
QHash< OsmIdentifier, QString >::const_iterator Marble::OsmPlacemarkData::relationReferencesBegin | ( | ) | const |
Definition at line 254 of file OsmPlacemarkData.cpp.
◆ relationReferencesEnd()
QHash< OsmIdentifier, QString >::const_iterator Marble::OsmPlacemarkData::relationReferencesEnd | ( | ) | const |
Definition at line 259 of file OsmPlacemarkData.cpp.
◆ removeMemberReference()
void Marble::OsmPlacemarkData::removeMemberReference | ( | int | key | ) |
Definition at line 209 of file OsmPlacemarkData.cpp.
◆ removeNodeReference()
void Marble::OsmPlacemarkData::removeNodeReference | ( | const GeoDataCoordinates & | key | ) |
Definition at line 178 of file OsmPlacemarkData.cpp.
◆ removeRelation()
void Marble::OsmPlacemarkData::removeRelation | ( | qint64 | id | ) |
this is wrong and just done this way for backward behavior compatible
this method should probably take type as an additional argument
Definition at line 237 of file OsmPlacemarkData.cpp.
◆ removeTag()
void Marble::OsmPlacemarkData::removeTag | ( | const QString & | key | ) |
removeTag removes the tag from the tag hash
Definition at line 132 of file OsmPlacemarkData.cpp.
◆ setAction()
void Marble::OsmPlacemarkData::setAction | ( | const QString & | action | ) |
Definition at line 117 of file OsmPlacemarkData.cpp.
◆ setChangeset()
void Marble::OsmPlacemarkData::setChangeset | ( | const QString & | changeset | ) |
Definition at line 92 of file OsmPlacemarkData.cpp.
◆ setId()
void Marble::OsmPlacemarkData::setId | ( | qint64 | id | ) |
Definition at line 82 of file OsmPlacemarkData.cpp.
◆ setTimestamp()
void Marble::OsmPlacemarkData::setTimestamp | ( | const QString & | timestamp | ) |
Definition at line 112 of file OsmPlacemarkData.cpp.
◆ setUid()
void Marble::OsmPlacemarkData::setUid | ( | const QString & | uid | ) |
Definition at line 97 of file OsmPlacemarkData.cpp.
◆ setUser()
void Marble::OsmPlacemarkData::setUser | ( | const QString & | user | ) |
Definition at line 107 of file OsmPlacemarkData.cpp.
◆ setVersion()
void Marble::OsmPlacemarkData::setVersion | ( | const QString & | version | ) |
Definition at line 87 of file OsmPlacemarkData.cpp.
◆ setVisible()
void Marble::OsmPlacemarkData::setVisible | ( | const QString & | visible | ) |
Definition at line 102 of file OsmPlacemarkData.cpp.
◆ tagsBegin()
iterators for the tags hash.
Definition at line 153 of file OsmPlacemarkData.cpp.
◆ tagsEnd()
Definition at line 158 of file OsmPlacemarkData.cpp.
◆ tagValue()
tagValue returns the value of the tag that has key
as key or an empty qstring if there is no such tag
Definition at line 122 of file OsmPlacemarkData.cpp.
◆ timestamp()
QString Marble::OsmPlacemarkData::timestamp | ( | ) | const |
Definition at line 72 of file OsmPlacemarkData.cpp.
◆ uid()
QString Marble::OsmPlacemarkData::uid | ( | ) | const |
Definition at line 57 of file OsmPlacemarkData.cpp.
◆ user()
QString Marble::OsmPlacemarkData::user | ( | ) | const |
Definition at line 67 of file OsmPlacemarkData.cpp.
◆ version()
QString Marble::OsmPlacemarkData::version | ( | ) | const |
Definition at line 52 of file OsmPlacemarkData.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:04:12 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.