QCA::Console
#include <QtCrypto>
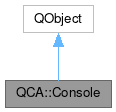
Public Types | |
enum | ChannelMode { Read , ReadWrite } |
enum | TerminalMode { Default , Interactive } |
enum | Type { Tty , Stdio } |
Public Member Functions | |
Console (Type type, ChannelMode cmode, TerminalMode tmode, QObject *parent=nullptr) | |
QByteArray | bytesLeftToRead () |
QByteArray | bytesLeftToWrite () |
ChannelMode | channelMode () const |
void | release () |
TerminalMode | terminalMode () const |
Type | type () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static bool | isStdinRedirected () |
static bool | isStdoutRedirected () |
static Console * | stdioInstance () |
static Console * | ttyInstance () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
QCA Console system
QCA provides an API for asynchronous, event-based access to the console and stdin/stdout, as these facilities are otherwise not portable. The primary use of this system within QCA is for passphrase prompting in command-line applications, using the tty console type.
How it works: Create a Console object for the type of console desired, and then use ConsoleReference to act on the console. Only one ConsoleReference may operate on a Console at a time.
A Console object takes over either the physical console (Console::Tty type) or stdin/stdout (Console::Stdio type). Only one of each type may be created at a time.
Whenever code is written that needs a tty or stdio object, the code should first call one of the static methods (ttyInstance() or stdioInstance()) to see if a console object for the desired type exists already. If the object exists, use it. If it does not exist, the rule is that the relevant code should create the object, use the object, and then destroy the object when the operation is completed.
By following the above rule, you can write code that utilizes a console without the application having to create some master console object for you. Of course, if the application has created a console then it will be used.
The reason why there is a master console object is that it is not guaranteed that all I/O will survive creation and destruction of a console object. If you are using the Stdio Type, then you probably want a long-lived console object. It is possible to capture unprocessed I/O by calling bytesLeftToRead or bytesLeftToWrite. However, it is not expected that general console-needing code will call these functions when utilizing a temporary console. Thus, an application developer would need to create his own console object, invoke the console-needing code, and then do his own extraction of the unprocessed I/O if necessary. Another reason to extract unprocessed I/O is if you need to switch from Console back to standard functions (e.g. fgets() ).
Definition at line 560 of file qca_support.h.
Member Enumeration Documentation
◆ ChannelMode
The type of I/O to use with the console object.
Enumerator | |
---|---|
Read | Read only (equivalent to stdin) |
ReadWrite | Read/write (equivalent to stdin and stdout) |
Definition at line 575 of file qca_support.h.
◆ TerminalMode
The nature of the console operation.
Enumerator | |
---|---|
Default | use default terminal settings |
Interactive | char-by-char input, no echo |
Definition at line 584 of file qca_support.h.
◆ Type
enum QCA::Console::Type |
The type of console object.
Enumerator | |
---|---|
Tty | physical console |
Stdio | stdin/stdout |
Definition at line 567 of file qca_support.h.
Constructor & Destructor Documentation
◆ Console()
QCA::Console::Console | ( | Type | type, |
ChannelMode | cmode, | ||
TerminalMode | tmode, | ||
QObject * | parent = nullptr ) |
Standard constructor.
Note that library code should not create a new Console object without checking whether there is already a Console object of the required Type. See the main documentation for Console for the rationale for this.
- Parameters
-
type the Type of Console object to create cmode the ChannelMode (I/O type) to use tmode the TerminalMode to use parent the parent object for this object
- See also
- ttyInstance() and stdioInstance for static methods that allow you to test whether there is already a Console object of the required Type, and if there is, obtain a reference to that object.
Member Function Documentation
◆ bytesLeftToRead()
QByteArray QCA::Console::bytesLeftToRead | ( | ) |
Obtain remaining data from the Console, awaiting a read operation.
◆ bytesLeftToWrite()
QByteArray QCA::Console::bytesLeftToWrite | ( | ) |
Obtain remaining data from the Console, awaiting a write operation.
◆ channelMode()
ChannelMode QCA::Console::channelMode | ( | ) | const |
The ChannelMode of this Console object.
◆ isStdinRedirected()
|
static |
Test whether standard input is redirected.
- See also
- type() and channelMode()
◆ isStdoutRedirected()
|
static |
Test whether standard output is redirected.
- See also
- type() and channelMode()
◆ release()
void QCA::Console::release | ( | ) |
Release the Console.
This allows access to buffers containing any remaining data
◆ stdioInstance()
|
static |
◆ terminalMode()
TerminalMode QCA::Console::terminalMode | ( | ) | const |
The TerminalMode of this Console object.
◆ ttyInstance()
|
static |
◆ type()
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:48 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.